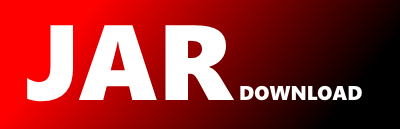
com.azure.resourcemanager.servicebus.fluent.models.Ruleproperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-servicebus Show documentation
Show all versions of azure-resourcemanager-servicebus Show documentation
This package contains Microsoft Azure ServiceBus Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.servicebus.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.servicebus.models.Action;
import com.azure.resourcemanager.servicebus.models.CorrelationFilter;
import com.azure.resourcemanager.servicebus.models.FilterType;
import com.azure.resourcemanager.servicebus.models.SqlFilter;
import java.io.IOException;
/**
* Description of Rule Resource.
*/
@Fluent
public final class Ruleproperties implements JsonSerializable {
/*
* Represents the filter actions which are allowed for the transformation of a message that have been matched by a
* filter expression.
*/
private Action action;
/*
* Filter type that is evaluated against a BrokeredMessage.
*/
private FilterType filterType;
/*
* Properties of sqlFilter
*/
private SqlFilter sqlFilter;
/*
* Properties of correlationFilter
*/
private CorrelationFilter correlationFilter;
/**
* Creates an instance of Ruleproperties class.
*/
public Ruleproperties() {
}
/**
* Get the action property: Represents the filter actions which are allowed for the transformation of a message that
* have been matched by a filter expression.
*
* @return the action value.
*/
public Action action() {
return this.action;
}
/**
* Set the action property: Represents the filter actions which are allowed for the transformation of a message that
* have been matched by a filter expression.
*
* @param action the action value to set.
* @return the Ruleproperties object itself.
*/
public Ruleproperties withAction(Action action) {
this.action = action;
return this;
}
/**
* Get the filterType property: Filter type that is evaluated against a BrokeredMessage.
*
* @return the filterType value.
*/
public FilterType filterType() {
return this.filterType;
}
/**
* Set the filterType property: Filter type that is evaluated against a BrokeredMessage.
*
* @param filterType the filterType value to set.
* @return the Ruleproperties object itself.
*/
public Ruleproperties withFilterType(FilterType filterType) {
this.filterType = filterType;
return this;
}
/**
* Get the sqlFilter property: Properties of sqlFilter.
*
* @return the sqlFilter value.
*/
public SqlFilter sqlFilter() {
return this.sqlFilter;
}
/**
* Set the sqlFilter property: Properties of sqlFilter.
*
* @param sqlFilter the sqlFilter value to set.
* @return the Ruleproperties object itself.
*/
public Ruleproperties withSqlFilter(SqlFilter sqlFilter) {
this.sqlFilter = sqlFilter;
return this;
}
/**
* Get the correlationFilter property: Properties of correlationFilter.
*
* @return the correlationFilter value.
*/
public CorrelationFilter correlationFilter() {
return this.correlationFilter;
}
/**
* Set the correlationFilter property: Properties of correlationFilter.
*
* @param correlationFilter the correlationFilter value to set.
* @return the Ruleproperties object itself.
*/
public Ruleproperties withCorrelationFilter(CorrelationFilter correlationFilter) {
this.correlationFilter = correlationFilter;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (action() != null) {
action().validate();
}
if (sqlFilter() != null) {
sqlFilter().validate();
}
if (correlationFilter() != null) {
correlationFilter().validate();
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeJsonField("action", this.action);
jsonWriter.writeStringField("filterType", this.filterType == null ? null : this.filterType.toString());
jsonWriter.writeJsonField("sqlFilter", this.sqlFilter);
jsonWriter.writeJsonField("correlationFilter", this.correlationFilter);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of Ruleproperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of Ruleproperties if the JsonReader was pointing to an instance of it, or null if it was
* pointing to JSON null.
* @throws IOException If an error occurs while reading the Ruleproperties.
*/
public static Ruleproperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
Ruleproperties deserializedRuleproperties = new Ruleproperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("action".equals(fieldName)) {
deserializedRuleproperties.action = Action.fromJson(reader);
} else if ("filterType".equals(fieldName)) {
deserializedRuleproperties.filterType = FilterType.fromString(reader.getString());
} else if ("sqlFilter".equals(fieldName)) {
deserializedRuleproperties.sqlFilter = SqlFilter.fromJson(reader);
} else if ("correlationFilter".equals(fieldName)) {
deserializedRuleproperties.correlationFilter = CorrelationFilter.fromJson(reader);
} else {
reader.skipChildren();
}
}
return deserializedRuleproperties;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy