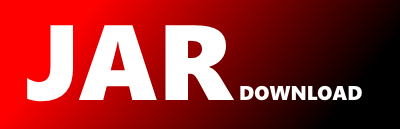
com.azure.resourcemanager.servicebus.implementation.ServiceBusChildResourcesImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-servicebus Show documentation
Show all versions of azure-resourcemanager-servicebus Show documentation
This package contains Microsoft Azure ServiceBus Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
package com.azure.resourcemanager.servicebus.implementation;
import com.azure.core.http.rest.PagedFlux;
import com.azure.core.http.rest.PagedIterable;
import com.azure.resourcemanager.resources.fluentcore.arm.Manager;
import com.azure.resourcemanager.resources.fluentcore.arm.collection.SupportsGettingByName;
import com.azure.resourcemanager.resources.fluentcore.arm.collection.implementation.IndependentChildResourcesImpl;
import com.azure.resourcemanager.resources.fluentcore.arm.models.HasResourceGroup;
import com.azure.resourcemanager.resources.fluentcore.arm.models.IndependentChildResource;
import com.azure.resourcemanager.resources.fluentcore.arm.models.Resource;
import com.azure.resourcemanager.resources.fluentcore.collection.SupportsDeletingByName;
import com.azure.resourcemanager.resources.fluentcore.collection.SupportsListing;
import reactor.core.publisher.Flux;
import reactor.core.publisher.Mono;
import java.util.List;
import com.azure.resourcemanager.resources.fluentcore.utils.PagedConverter;
/**
* Base class for Service Bus child entities.
* Note: When we refactor 'IndependentChildResourcesImpl', move features of this type
* to 'IndependentChildResourcesImpl' and remove this type.
*
* @param the model interface type
* @param the model interface implementation
* @param the inner model
* @param the inner collection
* @param the manager
* @param the parent model interface type
*/
abstract class ServiceBusChildResourcesImpl<
T extends IndependentChildResource,
ImplT extends T,
InnerT,
InnerCollectionT,
ManagerT extends Manager>,
ParentT extends Resource & HasResourceGroup>
extends IndependentChildResourcesImpl
implements SupportsGettingByName, SupportsListing, SupportsDeletingByName {
protected ServiceBusChildResourcesImpl(InnerCollectionT innerCollection, ManagerT manager) {
super(innerCollection, manager);
}
@Override
public Mono getByNameAsync(String name) {
return getInnerByNameAsync(name)
.map(this::wrapModel);
}
@Override
public T getByName(String name) {
return getByNameAsync(name).block();
}
@Override
public PagedFlux listAsync() {
return PagedConverter.mapPage(this.listInnerAsync(),
this::wrapModel);
}
@Override
public PagedIterable list() {
return this.wrapList(this.listInner());
}
@Override
public void deleteByName(String name) {
this.deleteByNameAsync(name).block();
}
public Flux deleteByNameAsync(List names) {
if (names == null) {
return Flux.empty();
}
return Flux.fromIterable(names)
.flatMapDelayError(name -> deleteByNameAsync(name), 32, 32);
}
protected abstract Mono getInnerByNameAsync(String name);
protected abstract PagedFlux listInnerAsync();
protected abstract PagedIterable listInner();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy