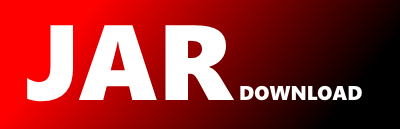
com.azure.resourcemanager.securityinsights.models.ThreatIntelligenceIndicatorModel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-securityinsights Show documentation
Show all versions of azure-resourcemanager-securityinsights Show documentation
This package contains Microsoft Azure SDK for SecurityInsights Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. API spec for Microsoft.SecurityInsights (Azure Security Insights) resource provider. Package tag package-preview-2021-09.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.securityinsights.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.management.SystemData;
import com.azure.json.JsonReader;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.securityinsights.fluent.models.ThreatIntelligenceIndicatorProperties;
import com.azure.resourcemanager.securityinsights.fluent.models.ThreatIntelligenceInformationInner;
import java.io.IOException;
import java.util.List;
import java.util.Map;
/**
* Threat intelligence indicator entity.
*/
@Fluent
public final class ThreatIntelligenceIndicatorModel extends ThreatIntelligenceInformationInner {
/*
* The kind of the entity.
*/
private ThreatIntelligenceResourceKindEnum kind = ThreatIntelligenceResourceKindEnum.INDICATOR;
/*
* Threat Intelligence Entity properties
*/
private ThreatIntelligenceIndicatorProperties innerProperties;
/*
* Azure Resource Manager metadata containing createdBy and modifiedBy information.
*/
private SystemData systemData;
/*
* The type of the resource.
*/
private String type;
/*
* The name of the resource.
*/
private String name;
/*
* Fully qualified resource Id for the resource.
*/
private String id;
/**
* Creates an instance of ThreatIntelligenceIndicatorModel class.
*/
public ThreatIntelligenceIndicatorModel() {
}
/**
* Get the kind property: The kind of the entity.
*
* @return the kind value.
*/
@Override
public ThreatIntelligenceResourceKindEnum kind() {
return this.kind;
}
/**
* Get the innerProperties property: Threat Intelligence Entity properties.
*
* @return the innerProperties value.
*/
private ThreatIntelligenceIndicatorProperties innerProperties() {
return this.innerProperties;
}
/**
* Get the systemData property: Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
* @return the systemData value.
*/
@Override
public SystemData systemData() {
return this.systemData;
}
/**
* Get the type property: The type of the resource.
*
* @return the type value.
*/
@Override
public String type() {
return this.type;
}
/**
* Get the name property: The name of the resource.
*
* @return the name value.
*/
@Override
public String name() {
return this.name;
}
/**
* Get the id property: Fully qualified resource Id for the resource.
*
* @return the id value.
*/
@Override
public String id() {
return this.id;
}
/**
* {@inheritDoc}
*/
@Override
public ThreatIntelligenceIndicatorModel withEtag(String etag) {
super.withEtag(etag);
return this;
}
/**
* Get the threatIntelligenceTags property: List of tags.
*
* @return the threatIntelligenceTags value.
*/
public List threatIntelligenceTags() {
return this.innerProperties() == null ? null : this.innerProperties().threatIntelligenceTags();
}
/**
* Set the threatIntelligenceTags property: List of tags.
*
* @param threatIntelligenceTags the threatIntelligenceTags value to set.
* @return the ThreatIntelligenceIndicatorModel object itself.
*/
public ThreatIntelligenceIndicatorModel withThreatIntelligenceTags(List threatIntelligenceTags) {
if (this.innerProperties() == null) {
this.innerProperties = new ThreatIntelligenceIndicatorProperties();
}
this.innerProperties().withThreatIntelligenceTags(threatIntelligenceTags);
return this;
}
/**
* Get the lastUpdatedTimeUtc property: Last updated time in UTC.
*
* @return the lastUpdatedTimeUtc value.
*/
public String lastUpdatedTimeUtc() {
return this.innerProperties() == null ? null : this.innerProperties().lastUpdatedTimeUtc();
}
/**
* Set the lastUpdatedTimeUtc property: Last updated time in UTC.
*
* @param lastUpdatedTimeUtc the lastUpdatedTimeUtc value to set.
* @return the ThreatIntelligenceIndicatorModel object itself.
*/
public ThreatIntelligenceIndicatorModel withLastUpdatedTimeUtc(String lastUpdatedTimeUtc) {
if (this.innerProperties() == null) {
this.innerProperties = new ThreatIntelligenceIndicatorProperties();
}
this.innerProperties().withLastUpdatedTimeUtc(lastUpdatedTimeUtc);
return this;
}
/**
* Get the source property: Source of a threat intelligence entity.
*
* @return the source value.
*/
public String source() {
return this.innerProperties() == null ? null : this.innerProperties().source();
}
/**
* Set the source property: Source of a threat intelligence entity.
*
* @param source the source value to set.
* @return the ThreatIntelligenceIndicatorModel object itself.
*/
public ThreatIntelligenceIndicatorModel withSource(String source) {
if (this.innerProperties() == null) {
this.innerProperties = new ThreatIntelligenceIndicatorProperties();
}
this.innerProperties().withSource(source);
return this;
}
/**
* Get the displayName property: Display name of a threat intelligence entity.
*
* @return the displayName value.
*/
public String displayName() {
return this.innerProperties() == null ? null : this.innerProperties().displayName();
}
/**
* Set the displayName property: Display name of a threat intelligence entity.
*
* @param displayName the displayName value to set.
* @return the ThreatIntelligenceIndicatorModel object itself.
*/
public ThreatIntelligenceIndicatorModel withDisplayName(String displayName) {
if (this.innerProperties() == null) {
this.innerProperties = new ThreatIntelligenceIndicatorProperties();
}
this.innerProperties().withDisplayName(displayName);
return this;
}
/**
* Get the description property: Description of a threat intelligence entity.
*
* @return the description value.
*/
public String description() {
return this.innerProperties() == null ? null : this.innerProperties().description();
}
/**
* Set the description property: Description of a threat intelligence entity.
*
* @param description the description value to set.
* @return the ThreatIntelligenceIndicatorModel object itself.
*/
public ThreatIntelligenceIndicatorModel withDescription(String description) {
if (this.innerProperties() == null) {
this.innerProperties = new ThreatIntelligenceIndicatorProperties();
}
this.innerProperties().withDescription(description);
return this;
}
/**
* Get the indicatorTypes property: Indicator types of threat intelligence entities.
*
* @return the indicatorTypes value.
*/
public List indicatorTypes() {
return this.innerProperties() == null ? null : this.innerProperties().indicatorTypes();
}
/**
* Set the indicatorTypes property: Indicator types of threat intelligence entities.
*
* @param indicatorTypes the indicatorTypes value to set.
* @return the ThreatIntelligenceIndicatorModel object itself.
*/
public ThreatIntelligenceIndicatorModel withIndicatorTypes(List indicatorTypes) {
if (this.innerProperties() == null) {
this.innerProperties = new ThreatIntelligenceIndicatorProperties();
}
this.innerProperties().withIndicatorTypes(indicatorTypes);
return this;
}
/**
* Get the pattern property: Pattern of a threat intelligence entity.
*
* @return the pattern value.
*/
public String pattern() {
return this.innerProperties() == null ? null : this.innerProperties().pattern();
}
/**
* Set the pattern property: Pattern of a threat intelligence entity.
*
* @param pattern the pattern value to set.
* @return the ThreatIntelligenceIndicatorModel object itself.
*/
public ThreatIntelligenceIndicatorModel withPattern(String pattern) {
if (this.innerProperties() == null) {
this.innerProperties = new ThreatIntelligenceIndicatorProperties();
}
this.innerProperties().withPattern(pattern);
return this;
}
/**
* Get the patternType property: Pattern type of a threat intelligence entity.
*
* @return the patternType value.
*/
public String patternType() {
return this.innerProperties() == null ? null : this.innerProperties().patternType();
}
/**
* Set the patternType property: Pattern type of a threat intelligence entity.
*
* @param patternType the patternType value to set.
* @return the ThreatIntelligenceIndicatorModel object itself.
*/
public ThreatIntelligenceIndicatorModel withPatternType(String patternType) {
if (this.innerProperties() == null) {
this.innerProperties = new ThreatIntelligenceIndicatorProperties();
}
this.innerProperties().withPatternType(patternType);
return this;
}
/**
* Get the patternVersion property: Pattern version of a threat intelligence entity.
*
* @return the patternVersion value.
*/
public String patternVersion() {
return this.innerProperties() == null ? null : this.innerProperties().patternVersion();
}
/**
* Set the patternVersion property: Pattern version of a threat intelligence entity.
*
* @param patternVersion the patternVersion value to set.
* @return the ThreatIntelligenceIndicatorModel object itself.
*/
public ThreatIntelligenceIndicatorModel withPatternVersion(String patternVersion) {
if (this.innerProperties() == null) {
this.innerProperties = new ThreatIntelligenceIndicatorProperties();
}
this.innerProperties().withPatternVersion(patternVersion);
return this;
}
/**
* Get the killChainPhases property: Kill chain phases.
*
* @return the killChainPhases value.
*/
public List killChainPhases() {
return this.innerProperties() == null ? null : this.innerProperties().killChainPhases();
}
/**
* Set the killChainPhases property: Kill chain phases.
*
* @param killChainPhases the killChainPhases value to set.
* @return the ThreatIntelligenceIndicatorModel object itself.
*/
public ThreatIntelligenceIndicatorModel
withKillChainPhases(List killChainPhases) {
if (this.innerProperties() == null) {
this.innerProperties = new ThreatIntelligenceIndicatorProperties();
}
this.innerProperties().withKillChainPhases(killChainPhases);
return this;
}
/**
* Get the parsedPattern property: Parsed patterns.
*
* @return the parsedPattern value.
*/
public List parsedPattern() {
return this.innerProperties() == null ? null : this.innerProperties().parsedPattern();
}
/**
* Set the parsedPattern property: Parsed patterns.
*
* @param parsedPattern the parsedPattern value to set.
* @return the ThreatIntelligenceIndicatorModel object itself.
*/
public ThreatIntelligenceIndicatorModel withParsedPattern(List parsedPattern) {
if (this.innerProperties() == null) {
this.innerProperties = new ThreatIntelligenceIndicatorProperties();
}
this.innerProperties().withParsedPattern(parsedPattern);
return this;
}
/**
* Get the externalId property: External ID of threat intelligence entity.
*
* @return the externalId value.
*/
public String externalId() {
return this.innerProperties() == null ? null : this.innerProperties().externalId();
}
/**
* Set the externalId property: External ID of threat intelligence entity.
*
* @param externalId the externalId value to set.
* @return the ThreatIntelligenceIndicatorModel object itself.
*/
public ThreatIntelligenceIndicatorModel withExternalId(String externalId) {
if (this.innerProperties() == null) {
this.innerProperties = new ThreatIntelligenceIndicatorProperties();
}
this.innerProperties().withExternalId(externalId);
return this;
}
/**
* Get the createdByRef property: Created by reference of threat intelligence entity.
*
* @return the createdByRef value.
*/
public String createdByRef() {
return this.innerProperties() == null ? null : this.innerProperties().createdByRef();
}
/**
* Set the createdByRef property: Created by reference of threat intelligence entity.
*
* @param createdByRef the createdByRef value to set.
* @return the ThreatIntelligenceIndicatorModel object itself.
*/
public ThreatIntelligenceIndicatorModel withCreatedByRef(String createdByRef) {
if (this.innerProperties() == null) {
this.innerProperties = new ThreatIntelligenceIndicatorProperties();
}
this.innerProperties().withCreatedByRef(createdByRef);
return this;
}
/**
* Get the defanged property: Is threat intelligence entity defanged.
*
* @return the defanged value.
*/
public Boolean defanged() {
return this.innerProperties() == null ? null : this.innerProperties().defanged();
}
/**
* Set the defanged property: Is threat intelligence entity defanged.
*
* @param defanged the defanged value to set.
* @return the ThreatIntelligenceIndicatorModel object itself.
*/
public ThreatIntelligenceIndicatorModel withDefanged(Boolean defanged) {
if (this.innerProperties() == null) {
this.innerProperties = new ThreatIntelligenceIndicatorProperties();
}
this.innerProperties().withDefanged(defanged);
return this;
}
/**
* Get the externalLastUpdatedTimeUtc property: External last updated time in UTC.
*
* @return the externalLastUpdatedTimeUtc value.
*/
public String externalLastUpdatedTimeUtc() {
return this.innerProperties() == null ? null : this.innerProperties().externalLastUpdatedTimeUtc();
}
/**
* Set the externalLastUpdatedTimeUtc property: External last updated time in UTC.
*
* @param externalLastUpdatedTimeUtc the externalLastUpdatedTimeUtc value to set.
* @return the ThreatIntelligenceIndicatorModel object itself.
*/
public ThreatIntelligenceIndicatorModel withExternalLastUpdatedTimeUtc(String externalLastUpdatedTimeUtc) {
if (this.innerProperties() == null) {
this.innerProperties = new ThreatIntelligenceIndicatorProperties();
}
this.innerProperties().withExternalLastUpdatedTimeUtc(externalLastUpdatedTimeUtc);
return this;
}
/**
* Get the externalReferences property: External References.
*
* @return the externalReferences value.
*/
public List externalReferences() {
return this.innerProperties() == null ? null : this.innerProperties().externalReferences();
}
/**
* Set the externalReferences property: External References.
*
* @param externalReferences the externalReferences value to set.
* @return the ThreatIntelligenceIndicatorModel object itself.
*/
public ThreatIntelligenceIndicatorModel
withExternalReferences(List externalReferences) {
if (this.innerProperties() == null) {
this.innerProperties = new ThreatIntelligenceIndicatorProperties();
}
this.innerProperties().withExternalReferences(externalReferences);
return this;
}
/**
* Get the granularMarkings property: Granular Markings.
*
* @return the granularMarkings value.
*/
public List granularMarkings() {
return this.innerProperties() == null ? null : this.innerProperties().granularMarkings();
}
/**
* Set the granularMarkings property: Granular Markings.
*
* @param granularMarkings the granularMarkings value to set.
* @return the ThreatIntelligenceIndicatorModel object itself.
*/
public ThreatIntelligenceIndicatorModel
withGranularMarkings(List granularMarkings) {
if (this.innerProperties() == null) {
this.innerProperties = new ThreatIntelligenceIndicatorProperties();
}
this.innerProperties().withGranularMarkings(granularMarkings);
return this;
}
/**
* Get the labels property: Labels of threat intelligence entity.
*
* @return the labels value.
*/
public List labels() {
return this.innerProperties() == null ? null : this.innerProperties().labels();
}
/**
* Set the labels property: Labels of threat intelligence entity.
*
* @param labels the labels value to set.
* @return the ThreatIntelligenceIndicatorModel object itself.
*/
public ThreatIntelligenceIndicatorModel withLabels(List labels) {
if (this.innerProperties() == null) {
this.innerProperties = new ThreatIntelligenceIndicatorProperties();
}
this.innerProperties().withLabels(labels);
return this;
}
/**
* Get the revoked property: Is threat intelligence entity revoked.
*
* @return the revoked value.
*/
public Boolean revoked() {
return this.innerProperties() == null ? null : this.innerProperties().revoked();
}
/**
* Set the revoked property: Is threat intelligence entity revoked.
*
* @param revoked the revoked value to set.
* @return the ThreatIntelligenceIndicatorModel object itself.
*/
public ThreatIntelligenceIndicatorModel withRevoked(Boolean revoked) {
if (this.innerProperties() == null) {
this.innerProperties = new ThreatIntelligenceIndicatorProperties();
}
this.innerProperties().withRevoked(revoked);
return this;
}
/**
* Get the confidence property: Confidence of threat intelligence entity.
*
* @return the confidence value.
*/
public Integer confidence() {
return this.innerProperties() == null ? null : this.innerProperties().confidence();
}
/**
* Set the confidence property: Confidence of threat intelligence entity.
*
* @param confidence the confidence value to set.
* @return the ThreatIntelligenceIndicatorModel object itself.
*/
public ThreatIntelligenceIndicatorModel withConfidence(Integer confidence) {
if (this.innerProperties() == null) {
this.innerProperties = new ThreatIntelligenceIndicatorProperties();
}
this.innerProperties().withConfidence(confidence);
return this;
}
/**
* Get the objectMarkingRefs property: Threat intelligence entity object marking references.
*
* @return the objectMarkingRefs value.
*/
public List objectMarkingRefs() {
return this.innerProperties() == null ? null : this.innerProperties().objectMarkingRefs();
}
/**
* Set the objectMarkingRefs property: Threat intelligence entity object marking references.
*
* @param objectMarkingRefs the objectMarkingRefs value to set.
* @return the ThreatIntelligenceIndicatorModel object itself.
*/
public ThreatIntelligenceIndicatorModel withObjectMarkingRefs(List objectMarkingRefs) {
if (this.innerProperties() == null) {
this.innerProperties = new ThreatIntelligenceIndicatorProperties();
}
this.innerProperties().withObjectMarkingRefs(objectMarkingRefs);
return this;
}
/**
* Get the language property: Language of threat intelligence entity.
*
* @return the language value.
*/
public String language() {
return this.innerProperties() == null ? null : this.innerProperties().language();
}
/**
* Set the language property: Language of threat intelligence entity.
*
* @param language the language value to set.
* @return the ThreatIntelligenceIndicatorModel object itself.
*/
public ThreatIntelligenceIndicatorModel withLanguage(String language) {
if (this.innerProperties() == null) {
this.innerProperties = new ThreatIntelligenceIndicatorProperties();
}
this.innerProperties().withLanguage(language);
return this;
}
/**
* Get the threatTypes property: Threat types.
*
* @return the threatTypes value.
*/
public List threatTypes() {
return this.innerProperties() == null ? null : this.innerProperties().threatTypes();
}
/**
* Set the threatTypes property: Threat types.
*
* @param threatTypes the threatTypes value to set.
* @return the ThreatIntelligenceIndicatorModel object itself.
*/
public ThreatIntelligenceIndicatorModel withThreatTypes(List threatTypes) {
if (this.innerProperties() == null) {
this.innerProperties = new ThreatIntelligenceIndicatorProperties();
}
this.innerProperties().withThreatTypes(threatTypes);
return this;
}
/**
* Get the validFrom property: Valid from.
*
* @return the validFrom value.
*/
public String validFrom() {
return this.innerProperties() == null ? null : this.innerProperties().validFrom();
}
/**
* Set the validFrom property: Valid from.
*
* @param validFrom the validFrom value to set.
* @return the ThreatIntelligenceIndicatorModel object itself.
*/
public ThreatIntelligenceIndicatorModel withValidFrom(String validFrom) {
if (this.innerProperties() == null) {
this.innerProperties = new ThreatIntelligenceIndicatorProperties();
}
this.innerProperties().withValidFrom(validFrom);
return this;
}
/**
* Get the validUntil property: Valid until.
*
* @return the validUntil value.
*/
public String validUntil() {
return this.innerProperties() == null ? null : this.innerProperties().validUntil();
}
/**
* Set the validUntil property: Valid until.
*
* @param validUntil the validUntil value to set.
* @return the ThreatIntelligenceIndicatorModel object itself.
*/
public ThreatIntelligenceIndicatorModel withValidUntil(String validUntil) {
if (this.innerProperties() == null) {
this.innerProperties = new ThreatIntelligenceIndicatorProperties();
}
this.innerProperties().withValidUntil(validUntil);
return this;
}
/**
* Get the created property: Created by.
*
* @return the created value.
*/
public String created() {
return this.innerProperties() == null ? null : this.innerProperties().created();
}
/**
* Set the created property: Created by.
*
* @param created the created value to set.
* @return the ThreatIntelligenceIndicatorModel object itself.
*/
public ThreatIntelligenceIndicatorModel withCreated(String created) {
if (this.innerProperties() == null) {
this.innerProperties = new ThreatIntelligenceIndicatorProperties();
}
this.innerProperties().withCreated(created);
return this;
}
/**
* Get the modified property: Modified by.
*
* @return the modified value.
*/
public String modified() {
return this.innerProperties() == null ? null : this.innerProperties().modified();
}
/**
* Set the modified property: Modified by.
*
* @param modified the modified value to set.
* @return the ThreatIntelligenceIndicatorModel object itself.
*/
public ThreatIntelligenceIndicatorModel withModified(String modified) {
if (this.innerProperties() == null) {
this.innerProperties = new ThreatIntelligenceIndicatorProperties();
}
this.innerProperties().withModified(modified);
return this;
}
/**
* Get the extensions property: Extensions map.
*
* @return the extensions value.
*/
public Map extensions() {
return this.innerProperties() == null ? null : this.innerProperties().extensions();
}
/**
* Set the extensions property: Extensions map.
*
* @param extensions the extensions value to set.
* @return the ThreatIntelligenceIndicatorModel object itself.
*/
public ThreatIntelligenceIndicatorModel withExtensions(Map extensions) {
if (this.innerProperties() == null) {
this.innerProperties = new ThreatIntelligenceIndicatorProperties();
}
this.innerProperties().withExtensions(extensions);
return this;
}
/**
* Get the additionalData property: A bag of custom fields that should be part of the entity and will be presented
* to the user.
*
* @return the additionalData value.
*/
public Map additionalData() {
return this.innerProperties() == null ? null : this.innerProperties().additionalData();
}
/**
* Get the friendlyName property: The graph item display name which is a short humanly readable description of the
* graph item instance. This property is optional and might be system generated.
*
* @return the friendlyName value.
*/
public String friendlyName() {
return this.innerProperties() == null ? null : this.innerProperties().friendlyName();
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
@Override
public void validate() {
if (innerProperties() != null) {
innerProperties().validate();
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("etag", etag());
jsonWriter.writeStringField("kind", this.kind == null ? null : this.kind.toString());
jsonWriter.writeJsonField("properties", this.innerProperties);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of ThreatIntelligenceIndicatorModel from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of ThreatIntelligenceIndicatorModel if the JsonReader was pointing to an instance of it, or
* null if it was pointing to JSON null.
* @throws IllegalStateException If the deserialized JSON object was missing any required properties.
* @throws IOException If an error occurs while reading the ThreatIntelligenceIndicatorModel.
*/
public static ThreatIntelligenceIndicatorModel fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
ThreatIntelligenceIndicatorModel deserializedThreatIntelligenceIndicatorModel
= new ThreatIntelligenceIndicatorModel();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("id".equals(fieldName)) {
deserializedThreatIntelligenceIndicatorModel.id = reader.getString();
} else if ("name".equals(fieldName)) {
deserializedThreatIntelligenceIndicatorModel.name = reader.getString();
} else if ("type".equals(fieldName)) {
deserializedThreatIntelligenceIndicatorModel.type = reader.getString();
} else if ("etag".equals(fieldName)) {
deserializedThreatIntelligenceIndicatorModel.withEtag(reader.getString());
} else if ("systemData".equals(fieldName)) {
deserializedThreatIntelligenceIndicatorModel.systemData = SystemData.fromJson(reader);
} else if ("kind".equals(fieldName)) {
deserializedThreatIntelligenceIndicatorModel.kind
= ThreatIntelligenceResourceKindEnum.fromString(reader.getString());
} else if ("properties".equals(fieldName)) {
deserializedThreatIntelligenceIndicatorModel.innerProperties
= ThreatIntelligenceIndicatorProperties.fromJson(reader);
} else {
reader.skipChildren();
}
}
return deserializedThreatIntelligenceIndicatorModel;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy