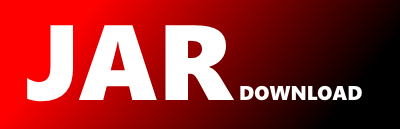
com.azure.resourcemanager.security.models.Pricing Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-security Show documentation
Show all versions of azure-resourcemanager-security Show documentation
This package contains Microsoft Azure SDK for Security Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. API spec for Microsoft.Security (Azure Security Center) resource provider. Package tag package-composite-v3.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.security.models;
import com.azure.resourcemanager.security.fluent.models.PricingInner;
import java.time.Duration;
import java.time.OffsetDateTime;
import java.util.List;
/**
* An immutable client-side representation of Pricing.
*/
public interface Pricing {
/**
* Gets the id property: Fully qualified resource Id for the resource.
*
* @return the id value.
*/
String id();
/**
* Gets the name property: The name of the resource.
*
* @return the name value.
*/
String name();
/**
* Gets the type property: The type of the resource.
*
* @return the type value.
*/
String type();
/**
* Gets the pricingTier property: Indicates whether the Defender plan is enabled on the selected scope. Microsoft
* Defender for Cloud is provided in two pricing tiers: free and standard. The standard tier offers advanced
* security capabilities, while the free tier offers basic security features.
*
* @return the pricingTier value.
*/
PricingTier pricingTier();
/**
* Gets the subPlan property: The sub-plan selected for a Standard pricing configuration, when more than one
* sub-plan is available. Each sub-plan enables a set of security features. When not specified, full plan is
* applied. For VirtualMachines plan, available sub plans are 'P1' & 'P2', where for resource level only 'P1'
* sub plan is supported.
*
* @return the subPlan value.
*/
String subPlan();
/**
* Gets the freeTrialRemainingTime property: The duration left for the subscriptions free trial period - in ISO 8601
* format (e.g. P3Y6M4DT12H30M5S).
*
* @return the freeTrialRemainingTime value.
*/
Duration freeTrialRemainingTime();
/**
* Gets the enablementTime property: Optional. If `pricingTier` is `Standard` then this property holds the date of
* the last time the `pricingTier` was set to `Standard`, when available (e.g 2023-03-01T12:42:42.1921106Z).
*
* @return the enablementTime value.
*/
OffsetDateTime enablementTime();
/**
* Gets the enforce property: If set to "False", it allows the descendants of this scope to override the pricing
* configuration set on this scope (allows setting inherited="False"). If set to "True", it prevents overrides and
* forces this pricing configuration on all the descendants of this scope. This field is only available for
* subscription-level pricing.
*
* @return the enforce value.
*/
Enforce enforce();
/**
* Gets the inherited property: "inherited" = "True" indicates that the current scope inherits its pricing
* configuration from its parent. The ID of the parent scope that provides the inherited configuration is displayed
* in the "inheritedFrom" field. On the other hand, "inherited" = "False" indicates that the current scope has its
* own pricing configuration explicitly set, and does not inherit from its parent. This field is read only and
* available only for resource-level pricing.
*
* @return the inherited value.
*/
Inherited inherited();
/**
* Gets the inheritedFrom property: The id of the scope inherited from. "Null" if not inherited. This field is only
* available for resource-level pricing.
*
* @return the inheritedFrom value.
*/
String inheritedFrom();
/**
* Gets the resourcesCoverageStatus property: This field is available for subscription-level only, and reflects the
* coverage status of the resources under the subscription. Please note: The "pricingTier" field reflects the plan
* status of the subscription. However, since the plan status can also be defined at the resource level, there might
* be misalignment between the subscription's plan status and the resource status. This field helps indicate the
* coverage status of the resources.
*
* @return the resourcesCoverageStatus value.
*/
ResourcesCoverageStatus resourcesCoverageStatus();
/**
* Gets the extensions property: Optional. List of extensions offered under a plan.
*
* @return the extensions value.
*/
List extensions();
/**
* Gets the deprecated property: Optional. True if the plan is deprecated. If there are replacing plans they will
* appear in `replacedBy` property.
*
* @return the deprecated value.
*/
Boolean deprecated();
/**
* Gets the replacedBy property: Optional. List of plans that replace this plan. This property exists only if this
* plan is deprecated.
*
* @return the replacedBy value.
*/
List replacedBy();
/**
* Gets the inner com.azure.resourcemanager.security.fluent.models.PricingInner object.
*
* @return the inner object.
*/
PricingInner innerModel();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy