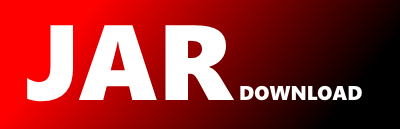
com.azure.resourcemanager.securityinsights.fluent.models.AnomalySecurityMLAnalyticsSettingsProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-securityinsights Show documentation
Show all versions of azure-resourcemanager-securityinsights Show documentation
This package contains Microsoft Azure SDK for SecurityInsights Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. API spec for Microsoft.SecurityInsights (Azure Security Insights) resource provider. Package tag package-preview-2021-09.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.securityinsights.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.CoreUtils;
import com.azure.core.util.logging.ClientLogger;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.securityinsights.models.AttackTactic;
import com.azure.resourcemanager.securityinsights.models.SecurityMLAnalyticsSettingsDataSource;
import com.azure.resourcemanager.securityinsights.models.SettingsStatus;
import java.io.IOException;
import java.time.Duration;
import java.time.OffsetDateTime;
import java.util.List;
import java.util.Objects;
import java.util.UUID;
/**
* AnomalySecurityMLAnalytics settings base property bag.
*/
@Fluent
public final class AnomalySecurityMLAnalyticsSettingsProperties
implements JsonSerializable {
/*
* The description of the SecurityMLAnalyticsSettings.
*/
private String description;
/*
* The display name for settings created by this SecurityMLAnalyticsSettings.
*/
private String displayName;
/*
* Determines whether this settings is enabled or disabled.
*/
private boolean enabled;
/*
* The last time that this SecurityMLAnalyticsSettings has been modified.
*/
private OffsetDateTime lastModifiedUtc;
/*
* The required data sources for this SecurityMLAnalyticsSettings
*/
private List requiredDataConnectors;
/*
* The tactics of the SecurityMLAnalyticsSettings
*/
private List tactics;
/*
* The techniques of the SecurityMLAnalyticsSettings
*/
private List techniques;
/*
* The anomaly version of the AnomalySecurityMLAnalyticsSettings.
*/
private String anomalyVersion;
/*
* The customizable observations of the AnomalySecurityMLAnalyticsSettings.
*/
private Object customizableObservations;
/*
* The frequency that this SecurityMLAnalyticsSettings will be run.
*/
private Duration frequency;
/*
* The anomaly SecurityMLAnalyticsSettings status
*/
private SettingsStatus settingsStatus;
/*
* Determines whether this anomaly security ml analytics settings is a default settings
*/
private boolean isDefaultSettings;
/*
* The anomaly settings version of the Anomaly security ml analytics settings that dictates whether job version gets
* updated or not.
*/
private Integer anomalySettingsVersion;
/*
* The anomaly settings definition Id
*/
private UUID settingsDefinitionId;
/**
* Creates an instance of AnomalySecurityMLAnalyticsSettingsProperties class.
*/
public AnomalySecurityMLAnalyticsSettingsProperties() {
}
/**
* Get the description property: The description of the SecurityMLAnalyticsSettings.
*
* @return the description value.
*/
public String description() {
return this.description;
}
/**
* Set the description property: The description of the SecurityMLAnalyticsSettings.
*
* @param description the description value to set.
* @return the AnomalySecurityMLAnalyticsSettingsProperties object itself.
*/
public AnomalySecurityMLAnalyticsSettingsProperties withDescription(String description) {
this.description = description;
return this;
}
/**
* Get the displayName property: The display name for settings created by this SecurityMLAnalyticsSettings.
*
* @return the displayName value.
*/
public String displayName() {
return this.displayName;
}
/**
* Set the displayName property: The display name for settings created by this SecurityMLAnalyticsSettings.
*
* @param displayName the displayName value to set.
* @return the AnomalySecurityMLAnalyticsSettingsProperties object itself.
*/
public AnomalySecurityMLAnalyticsSettingsProperties withDisplayName(String displayName) {
this.displayName = displayName;
return this;
}
/**
* Get the enabled property: Determines whether this settings is enabled or disabled.
*
* @return the enabled value.
*/
public boolean enabled() {
return this.enabled;
}
/**
* Set the enabled property: Determines whether this settings is enabled or disabled.
*
* @param enabled the enabled value to set.
* @return the AnomalySecurityMLAnalyticsSettingsProperties object itself.
*/
public AnomalySecurityMLAnalyticsSettingsProperties withEnabled(boolean enabled) {
this.enabled = enabled;
return this;
}
/**
* Get the lastModifiedUtc property: The last time that this SecurityMLAnalyticsSettings has been modified.
*
* @return the lastModifiedUtc value.
*/
public OffsetDateTime lastModifiedUtc() {
return this.lastModifiedUtc;
}
/**
* Get the requiredDataConnectors property: The required data sources for this SecurityMLAnalyticsSettings.
*
* @return the requiredDataConnectors value.
*/
public List requiredDataConnectors() {
return this.requiredDataConnectors;
}
/**
* Set the requiredDataConnectors property: The required data sources for this SecurityMLAnalyticsSettings.
*
* @param requiredDataConnectors the requiredDataConnectors value to set.
* @return the AnomalySecurityMLAnalyticsSettingsProperties object itself.
*/
public AnomalySecurityMLAnalyticsSettingsProperties
withRequiredDataConnectors(List requiredDataConnectors) {
this.requiredDataConnectors = requiredDataConnectors;
return this;
}
/**
* Get the tactics property: The tactics of the SecurityMLAnalyticsSettings.
*
* @return the tactics value.
*/
public List tactics() {
return this.tactics;
}
/**
* Set the tactics property: The tactics of the SecurityMLAnalyticsSettings.
*
* @param tactics the tactics value to set.
* @return the AnomalySecurityMLAnalyticsSettingsProperties object itself.
*/
public AnomalySecurityMLAnalyticsSettingsProperties withTactics(List tactics) {
this.tactics = tactics;
return this;
}
/**
* Get the techniques property: The techniques of the SecurityMLAnalyticsSettings.
*
* @return the techniques value.
*/
public List techniques() {
return this.techniques;
}
/**
* Set the techniques property: The techniques of the SecurityMLAnalyticsSettings.
*
* @param techniques the techniques value to set.
* @return the AnomalySecurityMLAnalyticsSettingsProperties object itself.
*/
public AnomalySecurityMLAnalyticsSettingsProperties withTechniques(List techniques) {
this.techniques = techniques;
return this;
}
/**
* Get the anomalyVersion property: The anomaly version of the AnomalySecurityMLAnalyticsSettings.
*
* @return the anomalyVersion value.
*/
public String anomalyVersion() {
return this.anomalyVersion;
}
/**
* Set the anomalyVersion property: The anomaly version of the AnomalySecurityMLAnalyticsSettings.
*
* @param anomalyVersion the anomalyVersion value to set.
* @return the AnomalySecurityMLAnalyticsSettingsProperties object itself.
*/
public AnomalySecurityMLAnalyticsSettingsProperties withAnomalyVersion(String anomalyVersion) {
this.anomalyVersion = anomalyVersion;
return this;
}
/**
* Get the customizableObservations property: The customizable observations of the
* AnomalySecurityMLAnalyticsSettings.
*
* @return the customizableObservations value.
*/
public Object customizableObservations() {
return this.customizableObservations;
}
/**
* Set the customizableObservations property: The customizable observations of the
* AnomalySecurityMLAnalyticsSettings.
*
* @param customizableObservations the customizableObservations value to set.
* @return the AnomalySecurityMLAnalyticsSettingsProperties object itself.
*/
public AnomalySecurityMLAnalyticsSettingsProperties withCustomizableObservations(Object customizableObservations) {
this.customizableObservations = customizableObservations;
return this;
}
/**
* Get the frequency property: The frequency that this SecurityMLAnalyticsSettings will be run.
*
* @return the frequency value.
*/
public Duration frequency() {
return this.frequency;
}
/**
* Set the frequency property: The frequency that this SecurityMLAnalyticsSettings will be run.
*
* @param frequency the frequency value to set.
* @return the AnomalySecurityMLAnalyticsSettingsProperties object itself.
*/
public AnomalySecurityMLAnalyticsSettingsProperties withFrequency(Duration frequency) {
this.frequency = frequency;
return this;
}
/**
* Get the settingsStatus property: The anomaly SecurityMLAnalyticsSettings status.
*
* @return the settingsStatus value.
*/
public SettingsStatus settingsStatus() {
return this.settingsStatus;
}
/**
* Set the settingsStatus property: The anomaly SecurityMLAnalyticsSettings status.
*
* @param settingsStatus the settingsStatus value to set.
* @return the AnomalySecurityMLAnalyticsSettingsProperties object itself.
*/
public AnomalySecurityMLAnalyticsSettingsProperties withSettingsStatus(SettingsStatus settingsStatus) {
this.settingsStatus = settingsStatus;
return this;
}
/**
* Get the isDefaultSettings property: Determines whether this anomaly security ml analytics settings is a default
* settings.
*
* @return the isDefaultSettings value.
*/
public boolean isDefaultSettings() {
return this.isDefaultSettings;
}
/**
* Set the isDefaultSettings property: Determines whether this anomaly security ml analytics settings is a default
* settings.
*
* @param isDefaultSettings the isDefaultSettings value to set.
* @return the AnomalySecurityMLAnalyticsSettingsProperties object itself.
*/
public AnomalySecurityMLAnalyticsSettingsProperties withIsDefaultSettings(boolean isDefaultSettings) {
this.isDefaultSettings = isDefaultSettings;
return this;
}
/**
* Get the anomalySettingsVersion property: The anomaly settings version of the Anomaly security ml analytics
* settings that dictates whether job version gets updated or not.
*
* @return the anomalySettingsVersion value.
*/
public Integer anomalySettingsVersion() {
return this.anomalySettingsVersion;
}
/**
* Set the anomalySettingsVersion property: The anomaly settings version of the Anomaly security ml analytics
* settings that dictates whether job version gets updated or not.
*
* @param anomalySettingsVersion the anomalySettingsVersion value to set.
* @return the AnomalySecurityMLAnalyticsSettingsProperties object itself.
*/
public AnomalySecurityMLAnalyticsSettingsProperties withAnomalySettingsVersion(Integer anomalySettingsVersion) {
this.anomalySettingsVersion = anomalySettingsVersion;
return this;
}
/**
* Get the settingsDefinitionId property: The anomaly settings definition Id.
*
* @return the settingsDefinitionId value.
*/
public UUID settingsDefinitionId() {
return this.settingsDefinitionId;
}
/**
* Set the settingsDefinitionId property: The anomaly settings definition Id.
*
* @param settingsDefinitionId the settingsDefinitionId value to set.
* @return the AnomalySecurityMLAnalyticsSettingsProperties object itself.
*/
public AnomalySecurityMLAnalyticsSettingsProperties withSettingsDefinitionId(UUID settingsDefinitionId) {
this.settingsDefinitionId = settingsDefinitionId;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (displayName() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property displayName in model AnomalySecurityMLAnalyticsSettingsProperties"));
}
if (requiredDataConnectors() != null) {
requiredDataConnectors().forEach(e -> e.validate());
}
if (anomalyVersion() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property anomalyVersion in model AnomalySecurityMLAnalyticsSettingsProperties"));
}
if (frequency() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property frequency in model AnomalySecurityMLAnalyticsSettingsProperties"));
}
if (settingsStatus() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property settingsStatus in model AnomalySecurityMLAnalyticsSettingsProperties"));
}
}
private static final ClientLogger LOGGER = new ClientLogger(AnomalySecurityMLAnalyticsSettingsProperties.class);
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("displayName", this.displayName);
jsonWriter.writeBooleanField("enabled", this.enabled);
jsonWriter.writeStringField("anomalyVersion", this.anomalyVersion);
jsonWriter.writeStringField("frequency", CoreUtils.durationToStringWithDays(this.frequency));
jsonWriter.writeStringField("settingsStatus",
this.settingsStatus == null ? null : this.settingsStatus.toString());
jsonWriter.writeBooleanField("isDefaultSettings", this.isDefaultSettings);
jsonWriter.writeStringField("description", this.description);
jsonWriter.writeArrayField("requiredDataConnectors", this.requiredDataConnectors,
(writer, element) -> writer.writeJson(element));
jsonWriter.writeArrayField("tactics", this.tactics,
(writer, element) -> writer.writeString(element == null ? null : element.toString()));
jsonWriter.writeArrayField("techniques", this.techniques, (writer, element) -> writer.writeString(element));
jsonWriter.writeUntypedField("customizableObservations", this.customizableObservations);
jsonWriter.writeNumberField("anomalySettingsVersion", this.anomalySettingsVersion);
jsonWriter.writeStringField("settingsDefinitionId", Objects.toString(this.settingsDefinitionId, null));
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of AnomalySecurityMLAnalyticsSettingsProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of AnomalySecurityMLAnalyticsSettingsProperties if the JsonReader was pointing to an instance
* of it, or null if it was pointing to JSON null.
* @throws IllegalStateException If the deserialized JSON object was missing any required properties.
* @throws IOException If an error occurs while reading the AnomalySecurityMLAnalyticsSettingsProperties.
*/
public static AnomalySecurityMLAnalyticsSettingsProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
AnomalySecurityMLAnalyticsSettingsProperties deserializedAnomalySecurityMLAnalyticsSettingsProperties
= new AnomalySecurityMLAnalyticsSettingsProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("displayName".equals(fieldName)) {
deserializedAnomalySecurityMLAnalyticsSettingsProperties.displayName = reader.getString();
} else if ("enabled".equals(fieldName)) {
deserializedAnomalySecurityMLAnalyticsSettingsProperties.enabled = reader.getBoolean();
} else if ("anomalyVersion".equals(fieldName)) {
deserializedAnomalySecurityMLAnalyticsSettingsProperties.anomalyVersion = reader.getString();
} else if ("frequency".equals(fieldName)) {
deserializedAnomalySecurityMLAnalyticsSettingsProperties.frequency
= reader.getNullable(nonNullReader -> Duration.parse(nonNullReader.getString()));
} else if ("settingsStatus".equals(fieldName)) {
deserializedAnomalySecurityMLAnalyticsSettingsProperties.settingsStatus
= SettingsStatus.fromString(reader.getString());
} else if ("isDefaultSettings".equals(fieldName)) {
deserializedAnomalySecurityMLAnalyticsSettingsProperties.isDefaultSettings = reader.getBoolean();
} else if ("description".equals(fieldName)) {
deserializedAnomalySecurityMLAnalyticsSettingsProperties.description = reader.getString();
} else if ("lastModifiedUtc".equals(fieldName)) {
deserializedAnomalySecurityMLAnalyticsSettingsProperties.lastModifiedUtc = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("requiredDataConnectors".equals(fieldName)) {
List requiredDataConnectors
= reader.readArray(reader1 -> SecurityMLAnalyticsSettingsDataSource.fromJson(reader1));
deserializedAnomalySecurityMLAnalyticsSettingsProperties.requiredDataConnectors
= requiredDataConnectors;
} else if ("tactics".equals(fieldName)) {
List tactics
= reader.readArray(reader1 -> AttackTactic.fromString(reader1.getString()));
deserializedAnomalySecurityMLAnalyticsSettingsProperties.tactics = tactics;
} else if ("techniques".equals(fieldName)) {
List techniques = reader.readArray(reader1 -> reader1.getString());
deserializedAnomalySecurityMLAnalyticsSettingsProperties.techniques = techniques;
} else if ("customizableObservations".equals(fieldName)) {
deserializedAnomalySecurityMLAnalyticsSettingsProperties.customizableObservations
= reader.readUntyped();
} else if ("anomalySettingsVersion".equals(fieldName)) {
deserializedAnomalySecurityMLAnalyticsSettingsProperties.anomalySettingsVersion
= reader.getNullable(JsonReader::getInt);
} else if ("settingsDefinitionId".equals(fieldName)) {
deserializedAnomalySecurityMLAnalyticsSettingsProperties.settingsDefinitionId
= reader.getNullable(nonNullReader -> UUID.fromString(nonNullReader.getString()));
} else {
reader.skipChildren();
}
}
return deserializedAnomalySecurityMLAnalyticsSettingsProperties;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy