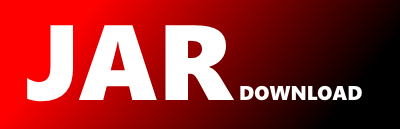
com.azure.resourcemanager.securityinsights.models.Bookmark Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-securityinsights Show documentation
Show all versions of azure-resourcemanager-securityinsights Show documentation
This package contains Microsoft Azure SDK for SecurityInsights Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. API spec for Microsoft.SecurityInsights (Azure Security Insights) resource provider. Package tag package-preview-2021-09.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.securityinsights.models;
import com.azure.core.management.SystemData;
import com.azure.core.util.Context;
import com.azure.resourcemanager.securityinsights.fluent.models.BookmarkInner;
import java.time.OffsetDateTime;
import java.util.List;
/**
* An immutable client-side representation of Bookmark.
*/
public interface Bookmark {
/**
* Gets the id property: Fully qualified resource Id for the resource.
*
* @return the id value.
*/
String id();
/**
* Gets the name property: The name of the resource.
*
* @return the name value.
*/
String name();
/**
* Gets the type property: The type of the resource.
*
* @return the type value.
*/
String type();
/**
* Gets the etag property: Etag of the azure resource.
*
* @return the etag value.
*/
String etag();
/**
* Gets the systemData property: Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
* @return the systemData value.
*/
SystemData systemData();
/**
* Gets the created property: The time the bookmark was created.
*
* @return the created value.
*/
OffsetDateTime created();
/**
* Gets the createdBy property: Describes a user that created the bookmark.
*
* @return the createdBy value.
*/
UserInfo createdBy();
/**
* Gets the displayName property: The display name of the bookmark.
*
* @return the displayName value.
*/
String displayName();
/**
* Gets the labels property: List of labels relevant to this bookmark.
*
* @return the labels value.
*/
List labels();
/**
* Gets the notes property: The notes of the bookmark.
*
* @return the notes value.
*/
String notes();
/**
* Gets the query property: The query of the bookmark.
*
* @return the query value.
*/
String query();
/**
* Gets the queryResult property: The query result of the bookmark.
*
* @return the queryResult value.
*/
String queryResult();
/**
* Gets the updated property: The last time the bookmark was updated.
*
* @return the updated value.
*/
OffsetDateTime updated();
/**
* Gets the updatedBy property: Describes a user that updated the bookmark.
*
* @return the updatedBy value.
*/
UserInfo updatedBy();
/**
* Gets the eventTime property: The bookmark event time.
*
* @return the eventTime value.
*/
OffsetDateTime eventTime();
/**
* Gets the queryStartTime property: The start time for the query.
*
* @return the queryStartTime value.
*/
OffsetDateTime queryStartTime();
/**
* Gets the queryEndTime property: The end time for the query.
*
* @return the queryEndTime value.
*/
OffsetDateTime queryEndTime();
/**
* Gets the incidentInfo property: Describes an incident that relates to bookmark.
*
* @return the incidentInfo value.
*/
IncidentInfo incidentInfo();
/**
* Gets the entityMappings property: Describes the entity mappings of the bookmark.
*
* @return the entityMappings value.
*/
List entityMappings();
/**
* Gets the tactics property: A list of relevant mitre attacks.
*
* @return the tactics value.
*/
List tactics();
/**
* Gets the techniques property: A list of relevant mitre techniques.
*
* @return the techniques value.
*/
List techniques();
/**
* Gets the name of the resource group.
*
* @return the name of the resource group.
*/
String resourceGroupName();
/**
* Gets the inner com.azure.resourcemanager.securityinsights.fluent.models.BookmarkInner object.
*
* @return the inner object.
*/
BookmarkInner innerModel();
/**
* The entirety of the Bookmark definition.
*/
interface Definition
extends DefinitionStages.Blank, DefinitionStages.WithParentResource, DefinitionStages.WithCreate {
}
/**
* The Bookmark definition stages.
*/
interface DefinitionStages {
/**
* The first stage of the Bookmark definition.
*/
interface Blank extends WithParentResource {
}
/**
* The stage of the Bookmark definition allowing to specify parent resource.
*/
interface WithParentResource {
/**
* Specifies resourceGroupName, workspaceName.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param workspaceName The name of the workspace.
* @return the next definition stage.
*/
WithCreate withExistingWorkspace(String resourceGroupName, String workspaceName);
}
/**
* The stage of the Bookmark definition which contains all the minimum required properties for the resource to
* be created, but also allows for any other optional properties to be specified.
*/
interface WithCreate extends DefinitionStages.WithEtag, DefinitionStages.WithCreated,
DefinitionStages.WithCreatedBy, DefinitionStages.WithDisplayName, DefinitionStages.WithLabels,
DefinitionStages.WithNotes, DefinitionStages.WithQuery, DefinitionStages.WithQueryResult,
DefinitionStages.WithUpdated, DefinitionStages.WithUpdatedBy, DefinitionStages.WithEventTime,
DefinitionStages.WithQueryStartTime, DefinitionStages.WithQueryEndTime, DefinitionStages.WithIncidentInfo,
DefinitionStages.WithEntityMappings, DefinitionStages.WithTactics, DefinitionStages.WithTechniques {
/**
* Executes the create request.
*
* @return the created resource.
*/
Bookmark create();
/**
* Executes the create request.
*
* @param context The context to associate with this operation.
* @return the created resource.
*/
Bookmark create(Context context);
}
/**
* The stage of the Bookmark definition allowing to specify etag.
*/
interface WithEtag {
/**
* Specifies the etag property: Etag of the azure resource.
*
* @param etag Etag of the azure resource.
* @return the next definition stage.
*/
WithCreate withEtag(String etag);
}
/**
* The stage of the Bookmark definition allowing to specify created.
*/
interface WithCreated {
/**
* Specifies the created property: The time the bookmark was created.
*
* @param created The time the bookmark was created.
* @return the next definition stage.
*/
WithCreate withCreated(OffsetDateTime created);
}
/**
* The stage of the Bookmark definition allowing to specify createdBy.
*/
interface WithCreatedBy {
/**
* Specifies the createdBy property: Describes a user that created the bookmark.
*
* @param createdBy Describes a user that created the bookmark.
* @return the next definition stage.
*/
WithCreate withCreatedBy(UserInfo createdBy);
}
/**
* The stage of the Bookmark definition allowing to specify displayName.
*/
interface WithDisplayName {
/**
* Specifies the displayName property: The display name of the bookmark.
*
* @param displayName The display name of the bookmark.
* @return the next definition stage.
*/
WithCreate withDisplayName(String displayName);
}
/**
* The stage of the Bookmark definition allowing to specify labels.
*/
interface WithLabels {
/**
* Specifies the labels property: List of labels relevant to this bookmark.
*
* @param labels List of labels relevant to this bookmark.
* @return the next definition stage.
*/
WithCreate withLabels(List labels);
}
/**
* The stage of the Bookmark definition allowing to specify notes.
*/
interface WithNotes {
/**
* Specifies the notes property: The notes of the bookmark.
*
* @param notes The notes of the bookmark.
* @return the next definition stage.
*/
WithCreate withNotes(String notes);
}
/**
* The stage of the Bookmark definition allowing to specify query.
*/
interface WithQuery {
/**
* Specifies the query property: The query of the bookmark..
*
* @param query The query of the bookmark.
* @return the next definition stage.
*/
WithCreate withQuery(String query);
}
/**
* The stage of the Bookmark definition allowing to specify queryResult.
*/
interface WithQueryResult {
/**
* Specifies the queryResult property: The query result of the bookmark..
*
* @param queryResult The query result of the bookmark.
* @return the next definition stage.
*/
WithCreate withQueryResult(String queryResult);
}
/**
* The stage of the Bookmark definition allowing to specify updated.
*/
interface WithUpdated {
/**
* Specifies the updated property: The last time the bookmark was updated.
*
* @param updated The last time the bookmark was updated.
* @return the next definition stage.
*/
WithCreate withUpdated(OffsetDateTime updated);
}
/**
* The stage of the Bookmark definition allowing to specify updatedBy.
*/
interface WithUpdatedBy {
/**
* Specifies the updatedBy property: Describes a user that updated the bookmark.
*
* @param updatedBy Describes a user that updated the bookmark.
* @return the next definition stage.
*/
WithCreate withUpdatedBy(UserInfo updatedBy);
}
/**
* The stage of the Bookmark definition allowing to specify eventTime.
*/
interface WithEventTime {
/**
* Specifies the eventTime property: The bookmark event time.
*
* @param eventTime The bookmark event time.
* @return the next definition stage.
*/
WithCreate withEventTime(OffsetDateTime eventTime);
}
/**
* The stage of the Bookmark definition allowing to specify queryStartTime.
*/
interface WithQueryStartTime {
/**
* Specifies the queryStartTime property: The start time for the query.
*
* @param queryStartTime The start time for the query.
* @return the next definition stage.
*/
WithCreate withQueryStartTime(OffsetDateTime queryStartTime);
}
/**
* The stage of the Bookmark definition allowing to specify queryEndTime.
*/
interface WithQueryEndTime {
/**
* Specifies the queryEndTime property: The end time for the query.
*
* @param queryEndTime The end time for the query.
* @return the next definition stage.
*/
WithCreate withQueryEndTime(OffsetDateTime queryEndTime);
}
/**
* The stage of the Bookmark definition allowing to specify incidentInfo.
*/
interface WithIncidentInfo {
/**
* Specifies the incidentInfo property: Describes an incident that relates to bookmark.
*
* @param incidentInfo Describes an incident that relates to bookmark.
* @return the next definition stage.
*/
WithCreate withIncidentInfo(IncidentInfo incidentInfo);
}
/**
* The stage of the Bookmark definition allowing to specify entityMappings.
*/
interface WithEntityMappings {
/**
* Specifies the entityMappings property: Describes the entity mappings of the bookmark.
*
* @param entityMappings Describes the entity mappings of the bookmark.
* @return the next definition stage.
*/
WithCreate withEntityMappings(List entityMappings);
}
/**
* The stage of the Bookmark definition allowing to specify tactics.
*/
interface WithTactics {
/**
* Specifies the tactics property: A list of relevant mitre attacks.
*
* @param tactics A list of relevant mitre attacks.
* @return the next definition stage.
*/
WithCreate withTactics(List tactics);
}
/**
* The stage of the Bookmark definition allowing to specify techniques.
*/
interface WithTechniques {
/**
* Specifies the techniques property: A list of relevant mitre techniques.
*
* @param techniques A list of relevant mitre techniques.
* @return the next definition stage.
*/
WithCreate withTechniques(List techniques);
}
}
/**
* Begins update for the Bookmark resource.
*
* @return the stage of resource update.
*/
Bookmark.Update update();
/**
* The template for Bookmark update.
*/
interface Update extends UpdateStages.WithEtag, UpdateStages.WithCreated, UpdateStages.WithCreatedBy,
UpdateStages.WithDisplayName, UpdateStages.WithLabels, UpdateStages.WithNotes, UpdateStages.WithQuery,
UpdateStages.WithQueryResult, UpdateStages.WithUpdated, UpdateStages.WithUpdatedBy, UpdateStages.WithEventTime,
UpdateStages.WithQueryStartTime, UpdateStages.WithQueryEndTime, UpdateStages.WithIncidentInfo,
UpdateStages.WithEntityMappings, UpdateStages.WithTactics, UpdateStages.WithTechniques {
/**
* Executes the update request.
*
* @return the updated resource.
*/
Bookmark apply();
/**
* Executes the update request.
*
* @param context The context to associate with this operation.
* @return the updated resource.
*/
Bookmark apply(Context context);
}
/**
* The Bookmark update stages.
*/
interface UpdateStages {
/**
* The stage of the Bookmark update allowing to specify etag.
*/
interface WithEtag {
/**
* Specifies the etag property: Etag of the azure resource.
*
* @param etag Etag of the azure resource.
* @return the next definition stage.
*/
Update withEtag(String etag);
}
/**
* The stage of the Bookmark update allowing to specify created.
*/
interface WithCreated {
/**
* Specifies the created property: The time the bookmark was created.
*
* @param created The time the bookmark was created.
* @return the next definition stage.
*/
Update withCreated(OffsetDateTime created);
}
/**
* The stage of the Bookmark update allowing to specify createdBy.
*/
interface WithCreatedBy {
/**
* Specifies the createdBy property: Describes a user that created the bookmark.
*
* @param createdBy Describes a user that created the bookmark.
* @return the next definition stage.
*/
Update withCreatedBy(UserInfo createdBy);
}
/**
* The stage of the Bookmark update allowing to specify displayName.
*/
interface WithDisplayName {
/**
* Specifies the displayName property: The display name of the bookmark.
*
* @param displayName The display name of the bookmark.
* @return the next definition stage.
*/
Update withDisplayName(String displayName);
}
/**
* The stage of the Bookmark update allowing to specify labels.
*/
interface WithLabels {
/**
* Specifies the labels property: List of labels relevant to this bookmark.
*
* @param labels List of labels relevant to this bookmark.
* @return the next definition stage.
*/
Update withLabels(List labels);
}
/**
* The stage of the Bookmark update allowing to specify notes.
*/
interface WithNotes {
/**
* Specifies the notes property: The notes of the bookmark.
*
* @param notes The notes of the bookmark.
* @return the next definition stage.
*/
Update withNotes(String notes);
}
/**
* The stage of the Bookmark update allowing to specify query.
*/
interface WithQuery {
/**
* Specifies the query property: The query of the bookmark..
*
* @param query The query of the bookmark.
* @return the next definition stage.
*/
Update withQuery(String query);
}
/**
* The stage of the Bookmark update allowing to specify queryResult.
*/
interface WithQueryResult {
/**
* Specifies the queryResult property: The query result of the bookmark..
*
* @param queryResult The query result of the bookmark.
* @return the next definition stage.
*/
Update withQueryResult(String queryResult);
}
/**
* The stage of the Bookmark update allowing to specify updated.
*/
interface WithUpdated {
/**
* Specifies the updated property: The last time the bookmark was updated.
*
* @param updated The last time the bookmark was updated.
* @return the next definition stage.
*/
Update withUpdated(OffsetDateTime updated);
}
/**
* The stage of the Bookmark update allowing to specify updatedBy.
*/
interface WithUpdatedBy {
/**
* Specifies the updatedBy property: Describes a user that updated the bookmark.
*
* @param updatedBy Describes a user that updated the bookmark.
* @return the next definition stage.
*/
Update withUpdatedBy(UserInfo updatedBy);
}
/**
* The stage of the Bookmark update allowing to specify eventTime.
*/
interface WithEventTime {
/**
* Specifies the eventTime property: The bookmark event time.
*
* @param eventTime The bookmark event time.
* @return the next definition stage.
*/
Update withEventTime(OffsetDateTime eventTime);
}
/**
* The stage of the Bookmark update allowing to specify queryStartTime.
*/
interface WithQueryStartTime {
/**
* Specifies the queryStartTime property: The start time for the query.
*
* @param queryStartTime The start time for the query.
* @return the next definition stage.
*/
Update withQueryStartTime(OffsetDateTime queryStartTime);
}
/**
* The stage of the Bookmark update allowing to specify queryEndTime.
*/
interface WithQueryEndTime {
/**
* Specifies the queryEndTime property: The end time for the query.
*
* @param queryEndTime The end time for the query.
* @return the next definition stage.
*/
Update withQueryEndTime(OffsetDateTime queryEndTime);
}
/**
* The stage of the Bookmark update allowing to specify incidentInfo.
*/
interface WithIncidentInfo {
/**
* Specifies the incidentInfo property: Describes an incident that relates to bookmark.
*
* @param incidentInfo Describes an incident that relates to bookmark.
* @return the next definition stage.
*/
Update withIncidentInfo(IncidentInfo incidentInfo);
}
/**
* The stage of the Bookmark update allowing to specify entityMappings.
*/
interface WithEntityMappings {
/**
* Specifies the entityMappings property: Describes the entity mappings of the bookmark.
*
* @param entityMappings Describes the entity mappings of the bookmark.
* @return the next definition stage.
*/
Update withEntityMappings(List entityMappings);
}
/**
* The stage of the Bookmark update allowing to specify tactics.
*/
interface WithTactics {
/**
* Specifies the tactics property: A list of relevant mitre attacks.
*
* @param tactics A list of relevant mitre attacks.
* @return the next definition stage.
*/
Update withTactics(List tactics);
}
/**
* The stage of the Bookmark update allowing to specify techniques.
*/
interface WithTechniques {
/**
* Specifies the techniques property: A list of relevant mitre techniques.
*
* @param techniques A list of relevant mitre techniques.
* @return the next definition stage.
*/
Update withTechniques(List techniques);
}
}
/**
* Refreshes the resource to sync with Azure.
*
* @return the refreshed resource.
*/
Bookmark refresh();
/**
* Refreshes the resource to sync with Azure.
*
* @param context The context to associate with this operation.
* @return the refreshed resource.
*/
Bookmark refresh(Context context);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy