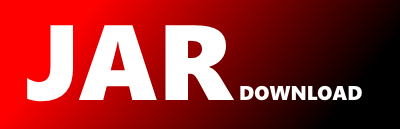
com.azure.resourcemanager.security.models.GcpCredentialsDetailsProperties Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.security.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.logging.ClientLogger;
import com.azure.json.JsonReader;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.util.List;
/**
* GCP cloud account connector based service to service credentials, the credentials are composed of the organization ID
* and a JSON API key (write only).
*/
@Fluent
public final class GcpCredentialsDetailsProperties extends AuthenticationDetailsProperties {
/*
* Connect to your cloud account, for AWS use either account credentials or role-based authentication. For GCP use
* account organization credentials.
*/
private AuthenticationType authenticationType = AuthenticationType.GCP_CREDENTIALS;
/*
* The organization ID of the GCP cloud account
*/
private String organizationId;
/*
* Type field of the API key (write only)
*/
private String type;
/*
* Project ID field of the API key (write only)
*/
private String projectId;
/*
* Private key ID field of the API key (write only)
*/
private String privateKeyId;
/*
* Private key field of the API key (write only)
*/
private String privateKey;
/*
* Client email field of the API key (write only)
*/
private String clientEmail;
/*
* Client ID field of the API key (write only)
*/
private String clientId;
/*
* Auth URI field of the API key (write only)
*/
private String authUri;
/*
* Token URI field of the API key (write only)
*/
private String tokenUri;
/*
* Auth provider x509 certificate URL field of the API key (write only)
*/
private String authProviderX509CertUrl;
/*
* Client x509 certificate URL field of the API key (write only)
*/
private String clientX509CertUrl;
/**
* Creates an instance of GcpCredentialsDetailsProperties class.
*/
public GcpCredentialsDetailsProperties() {
}
/**
* Get the authenticationType property: Connect to your cloud account, for AWS use either account credentials or
* role-based authentication. For GCP use account organization credentials.
*
* @return the authenticationType value.
*/
@Override
public AuthenticationType authenticationType() {
return this.authenticationType;
}
/**
* Get the organizationId property: The organization ID of the GCP cloud account.
*
* @return the organizationId value.
*/
public String organizationId() {
return this.organizationId;
}
/**
* Set the organizationId property: The organization ID of the GCP cloud account.
*
* @param organizationId the organizationId value to set.
* @return the GcpCredentialsDetailsProperties object itself.
*/
public GcpCredentialsDetailsProperties withOrganizationId(String organizationId) {
this.organizationId = organizationId;
return this;
}
/**
* Get the type property: Type field of the API key (write only).
*
* @return the type value.
*/
public String type() {
return this.type;
}
/**
* Set the type property: Type field of the API key (write only).
*
* @param type the type value to set.
* @return the GcpCredentialsDetailsProperties object itself.
*/
public GcpCredentialsDetailsProperties withType(String type) {
this.type = type;
return this;
}
/**
* Get the projectId property: Project ID field of the API key (write only).
*
* @return the projectId value.
*/
public String projectId() {
return this.projectId;
}
/**
* Set the projectId property: Project ID field of the API key (write only).
*
* @param projectId the projectId value to set.
* @return the GcpCredentialsDetailsProperties object itself.
*/
public GcpCredentialsDetailsProperties withProjectId(String projectId) {
this.projectId = projectId;
return this;
}
/**
* Get the privateKeyId property: Private key ID field of the API key (write only).
*
* @return the privateKeyId value.
*/
public String privateKeyId() {
return this.privateKeyId;
}
/**
* Set the privateKeyId property: Private key ID field of the API key (write only).
*
* @param privateKeyId the privateKeyId value to set.
* @return the GcpCredentialsDetailsProperties object itself.
*/
public GcpCredentialsDetailsProperties withPrivateKeyId(String privateKeyId) {
this.privateKeyId = privateKeyId;
return this;
}
/**
* Get the privateKey property: Private key field of the API key (write only).
*
* @return the privateKey value.
*/
public String privateKey() {
return this.privateKey;
}
/**
* Set the privateKey property: Private key field of the API key (write only).
*
* @param privateKey the privateKey value to set.
* @return the GcpCredentialsDetailsProperties object itself.
*/
public GcpCredentialsDetailsProperties withPrivateKey(String privateKey) {
this.privateKey = privateKey;
return this;
}
/**
* Get the clientEmail property: Client email field of the API key (write only).
*
* @return the clientEmail value.
*/
public String clientEmail() {
return this.clientEmail;
}
/**
* Set the clientEmail property: Client email field of the API key (write only).
*
* @param clientEmail the clientEmail value to set.
* @return the GcpCredentialsDetailsProperties object itself.
*/
public GcpCredentialsDetailsProperties withClientEmail(String clientEmail) {
this.clientEmail = clientEmail;
return this;
}
/**
* Get the clientId property: Client ID field of the API key (write only).
*
* @return the clientId value.
*/
public String clientId() {
return this.clientId;
}
/**
* Set the clientId property: Client ID field of the API key (write only).
*
* @param clientId the clientId value to set.
* @return the GcpCredentialsDetailsProperties object itself.
*/
public GcpCredentialsDetailsProperties withClientId(String clientId) {
this.clientId = clientId;
return this;
}
/**
* Get the authUri property: Auth URI field of the API key (write only).
*
* @return the authUri value.
*/
public String authUri() {
return this.authUri;
}
/**
* Set the authUri property: Auth URI field of the API key (write only).
*
* @param authUri the authUri value to set.
* @return the GcpCredentialsDetailsProperties object itself.
*/
public GcpCredentialsDetailsProperties withAuthUri(String authUri) {
this.authUri = authUri;
return this;
}
/**
* Get the tokenUri property: Token URI field of the API key (write only).
*
* @return the tokenUri value.
*/
public String tokenUri() {
return this.tokenUri;
}
/**
* Set the tokenUri property: Token URI field of the API key (write only).
*
* @param tokenUri the tokenUri value to set.
* @return the GcpCredentialsDetailsProperties object itself.
*/
public GcpCredentialsDetailsProperties withTokenUri(String tokenUri) {
this.tokenUri = tokenUri;
return this;
}
/**
* Get the authProviderX509CertUrl property: Auth provider x509 certificate URL field of the API key (write only).
*
* @return the authProviderX509CertUrl value.
*/
public String authProviderX509CertUrl() {
return this.authProviderX509CertUrl;
}
/**
* Set the authProviderX509CertUrl property: Auth provider x509 certificate URL field of the API key (write only).
*
* @param authProviderX509CertUrl the authProviderX509CertUrl value to set.
* @return the GcpCredentialsDetailsProperties object itself.
*/
public GcpCredentialsDetailsProperties withAuthProviderX509CertUrl(String authProviderX509CertUrl) {
this.authProviderX509CertUrl = authProviderX509CertUrl;
return this;
}
/**
* Get the clientX509CertUrl property: Client x509 certificate URL field of the API key (write only).
*
* @return the clientX509CertUrl value.
*/
public String clientX509CertUrl() {
return this.clientX509CertUrl;
}
/**
* Set the clientX509CertUrl property: Client x509 certificate URL field of the API key (write only).
*
* @param clientX509CertUrl the clientX509CertUrl value to set.
* @return the GcpCredentialsDetailsProperties object itself.
*/
public GcpCredentialsDetailsProperties withClientX509CertUrl(String clientX509CertUrl) {
this.clientX509CertUrl = clientX509CertUrl;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
@Override
public void validate() {
if (organizationId() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property organizationId in model GcpCredentialsDetailsProperties"));
}
if (type() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property type in model GcpCredentialsDetailsProperties"));
}
if (projectId() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property projectId in model GcpCredentialsDetailsProperties"));
}
if (privateKeyId() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property privateKeyId in model GcpCredentialsDetailsProperties"));
}
if (privateKey() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property privateKey in model GcpCredentialsDetailsProperties"));
}
if (clientEmail() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property clientEmail in model GcpCredentialsDetailsProperties"));
}
if (clientId() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property clientId in model GcpCredentialsDetailsProperties"));
}
if (authUri() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property authUri in model GcpCredentialsDetailsProperties"));
}
if (tokenUri() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property tokenUri in model GcpCredentialsDetailsProperties"));
}
if (authProviderX509CertUrl() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property authProviderX509CertUrl in model GcpCredentialsDetailsProperties"));
}
if (clientX509CertUrl() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property clientX509CertUrl in model GcpCredentialsDetailsProperties"));
}
}
private static final ClientLogger LOGGER = new ClientLogger(GcpCredentialsDetailsProperties.class);
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("organizationId", this.organizationId);
jsonWriter.writeStringField("type", this.type);
jsonWriter.writeStringField("projectId", this.projectId);
jsonWriter.writeStringField("privateKeyId", this.privateKeyId);
jsonWriter.writeStringField("privateKey", this.privateKey);
jsonWriter.writeStringField("clientEmail", this.clientEmail);
jsonWriter.writeStringField("clientId", this.clientId);
jsonWriter.writeStringField("authUri", this.authUri);
jsonWriter.writeStringField("tokenUri", this.tokenUri);
jsonWriter.writeStringField("authProviderX509CertUrl", this.authProviderX509CertUrl);
jsonWriter.writeStringField("clientX509CertUrl", this.clientX509CertUrl);
jsonWriter.writeStringField("authenticationType",
this.authenticationType == null ? null : this.authenticationType.toString());
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of GcpCredentialsDetailsProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of GcpCredentialsDetailsProperties if the JsonReader was pointing to an instance of it, or
* null if it was pointing to JSON null.
* @throws IllegalStateException If the deserialized JSON object was missing any required properties.
* @throws IOException If an error occurs while reading the GcpCredentialsDetailsProperties.
*/
public static GcpCredentialsDetailsProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
GcpCredentialsDetailsProperties deserializedGcpCredentialsDetailsProperties
= new GcpCredentialsDetailsProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("authenticationProvisioningState".equals(fieldName)) {
deserializedGcpCredentialsDetailsProperties.withAuthenticationProvisioningState(
AuthenticationProvisioningState.fromString(reader.getString()));
} else if ("grantedPermissions".equals(fieldName)) {
List grantedPermissions
= reader.readArray(reader1 -> PermissionProperty.fromString(reader1.getString()));
deserializedGcpCredentialsDetailsProperties.withGrantedPermissions(grantedPermissions);
} else if ("organizationId".equals(fieldName)) {
deserializedGcpCredentialsDetailsProperties.organizationId = reader.getString();
} else if ("type".equals(fieldName)) {
deserializedGcpCredentialsDetailsProperties.type = reader.getString();
} else if ("projectId".equals(fieldName)) {
deserializedGcpCredentialsDetailsProperties.projectId = reader.getString();
} else if ("privateKeyId".equals(fieldName)) {
deserializedGcpCredentialsDetailsProperties.privateKeyId = reader.getString();
} else if ("privateKey".equals(fieldName)) {
deserializedGcpCredentialsDetailsProperties.privateKey = reader.getString();
} else if ("clientEmail".equals(fieldName)) {
deserializedGcpCredentialsDetailsProperties.clientEmail = reader.getString();
} else if ("clientId".equals(fieldName)) {
deserializedGcpCredentialsDetailsProperties.clientId = reader.getString();
} else if ("authUri".equals(fieldName)) {
deserializedGcpCredentialsDetailsProperties.authUri = reader.getString();
} else if ("tokenUri".equals(fieldName)) {
deserializedGcpCredentialsDetailsProperties.tokenUri = reader.getString();
} else if ("authProviderX509CertUrl".equals(fieldName)) {
deserializedGcpCredentialsDetailsProperties.authProviderX509CertUrl = reader.getString();
} else if ("clientX509CertUrl".equals(fieldName)) {
deserializedGcpCredentialsDetailsProperties.clientX509CertUrl = reader.getString();
} else if ("authenticationType".equals(fieldName)) {
deserializedGcpCredentialsDetailsProperties.authenticationType
= AuthenticationType.fromString(reader.getString());
} else {
reader.skipChildren();
}
}
return deserializedGcpCredentialsDetailsProperties;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy