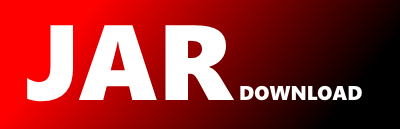
com.azure.resourcemanager.securityinsights.fluent.models.ActivityEntityQueryTemplateProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-securityinsights Show documentation
Show all versions of azure-resourcemanager-securityinsights Show documentation
This package contains Microsoft Azure SDK for SecurityInsights Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. API spec for Microsoft.SecurityInsights (Azure Security Insights) resource provider. Package tag package-preview-2021-09.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.securityinsights.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.securityinsights.models.ActivityEntityQueryTemplatePropertiesQueryDefinitions;
import com.azure.resourcemanager.securityinsights.models.DataTypeDefinitions;
import com.azure.resourcemanager.securityinsights.models.EntityType;
import java.io.IOException;
import java.util.List;
import java.util.Map;
/**
* Describes activity entity query properties.
*/
@Fluent
public final class ActivityEntityQueryTemplateProperties
implements JsonSerializable {
/*
* The entity query title
*/
private String title;
/*
* The entity query content to display in timeline
*/
private String content;
/*
* The entity query description
*/
private String description;
/*
* The Activity query definitions
*/
private ActivityEntityQueryTemplatePropertiesQueryDefinitions queryDefinitions;
/*
* List of required data types for the given entity query template
*/
private List dataTypes;
/*
* The type of the query's source entity
*/
private EntityType inputEntityType;
/*
* List of the fields of the source entity that are required to run the query
*/
private List> requiredInputFieldsSets;
/*
* The query applied only to entities matching to all filters
*/
private Map> entitiesFilter;
/**
* Creates an instance of ActivityEntityQueryTemplateProperties class.
*/
public ActivityEntityQueryTemplateProperties() {
}
/**
* Get the title property: The entity query title.
*
* @return the title value.
*/
public String title() {
return this.title;
}
/**
* Set the title property: The entity query title.
*
* @param title the title value to set.
* @return the ActivityEntityQueryTemplateProperties object itself.
*/
public ActivityEntityQueryTemplateProperties withTitle(String title) {
this.title = title;
return this;
}
/**
* Get the content property: The entity query content to display in timeline.
*
* @return the content value.
*/
public String content() {
return this.content;
}
/**
* Set the content property: The entity query content to display in timeline.
*
* @param content the content value to set.
* @return the ActivityEntityQueryTemplateProperties object itself.
*/
public ActivityEntityQueryTemplateProperties withContent(String content) {
this.content = content;
return this;
}
/**
* Get the description property: The entity query description.
*
* @return the description value.
*/
public String description() {
return this.description;
}
/**
* Set the description property: The entity query description.
*
* @param description the description value to set.
* @return the ActivityEntityQueryTemplateProperties object itself.
*/
public ActivityEntityQueryTemplateProperties withDescription(String description) {
this.description = description;
return this;
}
/**
* Get the queryDefinitions property: The Activity query definitions.
*
* @return the queryDefinitions value.
*/
public ActivityEntityQueryTemplatePropertiesQueryDefinitions queryDefinitions() {
return this.queryDefinitions;
}
/**
* Set the queryDefinitions property: The Activity query definitions.
*
* @param queryDefinitions the queryDefinitions value to set.
* @return the ActivityEntityQueryTemplateProperties object itself.
*/
public ActivityEntityQueryTemplateProperties
withQueryDefinitions(ActivityEntityQueryTemplatePropertiesQueryDefinitions queryDefinitions) {
this.queryDefinitions = queryDefinitions;
return this;
}
/**
* Get the dataTypes property: List of required data types for the given entity query template.
*
* @return the dataTypes value.
*/
public List dataTypes() {
return this.dataTypes;
}
/**
* Set the dataTypes property: List of required data types for the given entity query template.
*
* @param dataTypes the dataTypes value to set.
* @return the ActivityEntityQueryTemplateProperties object itself.
*/
public ActivityEntityQueryTemplateProperties withDataTypes(List dataTypes) {
this.dataTypes = dataTypes;
return this;
}
/**
* Get the inputEntityType property: The type of the query's source entity.
*
* @return the inputEntityType value.
*/
public EntityType inputEntityType() {
return this.inputEntityType;
}
/**
* Set the inputEntityType property: The type of the query's source entity.
*
* @param inputEntityType the inputEntityType value to set.
* @return the ActivityEntityQueryTemplateProperties object itself.
*/
public ActivityEntityQueryTemplateProperties withInputEntityType(EntityType inputEntityType) {
this.inputEntityType = inputEntityType;
return this;
}
/**
* Get the requiredInputFieldsSets property: List of the fields of the source entity that are required to run the
* query.
*
* @return the requiredInputFieldsSets value.
*/
public List> requiredInputFieldsSets() {
return this.requiredInputFieldsSets;
}
/**
* Set the requiredInputFieldsSets property: List of the fields of the source entity that are required to run the
* query.
*
* @param requiredInputFieldsSets the requiredInputFieldsSets value to set.
* @return the ActivityEntityQueryTemplateProperties object itself.
*/
public ActivityEntityQueryTemplateProperties
withRequiredInputFieldsSets(List> requiredInputFieldsSets) {
this.requiredInputFieldsSets = requiredInputFieldsSets;
return this;
}
/**
* Get the entitiesFilter property: The query applied only to entities matching to all filters.
*
* @return the entitiesFilter value.
*/
public Map> entitiesFilter() {
return this.entitiesFilter;
}
/**
* Set the entitiesFilter property: The query applied only to entities matching to all filters.
*
* @param entitiesFilter the entitiesFilter value to set.
* @return the ActivityEntityQueryTemplateProperties object itself.
*/
public ActivityEntityQueryTemplateProperties withEntitiesFilter(Map> entitiesFilter) {
this.entitiesFilter = entitiesFilter;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (queryDefinitions() != null) {
queryDefinitions().validate();
}
if (dataTypes() != null) {
dataTypes().forEach(e -> e.validate());
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("title", this.title);
jsonWriter.writeStringField("content", this.content);
jsonWriter.writeStringField("description", this.description);
jsonWriter.writeJsonField("queryDefinitions", this.queryDefinitions);
jsonWriter.writeArrayField("dataTypes", this.dataTypes, (writer, element) -> writer.writeJson(element));
jsonWriter.writeStringField("inputEntityType",
this.inputEntityType == null ? null : this.inputEntityType.toString());
jsonWriter.writeArrayField("requiredInputFieldsSets", this.requiredInputFieldsSets,
(writer, element) -> writer.writeArray(element, (writer1, element1) -> writer1.writeString(element1)));
jsonWriter.writeMapField("entitiesFilter", this.entitiesFilter,
(writer, element) -> writer.writeArray(element, (writer1, element1) -> writer1.writeString(element1)));
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of ActivityEntityQueryTemplateProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of ActivityEntityQueryTemplateProperties if the JsonReader was pointing to an instance of it,
* or null if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the ActivityEntityQueryTemplateProperties.
*/
public static ActivityEntityQueryTemplateProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
ActivityEntityQueryTemplateProperties deserializedActivityEntityQueryTemplateProperties
= new ActivityEntityQueryTemplateProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("title".equals(fieldName)) {
deserializedActivityEntityQueryTemplateProperties.title = reader.getString();
} else if ("content".equals(fieldName)) {
deserializedActivityEntityQueryTemplateProperties.content = reader.getString();
} else if ("description".equals(fieldName)) {
deserializedActivityEntityQueryTemplateProperties.description = reader.getString();
} else if ("queryDefinitions".equals(fieldName)) {
deserializedActivityEntityQueryTemplateProperties.queryDefinitions
= ActivityEntityQueryTemplatePropertiesQueryDefinitions.fromJson(reader);
} else if ("dataTypes".equals(fieldName)) {
List dataTypes
= reader.readArray(reader1 -> DataTypeDefinitions.fromJson(reader1));
deserializedActivityEntityQueryTemplateProperties.dataTypes = dataTypes;
} else if ("inputEntityType".equals(fieldName)) {
deserializedActivityEntityQueryTemplateProperties.inputEntityType
= EntityType.fromString(reader.getString());
} else if ("requiredInputFieldsSets".equals(fieldName)) {
List> requiredInputFieldsSets
= reader.readArray(reader1 -> reader1.readArray(reader2 -> reader2.getString()));
deserializedActivityEntityQueryTemplateProperties.requiredInputFieldsSets = requiredInputFieldsSets;
} else if ("entitiesFilter".equals(fieldName)) {
Map> entitiesFilter
= reader.readMap(reader1 -> reader1.readArray(reader2 -> reader2.getString()));
deserializedActivityEntityQueryTemplateProperties.entitiesFilter = entitiesFilter;
} else {
reader.skipChildren();
}
}
return deserializedActivityEntityQueryTemplateProperties;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy