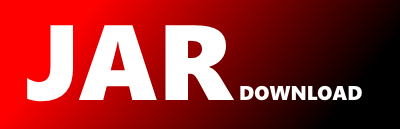
com.azure.resourcemanager.securityinsights.models.WatchlistItem Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-securityinsights Show documentation
Show all versions of azure-resourcemanager-securityinsights Show documentation
This package contains Microsoft Azure SDK for SecurityInsights Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. API spec for Microsoft.SecurityInsights (Azure Security Insights) resource provider. Package tag package-preview-2021-09.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.securityinsights.models;
import com.azure.core.management.SystemData;
import com.azure.core.util.Context;
import com.azure.resourcemanager.securityinsights.fluent.models.WatchlistItemInner;
import java.time.OffsetDateTime;
import java.util.Map;
/**
* An immutable client-side representation of WatchlistItem.
*/
public interface WatchlistItem {
/**
* Gets the id property: Fully qualified resource Id for the resource.
*
* @return the id value.
*/
String id();
/**
* Gets the name property: The name of the resource.
*
* @return the name value.
*/
String name();
/**
* Gets the type property: The type of the resource.
*
* @return the type value.
*/
String type();
/**
* Gets the etag property: Etag of the azure resource.
*
* @return the etag value.
*/
String etag();
/**
* Gets the systemData property: Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
* @return the systemData value.
*/
SystemData systemData();
/**
* Gets the watchlistItemType property: The type of the watchlist item.
*
* @return the watchlistItemType value.
*/
String watchlistItemType();
/**
* Gets the watchlistItemId property: The id (a Guid) of the watchlist item.
*
* @return the watchlistItemId value.
*/
String watchlistItemId();
/**
* Gets the tenantId property: The tenantId to which the watchlist item belongs to.
*
* @return the tenantId value.
*/
String tenantId();
/**
* Gets the isDeleted property: A flag that indicates if the watchlist item is deleted or not.
*
* @return the isDeleted value.
*/
Boolean isDeleted();
/**
* Gets the created property: The time the watchlist item was created.
*
* @return the created value.
*/
OffsetDateTime created();
/**
* Gets the updated property: The last time the watchlist item was updated.
*
* @return the updated value.
*/
OffsetDateTime updated();
/**
* Gets the createdBy property: Describes a user that created the watchlist item.
*
* @return the createdBy value.
*/
UserInfo createdBy();
/**
* Gets the updatedBy property: Describes a user that updated the watchlist item.
*
* @return the updatedBy value.
*/
UserInfo updatedBy();
/**
* Gets the itemsKeyValue property: key-value pairs for a watchlist item.
*
* @return the itemsKeyValue value.
*/
Map itemsKeyValue();
/**
* Gets the entityMapping property: key-value pairs for a watchlist item entity mapping.
*
* @return the entityMapping value.
*/
Map entityMapping();
/**
* Gets the name of the resource group.
*
* @return the name of the resource group.
*/
String resourceGroupName();
/**
* Gets the inner com.azure.resourcemanager.securityinsights.fluent.models.WatchlistItemInner object.
*
* @return the inner object.
*/
WatchlistItemInner innerModel();
/**
* The entirety of the WatchlistItem definition.
*/
interface Definition
extends DefinitionStages.Blank, DefinitionStages.WithParentResource, DefinitionStages.WithCreate {
}
/**
* The WatchlistItem definition stages.
*/
interface DefinitionStages {
/**
* The first stage of the WatchlistItem definition.
*/
interface Blank extends WithParentResource {
}
/**
* The stage of the WatchlistItem definition allowing to specify parent resource.
*/
interface WithParentResource {
/**
* Specifies resourceGroupName, workspaceName, watchlistAlias.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param workspaceName The name of the workspace.
* @param watchlistAlias Watchlist Alias.
* @return the next definition stage.
*/
WithCreate withExistingWatchlist(String resourceGroupName, String workspaceName, String watchlistAlias);
}
/**
* The stage of the WatchlistItem definition which contains all the minimum required properties for the resource
* to be created, but also allows for any other optional properties to be specified.
*/
interface WithCreate extends DefinitionStages.WithEtag, DefinitionStages.WithWatchlistItemType,
DefinitionStages.WithWatchlistItemId, DefinitionStages.WithTenantId, DefinitionStages.WithIsDeleted,
DefinitionStages.WithCreated, DefinitionStages.WithUpdated, DefinitionStages.WithCreatedBy,
DefinitionStages.WithUpdatedBy, DefinitionStages.WithItemsKeyValue, DefinitionStages.WithEntityMapping {
/**
* Executes the create request.
*
* @return the created resource.
*/
WatchlistItem create();
/**
* Executes the create request.
*
* @param context The context to associate with this operation.
* @return the created resource.
*/
WatchlistItem create(Context context);
}
/**
* The stage of the WatchlistItem definition allowing to specify etag.
*/
interface WithEtag {
/**
* Specifies the etag property: Etag of the azure resource.
*
* @param etag Etag of the azure resource.
* @return the next definition stage.
*/
WithCreate withEtag(String etag);
}
/**
* The stage of the WatchlistItem definition allowing to specify watchlistItemType.
*/
interface WithWatchlistItemType {
/**
* Specifies the watchlistItemType property: The type of the watchlist item.
*
* @param watchlistItemType The type of the watchlist item.
* @return the next definition stage.
*/
WithCreate withWatchlistItemType(String watchlistItemType);
}
/**
* The stage of the WatchlistItem definition allowing to specify watchlistItemId.
*/
interface WithWatchlistItemId {
/**
* Specifies the watchlistItemId property: The id (a Guid) of the watchlist item.
*
* @param watchlistItemId The id (a Guid) of the watchlist item.
* @return the next definition stage.
*/
WithCreate withWatchlistItemId(String watchlistItemId);
}
/**
* The stage of the WatchlistItem definition allowing to specify tenantId.
*/
interface WithTenantId {
/**
* Specifies the tenantId property: The tenantId to which the watchlist item belongs to.
*
* @param tenantId The tenantId to which the watchlist item belongs to.
* @return the next definition stage.
*/
WithCreate withTenantId(String tenantId);
}
/**
* The stage of the WatchlistItem definition allowing to specify isDeleted.
*/
interface WithIsDeleted {
/**
* Specifies the isDeleted property: A flag that indicates if the watchlist item is deleted or not.
*
* @param isDeleted A flag that indicates if the watchlist item is deleted or not.
* @return the next definition stage.
*/
WithCreate withIsDeleted(Boolean isDeleted);
}
/**
* The stage of the WatchlistItem definition allowing to specify created.
*/
interface WithCreated {
/**
* Specifies the created property: The time the watchlist item was created.
*
* @param created The time the watchlist item was created.
* @return the next definition stage.
*/
WithCreate withCreated(OffsetDateTime created);
}
/**
* The stage of the WatchlistItem definition allowing to specify updated.
*/
interface WithUpdated {
/**
* Specifies the updated property: The last time the watchlist item was updated.
*
* @param updated The last time the watchlist item was updated.
* @return the next definition stage.
*/
WithCreate withUpdated(OffsetDateTime updated);
}
/**
* The stage of the WatchlistItem definition allowing to specify createdBy.
*/
interface WithCreatedBy {
/**
* Specifies the createdBy property: Describes a user that created the watchlist item.
*
* @param createdBy Describes a user that created the watchlist item.
* @return the next definition stage.
*/
WithCreate withCreatedBy(UserInfo createdBy);
}
/**
* The stage of the WatchlistItem definition allowing to specify updatedBy.
*/
interface WithUpdatedBy {
/**
* Specifies the updatedBy property: Describes a user that updated the watchlist item.
*
* @param updatedBy Describes a user that updated the watchlist item.
* @return the next definition stage.
*/
WithCreate withUpdatedBy(UserInfo updatedBy);
}
/**
* The stage of the WatchlistItem definition allowing to specify itemsKeyValue.
*/
interface WithItemsKeyValue {
/**
* Specifies the itemsKeyValue property: key-value pairs for a watchlist item.
*
* @param itemsKeyValue key-value pairs for a watchlist item.
* @return the next definition stage.
*/
WithCreate withItemsKeyValue(Map itemsKeyValue);
}
/**
* The stage of the WatchlistItem definition allowing to specify entityMapping.
*/
interface WithEntityMapping {
/**
* Specifies the entityMapping property: key-value pairs for a watchlist item entity mapping.
*
* @param entityMapping key-value pairs for a watchlist item entity mapping.
* @return the next definition stage.
*/
WithCreate withEntityMapping(Map entityMapping);
}
}
/**
* Begins update for the WatchlistItem resource.
*
* @return the stage of resource update.
*/
WatchlistItem.Update update();
/**
* The template for WatchlistItem update.
*/
interface Update extends UpdateStages.WithEtag, UpdateStages.WithWatchlistItemType,
UpdateStages.WithWatchlistItemId, UpdateStages.WithTenantId, UpdateStages.WithIsDeleted,
UpdateStages.WithCreated, UpdateStages.WithUpdated, UpdateStages.WithCreatedBy, UpdateStages.WithUpdatedBy,
UpdateStages.WithItemsKeyValue, UpdateStages.WithEntityMapping {
/**
* Executes the update request.
*
* @return the updated resource.
*/
WatchlistItem apply();
/**
* Executes the update request.
*
* @param context The context to associate with this operation.
* @return the updated resource.
*/
WatchlistItem apply(Context context);
}
/**
* The WatchlistItem update stages.
*/
interface UpdateStages {
/**
* The stage of the WatchlistItem update allowing to specify etag.
*/
interface WithEtag {
/**
* Specifies the etag property: Etag of the azure resource.
*
* @param etag Etag of the azure resource.
* @return the next definition stage.
*/
Update withEtag(String etag);
}
/**
* The stage of the WatchlistItem update allowing to specify watchlistItemType.
*/
interface WithWatchlistItemType {
/**
* Specifies the watchlistItemType property: The type of the watchlist item.
*
* @param watchlistItemType The type of the watchlist item.
* @return the next definition stage.
*/
Update withWatchlistItemType(String watchlistItemType);
}
/**
* The stage of the WatchlistItem update allowing to specify watchlistItemId.
*/
interface WithWatchlistItemId {
/**
* Specifies the watchlistItemId property: The id (a Guid) of the watchlist item.
*
* @param watchlistItemId The id (a Guid) of the watchlist item.
* @return the next definition stage.
*/
Update withWatchlistItemId(String watchlistItemId);
}
/**
* The stage of the WatchlistItem update allowing to specify tenantId.
*/
interface WithTenantId {
/**
* Specifies the tenantId property: The tenantId to which the watchlist item belongs to.
*
* @param tenantId The tenantId to which the watchlist item belongs to.
* @return the next definition stage.
*/
Update withTenantId(String tenantId);
}
/**
* The stage of the WatchlistItem update allowing to specify isDeleted.
*/
interface WithIsDeleted {
/**
* Specifies the isDeleted property: A flag that indicates if the watchlist item is deleted or not.
*
* @param isDeleted A flag that indicates if the watchlist item is deleted or not.
* @return the next definition stage.
*/
Update withIsDeleted(Boolean isDeleted);
}
/**
* The stage of the WatchlistItem update allowing to specify created.
*/
interface WithCreated {
/**
* Specifies the created property: The time the watchlist item was created.
*
* @param created The time the watchlist item was created.
* @return the next definition stage.
*/
Update withCreated(OffsetDateTime created);
}
/**
* The stage of the WatchlistItem update allowing to specify updated.
*/
interface WithUpdated {
/**
* Specifies the updated property: The last time the watchlist item was updated.
*
* @param updated The last time the watchlist item was updated.
* @return the next definition stage.
*/
Update withUpdated(OffsetDateTime updated);
}
/**
* The stage of the WatchlistItem update allowing to specify createdBy.
*/
interface WithCreatedBy {
/**
* Specifies the createdBy property: Describes a user that created the watchlist item.
*
* @param createdBy Describes a user that created the watchlist item.
* @return the next definition stage.
*/
Update withCreatedBy(UserInfo createdBy);
}
/**
* The stage of the WatchlistItem update allowing to specify updatedBy.
*/
interface WithUpdatedBy {
/**
* Specifies the updatedBy property: Describes a user that updated the watchlist item.
*
* @param updatedBy Describes a user that updated the watchlist item.
* @return the next definition stage.
*/
Update withUpdatedBy(UserInfo updatedBy);
}
/**
* The stage of the WatchlistItem update allowing to specify itemsKeyValue.
*/
interface WithItemsKeyValue {
/**
* Specifies the itemsKeyValue property: key-value pairs for a watchlist item.
*
* @param itemsKeyValue key-value pairs for a watchlist item.
* @return the next definition stage.
*/
Update withItemsKeyValue(Map itemsKeyValue);
}
/**
* The stage of the WatchlistItem update allowing to specify entityMapping.
*/
interface WithEntityMapping {
/**
* Specifies the entityMapping property: key-value pairs for a watchlist item entity mapping.
*
* @param entityMapping key-value pairs for a watchlist item entity mapping.
* @return the next definition stage.
*/
Update withEntityMapping(Map entityMapping);
}
}
/**
* Refreshes the resource to sync with Azure.
*
* @return the refreshed resource.
*/
WatchlistItem refresh();
/**
* Refreshes the resource to sync with Azure.
*
* @param context The context to associate with this operation.
* @return the refreshed resource.
*/
WatchlistItem refresh(Context context);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy