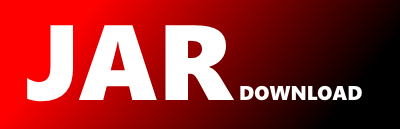
com.azure.resourcemanager.security.models.DefenderForContainersGcpOffering Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-security Show documentation
Show all versions of azure-resourcemanager-security Show documentation
This package contains Microsoft Azure SDK for Security Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. API spec for Microsoft.Security (Azure Security Center) resource provider. Package tag package-composite-v3.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.security.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
/**
* The containers GCP offering.
*/
@Fluent
public final class DefenderForContainersGcpOffering extends CloudOffering {
/*
* The type of the security offering.
*/
private OfferingType offeringType = OfferingType.DEFENDER_FOR_CONTAINERS_GCP;
/*
* The native cloud connection configuration
*/
private DefenderForContainersGcpOfferingNativeCloudConnection nativeCloudConnection;
/*
* The native cloud connection configuration
*/
private DefenderForContainersGcpOfferingDataPipelineNativeCloudConnection dataPipelineNativeCloudConnection;
/*
* Is audit logs data collection enabled
*/
private Boolean enableAuditLogsAutoProvisioning;
/*
* Is Microsoft Defender for Cloud Kubernetes agent auto provisioning enabled
*/
private Boolean enableDefenderAgentAutoProvisioning;
/*
* Is Policy Kubernetes agent auto provisioning enabled
*/
private Boolean enablePolicyAgentAutoProvisioning;
/*
* The Microsoft Defender Container image assessment configuration
*/
private DefenderForContainersGcpOfferingMdcContainersImageAssessment mdcContainersImageAssessment;
/*
* The Microsoft Defender Container agentless discovery configuration
*/
private DefenderForContainersGcpOfferingMdcContainersAgentlessDiscoveryK8S mdcContainersAgentlessDiscoveryK8S;
/*
* The Microsoft Defender for Container K8s VM host scanning configuration
*/
private DefenderForContainersGcpOfferingVmScanners vmScanners;
/**
* Creates an instance of DefenderForContainersGcpOffering class.
*/
public DefenderForContainersGcpOffering() {
}
/**
* Get the offeringType property: The type of the security offering.
*
* @return the offeringType value.
*/
@Override
public OfferingType offeringType() {
return this.offeringType;
}
/**
* Get the nativeCloudConnection property: The native cloud connection configuration.
*
* @return the nativeCloudConnection value.
*/
public DefenderForContainersGcpOfferingNativeCloudConnection nativeCloudConnection() {
return this.nativeCloudConnection;
}
/**
* Set the nativeCloudConnection property: The native cloud connection configuration.
*
* @param nativeCloudConnection the nativeCloudConnection value to set.
* @return the DefenderForContainersGcpOffering object itself.
*/
public DefenderForContainersGcpOffering
withNativeCloudConnection(DefenderForContainersGcpOfferingNativeCloudConnection nativeCloudConnection) {
this.nativeCloudConnection = nativeCloudConnection;
return this;
}
/**
* Get the dataPipelineNativeCloudConnection property: The native cloud connection configuration.
*
* @return the dataPipelineNativeCloudConnection value.
*/
public DefenderForContainersGcpOfferingDataPipelineNativeCloudConnection dataPipelineNativeCloudConnection() {
return this.dataPipelineNativeCloudConnection;
}
/**
* Set the dataPipelineNativeCloudConnection property: The native cloud connection configuration.
*
* @param dataPipelineNativeCloudConnection the dataPipelineNativeCloudConnection value to set.
* @return the DefenderForContainersGcpOffering object itself.
*/
public DefenderForContainersGcpOffering withDataPipelineNativeCloudConnection(
DefenderForContainersGcpOfferingDataPipelineNativeCloudConnection dataPipelineNativeCloudConnection) {
this.dataPipelineNativeCloudConnection = dataPipelineNativeCloudConnection;
return this;
}
/**
* Get the enableAuditLogsAutoProvisioning property: Is audit logs data collection enabled.
*
* @return the enableAuditLogsAutoProvisioning value.
*/
public Boolean enableAuditLogsAutoProvisioning() {
return this.enableAuditLogsAutoProvisioning;
}
/**
* Set the enableAuditLogsAutoProvisioning property: Is audit logs data collection enabled.
*
* @param enableAuditLogsAutoProvisioning the enableAuditLogsAutoProvisioning value to set.
* @return the DefenderForContainersGcpOffering object itself.
*/
public DefenderForContainersGcpOffering
withEnableAuditLogsAutoProvisioning(Boolean enableAuditLogsAutoProvisioning) {
this.enableAuditLogsAutoProvisioning = enableAuditLogsAutoProvisioning;
return this;
}
/**
* Get the enableDefenderAgentAutoProvisioning property: Is Microsoft Defender for Cloud Kubernetes agent auto
* provisioning enabled.
*
* @return the enableDefenderAgentAutoProvisioning value.
*/
public Boolean enableDefenderAgentAutoProvisioning() {
return this.enableDefenderAgentAutoProvisioning;
}
/**
* Set the enableDefenderAgentAutoProvisioning property: Is Microsoft Defender for Cloud Kubernetes agent auto
* provisioning enabled.
*
* @param enableDefenderAgentAutoProvisioning the enableDefenderAgentAutoProvisioning value to set.
* @return the DefenderForContainersGcpOffering object itself.
*/
public DefenderForContainersGcpOffering
withEnableDefenderAgentAutoProvisioning(Boolean enableDefenderAgentAutoProvisioning) {
this.enableDefenderAgentAutoProvisioning = enableDefenderAgentAutoProvisioning;
return this;
}
/**
* Get the enablePolicyAgentAutoProvisioning property: Is Policy Kubernetes agent auto provisioning enabled.
*
* @return the enablePolicyAgentAutoProvisioning value.
*/
public Boolean enablePolicyAgentAutoProvisioning() {
return this.enablePolicyAgentAutoProvisioning;
}
/**
* Set the enablePolicyAgentAutoProvisioning property: Is Policy Kubernetes agent auto provisioning enabled.
*
* @param enablePolicyAgentAutoProvisioning the enablePolicyAgentAutoProvisioning value to set.
* @return the DefenderForContainersGcpOffering object itself.
*/
public DefenderForContainersGcpOffering
withEnablePolicyAgentAutoProvisioning(Boolean enablePolicyAgentAutoProvisioning) {
this.enablePolicyAgentAutoProvisioning = enablePolicyAgentAutoProvisioning;
return this;
}
/**
* Get the mdcContainersImageAssessment property: The Microsoft Defender Container image assessment configuration.
*
* @return the mdcContainersImageAssessment value.
*/
public DefenderForContainersGcpOfferingMdcContainersImageAssessment mdcContainersImageAssessment() {
return this.mdcContainersImageAssessment;
}
/**
* Set the mdcContainersImageAssessment property: The Microsoft Defender Container image assessment configuration.
*
* @param mdcContainersImageAssessment the mdcContainersImageAssessment value to set.
* @return the DefenderForContainersGcpOffering object itself.
*/
public DefenderForContainersGcpOffering withMdcContainersImageAssessment(
DefenderForContainersGcpOfferingMdcContainersImageAssessment mdcContainersImageAssessment) {
this.mdcContainersImageAssessment = mdcContainersImageAssessment;
return this;
}
/**
* Get the mdcContainersAgentlessDiscoveryK8S property: The Microsoft Defender Container agentless discovery
* configuration.
*
* @return the mdcContainersAgentlessDiscoveryK8S value.
*/
public DefenderForContainersGcpOfferingMdcContainersAgentlessDiscoveryK8S mdcContainersAgentlessDiscoveryK8S() {
return this.mdcContainersAgentlessDiscoveryK8S;
}
/**
* Set the mdcContainersAgentlessDiscoveryK8S property: The Microsoft Defender Container agentless discovery
* configuration.
*
* @param mdcContainersAgentlessDiscoveryK8S the mdcContainersAgentlessDiscoveryK8S value to set.
* @return the DefenderForContainersGcpOffering object itself.
*/
public DefenderForContainersGcpOffering withMdcContainersAgentlessDiscoveryK8S(
DefenderForContainersGcpOfferingMdcContainersAgentlessDiscoveryK8S mdcContainersAgentlessDiscoveryK8S) {
this.mdcContainersAgentlessDiscoveryK8S = mdcContainersAgentlessDiscoveryK8S;
return this;
}
/**
* Get the vmScanners property: The Microsoft Defender for Container K8s VM host scanning configuration.
*
* @return the vmScanners value.
*/
public DefenderForContainersGcpOfferingVmScanners vmScanners() {
return this.vmScanners;
}
/**
* Set the vmScanners property: The Microsoft Defender for Container K8s VM host scanning configuration.
*
* @param vmScanners the vmScanners value to set.
* @return the DefenderForContainersGcpOffering object itself.
*/
public DefenderForContainersGcpOffering withVmScanners(DefenderForContainersGcpOfferingVmScanners vmScanners) {
this.vmScanners = vmScanners;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
@Override
public void validate() {
if (nativeCloudConnection() != null) {
nativeCloudConnection().validate();
}
if (dataPipelineNativeCloudConnection() != null) {
dataPipelineNativeCloudConnection().validate();
}
if (mdcContainersImageAssessment() != null) {
mdcContainersImageAssessment().validate();
}
if (mdcContainersAgentlessDiscoveryK8S() != null) {
mdcContainersAgentlessDiscoveryK8S().validate();
}
if (vmScanners() != null) {
vmScanners().validate();
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("offeringType", this.offeringType == null ? null : this.offeringType.toString());
jsonWriter.writeJsonField("nativeCloudConnection", this.nativeCloudConnection);
jsonWriter.writeJsonField("dataPipelineNativeCloudConnection", this.dataPipelineNativeCloudConnection);
jsonWriter.writeBooleanField("enableAuditLogsAutoProvisioning", this.enableAuditLogsAutoProvisioning);
jsonWriter.writeBooleanField("enableDefenderAgentAutoProvisioning", this.enableDefenderAgentAutoProvisioning);
jsonWriter.writeBooleanField("enablePolicyAgentAutoProvisioning", this.enablePolicyAgentAutoProvisioning);
jsonWriter.writeJsonField("mdcContainersImageAssessment", this.mdcContainersImageAssessment);
jsonWriter.writeJsonField("mdcContainersAgentlessDiscoveryK8s", this.mdcContainersAgentlessDiscoveryK8S);
jsonWriter.writeJsonField("vmScanners", this.vmScanners);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of DefenderForContainersGcpOffering from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of DefenderForContainersGcpOffering if the JsonReader was pointing to an instance of it, or
* null if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the DefenderForContainersGcpOffering.
*/
public static DefenderForContainersGcpOffering fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
DefenderForContainersGcpOffering deserializedDefenderForContainersGcpOffering
= new DefenderForContainersGcpOffering();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("description".equals(fieldName)) {
deserializedDefenderForContainersGcpOffering.withDescription(reader.getString());
} else if ("offeringType".equals(fieldName)) {
deserializedDefenderForContainersGcpOffering.offeringType
= OfferingType.fromString(reader.getString());
} else if ("nativeCloudConnection".equals(fieldName)) {
deserializedDefenderForContainersGcpOffering.nativeCloudConnection
= DefenderForContainersGcpOfferingNativeCloudConnection.fromJson(reader);
} else if ("dataPipelineNativeCloudConnection".equals(fieldName)) {
deserializedDefenderForContainersGcpOffering.dataPipelineNativeCloudConnection
= DefenderForContainersGcpOfferingDataPipelineNativeCloudConnection.fromJson(reader);
} else if ("enableAuditLogsAutoProvisioning".equals(fieldName)) {
deserializedDefenderForContainersGcpOffering.enableAuditLogsAutoProvisioning
= reader.getNullable(JsonReader::getBoolean);
} else if ("enableDefenderAgentAutoProvisioning".equals(fieldName)) {
deserializedDefenderForContainersGcpOffering.enableDefenderAgentAutoProvisioning
= reader.getNullable(JsonReader::getBoolean);
} else if ("enablePolicyAgentAutoProvisioning".equals(fieldName)) {
deserializedDefenderForContainersGcpOffering.enablePolicyAgentAutoProvisioning
= reader.getNullable(JsonReader::getBoolean);
} else if ("mdcContainersImageAssessment".equals(fieldName)) {
deserializedDefenderForContainersGcpOffering.mdcContainersImageAssessment
= DefenderForContainersGcpOfferingMdcContainersImageAssessment.fromJson(reader);
} else if ("mdcContainersAgentlessDiscoveryK8s".equals(fieldName)) {
deserializedDefenderForContainersGcpOffering.mdcContainersAgentlessDiscoveryK8S
= DefenderForContainersGcpOfferingMdcContainersAgentlessDiscoveryK8S.fromJson(reader);
} else if ("vmScanners".equals(fieldName)) {
deserializedDefenderForContainersGcpOffering.vmScanners
= DefenderForContainersGcpOfferingVmScanners.fromJson(reader);
} else {
reader.skipChildren();
}
}
return deserializedDefenderForContainersGcpOffering;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy