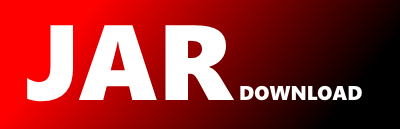
com.azure.resourcemanager.security.models.StandardAssignmentPropertiesAttestationData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-security Show documentation
Show all versions of azure-resourcemanager-security Show documentation
This package contains Microsoft Azure SDK for Security Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. API spec for Microsoft.Security (Azure Security Center) resource provider. Package tag package-composite-v3.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.security.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.CoreUtils;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.time.OffsetDateTime;
import java.util.List;
/**
* Additional data about assignment that has Attest effect.
*/
@Fluent
public final class StandardAssignmentPropertiesAttestationData
implements JsonSerializable {
/*
* Attest category of this assignment
*/
private AttestationComplianceState complianceState;
/*
* Component item with key as applied to this standard assignment over the given scope
*/
private AssignedAssessmentItem assignedAssessment;
/*
* Attestation compliance date
*/
private OffsetDateTime complianceDate;
/*
* Array of links to attestation evidence
*/
private List evidence;
/**
* Creates an instance of StandardAssignmentPropertiesAttestationData class.
*/
public StandardAssignmentPropertiesAttestationData() {
}
/**
* Get the complianceState property: Attest category of this assignment.
*
* @return the complianceState value.
*/
public AttestationComplianceState complianceState() {
return this.complianceState;
}
/**
* Set the complianceState property: Attest category of this assignment.
*
* @param complianceState the complianceState value to set.
* @return the StandardAssignmentPropertiesAttestationData object itself.
*/
public StandardAssignmentPropertiesAttestationData withComplianceState(AttestationComplianceState complianceState) {
this.complianceState = complianceState;
return this;
}
/**
* Get the assignedAssessment property: Component item with key as applied to this standard assignment over the
* given scope.
*
* @return the assignedAssessment value.
*/
public AssignedAssessmentItem assignedAssessment() {
return this.assignedAssessment;
}
/**
* Set the assignedAssessment property: Component item with key as applied to this standard assignment over the
* given scope.
*
* @param assignedAssessment the assignedAssessment value to set.
* @return the StandardAssignmentPropertiesAttestationData object itself.
*/
public StandardAssignmentPropertiesAttestationData
withAssignedAssessment(AssignedAssessmentItem assignedAssessment) {
this.assignedAssessment = assignedAssessment;
return this;
}
/**
* Get the complianceDate property: Attestation compliance date.
*
* @return the complianceDate value.
*/
public OffsetDateTime complianceDate() {
return this.complianceDate;
}
/**
* Get the evidence property: Array of links to attestation evidence.
*
* @return the evidence value.
*/
public List evidence() {
return this.evidence;
}
/**
* Set the evidence property: Array of links to attestation evidence.
*
* @param evidence the evidence value to set.
* @return the StandardAssignmentPropertiesAttestationData object itself.
*/
public StandardAssignmentPropertiesAttestationData withEvidence(List evidence) {
this.evidence = evidence;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (assignedAssessment() != null) {
assignedAssessment().validate();
}
if (evidence() != null) {
evidence().forEach(e -> e.validate());
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("complianceState",
this.complianceState == null ? null : this.complianceState.toString());
jsonWriter.writeJsonField("assignedAssessment", this.assignedAssessment);
jsonWriter.writeArrayField("evidence", this.evidence, (writer, element) -> writer.writeJson(element));
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of StandardAssignmentPropertiesAttestationData from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of StandardAssignmentPropertiesAttestationData if the JsonReader was pointing to an instance
* of it, or null if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the StandardAssignmentPropertiesAttestationData.
*/
public static StandardAssignmentPropertiesAttestationData fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
StandardAssignmentPropertiesAttestationData deserializedStandardAssignmentPropertiesAttestationData
= new StandardAssignmentPropertiesAttestationData();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("complianceState".equals(fieldName)) {
deserializedStandardAssignmentPropertiesAttestationData.complianceState
= AttestationComplianceState.fromString(reader.getString());
} else if ("assignedAssessment".equals(fieldName)) {
deserializedStandardAssignmentPropertiesAttestationData.assignedAssessment
= AssignedAssessmentItem.fromJson(reader);
} else if ("complianceDate".equals(fieldName)) {
deserializedStandardAssignmentPropertiesAttestationData.complianceDate = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("evidence".equals(fieldName)) {
List evidence
= reader.readArray(reader1 -> AttestationEvidence.fromJson(reader1));
deserializedStandardAssignmentPropertiesAttestationData.evidence = evidence;
} else {
reader.skipChildren();
}
}
return deserializedStandardAssignmentPropertiesAttestationData;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy