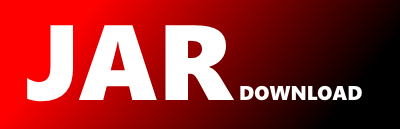
com.azure.resourcemanager.security.implementation.AssessmentsImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-security Show documentation
Show all versions of azure-resourcemanager-security Show documentation
This package contains Microsoft Azure SDK for Security Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. API spec for Microsoft.Security (Azure Security Center) resource provider. Package tag package-composite-v3.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.security.implementation;
import com.azure.core.http.rest.PagedIterable;
import com.azure.core.http.rest.Response;
import com.azure.core.http.rest.SimpleResponse;
import com.azure.core.util.Context;
import com.azure.core.util.logging.ClientLogger;
import com.azure.resourcemanager.security.fluent.AssessmentsClient;
import com.azure.resourcemanager.security.fluent.models.SecurityAssessmentResponseInner;
import com.azure.resourcemanager.security.models.Assessments;
import com.azure.resourcemanager.security.models.ExpandEnum;
import com.azure.resourcemanager.security.models.SecurityAssessmentResponse;
public final class AssessmentsImpl implements Assessments {
private static final ClientLogger LOGGER = new ClientLogger(AssessmentsImpl.class);
private final AssessmentsClient innerClient;
private final com.azure.resourcemanager.security.SecurityManager serviceManager;
public AssessmentsImpl(AssessmentsClient innerClient,
com.azure.resourcemanager.security.SecurityManager serviceManager) {
this.innerClient = innerClient;
this.serviceManager = serviceManager;
}
public PagedIterable list(String scope) {
PagedIterable inner = this.serviceClient().list(scope);
return ResourceManagerUtils.mapPage(inner,
inner1 -> new SecurityAssessmentResponseImpl(inner1, this.manager()));
}
public PagedIterable list(String scope, Context context) {
PagedIterable inner = this.serviceClient().list(scope, context);
return ResourceManagerUtils.mapPage(inner,
inner1 -> new SecurityAssessmentResponseImpl(inner1, this.manager()));
}
public Response getWithResponse(String resourceId, String assessmentName,
ExpandEnum expand, Context context) {
Response inner
= this.serviceClient().getWithResponse(resourceId, assessmentName, expand, context);
if (inner != null) {
return new SimpleResponse<>(inner.getRequest(), inner.getStatusCode(), inner.getHeaders(),
new SecurityAssessmentResponseImpl(inner.getValue(), this.manager()));
} else {
return null;
}
}
public SecurityAssessmentResponse get(String resourceId, String assessmentName) {
SecurityAssessmentResponseInner inner = this.serviceClient().get(resourceId, assessmentName);
if (inner != null) {
return new SecurityAssessmentResponseImpl(inner, this.manager());
} else {
return null;
}
}
public Response deleteByResourceGroupWithResponse(String resourceId, String assessmentName, Context context) {
return this.serviceClient().deleteWithResponse(resourceId, assessmentName, context);
}
public void deleteByResourceGroup(String resourceId, String assessmentName) {
this.serviceClient().delete(resourceId, assessmentName);
}
public SecurityAssessmentResponse getById(String id) {
String resourceId = ResourceManagerUtils.getValueFromIdByParameterName(id,
"/{resourceId}/providers/Microsoft.Security/assessments/{assessmentName}", "resourceId");
if (resourceId == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'resourceId'.", id)));
}
String assessmentName = ResourceManagerUtils.getValueFromIdByParameterName(id,
"/{resourceId}/providers/Microsoft.Security/assessments/{assessmentName}", "assessmentName");
if (assessmentName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'assessments'.", id)));
}
ExpandEnum localExpand = null;
return this.getWithResponse(resourceId, assessmentName, localExpand, Context.NONE).getValue();
}
public Response getByIdWithResponse(String id, ExpandEnum expand, Context context) {
String resourceId = ResourceManagerUtils.getValueFromIdByParameterName(id,
"/{resourceId}/providers/Microsoft.Security/assessments/{assessmentName}", "resourceId");
if (resourceId == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'resourceId'.", id)));
}
String assessmentName = ResourceManagerUtils.getValueFromIdByParameterName(id,
"/{resourceId}/providers/Microsoft.Security/assessments/{assessmentName}", "assessmentName");
if (assessmentName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'assessments'.", id)));
}
return this.getWithResponse(resourceId, assessmentName, expand, context);
}
public void deleteById(String id) {
String resourceId = ResourceManagerUtils.getValueFromIdByParameterName(id,
"/{resourceId}/providers/Microsoft.Security/assessments/{assessmentName}", "resourceId");
if (resourceId == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'resourceId'.", id)));
}
String assessmentName = ResourceManagerUtils.getValueFromIdByParameterName(id,
"/{resourceId}/providers/Microsoft.Security/assessments/{assessmentName}", "assessmentName");
if (assessmentName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'assessments'.", id)));
}
this.deleteByResourceGroupWithResponse(resourceId, assessmentName, Context.NONE);
}
public Response deleteByIdWithResponse(String id, Context context) {
String resourceId = ResourceManagerUtils.getValueFromIdByParameterName(id,
"/{resourceId}/providers/Microsoft.Security/assessments/{assessmentName}", "resourceId");
if (resourceId == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'resourceId'.", id)));
}
String assessmentName = ResourceManagerUtils.getValueFromIdByParameterName(id,
"/{resourceId}/providers/Microsoft.Security/assessments/{assessmentName}", "assessmentName");
if (assessmentName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'assessments'.", id)));
}
return this.deleteByResourceGroupWithResponse(resourceId, assessmentName, context);
}
private AssessmentsClient serviceClient() {
return this.innerClient;
}
private com.azure.resourcemanager.security.SecurityManager manager() {
return this.serviceManager;
}
public SecurityAssessmentResponseImpl define(String name) {
return new SecurityAssessmentResponseImpl(name, this.manager());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy