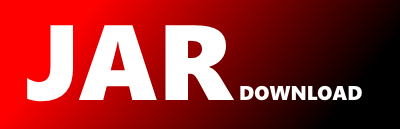
com.azure.resourcemanager.servicebus.fluent.models.AccessKeysInner Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-servicebus Show documentation
Show all versions of azure-resourcemanager-servicebus Show documentation
This package contains Microsoft Azure ServiceBus Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.servicebus.fluent.models;
import com.azure.core.annotation.Immutable;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
/**
* Namespace/ServiceBus Connection String.
*/
@Immutable
public final class AccessKeysInner implements JsonSerializable {
/*
* Primary connection string of the created namespace authorization rule.
*/
private String primaryConnectionString;
/*
* Secondary connection string of the created namespace authorization rule.
*/
private String secondaryConnectionString;
/*
* Primary connection string of the alias if GEO DR is enabled
*/
private String aliasPrimaryConnectionString;
/*
* Secondary connection string of the alias if GEO DR is enabled
*/
private String aliasSecondaryConnectionString;
/*
* A base64-encoded 256-bit primary key for signing and validating the SAS token.
*/
private String primaryKey;
/*
* A base64-encoded 256-bit primary key for signing and validating the SAS token.
*/
private String secondaryKey;
/*
* A string that describes the authorization rule.
*/
private String keyName;
/**
* Creates an instance of AccessKeysInner class.
*/
public AccessKeysInner() {
}
/**
* Get the primaryConnectionString property: Primary connection string of the created namespace authorization rule.
*
* @return the primaryConnectionString value.
*/
public String primaryConnectionString() {
return this.primaryConnectionString;
}
/**
* Get the secondaryConnectionString property: Secondary connection string of the created namespace authorization
* rule.
*
* @return the secondaryConnectionString value.
*/
public String secondaryConnectionString() {
return this.secondaryConnectionString;
}
/**
* Get the aliasPrimaryConnectionString property: Primary connection string of the alias if GEO DR is enabled.
*
* @return the aliasPrimaryConnectionString value.
*/
public String aliasPrimaryConnectionString() {
return this.aliasPrimaryConnectionString;
}
/**
* Get the aliasSecondaryConnectionString property: Secondary connection string of the alias if GEO DR is enabled.
*
* @return the aliasSecondaryConnectionString value.
*/
public String aliasSecondaryConnectionString() {
return this.aliasSecondaryConnectionString;
}
/**
* Get the primaryKey property: A base64-encoded 256-bit primary key for signing and validating the SAS token.
*
* @return the primaryKey value.
*/
public String primaryKey() {
return this.primaryKey;
}
/**
* Get the secondaryKey property: A base64-encoded 256-bit primary key for signing and validating the SAS token.
*
* @return the secondaryKey value.
*/
public String secondaryKey() {
return this.secondaryKey;
}
/**
* Get the keyName property: A string that describes the authorization rule.
*
* @return the keyName value.
*/
public String keyName() {
return this.keyName;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of AccessKeysInner from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of AccessKeysInner if the JsonReader was pointing to an instance of it, or null if it was
* pointing to JSON null.
* @throws IOException If an error occurs while reading the AccessKeysInner.
*/
public static AccessKeysInner fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
AccessKeysInner deserializedAccessKeysInner = new AccessKeysInner();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("primaryConnectionString".equals(fieldName)) {
deserializedAccessKeysInner.primaryConnectionString = reader.getString();
} else if ("secondaryConnectionString".equals(fieldName)) {
deserializedAccessKeysInner.secondaryConnectionString = reader.getString();
} else if ("aliasPrimaryConnectionString".equals(fieldName)) {
deserializedAccessKeysInner.aliasPrimaryConnectionString = reader.getString();
} else if ("aliasSecondaryConnectionString".equals(fieldName)) {
deserializedAccessKeysInner.aliasSecondaryConnectionString = reader.getString();
} else if ("primaryKey".equals(fieldName)) {
deserializedAccessKeysInner.primaryKey = reader.getString();
} else if ("secondaryKey".equals(fieldName)) {
deserializedAccessKeysInner.secondaryKey = reader.getString();
} else if ("keyName".equals(fieldName)) {
deserializedAccessKeysInner.keyName = reader.getString();
} else {
reader.skipChildren();
}
}
return deserializedAccessKeysInner;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy