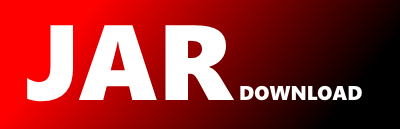
com.azure.resourcemanager.security.models.AwsCredsAuthenticationDetailsProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-security Show documentation
Show all versions of azure-resourcemanager-security Show documentation
This package contains Microsoft Azure SDK for Security Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. API spec for Microsoft.Security (Azure Security Center) resource provider. Package tag package-composite-v3.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.security.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.logging.ClientLogger;
import com.azure.json.JsonReader;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.util.List;
/**
* AWS cloud account connector based credentials, the credentials is composed of access key ID and secret key, for more
* details, refer to <a href="https://docs.aws.amazon.com/IAM/latest/UserGuide/id_users_create.html">Creating an
* IAM User in Your AWS Account (write only)</a>.
*/
@Fluent
public final class AwsCredsAuthenticationDetailsProperties extends AuthenticationDetailsProperties {
/*
* Connect to your cloud account, for AWS use either account credentials or role-based authentication. For GCP use
* account organization credentials.
*/
private AuthenticationType authenticationType = AuthenticationType.AWS_CREDS;
/*
* The ID of the cloud account
*/
private String accountId;
/*
* Public key element of the AWS credential object (write only)
*/
private String awsAccessKeyId;
/*
* Secret key element of the AWS credential object (write only)
*/
private String awsSecretAccessKey;
/**
* Creates an instance of AwsCredsAuthenticationDetailsProperties class.
*/
public AwsCredsAuthenticationDetailsProperties() {
}
/**
* Get the authenticationType property: Connect to your cloud account, for AWS use either account credentials or
* role-based authentication. For GCP use account organization credentials.
*
* @return the authenticationType value.
*/
@Override
public AuthenticationType authenticationType() {
return this.authenticationType;
}
/**
* Get the accountId property: The ID of the cloud account.
*
* @return the accountId value.
*/
public String accountId() {
return this.accountId;
}
/**
* Get the awsAccessKeyId property: Public key element of the AWS credential object (write only).
*
* @return the awsAccessKeyId value.
*/
public String awsAccessKeyId() {
return this.awsAccessKeyId;
}
/**
* Set the awsAccessKeyId property: Public key element of the AWS credential object (write only).
*
* @param awsAccessKeyId the awsAccessKeyId value to set.
* @return the AwsCredsAuthenticationDetailsProperties object itself.
*/
public AwsCredsAuthenticationDetailsProperties withAwsAccessKeyId(String awsAccessKeyId) {
this.awsAccessKeyId = awsAccessKeyId;
return this;
}
/**
* Get the awsSecretAccessKey property: Secret key element of the AWS credential object (write only).
*
* @return the awsSecretAccessKey value.
*/
public String awsSecretAccessKey() {
return this.awsSecretAccessKey;
}
/**
* Set the awsSecretAccessKey property: Secret key element of the AWS credential object (write only).
*
* @param awsSecretAccessKey the awsSecretAccessKey value to set.
* @return the AwsCredsAuthenticationDetailsProperties object itself.
*/
public AwsCredsAuthenticationDetailsProperties withAwsSecretAccessKey(String awsSecretAccessKey) {
this.awsSecretAccessKey = awsSecretAccessKey;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
@Override
public void validate() {
if (awsAccessKeyId() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property awsAccessKeyId in model AwsCredsAuthenticationDetailsProperties"));
}
if (awsSecretAccessKey() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property awsSecretAccessKey in model AwsCredsAuthenticationDetailsProperties"));
}
}
private static final ClientLogger LOGGER = new ClientLogger(AwsCredsAuthenticationDetailsProperties.class);
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("awsAccessKeyId", this.awsAccessKeyId);
jsonWriter.writeStringField("awsSecretAccessKey", this.awsSecretAccessKey);
jsonWriter.writeStringField("authenticationType",
this.authenticationType == null ? null : this.authenticationType.toString());
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of AwsCredsAuthenticationDetailsProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of AwsCredsAuthenticationDetailsProperties if the JsonReader was pointing to an instance of
* it, or null if it was pointing to JSON null.
* @throws IllegalStateException If the deserialized JSON object was missing any required properties.
* @throws IOException If an error occurs while reading the AwsCredsAuthenticationDetailsProperties.
*/
public static AwsCredsAuthenticationDetailsProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
AwsCredsAuthenticationDetailsProperties deserializedAwsCredsAuthenticationDetailsProperties
= new AwsCredsAuthenticationDetailsProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("authenticationProvisioningState".equals(fieldName)) {
deserializedAwsCredsAuthenticationDetailsProperties.withAuthenticationProvisioningState(
AuthenticationProvisioningState.fromString(reader.getString()));
} else if ("grantedPermissions".equals(fieldName)) {
List grantedPermissions
= reader.readArray(reader1 -> PermissionProperty.fromString(reader1.getString()));
deserializedAwsCredsAuthenticationDetailsProperties.withGrantedPermissions(grantedPermissions);
} else if ("awsAccessKeyId".equals(fieldName)) {
deserializedAwsCredsAuthenticationDetailsProperties.awsAccessKeyId = reader.getString();
} else if ("awsSecretAccessKey".equals(fieldName)) {
deserializedAwsCredsAuthenticationDetailsProperties.awsSecretAccessKey = reader.getString();
} else if ("authenticationType".equals(fieldName)) {
deserializedAwsCredsAuthenticationDetailsProperties.authenticationType
= AuthenticationType.fromString(reader.getString());
} else if ("accountId".equals(fieldName)) {
deserializedAwsCredsAuthenticationDetailsProperties.accountId = reader.getString();
} else {
reader.skipChildren();
}
}
return deserializedAwsCredsAuthenticationDetailsProperties;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy