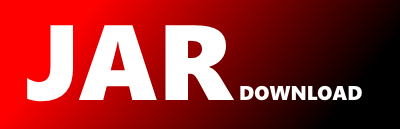
com.azure.resourcemanager.securityinsights.models.Deployment Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-securityinsights Show documentation
Show all versions of azure-resourcemanager-securityinsights Show documentation
This package contains Microsoft Azure SDK for SecurityInsights Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. API spec for Microsoft.SecurityInsights (Azure Security Insights) resource provider. Package tag package-preview-2021-09.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.securityinsights.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.CoreUtils;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.time.OffsetDateTime;
import java.time.format.DateTimeFormatter;
/**
* Description about a deployment.
*/
@Fluent
public final class Deployment implements JsonSerializable {
/*
* Deployment identifier.
*/
private String deploymentId;
/*
* Current status of the deployment.
*/
private DeploymentState deploymentState;
/*
* The outcome of the deployment.
*/
private DeploymentResult deploymentResult;
/*
* The time when the deployment finished.
*/
private OffsetDateTime deploymentTime;
/*
* Url to access repository action logs.
*/
private String deploymentLogsUrl;
/**
* Creates an instance of Deployment class.
*/
public Deployment() {
}
/**
* Get the deploymentId property: Deployment identifier.
*
* @return the deploymentId value.
*/
public String deploymentId() {
return this.deploymentId;
}
/**
* Set the deploymentId property: Deployment identifier.
*
* @param deploymentId the deploymentId value to set.
* @return the Deployment object itself.
*/
public Deployment withDeploymentId(String deploymentId) {
this.deploymentId = deploymentId;
return this;
}
/**
* Get the deploymentState property: Current status of the deployment.
*
* @return the deploymentState value.
*/
public DeploymentState deploymentState() {
return this.deploymentState;
}
/**
* Set the deploymentState property: Current status of the deployment.
*
* @param deploymentState the deploymentState value to set.
* @return the Deployment object itself.
*/
public Deployment withDeploymentState(DeploymentState deploymentState) {
this.deploymentState = deploymentState;
return this;
}
/**
* Get the deploymentResult property: The outcome of the deployment.
*
* @return the deploymentResult value.
*/
public DeploymentResult deploymentResult() {
return this.deploymentResult;
}
/**
* Set the deploymentResult property: The outcome of the deployment.
*
* @param deploymentResult the deploymentResult value to set.
* @return the Deployment object itself.
*/
public Deployment withDeploymentResult(DeploymentResult deploymentResult) {
this.deploymentResult = deploymentResult;
return this;
}
/**
* Get the deploymentTime property: The time when the deployment finished.
*
* @return the deploymentTime value.
*/
public OffsetDateTime deploymentTime() {
return this.deploymentTime;
}
/**
* Set the deploymentTime property: The time when the deployment finished.
*
* @param deploymentTime the deploymentTime value to set.
* @return the Deployment object itself.
*/
public Deployment withDeploymentTime(OffsetDateTime deploymentTime) {
this.deploymentTime = deploymentTime;
return this;
}
/**
* Get the deploymentLogsUrl property: Url to access repository action logs.
*
* @return the deploymentLogsUrl value.
*/
public String deploymentLogsUrl() {
return this.deploymentLogsUrl;
}
/**
* Set the deploymentLogsUrl property: Url to access repository action logs.
*
* @param deploymentLogsUrl the deploymentLogsUrl value to set.
* @return the Deployment object itself.
*/
public Deployment withDeploymentLogsUrl(String deploymentLogsUrl) {
this.deploymentLogsUrl = deploymentLogsUrl;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("deploymentId", this.deploymentId);
jsonWriter.writeStringField("deploymentState",
this.deploymentState == null ? null : this.deploymentState.toString());
jsonWriter.writeStringField("deploymentResult",
this.deploymentResult == null ? null : this.deploymentResult.toString());
jsonWriter.writeStringField("deploymentTime",
this.deploymentTime == null ? null : DateTimeFormatter.ISO_OFFSET_DATE_TIME.format(this.deploymentTime));
jsonWriter.writeStringField("deploymentLogsUrl", this.deploymentLogsUrl);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of Deployment from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of Deployment if the JsonReader was pointing to an instance of it, or null if it was pointing
* to JSON null.
* @throws IOException If an error occurs while reading the Deployment.
*/
public static Deployment fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
Deployment deserializedDeployment = new Deployment();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("deploymentId".equals(fieldName)) {
deserializedDeployment.deploymentId = reader.getString();
} else if ("deploymentState".equals(fieldName)) {
deserializedDeployment.deploymentState = DeploymentState.fromString(reader.getString());
} else if ("deploymentResult".equals(fieldName)) {
deserializedDeployment.deploymentResult = DeploymentResult.fromString(reader.getString());
} else if ("deploymentTime".equals(fieldName)) {
deserializedDeployment.deploymentTime = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("deploymentLogsUrl".equals(fieldName)) {
deserializedDeployment.deploymentLogsUrl = reader.getString();
} else {
reader.skipChildren();
}
}
return deserializedDeployment;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy