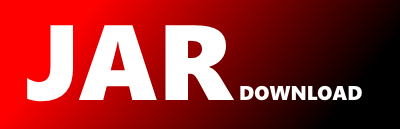
com.azure.resourcemanager.servicebus.implementation.TopicAuthorizationRuleImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-servicebus Show documentation
Show all versions of azure-resourcemanager-servicebus Show documentation
This package contains Microsoft Azure ServiceBus Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
package com.azure.resourcemanager.servicebus.implementation;
import com.azure.resourcemanager.servicebus.ServiceBusManager;
import com.azure.resourcemanager.servicebus.fluent.models.AccessKeysInner;
import com.azure.resourcemanager.servicebus.fluent.models.SBAuthorizationRuleInner;
import com.azure.resourcemanager.servicebus.models.RegenerateAccessKeyParameters;
import com.azure.resourcemanager.servicebus.models.TopicAuthorizationRule;
import reactor.core.publisher.Mono;
/**
* Implementation for TopicAuthorizationRule.
*/
class TopicAuthorizationRuleImpl
extends AuthorizationRuleBaseImpl
implements
TopicAuthorizationRule,
TopicAuthorizationRule.Definition,
TopicAuthorizationRule.Update {
private final String namespaceName;
TopicAuthorizationRuleImpl(String resourceGroupName,
String namespaceName,
String topicName,
String name,
SBAuthorizationRuleInner inner,
ServiceBusManager manager) {
super(name, inner, manager);
this.namespaceName = namespaceName;
this.withExistingParentResource(resourceGroupName, topicName);
}
@Override
public String namespaceName() {
return this.namespaceName;
}
@Override
public String topicName() {
return this.parentName;
}
@Override
protected Mono getInnerAsync() {
return this.manager().serviceClient().getTopics()
.getAuthorizationRuleAsync(this.resourceGroupName(),
this.namespaceName(),
this.topicName(),
this.name());
}
@Override
protected Mono createChildResourceAsync() {
final TopicAuthorizationRule self = this;
return this.manager().serviceClient().getTopics().createOrUpdateAuthorizationRuleAsync(this.resourceGroupName(),
this.namespaceName(),
this.topicName(),
this.name(),
this.innerModel())
.map(inner -> {
setInner(inner);
return self;
});
}
@Override
protected Mono getKeysInnerAsync() {
return this.manager().serviceClient().getTopics()
.listKeysAsync(this.resourceGroupName(),
this.namespaceName(),
this.topicName(),
this.name());
}
@Override
protected Mono regenerateKeysInnerAsync(RegenerateAccessKeyParameters regenerateAccessKeyParameters) {
return this.manager().serviceClient().getTopics().regenerateKeysAsync(this.resourceGroupName(),
this.namespaceName(),
this.topicName(),
this.name(),
regenerateAccessKeyParameters);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy