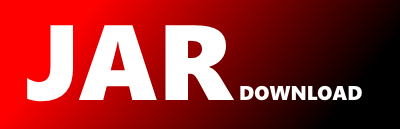
com.azure.resourcemanager.servicebus.implementation.ServiceBusManagementClientImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-servicebus Show documentation
Show all versions of azure-resourcemanager-servicebus Show documentation
This package contains Microsoft Azure ServiceBus Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.servicebus.implementation;
import com.azure.core.annotation.ServiceClient;
import com.azure.core.http.HttpPipeline;
import com.azure.core.management.AzureEnvironment;
import com.azure.core.util.serializer.SerializerAdapter;
import com.azure.resourcemanager.resources.fluentcore.AzureServiceClient;
import com.azure.resourcemanager.servicebus.fluent.DisasterRecoveryConfigsClient;
import com.azure.resourcemanager.servicebus.fluent.MigrationConfigsClient;
import com.azure.resourcemanager.servicebus.fluent.NamespacesClient;
import com.azure.resourcemanager.servicebus.fluent.OperationsClient;
import com.azure.resourcemanager.servicebus.fluent.PrivateEndpointConnectionsClient;
import com.azure.resourcemanager.servicebus.fluent.PrivateLinkResourcesClient;
import com.azure.resourcemanager.servicebus.fluent.QueuesClient;
import com.azure.resourcemanager.servicebus.fluent.RulesClient;
import com.azure.resourcemanager.servicebus.fluent.ServiceBusManagementClient;
import com.azure.resourcemanager.servicebus.fluent.SubscriptionsClient;
import com.azure.resourcemanager.servicebus.fluent.TopicsClient;
import java.time.Duration;
/**
* Initializes a new instance of the ServiceBusManagementClientImpl type.
*/
@ServiceClient(builder = ServiceBusManagementClientBuilder.class)
public final class ServiceBusManagementClientImpl extends AzureServiceClient implements ServiceBusManagementClient {
/**
* Subscription credentials that uniquely identify a Microsoft Azure subscription. The subscription ID forms part of
* the URI for every service call.
*/
private final String subscriptionId;
/**
* Gets Subscription credentials that uniquely identify a Microsoft Azure subscription. The subscription ID forms
* part of the URI for every service call.
*
* @return the subscriptionId value.
*/
public String getSubscriptionId() {
return this.subscriptionId;
}
/**
* server parameter.
*/
private final String endpoint;
/**
* Gets server parameter.
*
* @return the endpoint value.
*/
public String getEndpoint() {
return this.endpoint;
}
/**
* Api Version.
*/
private final String apiVersion;
/**
* Gets Api Version.
*
* @return the apiVersion value.
*/
public String getApiVersion() {
return this.apiVersion;
}
/**
* The HTTP pipeline to send requests through.
*/
private final HttpPipeline httpPipeline;
/**
* Gets The HTTP pipeline to send requests through.
*
* @return the httpPipeline value.
*/
public HttpPipeline getHttpPipeline() {
return this.httpPipeline;
}
/**
* The serializer to serialize an object into a string.
*/
private final SerializerAdapter serializerAdapter;
/**
* Gets The serializer to serialize an object into a string.
*
* @return the serializerAdapter value.
*/
SerializerAdapter getSerializerAdapter() {
return this.serializerAdapter;
}
/**
* The default poll interval for long-running operation.
*/
private final Duration defaultPollInterval;
/**
* Gets The default poll interval for long-running operation.
*
* @return the defaultPollInterval value.
*/
public Duration getDefaultPollInterval() {
return this.defaultPollInterval;
}
/**
* The NamespacesClient object to access its operations.
*/
private final NamespacesClient namespaces;
/**
* Gets the NamespacesClient object to access its operations.
*
* @return the NamespacesClient object.
*/
public NamespacesClient getNamespaces() {
return this.namespaces;
}
/**
* The PrivateEndpointConnectionsClient object to access its operations.
*/
private final PrivateEndpointConnectionsClient privateEndpointConnections;
/**
* Gets the PrivateEndpointConnectionsClient object to access its operations.
*
* @return the PrivateEndpointConnectionsClient object.
*/
public PrivateEndpointConnectionsClient getPrivateEndpointConnections() {
return this.privateEndpointConnections;
}
/**
* The PrivateLinkResourcesClient object to access its operations.
*/
private final PrivateLinkResourcesClient privateLinkResources;
/**
* Gets the PrivateLinkResourcesClient object to access its operations.
*
* @return the PrivateLinkResourcesClient object.
*/
public PrivateLinkResourcesClient getPrivateLinkResources() {
return this.privateLinkResources;
}
/**
* The OperationsClient object to access its operations.
*/
private final OperationsClient operations;
/**
* Gets the OperationsClient object to access its operations.
*
* @return the OperationsClient object.
*/
public OperationsClient getOperations() {
return this.operations;
}
/**
* The DisasterRecoveryConfigsClient object to access its operations.
*/
private final DisasterRecoveryConfigsClient disasterRecoveryConfigs;
/**
* Gets the DisasterRecoveryConfigsClient object to access its operations.
*
* @return the DisasterRecoveryConfigsClient object.
*/
public DisasterRecoveryConfigsClient getDisasterRecoveryConfigs() {
return this.disasterRecoveryConfigs;
}
/**
* The MigrationConfigsClient object to access its operations.
*/
private final MigrationConfigsClient migrationConfigs;
/**
* Gets the MigrationConfigsClient object to access its operations.
*
* @return the MigrationConfigsClient object.
*/
public MigrationConfigsClient getMigrationConfigs() {
return this.migrationConfigs;
}
/**
* The QueuesClient object to access its operations.
*/
private final QueuesClient queues;
/**
* Gets the QueuesClient object to access its operations.
*
* @return the QueuesClient object.
*/
public QueuesClient getQueues() {
return this.queues;
}
/**
* The TopicsClient object to access its operations.
*/
private final TopicsClient topics;
/**
* Gets the TopicsClient object to access its operations.
*
* @return the TopicsClient object.
*/
public TopicsClient getTopics() {
return this.topics;
}
/**
* The RulesClient object to access its operations.
*/
private final RulesClient rules;
/**
* Gets the RulesClient object to access its operations.
*
* @return the RulesClient object.
*/
public RulesClient getRules() {
return this.rules;
}
/**
* The SubscriptionsClient object to access its operations.
*/
private final SubscriptionsClient subscriptions;
/**
* Gets the SubscriptionsClient object to access its operations.
*
* @return the SubscriptionsClient object.
*/
public SubscriptionsClient getSubscriptions() {
return this.subscriptions;
}
/**
* Initializes an instance of ServiceBusManagementClient client.
*
* @param httpPipeline The HTTP pipeline to send requests through.
* @param serializerAdapter The serializer to serialize an object into a string.
* @param defaultPollInterval The default poll interval for long-running operation.
* @param environment The Azure environment.
* @param subscriptionId Subscription credentials that uniquely identify a Microsoft Azure subscription. The
* subscription ID forms part of the URI for every service call.
* @param endpoint server parameter.
*/
ServiceBusManagementClientImpl(HttpPipeline httpPipeline, SerializerAdapter serializerAdapter,
Duration defaultPollInterval, AzureEnvironment environment, String subscriptionId, String endpoint) {
super(httpPipeline, serializerAdapter, environment);
this.httpPipeline = httpPipeline;
this.serializerAdapter = serializerAdapter;
this.defaultPollInterval = defaultPollInterval;
this.subscriptionId = subscriptionId;
this.endpoint = endpoint;
this.apiVersion = "2021-11-01";
this.namespaces = new NamespacesClientImpl(this);
this.privateEndpointConnections = new PrivateEndpointConnectionsClientImpl(this);
this.privateLinkResources = new PrivateLinkResourcesClientImpl(this);
this.operations = new OperationsClientImpl(this);
this.disasterRecoveryConfigs = new DisasterRecoveryConfigsClientImpl(this);
this.migrationConfigs = new MigrationConfigsClientImpl(this);
this.queues = new QueuesClientImpl(this);
this.topics = new TopicsClientImpl(this);
this.rules = new RulesClientImpl(this);
this.subscriptions = new SubscriptionsClientImpl(this);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy