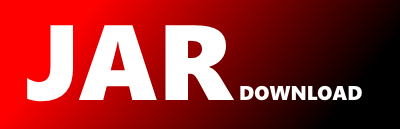
com.azure.resourcemanager.securityinsights.implementation.IncidentPropertiesImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-securityinsights Show documentation
Show all versions of azure-resourcemanager-securityinsights Show documentation
This package contains Microsoft Azure SDK for SecurityInsights Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. API spec for Microsoft.SecurityInsights (Azure Security Insights) resource provider. Package tag package-preview-2021-09.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.securityinsights.implementation;
import com.azure.resourcemanager.securityinsights.fluent.models.IncidentPropertiesInner;
import com.azure.resourcemanager.securityinsights.fluent.models.TeamInformationInner;
import com.azure.resourcemanager.securityinsights.models.IncidentAdditionalData;
import com.azure.resourcemanager.securityinsights.models.IncidentClassification;
import com.azure.resourcemanager.securityinsights.models.IncidentClassificationReason;
import com.azure.resourcemanager.securityinsights.models.IncidentLabel;
import com.azure.resourcemanager.securityinsights.models.IncidentOwnerInfo;
import com.azure.resourcemanager.securityinsights.models.IncidentProperties;
import com.azure.resourcemanager.securityinsights.models.IncidentSeverity;
import com.azure.resourcemanager.securityinsights.models.IncidentStatus;
import com.azure.resourcemanager.securityinsights.models.TeamInformation;
import java.time.OffsetDateTime;
import java.util.Collections;
import java.util.List;
public final class IncidentPropertiesImpl implements IncidentProperties {
private IncidentPropertiesInner innerObject;
private final com.azure.resourcemanager.securityinsights.SecurityInsightsManager serviceManager;
IncidentPropertiesImpl(IncidentPropertiesInner innerObject,
com.azure.resourcemanager.securityinsights.SecurityInsightsManager serviceManager) {
this.innerObject = innerObject;
this.serviceManager = serviceManager;
}
public IncidentAdditionalData additionalData() {
return this.innerModel().additionalData();
}
public IncidentClassification classification() {
return this.innerModel().classification();
}
public String classificationComment() {
return this.innerModel().classificationComment();
}
public IncidentClassificationReason classificationReason() {
return this.innerModel().classificationReason();
}
public OffsetDateTime createdTimeUtc() {
return this.innerModel().createdTimeUtc();
}
public String description() {
return this.innerModel().description();
}
public OffsetDateTime firstActivityTimeUtc() {
return this.innerModel().firstActivityTimeUtc();
}
public String incidentUrl() {
return this.innerModel().incidentUrl();
}
public Integer incidentNumber() {
return this.innerModel().incidentNumber();
}
public List labels() {
List inner = this.innerModel().labels();
if (inner != null) {
return Collections.unmodifiableList(inner);
} else {
return Collections.emptyList();
}
}
public String providerName() {
return this.innerModel().providerName();
}
public String providerIncidentId() {
return this.innerModel().providerIncidentId();
}
public OffsetDateTime lastActivityTimeUtc() {
return this.innerModel().lastActivityTimeUtc();
}
public OffsetDateTime lastModifiedTimeUtc() {
return this.innerModel().lastModifiedTimeUtc();
}
public IncidentOwnerInfo owner() {
return this.innerModel().owner();
}
public List relatedAnalyticRuleIds() {
List inner = this.innerModel().relatedAnalyticRuleIds();
if (inner != null) {
return Collections.unmodifiableList(inner);
} else {
return Collections.emptyList();
}
}
public IncidentSeverity severity() {
return this.innerModel().severity();
}
public IncidentStatus status() {
return this.innerModel().status();
}
public TeamInformation teamInformation() {
TeamInformationInner inner = this.innerModel().teamInformation();
if (inner != null) {
return new TeamInformationImpl(inner, this.manager());
} else {
return null;
}
}
public String title() {
return this.innerModel().title();
}
public IncidentPropertiesInner innerModel() {
return this.innerObject;
}
private com.azure.resourcemanager.securityinsights.SecurityInsightsManager manager() {
return this.serviceManager;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy