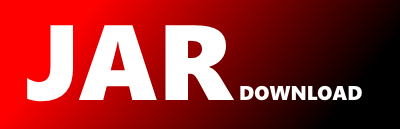
com.azure.resourcemanager.servicebus.fluent.models.SBSubscriptionProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-servicebus Show documentation
Show all versions of azure-resourcemanager-servicebus Show documentation
This package contains Microsoft Azure ServiceBus Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.servicebus.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.CoreUtils;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.servicebus.implementation.DurationSerializer;
import com.azure.resourcemanager.servicebus.models.EntityStatus;
import com.azure.resourcemanager.servicebus.models.MessageCountDetails;
import com.azure.resourcemanager.servicebus.models.SBClientAffineProperties;
import java.io.IOException;
import java.time.Duration;
import java.time.OffsetDateTime;
/**
* Description of Subscription Resource.
*/
@Fluent
public final class SBSubscriptionProperties implements JsonSerializable {
/*
* Number of messages.
*/
private Long messageCount;
/*
* Exact time the message was created.
*/
private OffsetDateTime createdAt;
/*
* Last time there was a receive request to this subscription.
*/
private OffsetDateTime accessedAt;
/*
* The exact time the message was updated.
*/
private OffsetDateTime updatedAt;
/*
* Message count details
*/
private MessageCountDetails countDetails;
/*
* ISO 8061 lock duration timespan for the subscription. The default value is 1 minute.
*/
private Duration lockDuration;
/*
* Value indicating if a subscription supports the concept of sessions.
*/
private Boolean requiresSession;
/*
* ISO 8061 Default message timespan to live value. This is the duration after which the message expires, starting
* from when the message is sent to Service Bus. This is the default value used when TimeToLive is not set on a
* message itself.
*/
private Duration defaultMessageTimeToLive;
/*
* Value that indicates whether a subscription has dead letter support on filter evaluation exceptions.
*/
private Boolean deadLetteringOnFilterEvaluationExceptions;
/*
* Value that indicates whether a subscription has dead letter support when a message expires.
*/
private Boolean deadLetteringOnMessageExpiration;
/*
* ISO 8601 timeSpan structure that defines the duration of the duplicate detection history. The default value is 10
* minutes.
*/
private Duration duplicateDetectionHistoryTimeWindow;
/*
* Number of maximum deliveries.
*/
private Integer maxDeliveryCount;
/*
* Enumerates the possible values for the status of a messaging entity.
*/
private EntityStatus status;
/*
* Value that indicates whether server-side batched operations are enabled.
*/
private Boolean enableBatchedOperations;
/*
* ISO 8061 timeSpan idle interval after which the topic is automatically deleted. The minimum duration is 5
* minutes.
*/
private Duration autoDeleteOnIdle;
/*
* Queue/Topic name to forward the messages
*/
private String forwardTo;
/*
* Queue/Topic name to forward the Dead Letter message
*/
private String forwardDeadLetteredMessagesTo;
/*
* Value that indicates whether the subscription has an affinity to the client id.
*/
private Boolean isClientAffine;
/*
* Properties specific to client affine subscriptions.
*/
private SBClientAffineProperties clientAffineProperties;
/**
* Creates an instance of SBSubscriptionProperties class.
*/
public SBSubscriptionProperties() {
}
/**
* Get the messageCount property: Number of messages.
*
* @return the messageCount value.
*/
public Long messageCount() {
return this.messageCount;
}
/**
* Get the createdAt property: Exact time the message was created.
*
* @return the createdAt value.
*/
public OffsetDateTime createdAt() {
return this.createdAt;
}
/**
* Get the accessedAt property: Last time there was a receive request to this subscription.
*
* @return the accessedAt value.
*/
public OffsetDateTime accessedAt() {
return this.accessedAt;
}
/**
* Get the updatedAt property: The exact time the message was updated.
*
* @return the updatedAt value.
*/
public OffsetDateTime updatedAt() {
return this.updatedAt;
}
/**
* Get the countDetails property: Message count details.
*
* @return the countDetails value.
*/
public MessageCountDetails countDetails() {
return this.countDetails;
}
/**
* Get the lockDuration property: ISO 8061 lock duration timespan for the subscription. The default value is 1
* minute.
*
* @return the lockDuration value.
*/
public Duration lockDuration() {
return this.lockDuration;
}
/**
* Set the lockDuration property: ISO 8061 lock duration timespan for the subscription. The default value is 1
* minute.
*
* @param lockDuration the lockDuration value to set.
* @return the SBSubscriptionProperties object itself.
*/
public SBSubscriptionProperties withLockDuration(Duration lockDuration) {
this.lockDuration = lockDuration;
return this;
}
/**
* Get the requiresSession property: Value indicating if a subscription supports the concept of sessions.
*
* @return the requiresSession value.
*/
public Boolean requiresSession() {
return this.requiresSession;
}
/**
* Set the requiresSession property: Value indicating if a subscription supports the concept of sessions.
*
* @param requiresSession the requiresSession value to set.
* @return the SBSubscriptionProperties object itself.
*/
public SBSubscriptionProperties withRequiresSession(Boolean requiresSession) {
this.requiresSession = requiresSession;
return this;
}
/**
* Get the defaultMessageTimeToLive property: ISO 8061 Default message timespan to live value. This is the duration
* after which the message expires, starting from when the message is sent to Service Bus. This is the default value
* used when TimeToLive is not set on a message itself.
*
* @return the defaultMessageTimeToLive value.
*/
public Duration defaultMessageTimeToLive() {
return this.defaultMessageTimeToLive;
}
/**
* Set the defaultMessageTimeToLive property: ISO 8061 Default message timespan to live value. This is the duration
* after which the message expires, starting from when the message is sent to Service Bus. This is the default value
* used when TimeToLive is not set on a message itself.
*
* @param defaultMessageTimeToLive the defaultMessageTimeToLive value to set.
* @return the SBSubscriptionProperties object itself.
*/
public SBSubscriptionProperties withDefaultMessageTimeToLive(Duration defaultMessageTimeToLive) {
this.defaultMessageTimeToLive = defaultMessageTimeToLive;
return this;
}
/**
* Get the deadLetteringOnFilterEvaluationExceptions property: Value that indicates whether a subscription has dead
* letter support on filter evaluation exceptions.
*
* @return the deadLetteringOnFilterEvaluationExceptions value.
*/
public Boolean deadLetteringOnFilterEvaluationExceptions() {
return this.deadLetteringOnFilterEvaluationExceptions;
}
/**
* Set the deadLetteringOnFilterEvaluationExceptions property: Value that indicates whether a subscription has dead
* letter support on filter evaluation exceptions.
*
* @param deadLetteringOnFilterEvaluationExceptions the deadLetteringOnFilterEvaluationExceptions value to set.
* @return the SBSubscriptionProperties object itself.
*/
public SBSubscriptionProperties
withDeadLetteringOnFilterEvaluationExceptions(Boolean deadLetteringOnFilterEvaluationExceptions) {
this.deadLetteringOnFilterEvaluationExceptions = deadLetteringOnFilterEvaluationExceptions;
return this;
}
/**
* Get the deadLetteringOnMessageExpiration property: Value that indicates whether a subscription has dead letter
* support when a message expires.
*
* @return the deadLetteringOnMessageExpiration value.
*/
public Boolean deadLetteringOnMessageExpiration() {
return this.deadLetteringOnMessageExpiration;
}
/**
* Set the deadLetteringOnMessageExpiration property: Value that indicates whether a subscription has dead letter
* support when a message expires.
*
* @param deadLetteringOnMessageExpiration the deadLetteringOnMessageExpiration value to set.
* @return the SBSubscriptionProperties object itself.
*/
public SBSubscriptionProperties withDeadLetteringOnMessageExpiration(Boolean deadLetteringOnMessageExpiration) {
this.deadLetteringOnMessageExpiration = deadLetteringOnMessageExpiration;
return this;
}
/**
* Get the duplicateDetectionHistoryTimeWindow property: ISO 8601 timeSpan structure that defines the duration of
* the duplicate detection history. The default value is 10 minutes.
*
* @return the duplicateDetectionHistoryTimeWindow value.
*/
public Duration duplicateDetectionHistoryTimeWindow() {
return this.duplicateDetectionHistoryTimeWindow;
}
/**
* Set the duplicateDetectionHistoryTimeWindow property: ISO 8601 timeSpan structure that defines the duration of
* the duplicate detection history. The default value is 10 minutes.
*
* @param duplicateDetectionHistoryTimeWindow the duplicateDetectionHistoryTimeWindow value to set.
* @return the SBSubscriptionProperties object itself.
*/
public SBSubscriptionProperties
withDuplicateDetectionHistoryTimeWindow(Duration duplicateDetectionHistoryTimeWindow) {
this.duplicateDetectionHistoryTimeWindow = duplicateDetectionHistoryTimeWindow;
return this;
}
/**
* Get the maxDeliveryCount property: Number of maximum deliveries.
*
* @return the maxDeliveryCount value.
*/
public Integer maxDeliveryCount() {
return this.maxDeliveryCount;
}
/**
* Set the maxDeliveryCount property: Number of maximum deliveries.
*
* @param maxDeliveryCount the maxDeliveryCount value to set.
* @return the SBSubscriptionProperties object itself.
*/
public SBSubscriptionProperties withMaxDeliveryCount(Integer maxDeliveryCount) {
this.maxDeliveryCount = maxDeliveryCount;
return this;
}
/**
* Get the status property: Enumerates the possible values for the status of a messaging entity.
*
* @return the status value.
*/
public EntityStatus status() {
return this.status;
}
/**
* Set the status property: Enumerates the possible values for the status of a messaging entity.
*
* @param status the status value to set.
* @return the SBSubscriptionProperties object itself.
*/
public SBSubscriptionProperties withStatus(EntityStatus status) {
this.status = status;
return this;
}
/**
* Get the enableBatchedOperations property: Value that indicates whether server-side batched operations are
* enabled.
*
* @return the enableBatchedOperations value.
*/
public Boolean enableBatchedOperations() {
return this.enableBatchedOperations;
}
/**
* Set the enableBatchedOperations property: Value that indicates whether server-side batched operations are
* enabled.
*
* @param enableBatchedOperations the enableBatchedOperations value to set.
* @return the SBSubscriptionProperties object itself.
*/
public SBSubscriptionProperties withEnableBatchedOperations(Boolean enableBatchedOperations) {
this.enableBatchedOperations = enableBatchedOperations;
return this;
}
/**
* Get the autoDeleteOnIdle property: ISO 8061 timeSpan idle interval after which the topic is automatically
* deleted. The minimum duration is 5 minutes.
*
* @return the autoDeleteOnIdle value.
*/
public Duration autoDeleteOnIdle() {
return this.autoDeleteOnIdle;
}
/**
* Set the autoDeleteOnIdle property: ISO 8061 timeSpan idle interval after which the topic is automatically
* deleted. The minimum duration is 5 minutes.
*
* @param autoDeleteOnIdle the autoDeleteOnIdle value to set.
* @return the SBSubscriptionProperties object itself.
*/
public SBSubscriptionProperties withAutoDeleteOnIdle(Duration autoDeleteOnIdle) {
this.autoDeleteOnIdle = autoDeleteOnIdle;
return this;
}
/**
* Get the forwardTo property: Queue/Topic name to forward the messages.
*
* @return the forwardTo value.
*/
public String forwardTo() {
return this.forwardTo;
}
/**
* Set the forwardTo property: Queue/Topic name to forward the messages.
*
* @param forwardTo the forwardTo value to set.
* @return the SBSubscriptionProperties object itself.
*/
public SBSubscriptionProperties withForwardTo(String forwardTo) {
this.forwardTo = forwardTo;
return this;
}
/**
* Get the forwardDeadLetteredMessagesTo property: Queue/Topic name to forward the Dead Letter message.
*
* @return the forwardDeadLetteredMessagesTo value.
*/
public String forwardDeadLetteredMessagesTo() {
return this.forwardDeadLetteredMessagesTo;
}
/**
* Set the forwardDeadLetteredMessagesTo property: Queue/Topic name to forward the Dead Letter message.
*
* @param forwardDeadLetteredMessagesTo the forwardDeadLetteredMessagesTo value to set.
* @return the SBSubscriptionProperties object itself.
*/
public SBSubscriptionProperties withForwardDeadLetteredMessagesTo(String forwardDeadLetteredMessagesTo) {
this.forwardDeadLetteredMessagesTo = forwardDeadLetteredMessagesTo;
return this;
}
/**
* Get the isClientAffine property: Value that indicates whether the subscription has an affinity to the client id.
*
* @return the isClientAffine value.
*/
public Boolean isClientAffine() {
return this.isClientAffine;
}
/**
* Set the isClientAffine property: Value that indicates whether the subscription has an affinity to the client id.
*
* @param isClientAffine the isClientAffine value to set.
* @return the SBSubscriptionProperties object itself.
*/
public SBSubscriptionProperties withIsClientAffine(Boolean isClientAffine) {
this.isClientAffine = isClientAffine;
return this;
}
/**
* Get the clientAffineProperties property: Properties specific to client affine subscriptions.
*
* @return the clientAffineProperties value.
*/
public SBClientAffineProperties clientAffineProperties() {
return this.clientAffineProperties;
}
/**
* Set the clientAffineProperties property: Properties specific to client affine subscriptions.
*
* @param clientAffineProperties the clientAffineProperties value to set.
* @return the SBSubscriptionProperties object itself.
*/
public SBSubscriptionProperties withClientAffineProperties(SBClientAffineProperties clientAffineProperties) {
this.clientAffineProperties = clientAffineProperties;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (countDetails() != null) {
countDetails().validate();
}
if (clientAffineProperties() != null) {
clientAffineProperties().validate();
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("lockDuration", CoreUtils.durationToStringWithDays(this.lockDuration));
jsonWriter.writeBooleanField("requiresSession", this.requiresSession);
jsonWriter.writeStringField("defaultMessageTimeToLive",
DurationSerializer.serialize(this.defaultMessageTimeToLive));
jsonWriter.writeBooleanField("deadLetteringOnFilterEvaluationExceptions",
this.deadLetteringOnFilterEvaluationExceptions);
jsonWriter.writeBooleanField("deadLetteringOnMessageExpiration", this.deadLetteringOnMessageExpiration);
jsonWriter.writeStringField("duplicateDetectionHistoryTimeWindow",
DurationSerializer.serialize(this.duplicateDetectionHistoryTimeWindow));
jsonWriter.writeNumberField("maxDeliveryCount", this.maxDeliveryCount);
jsonWriter.writeStringField("status", this.status == null ? null : this.status.toString());
jsonWriter.writeBooleanField("enableBatchedOperations", this.enableBatchedOperations);
jsonWriter.writeStringField("autoDeleteOnIdle", DurationSerializer.serialize(this.autoDeleteOnIdle));
jsonWriter.writeStringField("forwardTo", this.forwardTo);
jsonWriter.writeStringField("forwardDeadLetteredMessagesTo", this.forwardDeadLetteredMessagesTo);
jsonWriter.writeBooleanField("isClientAffine", this.isClientAffine);
jsonWriter.writeJsonField("clientAffineProperties", this.clientAffineProperties);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of SBSubscriptionProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of SBSubscriptionProperties if the JsonReader was pointing to an instance of it, or null if
* it was pointing to JSON null.
* @throws IOException If an error occurs while reading the SBSubscriptionProperties.
*/
public static SBSubscriptionProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
SBSubscriptionProperties deserializedSBSubscriptionProperties = new SBSubscriptionProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("messageCount".equals(fieldName)) {
deserializedSBSubscriptionProperties.messageCount = reader.getNullable(JsonReader::getLong);
} else if ("createdAt".equals(fieldName)) {
deserializedSBSubscriptionProperties.createdAt = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("accessedAt".equals(fieldName)) {
deserializedSBSubscriptionProperties.accessedAt = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("updatedAt".equals(fieldName)) {
deserializedSBSubscriptionProperties.updatedAt = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("countDetails".equals(fieldName)) {
deserializedSBSubscriptionProperties.countDetails = MessageCountDetails.fromJson(reader);
} else if ("lockDuration".equals(fieldName)) {
deserializedSBSubscriptionProperties.lockDuration
= reader.getNullable(nonNullReader -> Duration.parse(nonNullReader.getString()));
} else if ("requiresSession".equals(fieldName)) {
deserializedSBSubscriptionProperties.requiresSession = reader.getNullable(JsonReader::getBoolean);
} else if ("defaultMessageTimeToLive".equals(fieldName)) {
deserializedSBSubscriptionProperties.defaultMessageTimeToLive
= reader.getNullable(nonNullReader -> Duration.parse(nonNullReader.getString()));
} else if ("deadLetteringOnFilterEvaluationExceptions".equals(fieldName)) {
deserializedSBSubscriptionProperties.deadLetteringOnFilterEvaluationExceptions
= reader.getNullable(JsonReader::getBoolean);
} else if ("deadLetteringOnMessageExpiration".equals(fieldName)) {
deserializedSBSubscriptionProperties.deadLetteringOnMessageExpiration
= reader.getNullable(JsonReader::getBoolean);
} else if ("duplicateDetectionHistoryTimeWindow".equals(fieldName)) {
deserializedSBSubscriptionProperties.duplicateDetectionHistoryTimeWindow
= reader.getNullable(nonNullReader -> Duration.parse(nonNullReader.getString()));
} else if ("maxDeliveryCount".equals(fieldName)) {
deserializedSBSubscriptionProperties.maxDeliveryCount = reader.getNullable(JsonReader::getInt);
} else if ("status".equals(fieldName)) {
deserializedSBSubscriptionProperties.status = EntityStatus.fromString(reader.getString());
} else if ("enableBatchedOperations".equals(fieldName)) {
deserializedSBSubscriptionProperties.enableBatchedOperations
= reader.getNullable(JsonReader::getBoolean);
} else if ("autoDeleteOnIdle".equals(fieldName)) {
deserializedSBSubscriptionProperties.autoDeleteOnIdle
= reader.getNullable(nonNullReader -> Duration.parse(nonNullReader.getString()));
} else if ("forwardTo".equals(fieldName)) {
deserializedSBSubscriptionProperties.forwardTo = reader.getString();
} else if ("forwardDeadLetteredMessagesTo".equals(fieldName)) {
deserializedSBSubscriptionProperties.forwardDeadLetteredMessagesTo = reader.getString();
} else if ("isClientAffine".equals(fieldName)) {
deserializedSBSubscriptionProperties.isClientAffine = reader.getNullable(JsonReader::getBoolean);
} else if ("clientAffineProperties".equals(fieldName)) {
deserializedSBSubscriptionProperties.clientAffineProperties
= SBClientAffineProperties.fromJson(reader);
} else {
reader.skipChildren();
}
}
return deserializedSBSubscriptionProperties;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy