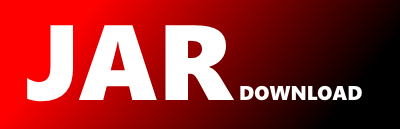
com.azure.resourcemanager.security.models.StandardAssignment Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-security Show documentation
Show all versions of azure-resourcemanager-security Show documentation
This package contains Microsoft Azure SDK for Security Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. API spec for Microsoft.Security (Azure Security Center) resource provider. Package tag package-composite-v3.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.security.models;
import com.azure.core.util.Context;
import com.azure.resourcemanager.security.fluent.models.StandardAssignmentInner;
import java.time.OffsetDateTime;
import java.util.List;
/**
* An immutable client-side representation of StandardAssignment.
*/
public interface StandardAssignment {
/**
* Gets the id property: Fully qualified resource Id for the resource.
*
* @return the id value.
*/
String id();
/**
* Gets the name property: The name of the resource.
*
* @return the name value.
*/
String name();
/**
* Gets the type property: The type of the resource.
*
* @return the type value.
*/
String type();
/**
* Gets the displayName property: Display name of the standardAssignment.
*
* @return the displayName value.
*/
String displayName();
/**
* Gets the description property: Description of the standardAssignment.
*
* @return the description value.
*/
String description();
/**
* Gets the assignedStandard property: Standard item with key as applied to this standard assignment over the given
* scope.
*
* @return the assignedStandard value.
*/
AssignedStandardItem assignedStandard();
/**
* Gets the effect property: Expected effect of this assignment (Audit/Exempt/Attest).
*
* @return the effect value.
*/
Effect effect();
/**
* Gets the excludedScopes property: Excluded scopes, filter out the descendants of the scope (on management
* scopes).
*
* @return the excludedScopes value.
*/
List excludedScopes();
/**
* Gets the expiresOn property: Expiration date of this assignment as a full ISO date.
*
* @return the expiresOn value.
*/
OffsetDateTime expiresOn();
/**
* Gets the exemptionData property: Additional data about assignment that has Exempt effect.
*
* @return the exemptionData value.
*/
StandardAssignmentPropertiesExemptionData exemptionData();
/**
* Gets the attestationData property: Additional data about assignment that has Attest effect.
*
* @return the attestationData value.
*/
StandardAssignmentPropertiesAttestationData attestationData();
/**
* Gets the metadata property: The standard assignment metadata.
*
* @return the metadata value.
*/
StandardAssignmentMetadata metadata();
/**
* Gets the inner com.azure.resourcemanager.security.fluent.models.StandardAssignmentInner object.
*
* @return the inner object.
*/
StandardAssignmentInner innerModel();
/**
* The entirety of the StandardAssignment definition.
*/
interface Definition extends DefinitionStages.Blank, DefinitionStages.WithScope, DefinitionStages.WithCreate {
}
/**
* The StandardAssignment definition stages.
*/
interface DefinitionStages {
/**
* The first stage of the StandardAssignment definition.
*/
interface Blank extends WithScope {
}
/**
* The stage of the StandardAssignment definition allowing to specify parent resource.
*/
interface WithScope {
/**
* Specifies resourceId.
*
* @param resourceId The identifier of the resource.
* @return the next definition stage.
*/
WithCreate withExistingResourceId(String resourceId);
}
/**
* The stage of the StandardAssignment definition which contains all the minimum required properties for the
* resource to be created, but also allows for any other optional properties to be specified.
*/
interface WithCreate extends DefinitionStages.WithDisplayName, DefinitionStages.WithDescription,
DefinitionStages.WithAssignedStandard, DefinitionStages.WithEffect, DefinitionStages.WithExcludedScopes,
DefinitionStages.WithExpiresOn, DefinitionStages.WithExemptionData, DefinitionStages.WithAttestationData,
DefinitionStages.WithMetadata {
/**
* Executes the create request.
*
* @return the created resource.
*/
StandardAssignment create();
/**
* Executes the create request.
*
* @param context The context to associate with this operation.
* @return the created resource.
*/
StandardAssignment create(Context context);
}
/**
* The stage of the StandardAssignment definition allowing to specify displayName.
*/
interface WithDisplayName {
/**
* Specifies the displayName property: Display name of the standardAssignment.
*
* @param displayName Display name of the standardAssignment.
* @return the next definition stage.
*/
WithCreate withDisplayName(String displayName);
}
/**
* The stage of the StandardAssignment definition allowing to specify description.
*/
interface WithDescription {
/**
* Specifies the description property: Description of the standardAssignment.
*
* @param description Description of the standardAssignment.
* @return the next definition stage.
*/
WithCreate withDescription(String description);
}
/**
* The stage of the StandardAssignment definition allowing to specify assignedStandard.
*/
interface WithAssignedStandard {
/**
* Specifies the assignedStandard property: Standard item with key as applied to this standard assignment
* over the given scope.
*
* @param assignedStandard Standard item with key as applied to this standard assignment over the given
* scope.
* @return the next definition stage.
*/
WithCreate withAssignedStandard(AssignedStandardItem assignedStandard);
}
/**
* The stage of the StandardAssignment definition allowing to specify effect.
*/
interface WithEffect {
/**
* Specifies the effect property: Expected effect of this assignment (Audit/Exempt/Attest).
*
* @param effect Expected effect of this assignment (Audit/Exempt/Attest).
* @return the next definition stage.
*/
WithCreate withEffect(Effect effect);
}
/**
* The stage of the StandardAssignment definition allowing to specify excludedScopes.
*/
interface WithExcludedScopes {
/**
* Specifies the excludedScopes property: Excluded scopes, filter out the descendants of the scope (on
* management scopes).
*
* @param excludedScopes Excluded scopes, filter out the descendants of the scope (on management scopes).
* @return the next definition stage.
*/
WithCreate withExcludedScopes(List excludedScopes);
}
/**
* The stage of the StandardAssignment definition allowing to specify expiresOn.
*/
interface WithExpiresOn {
/**
* Specifies the expiresOn property: Expiration date of this assignment as a full ISO date.
*
* @param expiresOn Expiration date of this assignment as a full ISO date.
* @return the next definition stage.
*/
WithCreate withExpiresOn(OffsetDateTime expiresOn);
}
/**
* The stage of the StandardAssignment definition allowing to specify exemptionData.
*/
interface WithExemptionData {
/**
* Specifies the exemptionData property: Additional data about assignment that has Exempt effect.
*
* @param exemptionData Additional data about assignment that has Exempt effect.
* @return the next definition stage.
*/
WithCreate withExemptionData(StandardAssignmentPropertiesExemptionData exemptionData);
}
/**
* The stage of the StandardAssignment definition allowing to specify attestationData.
*/
interface WithAttestationData {
/**
* Specifies the attestationData property: Additional data about assignment that has Attest effect.
*
* @param attestationData Additional data about assignment that has Attest effect.
* @return the next definition stage.
*/
WithCreate withAttestationData(StandardAssignmentPropertiesAttestationData attestationData);
}
/**
* The stage of the StandardAssignment definition allowing to specify metadata.
*/
interface WithMetadata {
/**
* Specifies the metadata property: The standard assignment metadata..
*
* @param metadata The standard assignment metadata.
* @return the next definition stage.
*/
WithCreate withMetadata(StandardAssignmentMetadata metadata);
}
}
/**
* Refreshes the resource to sync with Azure.
*
* @return the refreshed resource.
*/
StandardAssignment refresh();
/**
* Refreshes the resource to sync with Azure.
*
* @param context The context to associate with this operation.
* @return the refreshed resource.
*/
StandardAssignment refresh(Context context);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy