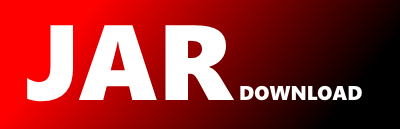
com.azure.resourcemanager.securityinsights.models.ScheduledAlertRuleCommonProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-securityinsights Show documentation
Show all versions of azure-resourcemanager-securityinsights Show documentation
This package contains Microsoft Azure SDK for SecurityInsights Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. API spec for Microsoft.SecurityInsights (Azure Security Insights) resource provider. Package tag package-preview-2021-09.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.securityinsights.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.CoreUtils;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.time.Duration;
import java.util.List;
import java.util.Map;
/**
* Scheduled alert rule template property bag.
*/
@Fluent
public class ScheduledAlertRuleCommonProperties implements JsonSerializable {
/*
* The query that creates alerts for this rule.
*/
private String query;
/*
* The frequency (in ISO 8601 duration format) for this alert rule to run.
*/
private Duration queryFrequency;
/*
* The period (in ISO 8601 duration format) that this alert rule looks at.
*/
private Duration queryPeriod;
/*
* The severity for alerts created by this alert rule.
*/
private AlertSeverity severity;
/*
* The operation against the threshold that triggers alert rule.
*/
private TriggerOperator triggerOperator;
/*
* The threshold triggers this alert rule.
*/
private Integer triggerThreshold;
/*
* The event grouping settings.
*/
private EventGroupingSettings eventGroupingSettings;
/*
* Dictionary of string key-value pairs of columns to be attached to the alert
*/
private Map customDetails;
/*
* Array of the entity mappings of the alert rule
*/
private List entityMappings;
/*
* The alert details override settings
*/
private AlertDetailsOverride alertDetailsOverride;
/**
* Creates an instance of ScheduledAlertRuleCommonProperties class.
*/
public ScheduledAlertRuleCommonProperties() {
}
/**
* Get the query property: The query that creates alerts for this rule.
*
* @return the query value.
*/
public String query() {
return this.query;
}
/**
* Set the query property: The query that creates alerts for this rule.
*
* @param query the query value to set.
* @return the ScheduledAlertRuleCommonProperties object itself.
*/
public ScheduledAlertRuleCommonProperties withQuery(String query) {
this.query = query;
return this;
}
/**
* Get the queryFrequency property: The frequency (in ISO 8601 duration format) for this alert rule to run.
*
* @return the queryFrequency value.
*/
public Duration queryFrequency() {
return this.queryFrequency;
}
/**
* Set the queryFrequency property: The frequency (in ISO 8601 duration format) for this alert rule to run.
*
* @param queryFrequency the queryFrequency value to set.
* @return the ScheduledAlertRuleCommonProperties object itself.
*/
public ScheduledAlertRuleCommonProperties withQueryFrequency(Duration queryFrequency) {
this.queryFrequency = queryFrequency;
return this;
}
/**
* Get the queryPeriod property: The period (in ISO 8601 duration format) that this alert rule looks at.
*
* @return the queryPeriod value.
*/
public Duration queryPeriod() {
return this.queryPeriod;
}
/**
* Set the queryPeriod property: The period (in ISO 8601 duration format) that this alert rule looks at.
*
* @param queryPeriod the queryPeriod value to set.
* @return the ScheduledAlertRuleCommonProperties object itself.
*/
public ScheduledAlertRuleCommonProperties withQueryPeriod(Duration queryPeriod) {
this.queryPeriod = queryPeriod;
return this;
}
/**
* Get the severity property: The severity for alerts created by this alert rule.
*
* @return the severity value.
*/
public AlertSeverity severity() {
return this.severity;
}
/**
* Set the severity property: The severity for alerts created by this alert rule.
*
* @param severity the severity value to set.
* @return the ScheduledAlertRuleCommonProperties object itself.
*/
public ScheduledAlertRuleCommonProperties withSeverity(AlertSeverity severity) {
this.severity = severity;
return this;
}
/**
* Get the triggerOperator property: The operation against the threshold that triggers alert rule.
*
* @return the triggerOperator value.
*/
public TriggerOperator triggerOperator() {
return this.triggerOperator;
}
/**
* Set the triggerOperator property: The operation against the threshold that triggers alert rule.
*
* @param triggerOperator the triggerOperator value to set.
* @return the ScheduledAlertRuleCommonProperties object itself.
*/
public ScheduledAlertRuleCommonProperties withTriggerOperator(TriggerOperator triggerOperator) {
this.triggerOperator = triggerOperator;
return this;
}
/**
* Get the triggerThreshold property: The threshold triggers this alert rule.
*
* @return the triggerThreshold value.
*/
public Integer triggerThreshold() {
return this.triggerThreshold;
}
/**
* Set the triggerThreshold property: The threshold triggers this alert rule.
*
* @param triggerThreshold the triggerThreshold value to set.
* @return the ScheduledAlertRuleCommonProperties object itself.
*/
public ScheduledAlertRuleCommonProperties withTriggerThreshold(Integer triggerThreshold) {
this.triggerThreshold = triggerThreshold;
return this;
}
/**
* Get the eventGroupingSettings property: The event grouping settings.
*
* @return the eventGroupingSettings value.
*/
public EventGroupingSettings eventGroupingSettings() {
return this.eventGroupingSettings;
}
/**
* Set the eventGroupingSettings property: The event grouping settings.
*
* @param eventGroupingSettings the eventGroupingSettings value to set.
* @return the ScheduledAlertRuleCommonProperties object itself.
*/
public ScheduledAlertRuleCommonProperties withEventGroupingSettings(EventGroupingSettings eventGroupingSettings) {
this.eventGroupingSettings = eventGroupingSettings;
return this;
}
/**
* Get the customDetails property: Dictionary of string key-value pairs of columns to be attached to the alert.
*
* @return the customDetails value.
*/
public Map customDetails() {
return this.customDetails;
}
/**
* Set the customDetails property: Dictionary of string key-value pairs of columns to be attached to the alert.
*
* @param customDetails the customDetails value to set.
* @return the ScheduledAlertRuleCommonProperties object itself.
*/
public ScheduledAlertRuleCommonProperties withCustomDetails(Map customDetails) {
this.customDetails = customDetails;
return this;
}
/**
* Get the entityMappings property: Array of the entity mappings of the alert rule.
*
* @return the entityMappings value.
*/
public List entityMappings() {
return this.entityMappings;
}
/**
* Set the entityMappings property: Array of the entity mappings of the alert rule.
*
* @param entityMappings the entityMappings value to set.
* @return the ScheduledAlertRuleCommonProperties object itself.
*/
public ScheduledAlertRuleCommonProperties withEntityMappings(List entityMappings) {
this.entityMappings = entityMappings;
return this;
}
/**
* Get the alertDetailsOverride property: The alert details override settings.
*
* @return the alertDetailsOverride value.
*/
public AlertDetailsOverride alertDetailsOverride() {
return this.alertDetailsOverride;
}
/**
* Set the alertDetailsOverride property: The alert details override settings.
*
* @param alertDetailsOverride the alertDetailsOverride value to set.
* @return the ScheduledAlertRuleCommonProperties object itself.
*/
public ScheduledAlertRuleCommonProperties withAlertDetailsOverride(AlertDetailsOverride alertDetailsOverride) {
this.alertDetailsOverride = alertDetailsOverride;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (eventGroupingSettings() != null) {
eventGroupingSettings().validate();
}
if (entityMappings() != null) {
entityMappings().forEach(e -> e.validate());
}
if (alertDetailsOverride() != null) {
alertDetailsOverride().validate();
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("query", this.query);
jsonWriter.writeStringField("queryFrequency", CoreUtils.durationToStringWithDays(this.queryFrequency));
jsonWriter.writeStringField("queryPeriod", CoreUtils.durationToStringWithDays(this.queryPeriod));
jsonWriter.writeStringField("severity", this.severity == null ? null : this.severity.toString());
jsonWriter.writeStringField("triggerOperator",
this.triggerOperator == null ? null : this.triggerOperator.toString());
jsonWriter.writeNumberField("triggerThreshold", this.triggerThreshold);
jsonWriter.writeJsonField("eventGroupingSettings", this.eventGroupingSettings);
jsonWriter.writeMapField("customDetails", this.customDetails, (writer, element) -> writer.writeString(element));
jsonWriter.writeArrayField("entityMappings", this.entityMappings,
(writer, element) -> writer.writeJson(element));
jsonWriter.writeJsonField("alertDetailsOverride", this.alertDetailsOverride);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of ScheduledAlertRuleCommonProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of ScheduledAlertRuleCommonProperties if the JsonReader was pointing to an instance of it, or
* null if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the ScheduledAlertRuleCommonProperties.
*/
public static ScheduledAlertRuleCommonProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
ScheduledAlertRuleCommonProperties deserializedScheduledAlertRuleCommonProperties
= new ScheduledAlertRuleCommonProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("query".equals(fieldName)) {
deserializedScheduledAlertRuleCommonProperties.query = reader.getString();
} else if ("queryFrequency".equals(fieldName)) {
deserializedScheduledAlertRuleCommonProperties.queryFrequency
= reader.getNullable(nonNullReader -> Duration.parse(nonNullReader.getString()));
} else if ("queryPeriod".equals(fieldName)) {
deserializedScheduledAlertRuleCommonProperties.queryPeriod
= reader.getNullable(nonNullReader -> Duration.parse(nonNullReader.getString()));
} else if ("severity".equals(fieldName)) {
deserializedScheduledAlertRuleCommonProperties.severity
= AlertSeverity.fromString(reader.getString());
} else if ("triggerOperator".equals(fieldName)) {
deserializedScheduledAlertRuleCommonProperties.triggerOperator
= TriggerOperator.fromString(reader.getString());
} else if ("triggerThreshold".equals(fieldName)) {
deserializedScheduledAlertRuleCommonProperties.triggerThreshold
= reader.getNullable(JsonReader::getInt);
} else if ("eventGroupingSettings".equals(fieldName)) {
deserializedScheduledAlertRuleCommonProperties.eventGroupingSettings
= EventGroupingSettings.fromJson(reader);
} else if ("customDetails".equals(fieldName)) {
Map customDetails = reader.readMap(reader1 -> reader1.getString());
deserializedScheduledAlertRuleCommonProperties.customDetails = customDetails;
} else if ("entityMappings".equals(fieldName)) {
List entityMappings = reader.readArray(reader1 -> EntityMapping.fromJson(reader1));
deserializedScheduledAlertRuleCommonProperties.entityMappings = entityMappings;
} else if ("alertDetailsOverride".equals(fieldName)) {
deserializedScheduledAlertRuleCommonProperties.alertDetailsOverride
= AlertDetailsOverride.fromJson(reader);
} else {
reader.skipChildren();
}
}
return deserializedScheduledAlertRuleCommonProperties;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy