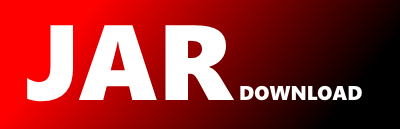
com.azure.resourcemanager.security.models.GcpOrganizationalDataMember Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.security.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
/**
* The gcpOrganization data for the member account.
*/
@Fluent
public final class GcpOrganizationalDataMember extends GcpOrganizationalData {
/*
* The multi cloud account's membership type in the organization
*/
private OrganizationMembershipType organizationMembershipType = OrganizationMembershipType.MEMBER;
/*
* If the multi cloud account is not of membership type organization, this will be the ID of the project's parent
*/
private String parentHierarchyId;
/*
* The GCP management project number from organizational onboarding
*/
private String managementProjectNumber;
/**
* Creates an instance of GcpOrganizationalDataMember class.
*/
public GcpOrganizationalDataMember() {
}
/**
* Get the organizationMembershipType property: The multi cloud account's membership type in the organization.
*
* @return the organizationMembershipType value.
*/
@Override
public OrganizationMembershipType organizationMembershipType() {
return this.organizationMembershipType;
}
/**
* Get the parentHierarchyId property: If the multi cloud account is not of membership type organization, this will
* be the ID of the project's parent.
*
* @return the parentHierarchyId value.
*/
public String parentHierarchyId() {
return this.parentHierarchyId;
}
/**
* Set the parentHierarchyId property: If the multi cloud account is not of membership type organization, this will
* be the ID of the project's parent.
*
* @param parentHierarchyId the parentHierarchyId value to set.
* @return the GcpOrganizationalDataMember object itself.
*/
public GcpOrganizationalDataMember withParentHierarchyId(String parentHierarchyId) {
this.parentHierarchyId = parentHierarchyId;
return this;
}
/**
* Get the managementProjectNumber property: The GCP management project number from organizational onboarding.
*
* @return the managementProjectNumber value.
*/
public String managementProjectNumber() {
return this.managementProjectNumber;
}
/**
* Set the managementProjectNumber property: The GCP management project number from organizational onboarding.
*
* @param managementProjectNumber the managementProjectNumber value to set.
* @return the GcpOrganizationalDataMember object itself.
*/
public GcpOrganizationalDataMember withManagementProjectNumber(String managementProjectNumber) {
this.managementProjectNumber = managementProjectNumber;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
@Override
public void validate() {
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("organizationMembershipType",
this.organizationMembershipType == null ? null : this.organizationMembershipType.toString());
jsonWriter.writeStringField("parentHierarchyId", this.parentHierarchyId);
jsonWriter.writeStringField("managementProjectNumber", this.managementProjectNumber);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of GcpOrganizationalDataMember from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of GcpOrganizationalDataMember if the JsonReader was pointing to an instance of it, or null
* if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the GcpOrganizationalDataMember.
*/
public static GcpOrganizationalDataMember fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
GcpOrganizationalDataMember deserializedGcpOrganizationalDataMember = new GcpOrganizationalDataMember();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("organizationMembershipType".equals(fieldName)) {
deserializedGcpOrganizationalDataMember.organizationMembershipType
= OrganizationMembershipType.fromString(reader.getString());
} else if ("parentHierarchyId".equals(fieldName)) {
deserializedGcpOrganizationalDataMember.parentHierarchyId = reader.getString();
} else if ("managementProjectNumber".equals(fieldName)) {
deserializedGcpOrganizationalDataMember.managementProjectNumber = reader.getString();
} else {
reader.skipChildren();
}
}
return deserializedGcpOrganizationalDataMember;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy