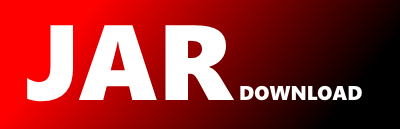
com.azure.resourcemanager.security.models.ScanResultProperties Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.security.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.util.List;
/**
* A vulnerability assessment scan result properties for a single rule.
*/
@Fluent
public final class ScanResultProperties implements JsonSerializable {
/*
* The rule Id.
*/
private String ruleId;
/*
* The rule result status.
*/
private RuleStatus status;
/*
* Indicated whether the results specified here are trimmed.
*/
private Boolean isTrimmed;
/*
* The results of the query that was run.
*/
private List> queryResults;
/*
* Remediation details.
*/
private Remediation remediation;
/*
* The rule result adjusted with baseline.
*/
private BaselineAdjustedResult baselineAdjustedResult;
/*
* vulnerability assessment rule metadata details.
*/
private VaRule ruleMetadata;
/**
* Creates an instance of ScanResultProperties class.
*/
public ScanResultProperties() {
}
/**
* Get the ruleId property: The rule Id.
*
* @return the ruleId value.
*/
public String ruleId() {
return this.ruleId;
}
/**
* Set the ruleId property: The rule Id.
*
* @param ruleId the ruleId value to set.
* @return the ScanResultProperties object itself.
*/
public ScanResultProperties withRuleId(String ruleId) {
this.ruleId = ruleId;
return this;
}
/**
* Get the status property: The rule result status.
*
* @return the status value.
*/
public RuleStatus status() {
return this.status;
}
/**
* Set the status property: The rule result status.
*
* @param status the status value to set.
* @return the ScanResultProperties object itself.
*/
public ScanResultProperties withStatus(RuleStatus status) {
this.status = status;
return this;
}
/**
* Get the isTrimmed property: Indicated whether the results specified here are trimmed.
*
* @return the isTrimmed value.
*/
public Boolean isTrimmed() {
return this.isTrimmed;
}
/**
* Set the isTrimmed property: Indicated whether the results specified here are trimmed.
*
* @param isTrimmed the isTrimmed value to set.
* @return the ScanResultProperties object itself.
*/
public ScanResultProperties withIsTrimmed(Boolean isTrimmed) {
this.isTrimmed = isTrimmed;
return this;
}
/**
* Get the queryResults property: The results of the query that was run.
*
* @return the queryResults value.
*/
public List> queryResults() {
return this.queryResults;
}
/**
* Set the queryResults property: The results of the query that was run.
*
* @param queryResults the queryResults value to set.
* @return the ScanResultProperties object itself.
*/
public ScanResultProperties withQueryResults(List> queryResults) {
this.queryResults = queryResults;
return this;
}
/**
* Get the remediation property: Remediation details.
*
* @return the remediation value.
*/
public Remediation remediation() {
return this.remediation;
}
/**
* Set the remediation property: Remediation details.
*
* @param remediation the remediation value to set.
* @return the ScanResultProperties object itself.
*/
public ScanResultProperties withRemediation(Remediation remediation) {
this.remediation = remediation;
return this;
}
/**
* Get the baselineAdjustedResult property: The rule result adjusted with baseline.
*
* @return the baselineAdjustedResult value.
*/
public BaselineAdjustedResult baselineAdjustedResult() {
return this.baselineAdjustedResult;
}
/**
* Set the baselineAdjustedResult property: The rule result adjusted with baseline.
*
* @param baselineAdjustedResult the baselineAdjustedResult value to set.
* @return the ScanResultProperties object itself.
*/
public ScanResultProperties withBaselineAdjustedResult(BaselineAdjustedResult baselineAdjustedResult) {
this.baselineAdjustedResult = baselineAdjustedResult;
return this;
}
/**
* Get the ruleMetadata property: vulnerability assessment rule metadata details.
*
* @return the ruleMetadata value.
*/
public VaRule ruleMetadata() {
return this.ruleMetadata;
}
/**
* Set the ruleMetadata property: vulnerability assessment rule metadata details.
*
* @param ruleMetadata the ruleMetadata value to set.
* @return the ScanResultProperties object itself.
*/
public ScanResultProperties withRuleMetadata(VaRule ruleMetadata) {
this.ruleMetadata = ruleMetadata;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (remediation() != null) {
remediation().validate();
}
if (baselineAdjustedResult() != null) {
baselineAdjustedResult().validate();
}
if (ruleMetadata() != null) {
ruleMetadata().validate();
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("ruleId", this.ruleId);
jsonWriter.writeStringField("status", this.status == null ? null : this.status.toString());
jsonWriter.writeBooleanField("isTrimmed", this.isTrimmed);
jsonWriter.writeArrayField("queryResults", this.queryResults,
(writer, element) -> writer.writeArray(element, (writer1, element1) -> writer1.writeString(element1)));
jsonWriter.writeJsonField("remediation", this.remediation);
jsonWriter.writeJsonField("baselineAdjustedResult", this.baselineAdjustedResult);
jsonWriter.writeJsonField("ruleMetadata", this.ruleMetadata);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of ScanResultProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of ScanResultProperties if the JsonReader was pointing to an instance of it, or null if it
* was pointing to JSON null.
* @throws IOException If an error occurs while reading the ScanResultProperties.
*/
public static ScanResultProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
ScanResultProperties deserializedScanResultProperties = new ScanResultProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("ruleId".equals(fieldName)) {
deserializedScanResultProperties.ruleId = reader.getString();
} else if ("status".equals(fieldName)) {
deserializedScanResultProperties.status = RuleStatus.fromString(reader.getString());
} else if ("isTrimmed".equals(fieldName)) {
deserializedScanResultProperties.isTrimmed = reader.getNullable(JsonReader::getBoolean);
} else if ("queryResults".equals(fieldName)) {
List> queryResults
= reader.readArray(reader1 -> reader1.readArray(reader2 -> reader2.getString()));
deserializedScanResultProperties.queryResults = queryResults;
} else if ("remediation".equals(fieldName)) {
deserializedScanResultProperties.remediation = Remediation.fromJson(reader);
} else if ("baselineAdjustedResult".equals(fieldName)) {
deserializedScanResultProperties.baselineAdjustedResult = BaselineAdjustedResult.fromJson(reader);
} else if ("ruleMetadata".equals(fieldName)) {
deserializedScanResultProperties.ruleMetadata = VaRule.fromJson(reader);
} else {
reader.skipChildren();
}
}
return deserializedScanResultProperties;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy