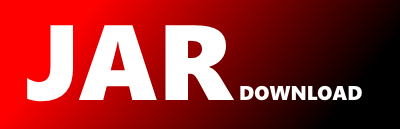
com.azure.resourcemanager.security.implementation.AlertImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-security Show documentation
Show all versions of azure-resourcemanager-security Show documentation
This package contains Microsoft Azure SDK for Security Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. API spec for Microsoft.Security (Azure Security Center) resource provider. Package tag package-composite-v3.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.security.implementation;
import com.azure.resourcemanager.security.fluent.models.AlertInner;
import com.azure.resourcemanager.security.models.Alert;
import com.azure.resourcemanager.security.models.AlertEntity;
import com.azure.resourcemanager.security.models.AlertPropertiesSupportingEvidence;
import com.azure.resourcemanager.security.models.AlertSeverity;
import com.azure.resourcemanager.security.models.AlertStatus;
import com.azure.resourcemanager.security.models.Intent;
import com.azure.resourcemanager.security.models.ResourceIdentifier;
import java.time.OffsetDateTime;
import java.util.Collections;
import java.util.List;
import java.util.Map;
public final class AlertImpl implements Alert {
private AlertInner innerObject;
private final com.azure.resourcemanager.security.SecurityManager serviceManager;
AlertImpl(AlertInner innerObject, com.azure.resourcemanager.security.SecurityManager serviceManager) {
this.innerObject = innerObject;
this.serviceManager = serviceManager;
}
public String id() {
return this.innerModel().id();
}
public String name() {
return this.innerModel().name();
}
public String type() {
return this.innerModel().type();
}
public String version() {
return this.innerModel().version();
}
public String alertType() {
return this.innerModel().alertType();
}
public String systemAlertId() {
return this.innerModel().systemAlertId();
}
public String productComponentName() {
return this.innerModel().productComponentName();
}
public String alertDisplayName() {
return this.innerModel().alertDisplayName();
}
public String description() {
return this.innerModel().description();
}
public AlertSeverity severity() {
return this.innerModel().severity();
}
public Intent intent() {
return this.innerModel().intent();
}
public OffsetDateTime startTimeUtc() {
return this.innerModel().startTimeUtc();
}
public OffsetDateTime endTimeUtc() {
return this.innerModel().endTimeUtc();
}
public List resourceIdentifiers() {
List inner = this.innerModel().resourceIdentifiers();
if (inner != null) {
return Collections.unmodifiableList(inner);
} else {
return Collections.emptyList();
}
}
public List remediationSteps() {
List inner = this.innerModel().remediationSteps();
if (inner != null) {
return Collections.unmodifiableList(inner);
} else {
return Collections.emptyList();
}
}
public String vendorName() {
return this.innerModel().vendorName();
}
public AlertStatus status() {
return this.innerModel().status();
}
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy