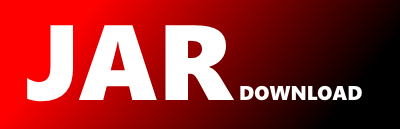
com.azure.resourcemanager.securityinsights.models.CodelessConnectorPollingPagingProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-securityinsights Show documentation
Show all versions of azure-resourcemanager-securityinsights Show documentation
This package contains Microsoft Azure SDK for SecurityInsights Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. API spec for Microsoft.SecurityInsights (Azure Security Insights) resource provider. Package tag package-preview-2021-09.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.securityinsights.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.logging.ClientLogger;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
/**
* Describe the properties needed to make a pagination call.
*/
@Fluent
public final class CodelessConnectorPollingPagingProperties
implements JsonSerializable {
/*
* Describes the type. could be 'None', 'PageToken', 'PageCount', 'TimeStamp'
*/
private String pagingType;
/*
* Defines the name of a next page attribute
*/
private String nextPageParaName;
/*
* Defines the path to a next page token JSON
*/
private String nextPageTokenJsonPath;
/*
* Defines the path to a page count attribute
*/
private String pageCountAttributePath;
/*
* Defines the path to a page total count attribute
*/
private String pageTotalCountAttributePath;
/*
* Defines the path to a paging time stamp attribute
*/
private String pageTimestampAttributePath;
/*
* Determines whether to search for the latest time stamp in the events list
*/
private String searchTheLatestTimestampFromEventsList;
/*
* Defines the name of the page size parameter
*/
private String pageSizeParaName;
/*
* Defines the paging size
*/
private Integer pageSize;
/**
* Creates an instance of CodelessConnectorPollingPagingProperties class.
*/
public CodelessConnectorPollingPagingProperties() {
}
/**
* Get the pagingType property: Describes the type. could be 'None', 'PageToken', 'PageCount', 'TimeStamp'.
*
* @return the pagingType value.
*/
public String pagingType() {
return this.pagingType;
}
/**
* Set the pagingType property: Describes the type. could be 'None', 'PageToken', 'PageCount', 'TimeStamp'.
*
* @param pagingType the pagingType value to set.
* @return the CodelessConnectorPollingPagingProperties object itself.
*/
public CodelessConnectorPollingPagingProperties withPagingType(String pagingType) {
this.pagingType = pagingType;
return this;
}
/**
* Get the nextPageParaName property: Defines the name of a next page attribute.
*
* @return the nextPageParaName value.
*/
public String nextPageParaName() {
return this.nextPageParaName;
}
/**
* Set the nextPageParaName property: Defines the name of a next page attribute.
*
* @param nextPageParaName the nextPageParaName value to set.
* @return the CodelessConnectorPollingPagingProperties object itself.
*/
public CodelessConnectorPollingPagingProperties withNextPageParaName(String nextPageParaName) {
this.nextPageParaName = nextPageParaName;
return this;
}
/**
* Get the nextPageTokenJsonPath property: Defines the path to a next page token JSON.
*
* @return the nextPageTokenJsonPath value.
*/
public String nextPageTokenJsonPath() {
return this.nextPageTokenJsonPath;
}
/**
* Set the nextPageTokenJsonPath property: Defines the path to a next page token JSON.
*
* @param nextPageTokenJsonPath the nextPageTokenJsonPath value to set.
* @return the CodelessConnectorPollingPagingProperties object itself.
*/
public CodelessConnectorPollingPagingProperties withNextPageTokenJsonPath(String nextPageTokenJsonPath) {
this.nextPageTokenJsonPath = nextPageTokenJsonPath;
return this;
}
/**
* Get the pageCountAttributePath property: Defines the path to a page count attribute.
*
* @return the pageCountAttributePath value.
*/
public String pageCountAttributePath() {
return this.pageCountAttributePath;
}
/**
* Set the pageCountAttributePath property: Defines the path to a page count attribute.
*
* @param pageCountAttributePath the pageCountAttributePath value to set.
* @return the CodelessConnectorPollingPagingProperties object itself.
*/
public CodelessConnectorPollingPagingProperties withPageCountAttributePath(String pageCountAttributePath) {
this.pageCountAttributePath = pageCountAttributePath;
return this;
}
/**
* Get the pageTotalCountAttributePath property: Defines the path to a page total count attribute.
*
* @return the pageTotalCountAttributePath value.
*/
public String pageTotalCountAttributePath() {
return this.pageTotalCountAttributePath;
}
/**
* Set the pageTotalCountAttributePath property: Defines the path to a page total count attribute.
*
* @param pageTotalCountAttributePath the pageTotalCountAttributePath value to set.
* @return the CodelessConnectorPollingPagingProperties object itself.
*/
public CodelessConnectorPollingPagingProperties
withPageTotalCountAttributePath(String pageTotalCountAttributePath) {
this.pageTotalCountAttributePath = pageTotalCountAttributePath;
return this;
}
/**
* Get the pageTimestampAttributePath property: Defines the path to a paging time stamp attribute.
*
* @return the pageTimestampAttributePath value.
*/
public String pageTimestampAttributePath() {
return this.pageTimestampAttributePath;
}
/**
* Set the pageTimestampAttributePath property: Defines the path to a paging time stamp attribute.
*
* @param pageTimestampAttributePath the pageTimestampAttributePath value to set.
* @return the CodelessConnectorPollingPagingProperties object itself.
*/
public CodelessConnectorPollingPagingProperties withPageTimestampAttributePath(String pageTimestampAttributePath) {
this.pageTimestampAttributePath = pageTimestampAttributePath;
return this;
}
/**
* Get the searchTheLatestTimestampFromEventsList property: Determines whether to search for the latest time stamp
* in the events list.
*
* @return the searchTheLatestTimestampFromEventsList value.
*/
public String searchTheLatestTimestampFromEventsList() {
return this.searchTheLatestTimestampFromEventsList;
}
/**
* Set the searchTheLatestTimestampFromEventsList property: Determines whether to search for the latest time stamp
* in the events list.
*
* @param searchTheLatestTimestampFromEventsList the searchTheLatestTimestampFromEventsList value to set.
* @return the CodelessConnectorPollingPagingProperties object itself.
*/
public CodelessConnectorPollingPagingProperties
withSearchTheLatestTimestampFromEventsList(String searchTheLatestTimestampFromEventsList) {
this.searchTheLatestTimestampFromEventsList = searchTheLatestTimestampFromEventsList;
return this;
}
/**
* Get the pageSizeParaName property: Defines the name of the page size parameter.
*
* @return the pageSizeParaName value.
*/
public String pageSizeParaName() {
return this.pageSizeParaName;
}
/**
* Set the pageSizeParaName property: Defines the name of the page size parameter.
*
* @param pageSizeParaName the pageSizeParaName value to set.
* @return the CodelessConnectorPollingPagingProperties object itself.
*/
public CodelessConnectorPollingPagingProperties withPageSizeParaName(String pageSizeParaName) {
this.pageSizeParaName = pageSizeParaName;
return this;
}
/**
* Get the pageSize property: Defines the paging size.
*
* @return the pageSize value.
*/
public Integer pageSize() {
return this.pageSize;
}
/**
* Set the pageSize property: Defines the paging size.
*
* @param pageSize the pageSize value to set.
* @return the CodelessConnectorPollingPagingProperties object itself.
*/
public CodelessConnectorPollingPagingProperties withPageSize(Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (pagingType() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property pagingType in model CodelessConnectorPollingPagingProperties"));
}
}
private static final ClientLogger LOGGER = new ClientLogger(CodelessConnectorPollingPagingProperties.class);
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("pagingType", this.pagingType);
jsonWriter.writeStringField("nextPageParaName", this.nextPageParaName);
jsonWriter.writeStringField("nextPageTokenJsonPath", this.nextPageTokenJsonPath);
jsonWriter.writeStringField("pageCountAttributePath", this.pageCountAttributePath);
jsonWriter.writeStringField("pageTotalCountAttributePath", this.pageTotalCountAttributePath);
jsonWriter.writeStringField("pageTimeStampAttributePath", this.pageTimestampAttributePath);
jsonWriter.writeStringField("searchTheLatestTimeStampFromEventsList",
this.searchTheLatestTimestampFromEventsList);
jsonWriter.writeStringField("pageSizeParaName", this.pageSizeParaName);
jsonWriter.writeNumberField("pageSize", this.pageSize);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of CodelessConnectorPollingPagingProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of CodelessConnectorPollingPagingProperties if the JsonReader was pointing to an instance of
* it, or null if it was pointing to JSON null.
* @throws IllegalStateException If the deserialized JSON object was missing any required properties.
* @throws IOException If an error occurs while reading the CodelessConnectorPollingPagingProperties.
*/
public static CodelessConnectorPollingPagingProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
CodelessConnectorPollingPagingProperties deserializedCodelessConnectorPollingPagingProperties
= new CodelessConnectorPollingPagingProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("pagingType".equals(fieldName)) {
deserializedCodelessConnectorPollingPagingProperties.pagingType = reader.getString();
} else if ("nextPageParaName".equals(fieldName)) {
deserializedCodelessConnectorPollingPagingProperties.nextPageParaName = reader.getString();
} else if ("nextPageTokenJsonPath".equals(fieldName)) {
deserializedCodelessConnectorPollingPagingProperties.nextPageTokenJsonPath = reader.getString();
} else if ("pageCountAttributePath".equals(fieldName)) {
deserializedCodelessConnectorPollingPagingProperties.pageCountAttributePath = reader.getString();
} else if ("pageTotalCountAttributePath".equals(fieldName)) {
deserializedCodelessConnectorPollingPagingProperties.pageTotalCountAttributePath
= reader.getString();
} else if ("pageTimeStampAttributePath".equals(fieldName)) {
deserializedCodelessConnectorPollingPagingProperties.pageTimestampAttributePath
= reader.getString();
} else if ("searchTheLatestTimeStampFromEventsList".equals(fieldName)) {
deserializedCodelessConnectorPollingPagingProperties.searchTheLatestTimestampFromEventsList
= reader.getString();
} else if ("pageSizeParaName".equals(fieldName)) {
deserializedCodelessConnectorPollingPagingProperties.pageSizeParaName = reader.getString();
} else if ("pageSize".equals(fieldName)) {
deserializedCodelessConnectorPollingPagingProperties.pageSize
= reader.getNullable(JsonReader::getInt);
} else {
reader.skipChildren();
}
}
return deserializedCodelessConnectorPollingPagingProperties;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy