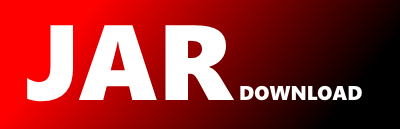
com.azure.resourcemanager.securityinsights.models.Incident Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-securityinsights Show documentation
Show all versions of azure-resourcemanager-securityinsights Show documentation
This package contains Microsoft Azure SDK for SecurityInsights Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. API spec for Microsoft.SecurityInsights (Azure Security Insights) resource provider. Package tag package-preview-2021-09.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.securityinsights.models;
import com.azure.core.http.rest.Response;
import com.azure.core.management.SystemData;
import com.azure.core.util.Context;
import com.azure.resourcemanager.securityinsights.fluent.models.IncidentInner;
import com.azure.resourcemanager.securityinsights.fluent.models.TeamInformationInner;
import java.time.OffsetDateTime;
import java.util.List;
/**
* An immutable client-side representation of Incident.
*/
public interface Incident {
/**
* Gets the id property: Fully qualified resource Id for the resource.
*
* @return the id value.
*/
String id();
/**
* Gets the name property: The name of the resource.
*
* @return the name value.
*/
String name();
/**
* Gets the type property: The type of the resource.
*
* @return the type value.
*/
String type();
/**
* Gets the etag property: Etag of the azure resource.
*
* @return the etag value.
*/
String etag();
/**
* Gets the systemData property: Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
* @return the systemData value.
*/
SystemData systemData();
/**
* Gets the additionalData property: Additional data on the incident.
*
* @return the additionalData value.
*/
IncidentAdditionalData additionalData();
/**
* Gets the classification property: The reason the incident was closed.
*
* @return the classification value.
*/
IncidentClassification classification();
/**
* Gets the classificationComment property: Describes the reason the incident was closed.
*
* @return the classificationComment value.
*/
String classificationComment();
/**
* Gets the classificationReason property: The classification reason the incident was closed with.
*
* @return the classificationReason value.
*/
IncidentClassificationReason classificationReason();
/**
* Gets the createdTimeUtc property: The time the incident was created.
*
* @return the createdTimeUtc value.
*/
OffsetDateTime createdTimeUtc();
/**
* Gets the description property: The description of the incident.
*
* @return the description value.
*/
String description();
/**
* Gets the firstActivityTimeUtc property: The time of the first activity in the incident.
*
* @return the firstActivityTimeUtc value.
*/
OffsetDateTime firstActivityTimeUtc();
/**
* Gets the incidentUrl property: The deep-link url to the incident in Azure portal.
*
* @return the incidentUrl value.
*/
String incidentUrl();
/**
* Gets the incidentNumber property: A sequential number.
*
* @return the incidentNumber value.
*/
Integer incidentNumber();
/**
* Gets the labels property: List of labels relevant to this incident.
*
* @return the labels value.
*/
List labels();
/**
* Gets the providerName property: The name of the source provider that generated the incident.
*
* @return the providerName value.
*/
String providerName();
/**
* Gets the providerIncidentId property: The incident ID assigned by the incident provider.
*
* @return the providerIncidentId value.
*/
String providerIncidentId();
/**
* Gets the lastActivityTimeUtc property: The time of the last activity in the incident.
*
* @return the lastActivityTimeUtc value.
*/
OffsetDateTime lastActivityTimeUtc();
/**
* Gets the lastModifiedTimeUtc property: The last time the incident was updated.
*
* @return the lastModifiedTimeUtc value.
*/
OffsetDateTime lastModifiedTimeUtc();
/**
* Gets the owner property: Describes a user that the incident is assigned to.
*
* @return the owner value.
*/
IncidentOwnerInfo owner();
/**
* Gets the relatedAnalyticRuleIds property: List of resource ids of Analytic rules related to the incident.
*
* @return the relatedAnalyticRuleIds value.
*/
List relatedAnalyticRuleIds();
/**
* Gets the severity property: The severity of the incident.
*
* @return the severity value.
*/
IncidentSeverity severity();
/**
* Gets the status property: The status of the incident.
*
* @return the status value.
*/
IncidentStatus status();
/**
* Gets the teamInformation property: Describes a team for the incident.
*
* @return the teamInformation value.
*/
TeamInformation teamInformation();
/**
* Gets the title property: The title of the incident.
*
* @return the title value.
*/
String title();
/**
* Gets the name of the resource group.
*
* @return the name of the resource group.
*/
String resourceGroupName();
/**
* Gets the inner com.azure.resourcemanager.securityinsights.fluent.models.IncidentInner object.
*
* @return the inner object.
*/
IncidentInner innerModel();
/**
* The entirety of the Incident definition.
*/
interface Definition
extends DefinitionStages.Blank, DefinitionStages.WithParentResource, DefinitionStages.WithCreate {
}
/**
* The Incident definition stages.
*/
interface DefinitionStages {
/**
* The first stage of the Incident definition.
*/
interface Blank extends WithParentResource {
}
/**
* The stage of the Incident definition allowing to specify parent resource.
*/
interface WithParentResource {
/**
* Specifies resourceGroupName, workspaceName.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param workspaceName The name of the workspace.
* @return the next definition stage.
*/
WithCreate withExistingWorkspace(String resourceGroupName, String workspaceName);
}
/**
* The stage of the Incident definition which contains all the minimum required properties for the resource to
* be created, but also allows for any other optional properties to be specified.
*/
interface WithCreate extends DefinitionStages.WithEtag, DefinitionStages.WithClassification,
DefinitionStages.WithClassificationComment, DefinitionStages.WithClassificationReason,
DefinitionStages.WithDescription, DefinitionStages.WithFirstActivityTimeUtc, DefinitionStages.WithLabels,
DefinitionStages.WithProviderName, DefinitionStages.WithProviderIncidentId,
DefinitionStages.WithLastActivityTimeUtc, DefinitionStages.WithOwner, DefinitionStages.WithSeverity,
DefinitionStages.WithStatus, DefinitionStages.WithTeamInformation, DefinitionStages.WithTitle {
/**
* Executes the create request.
*
* @return the created resource.
*/
Incident create();
/**
* Executes the create request.
*
* @param context The context to associate with this operation.
* @return the created resource.
*/
Incident create(Context context);
}
/**
* The stage of the Incident definition allowing to specify etag.
*/
interface WithEtag {
/**
* Specifies the etag property: Etag of the azure resource.
*
* @param etag Etag of the azure resource.
* @return the next definition stage.
*/
WithCreate withEtag(String etag);
}
/**
* The stage of the Incident definition allowing to specify classification.
*/
interface WithClassification {
/**
* Specifies the classification property: The reason the incident was closed.
*
* @param classification The reason the incident was closed.
* @return the next definition stage.
*/
WithCreate withClassification(IncidentClassification classification);
}
/**
* The stage of the Incident definition allowing to specify classificationComment.
*/
interface WithClassificationComment {
/**
* Specifies the classificationComment property: Describes the reason the incident was closed.
*
* @param classificationComment Describes the reason the incident was closed.
* @return the next definition stage.
*/
WithCreate withClassificationComment(String classificationComment);
}
/**
* The stage of the Incident definition allowing to specify classificationReason.
*/
interface WithClassificationReason {
/**
* Specifies the classificationReason property: The classification reason the incident was closed with.
*
* @param classificationReason The classification reason the incident was closed with.
* @return the next definition stage.
*/
WithCreate withClassificationReason(IncidentClassificationReason classificationReason);
}
/**
* The stage of the Incident definition allowing to specify description.
*/
interface WithDescription {
/**
* Specifies the description property: The description of the incident.
*
* @param description The description of the incident.
* @return the next definition stage.
*/
WithCreate withDescription(String description);
}
/**
* The stage of the Incident definition allowing to specify firstActivityTimeUtc.
*/
interface WithFirstActivityTimeUtc {
/**
* Specifies the firstActivityTimeUtc property: The time of the first activity in the incident.
*
* @param firstActivityTimeUtc The time of the first activity in the incident.
* @return the next definition stage.
*/
WithCreate withFirstActivityTimeUtc(OffsetDateTime firstActivityTimeUtc);
}
/**
* The stage of the Incident definition allowing to specify labels.
*/
interface WithLabels {
/**
* Specifies the labels property: List of labels relevant to this incident.
*
* @param labels List of labels relevant to this incident.
* @return the next definition stage.
*/
WithCreate withLabels(List labels);
}
/**
* The stage of the Incident definition allowing to specify providerName.
*/
interface WithProviderName {
/**
* Specifies the providerName property: The name of the source provider that generated the incident.
*
* @param providerName The name of the source provider that generated the incident.
* @return the next definition stage.
*/
WithCreate withProviderName(String providerName);
}
/**
* The stage of the Incident definition allowing to specify providerIncidentId.
*/
interface WithProviderIncidentId {
/**
* Specifies the providerIncidentId property: The incident ID assigned by the incident provider.
*
* @param providerIncidentId The incident ID assigned by the incident provider.
* @return the next definition stage.
*/
WithCreate withProviderIncidentId(String providerIncidentId);
}
/**
* The stage of the Incident definition allowing to specify lastActivityTimeUtc.
*/
interface WithLastActivityTimeUtc {
/**
* Specifies the lastActivityTimeUtc property: The time of the last activity in the incident.
*
* @param lastActivityTimeUtc The time of the last activity in the incident.
* @return the next definition stage.
*/
WithCreate withLastActivityTimeUtc(OffsetDateTime lastActivityTimeUtc);
}
/**
* The stage of the Incident definition allowing to specify owner.
*/
interface WithOwner {
/**
* Specifies the owner property: Describes a user that the incident is assigned to.
*
* @param owner Describes a user that the incident is assigned to.
* @return the next definition stage.
*/
WithCreate withOwner(IncidentOwnerInfo owner);
}
/**
* The stage of the Incident definition allowing to specify severity.
*/
interface WithSeverity {
/**
* Specifies the severity property: The severity of the incident.
*
* @param severity The severity of the incident.
* @return the next definition stage.
*/
WithCreate withSeverity(IncidentSeverity severity);
}
/**
* The stage of the Incident definition allowing to specify status.
*/
interface WithStatus {
/**
* Specifies the status property: The status of the incident.
*
* @param status The status of the incident.
* @return the next definition stage.
*/
WithCreate withStatus(IncidentStatus status);
}
/**
* The stage of the Incident definition allowing to specify teamInformation.
*/
interface WithTeamInformation {
/**
* Specifies the teamInformation property: Describes a team for the incident.
*
* @param teamInformation Describes a team for the incident.
* @return the next definition stage.
*/
WithCreate withTeamInformation(TeamInformationInner teamInformation);
}
/**
* The stage of the Incident definition allowing to specify title.
*/
interface WithTitle {
/**
* Specifies the title property: The title of the incident.
*
* @param title The title of the incident.
* @return the next definition stage.
*/
WithCreate withTitle(String title);
}
}
/**
* Begins update for the Incident resource.
*
* @return the stage of resource update.
*/
Incident.Update update();
/**
* The template for Incident update.
*/
interface Update extends UpdateStages.WithEtag, UpdateStages.WithClassification,
UpdateStages.WithClassificationComment, UpdateStages.WithClassificationReason, UpdateStages.WithDescription,
UpdateStages.WithFirstActivityTimeUtc, UpdateStages.WithLabels, UpdateStages.WithProviderName,
UpdateStages.WithProviderIncidentId, UpdateStages.WithLastActivityTimeUtc, UpdateStages.WithOwner,
UpdateStages.WithSeverity, UpdateStages.WithStatus, UpdateStages.WithTeamInformation, UpdateStages.WithTitle {
/**
* Executes the update request.
*
* @return the updated resource.
*/
Incident apply();
/**
* Executes the update request.
*
* @param context The context to associate with this operation.
* @return the updated resource.
*/
Incident apply(Context context);
}
/**
* The Incident update stages.
*/
interface UpdateStages {
/**
* The stage of the Incident update allowing to specify etag.
*/
interface WithEtag {
/**
* Specifies the etag property: Etag of the azure resource.
*
* @param etag Etag of the azure resource.
* @return the next definition stage.
*/
Update withEtag(String etag);
}
/**
* The stage of the Incident update allowing to specify classification.
*/
interface WithClassification {
/**
* Specifies the classification property: The reason the incident was closed.
*
* @param classification The reason the incident was closed.
* @return the next definition stage.
*/
Update withClassification(IncidentClassification classification);
}
/**
* The stage of the Incident update allowing to specify classificationComment.
*/
interface WithClassificationComment {
/**
* Specifies the classificationComment property: Describes the reason the incident was closed.
*
* @param classificationComment Describes the reason the incident was closed.
* @return the next definition stage.
*/
Update withClassificationComment(String classificationComment);
}
/**
* The stage of the Incident update allowing to specify classificationReason.
*/
interface WithClassificationReason {
/**
* Specifies the classificationReason property: The classification reason the incident was closed with.
*
* @param classificationReason The classification reason the incident was closed with.
* @return the next definition stage.
*/
Update withClassificationReason(IncidentClassificationReason classificationReason);
}
/**
* The stage of the Incident update allowing to specify description.
*/
interface WithDescription {
/**
* Specifies the description property: The description of the incident.
*
* @param description The description of the incident.
* @return the next definition stage.
*/
Update withDescription(String description);
}
/**
* The stage of the Incident update allowing to specify firstActivityTimeUtc.
*/
interface WithFirstActivityTimeUtc {
/**
* Specifies the firstActivityTimeUtc property: The time of the first activity in the incident.
*
* @param firstActivityTimeUtc The time of the first activity in the incident.
* @return the next definition stage.
*/
Update withFirstActivityTimeUtc(OffsetDateTime firstActivityTimeUtc);
}
/**
* The stage of the Incident update allowing to specify labels.
*/
interface WithLabels {
/**
* Specifies the labels property: List of labels relevant to this incident.
*
* @param labels List of labels relevant to this incident.
* @return the next definition stage.
*/
Update withLabels(List labels);
}
/**
* The stage of the Incident update allowing to specify providerName.
*/
interface WithProviderName {
/**
* Specifies the providerName property: The name of the source provider that generated the incident.
*
* @param providerName The name of the source provider that generated the incident.
* @return the next definition stage.
*/
Update withProviderName(String providerName);
}
/**
* The stage of the Incident update allowing to specify providerIncidentId.
*/
interface WithProviderIncidentId {
/**
* Specifies the providerIncidentId property: The incident ID assigned by the incident provider.
*
* @param providerIncidentId The incident ID assigned by the incident provider.
* @return the next definition stage.
*/
Update withProviderIncidentId(String providerIncidentId);
}
/**
* The stage of the Incident update allowing to specify lastActivityTimeUtc.
*/
interface WithLastActivityTimeUtc {
/**
* Specifies the lastActivityTimeUtc property: The time of the last activity in the incident.
*
* @param lastActivityTimeUtc The time of the last activity in the incident.
* @return the next definition stage.
*/
Update withLastActivityTimeUtc(OffsetDateTime lastActivityTimeUtc);
}
/**
* The stage of the Incident update allowing to specify owner.
*/
interface WithOwner {
/**
* Specifies the owner property: Describes a user that the incident is assigned to.
*
* @param owner Describes a user that the incident is assigned to.
* @return the next definition stage.
*/
Update withOwner(IncidentOwnerInfo owner);
}
/**
* The stage of the Incident update allowing to specify severity.
*/
interface WithSeverity {
/**
* Specifies the severity property: The severity of the incident.
*
* @param severity The severity of the incident.
* @return the next definition stage.
*/
Update withSeverity(IncidentSeverity severity);
}
/**
* The stage of the Incident update allowing to specify status.
*/
interface WithStatus {
/**
* Specifies the status property: The status of the incident.
*
* @param status The status of the incident.
* @return the next definition stage.
*/
Update withStatus(IncidentStatus status);
}
/**
* The stage of the Incident update allowing to specify teamInformation.
*/
interface WithTeamInformation {
/**
* Specifies the teamInformation property: Describes a team for the incident.
*
* @param teamInformation Describes a team for the incident.
* @return the next definition stage.
*/
Update withTeamInformation(TeamInformationInner teamInformation);
}
/**
* The stage of the Incident update allowing to specify title.
*/
interface WithTitle {
/**
* Specifies the title property: The title of the incident.
*
* @param title The title of the incident.
* @return the next definition stage.
*/
Update withTitle(String title);
}
}
/**
* Refreshes the resource to sync with Azure.
*
* @return the refreshed resource.
*/
Incident refresh();
/**
* Refreshes the resource to sync with Azure.
*
* @param context The context to associate with this operation.
* @return the refreshed resource.
*/
Incident refresh(Context context);
/**
* Creates a Microsoft team to investigate the incident by sharing information and insights between participants.
*
* @param teamProperties Team properties.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return describes team information along with {@link Response}.
*/
Response createTeamWithResponse(TeamProperties teamProperties, Context context);
/**
* Creates a Microsoft team to investigate the incident by sharing information and insights between participants.
*
* @param teamProperties Team properties.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return describes team information.
*/
TeamInformation createTeam(TeamProperties teamProperties);
/**
* Gets all incident alerts.
*
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return all incident alerts along with {@link Response}.
*/
Response listAlertsWithResponse(Context context);
/**
* Gets all incident alerts.
*
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return all incident alerts.
*/
IncidentAlertList listAlerts();
/**
* Gets all incident bookmarks.
*
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return all incident bookmarks along with {@link Response}.
*/
Response listBookmarksWithResponse(Context context);
/**
* Gets all incident bookmarks.
*
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return all incident bookmarks.
*/
IncidentBookmarkList listBookmarks();
/**
* Gets all incident related entities.
*
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return all incident related entities along with {@link Response}.
*/
Response listEntitiesWithResponse(Context context);
/**
* Gets all incident related entities.
*
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return all incident related entities.
*/
IncidentEntitiesResponse listEntities();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy