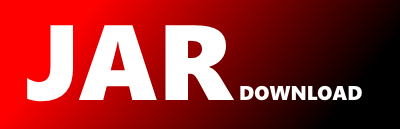
com.azure.resourcemanager.security.models.AutomationTriggeringRule Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-security Show documentation
Show all versions of azure-resourcemanager-security Show documentation
This package contains Microsoft Azure SDK for Security Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. API spec for Microsoft.Security (Azure Security Center) resource provider. Package tag package-composite-v3.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.security.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
/**
* A rule which is evaluated upon event interception. The rule is configured by comparing a specific value from the
* event model to an expected value. This comparison is done by using one of the supported operators set.
*/
@Fluent
public final class AutomationTriggeringRule implements JsonSerializable {
/*
* The JPath of the entity model property that should be checked.
*/
private String propertyJPath;
/*
* The data type of the compared operands (string, integer, floating point number or a boolean [true/false]]
*/
private PropertyType propertyType;
/*
* The expected value.
*/
private String expectedValue;
/*
* A valid comparer operator to use. A case-insensitive comparison will be applied for String PropertyType.
*/
private Operator operator;
/**
* Creates an instance of AutomationTriggeringRule class.
*/
public AutomationTriggeringRule() {
}
/**
* Get the propertyJPath property: The JPath of the entity model property that should be checked.
*
* @return the propertyJPath value.
*/
public String propertyJPath() {
return this.propertyJPath;
}
/**
* Set the propertyJPath property: The JPath of the entity model property that should be checked.
*
* @param propertyJPath the propertyJPath value to set.
* @return the AutomationTriggeringRule object itself.
*/
public AutomationTriggeringRule withPropertyJPath(String propertyJPath) {
this.propertyJPath = propertyJPath;
return this;
}
/**
* Get the propertyType property: The data type of the compared operands (string, integer, floating point number or
* a boolean [true/false]].
*
* @return the propertyType value.
*/
public PropertyType propertyType() {
return this.propertyType;
}
/**
* Set the propertyType property: The data type of the compared operands (string, integer, floating point number or
* a boolean [true/false]].
*
* @param propertyType the propertyType value to set.
* @return the AutomationTriggeringRule object itself.
*/
public AutomationTriggeringRule withPropertyType(PropertyType propertyType) {
this.propertyType = propertyType;
return this;
}
/**
* Get the expectedValue property: The expected value.
*
* @return the expectedValue value.
*/
public String expectedValue() {
return this.expectedValue;
}
/**
* Set the expectedValue property: The expected value.
*
* @param expectedValue the expectedValue value to set.
* @return the AutomationTriggeringRule object itself.
*/
public AutomationTriggeringRule withExpectedValue(String expectedValue) {
this.expectedValue = expectedValue;
return this;
}
/**
* Get the operator property: A valid comparer operator to use. A case-insensitive comparison will be applied for
* String PropertyType.
*
* @return the operator value.
*/
public Operator operator() {
return this.operator;
}
/**
* Set the operator property: A valid comparer operator to use. A case-insensitive comparison will be applied for
* String PropertyType.
*
* @param operator the operator value to set.
* @return the AutomationTriggeringRule object itself.
*/
public AutomationTriggeringRule withOperator(Operator operator) {
this.operator = operator;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("propertyJPath", this.propertyJPath);
jsonWriter.writeStringField("propertyType", this.propertyType == null ? null : this.propertyType.toString());
jsonWriter.writeStringField("expectedValue", this.expectedValue);
jsonWriter.writeStringField("operator", this.operator == null ? null : this.operator.toString());
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of AutomationTriggeringRule from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of AutomationTriggeringRule if the JsonReader was pointing to an instance of it, or null if
* it was pointing to JSON null.
* @throws IOException If an error occurs while reading the AutomationTriggeringRule.
*/
public static AutomationTriggeringRule fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
AutomationTriggeringRule deserializedAutomationTriggeringRule = new AutomationTriggeringRule();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("propertyJPath".equals(fieldName)) {
deserializedAutomationTriggeringRule.propertyJPath = reader.getString();
} else if ("propertyType".equals(fieldName)) {
deserializedAutomationTriggeringRule.propertyType = PropertyType.fromString(reader.getString());
} else if ("expectedValue".equals(fieldName)) {
deserializedAutomationTriggeringRule.expectedValue = reader.getString();
} else if ("operator".equals(fieldName)) {
deserializedAutomationTriggeringRule.operator = Operator.fromString(reader.getString());
} else {
reader.skipChildren();
}
}
return deserializedAutomationTriggeringRule;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy