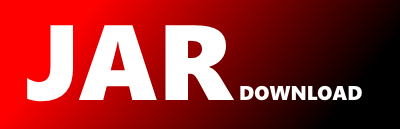
com.azure.resourcemanager.securityinsights.models.CodelessConnectorPollingAuthProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-securityinsights Show documentation
Show all versions of azure-resourcemanager-securityinsights Show documentation
This package contains Microsoft Azure SDK for SecurityInsights Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. API spec for Microsoft.SecurityInsights (Azure Security Insights) resource provider. Package tag package-preview-2021-09.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.securityinsights.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.logging.ClientLogger;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
/**
* Describe the authentication properties needed to successfully authenticate with the server.
*/
@Fluent
public final class CodelessConnectorPollingAuthProperties
implements JsonSerializable {
/*
* The authentication type
*/
private String authType;
/*
* The header name which the token is sent with
*/
private String apiKeyName;
/*
* A prefix send in the header before the actual token
*/
private String apiKeyIdentifier;
/*
* Marks if the key should sent in header
*/
private String isApiKeyInPostPayload;
/*
* Describes the flow name, for example 'AuthCode' for Oauth 2.0
*/
private String flowName;
/*
* The endpoint used to issue a token, used in Oauth 2.0 flow
*/
private String tokenEndpoint;
/*
* The endpoint used to authorize the user, used in Oauth 2.0 flow
*/
private String authorizationEndpoint;
/*
* The query parameters used in authorization request, used in Oauth 2.0 flow
*/
private Object authorizationEndpointQueryParameters;
/*
* The redirect endpoint where we will get the authorization code, used in Oauth 2.0 flow
*/
private String redirectionEndpoint;
/*
* The query headers used in token request, used in Oauth 2.0 flow
*/
private Object tokenEndpointHeaders;
/*
* The query parameters used in token request, used in Oauth 2.0 flow
*/
private Object tokenEndpointQueryParameters;
/*
* Marks if we should send the client secret in header or payload, used in Oauth 2.0 flow
*/
private Boolean isClientSecretInHeader;
/*
* The OAuth token scope
*/
private String scope;
/**
* Creates an instance of CodelessConnectorPollingAuthProperties class.
*/
public CodelessConnectorPollingAuthProperties() {
}
/**
* Get the authType property: The authentication type.
*
* @return the authType value.
*/
public String authType() {
return this.authType;
}
/**
* Set the authType property: The authentication type.
*
* @param authType the authType value to set.
* @return the CodelessConnectorPollingAuthProperties object itself.
*/
public CodelessConnectorPollingAuthProperties withAuthType(String authType) {
this.authType = authType;
return this;
}
/**
* Get the apiKeyName property: The header name which the token is sent with.
*
* @return the apiKeyName value.
*/
public String apiKeyName() {
return this.apiKeyName;
}
/**
* Set the apiKeyName property: The header name which the token is sent with.
*
* @param apiKeyName the apiKeyName value to set.
* @return the CodelessConnectorPollingAuthProperties object itself.
*/
public CodelessConnectorPollingAuthProperties withApiKeyName(String apiKeyName) {
this.apiKeyName = apiKeyName;
return this;
}
/**
* Get the apiKeyIdentifier property: A prefix send in the header before the actual token.
*
* @return the apiKeyIdentifier value.
*/
public String apiKeyIdentifier() {
return this.apiKeyIdentifier;
}
/**
* Set the apiKeyIdentifier property: A prefix send in the header before the actual token.
*
* @param apiKeyIdentifier the apiKeyIdentifier value to set.
* @return the CodelessConnectorPollingAuthProperties object itself.
*/
public CodelessConnectorPollingAuthProperties withApiKeyIdentifier(String apiKeyIdentifier) {
this.apiKeyIdentifier = apiKeyIdentifier;
return this;
}
/**
* Get the isApiKeyInPostPayload property: Marks if the key should sent in header.
*
* @return the isApiKeyInPostPayload value.
*/
public String isApiKeyInPostPayload() {
return this.isApiKeyInPostPayload;
}
/**
* Set the isApiKeyInPostPayload property: Marks if the key should sent in header.
*
* @param isApiKeyInPostPayload the isApiKeyInPostPayload value to set.
* @return the CodelessConnectorPollingAuthProperties object itself.
*/
public CodelessConnectorPollingAuthProperties withIsApiKeyInPostPayload(String isApiKeyInPostPayload) {
this.isApiKeyInPostPayload = isApiKeyInPostPayload;
return this;
}
/**
* Get the flowName property: Describes the flow name, for example 'AuthCode' for Oauth 2.0.
*
* @return the flowName value.
*/
public String flowName() {
return this.flowName;
}
/**
* Set the flowName property: Describes the flow name, for example 'AuthCode' for Oauth 2.0.
*
* @param flowName the flowName value to set.
* @return the CodelessConnectorPollingAuthProperties object itself.
*/
public CodelessConnectorPollingAuthProperties withFlowName(String flowName) {
this.flowName = flowName;
return this;
}
/**
* Get the tokenEndpoint property: The endpoint used to issue a token, used in Oauth 2.0 flow.
*
* @return the tokenEndpoint value.
*/
public String tokenEndpoint() {
return this.tokenEndpoint;
}
/**
* Set the tokenEndpoint property: The endpoint used to issue a token, used in Oauth 2.0 flow.
*
* @param tokenEndpoint the tokenEndpoint value to set.
* @return the CodelessConnectorPollingAuthProperties object itself.
*/
public CodelessConnectorPollingAuthProperties withTokenEndpoint(String tokenEndpoint) {
this.tokenEndpoint = tokenEndpoint;
return this;
}
/**
* Get the authorizationEndpoint property: The endpoint used to authorize the user, used in Oauth 2.0 flow.
*
* @return the authorizationEndpoint value.
*/
public String authorizationEndpoint() {
return this.authorizationEndpoint;
}
/**
* Set the authorizationEndpoint property: The endpoint used to authorize the user, used in Oauth 2.0 flow.
*
* @param authorizationEndpoint the authorizationEndpoint value to set.
* @return the CodelessConnectorPollingAuthProperties object itself.
*/
public CodelessConnectorPollingAuthProperties withAuthorizationEndpoint(String authorizationEndpoint) {
this.authorizationEndpoint = authorizationEndpoint;
return this;
}
/**
* Get the authorizationEndpointQueryParameters property: The query parameters used in authorization request, used
* in Oauth 2.0 flow.
*
* @return the authorizationEndpointQueryParameters value.
*/
public Object authorizationEndpointQueryParameters() {
return this.authorizationEndpointQueryParameters;
}
/**
* Set the authorizationEndpointQueryParameters property: The query parameters used in authorization request, used
* in Oauth 2.0 flow.
*
* @param authorizationEndpointQueryParameters the authorizationEndpointQueryParameters value to set.
* @return the CodelessConnectorPollingAuthProperties object itself.
*/
public CodelessConnectorPollingAuthProperties
withAuthorizationEndpointQueryParameters(Object authorizationEndpointQueryParameters) {
this.authorizationEndpointQueryParameters = authorizationEndpointQueryParameters;
return this;
}
/**
* Get the redirectionEndpoint property: The redirect endpoint where we will get the authorization code, used in
* Oauth 2.0 flow.
*
* @return the redirectionEndpoint value.
*/
public String redirectionEndpoint() {
return this.redirectionEndpoint;
}
/**
* Set the redirectionEndpoint property: The redirect endpoint where we will get the authorization code, used in
* Oauth 2.0 flow.
*
* @param redirectionEndpoint the redirectionEndpoint value to set.
* @return the CodelessConnectorPollingAuthProperties object itself.
*/
public CodelessConnectorPollingAuthProperties withRedirectionEndpoint(String redirectionEndpoint) {
this.redirectionEndpoint = redirectionEndpoint;
return this;
}
/**
* Get the tokenEndpointHeaders property: The query headers used in token request, used in Oauth 2.0 flow.
*
* @return the tokenEndpointHeaders value.
*/
public Object tokenEndpointHeaders() {
return this.tokenEndpointHeaders;
}
/**
* Set the tokenEndpointHeaders property: The query headers used in token request, used in Oauth 2.0 flow.
*
* @param tokenEndpointHeaders the tokenEndpointHeaders value to set.
* @return the CodelessConnectorPollingAuthProperties object itself.
*/
public CodelessConnectorPollingAuthProperties withTokenEndpointHeaders(Object tokenEndpointHeaders) {
this.tokenEndpointHeaders = tokenEndpointHeaders;
return this;
}
/**
* Get the tokenEndpointQueryParameters property: The query parameters used in token request, used in Oauth 2.0
* flow.
*
* @return the tokenEndpointQueryParameters value.
*/
public Object tokenEndpointQueryParameters() {
return this.tokenEndpointQueryParameters;
}
/**
* Set the tokenEndpointQueryParameters property: The query parameters used in token request, used in Oauth 2.0
* flow.
*
* @param tokenEndpointQueryParameters the tokenEndpointQueryParameters value to set.
* @return the CodelessConnectorPollingAuthProperties object itself.
*/
public CodelessConnectorPollingAuthProperties
withTokenEndpointQueryParameters(Object tokenEndpointQueryParameters) {
this.tokenEndpointQueryParameters = tokenEndpointQueryParameters;
return this;
}
/**
* Get the isClientSecretInHeader property: Marks if we should send the client secret in header or payload, used in
* Oauth 2.0 flow.
*
* @return the isClientSecretInHeader value.
*/
public Boolean isClientSecretInHeader() {
return this.isClientSecretInHeader;
}
/**
* Set the isClientSecretInHeader property: Marks if we should send the client secret in header or payload, used in
* Oauth 2.0 flow.
*
* @param isClientSecretInHeader the isClientSecretInHeader value to set.
* @return the CodelessConnectorPollingAuthProperties object itself.
*/
public CodelessConnectorPollingAuthProperties withIsClientSecretInHeader(Boolean isClientSecretInHeader) {
this.isClientSecretInHeader = isClientSecretInHeader;
return this;
}
/**
* Get the scope property: The OAuth token scope.
*
* @return the scope value.
*/
public String scope() {
return this.scope;
}
/**
* Set the scope property: The OAuth token scope.
*
* @param scope the scope value to set.
* @return the CodelessConnectorPollingAuthProperties object itself.
*/
public CodelessConnectorPollingAuthProperties withScope(String scope) {
this.scope = scope;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (authType() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property authType in model CodelessConnectorPollingAuthProperties"));
}
}
private static final ClientLogger LOGGER = new ClientLogger(CodelessConnectorPollingAuthProperties.class);
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("authType", this.authType);
jsonWriter.writeStringField("apiKeyName", this.apiKeyName);
jsonWriter.writeStringField("apiKeyIdentifier", this.apiKeyIdentifier);
jsonWriter.writeStringField("isApiKeyInPostPayload", this.isApiKeyInPostPayload);
jsonWriter.writeStringField("flowName", this.flowName);
jsonWriter.writeStringField("tokenEndpoint", this.tokenEndpoint);
jsonWriter.writeStringField("authorizationEndpoint", this.authorizationEndpoint);
jsonWriter.writeUntypedField("authorizationEndpointQueryParameters", this.authorizationEndpointQueryParameters);
jsonWriter.writeStringField("redirectionEndpoint", this.redirectionEndpoint);
jsonWriter.writeUntypedField("tokenEndpointHeaders", this.tokenEndpointHeaders);
jsonWriter.writeUntypedField("tokenEndpointQueryParameters", this.tokenEndpointQueryParameters);
jsonWriter.writeBooleanField("isClientSecretInHeader", this.isClientSecretInHeader);
jsonWriter.writeStringField("scope", this.scope);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of CodelessConnectorPollingAuthProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of CodelessConnectorPollingAuthProperties if the JsonReader was pointing to an instance of
* it, or null if it was pointing to JSON null.
* @throws IllegalStateException If the deserialized JSON object was missing any required properties.
* @throws IOException If an error occurs while reading the CodelessConnectorPollingAuthProperties.
*/
public static CodelessConnectorPollingAuthProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
CodelessConnectorPollingAuthProperties deserializedCodelessConnectorPollingAuthProperties
= new CodelessConnectorPollingAuthProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("authType".equals(fieldName)) {
deserializedCodelessConnectorPollingAuthProperties.authType = reader.getString();
} else if ("apiKeyName".equals(fieldName)) {
deserializedCodelessConnectorPollingAuthProperties.apiKeyName = reader.getString();
} else if ("apiKeyIdentifier".equals(fieldName)) {
deserializedCodelessConnectorPollingAuthProperties.apiKeyIdentifier = reader.getString();
} else if ("isApiKeyInPostPayload".equals(fieldName)) {
deserializedCodelessConnectorPollingAuthProperties.isApiKeyInPostPayload = reader.getString();
} else if ("flowName".equals(fieldName)) {
deserializedCodelessConnectorPollingAuthProperties.flowName = reader.getString();
} else if ("tokenEndpoint".equals(fieldName)) {
deserializedCodelessConnectorPollingAuthProperties.tokenEndpoint = reader.getString();
} else if ("authorizationEndpoint".equals(fieldName)) {
deserializedCodelessConnectorPollingAuthProperties.authorizationEndpoint = reader.getString();
} else if ("authorizationEndpointQueryParameters".equals(fieldName)) {
deserializedCodelessConnectorPollingAuthProperties.authorizationEndpointQueryParameters
= reader.readUntyped();
} else if ("redirectionEndpoint".equals(fieldName)) {
deserializedCodelessConnectorPollingAuthProperties.redirectionEndpoint = reader.getString();
} else if ("tokenEndpointHeaders".equals(fieldName)) {
deserializedCodelessConnectorPollingAuthProperties.tokenEndpointHeaders = reader.readUntyped();
} else if ("tokenEndpointQueryParameters".equals(fieldName)) {
deserializedCodelessConnectorPollingAuthProperties.tokenEndpointQueryParameters
= reader.readUntyped();
} else if ("isClientSecretInHeader".equals(fieldName)) {
deserializedCodelessConnectorPollingAuthProperties.isClientSecretInHeader
= reader.getNullable(JsonReader::getBoolean);
} else if ("scope".equals(fieldName)) {
deserializedCodelessConnectorPollingAuthProperties.scope = reader.getString();
} else {
reader.skipChildren();
}
}
return deserializedCodelessConnectorPollingAuthProperties;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy