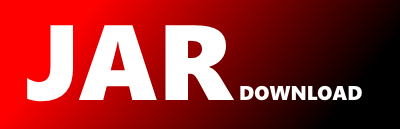
com.azure.resourcemanager.securityinsights.models.IncidentAdditionalData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-securityinsights Show documentation
Show all versions of azure-resourcemanager-securityinsights Show documentation
This package contains Microsoft Azure SDK for SecurityInsights Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. API spec for Microsoft.SecurityInsights (Azure Security Insights) resource provider. Package tag package-preview-2021-09.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.securityinsights.models;
import com.azure.core.annotation.Immutable;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.util.List;
/**
* Incident additional data property bag.
*/
@Immutable
public final class IncidentAdditionalData implements JsonSerializable {
/*
* The number of alerts in the incident
*/
private Integer alertsCount;
/*
* The number of bookmarks in the incident
*/
private Integer bookmarksCount;
/*
* The number of comments in the incident
*/
private Integer commentsCount;
/*
* List of product names of alerts in the incident
*/
private List alertProductNames;
/*
* The provider incident url to the incident in Microsoft 365 Defender portal
*/
private String providerIncidentUrl;
/*
* The tactics associated with incident
*/
private List tactics;
/*
* The techniques associated with incident's tactics'
*/
private List techniques;
/**
* Creates an instance of IncidentAdditionalData class.
*/
public IncidentAdditionalData() {
}
/**
* Get the alertsCount property: The number of alerts in the incident.
*
* @return the alertsCount value.
*/
public Integer alertsCount() {
return this.alertsCount;
}
/**
* Get the bookmarksCount property: The number of bookmarks in the incident.
*
* @return the bookmarksCount value.
*/
public Integer bookmarksCount() {
return this.bookmarksCount;
}
/**
* Get the commentsCount property: The number of comments in the incident.
*
* @return the commentsCount value.
*/
public Integer commentsCount() {
return this.commentsCount;
}
/**
* Get the alertProductNames property: List of product names of alerts in the incident.
*
* @return the alertProductNames value.
*/
public List alertProductNames() {
return this.alertProductNames;
}
/**
* Get the providerIncidentUrl property: The provider incident url to the incident in Microsoft 365 Defender portal.
*
* @return the providerIncidentUrl value.
*/
public String providerIncidentUrl() {
return this.providerIncidentUrl;
}
/**
* Get the tactics property: The tactics associated with incident.
*
* @return the tactics value.
*/
public List tactics() {
return this.tactics;
}
/**
* Get the techniques property: The techniques associated with incident's tactics'.
*
* @return the techniques value.
*/
public List techniques() {
return this.techniques;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of IncidentAdditionalData from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of IncidentAdditionalData if the JsonReader was pointing to an instance of it, or null if it
* was pointing to JSON null.
* @throws IOException If an error occurs while reading the IncidentAdditionalData.
*/
public static IncidentAdditionalData fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
IncidentAdditionalData deserializedIncidentAdditionalData = new IncidentAdditionalData();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("alertsCount".equals(fieldName)) {
deserializedIncidentAdditionalData.alertsCount = reader.getNullable(JsonReader::getInt);
} else if ("bookmarksCount".equals(fieldName)) {
deserializedIncidentAdditionalData.bookmarksCount = reader.getNullable(JsonReader::getInt);
} else if ("commentsCount".equals(fieldName)) {
deserializedIncidentAdditionalData.commentsCount = reader.getNullable(JsonReader::getInt);
} else if ("alertProductNames".equals(fieldName)) {
List alertProductNames = reader.readArray(reader1 -> reader1.getString());
deserializedIncidentAdditionalData.alertProductNames = alertProductNames;
} else if ("providerIncidentUrl".equals(fieldName)) {
deserializedIncidentAdditionalData.providerIncidentUrl = reader.getString();
} else if ("tactics".equals(fieldName)) {
List tactics
= reader.readArray(reader1 -> AttackTactic.fromString(reader1.getString()));
deserializedIncidentAdditionalData.tactics = tactics;
} else if ("techniques".equals(fieldName)) {
List techniques = reader.readArray(reader1 -> reader1.getString());
deserializedIncidentAdditionalData.techniques = techniques;
} else {
reader.skipChildren();
}
}
return deserializedIncidentAdditionalData;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy