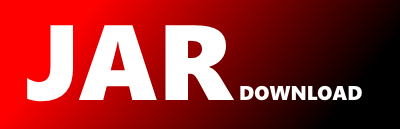
com.azure.resourcemanager.servicefabricmanagedclusters.ServiceFabricManagedClustersManager Maven / Gradle / Ivy
Show all versions of azure-resourcemanager-servicefabricmanagedclusters Show documentation
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.servicefabricmanagedclusters;
import com.azure.core.credential.TokenCredential;
import com.azure.core.http.HttpClient;
import com.azure.core.http.HttpPipeline;
import com.azure.core.http.HttpPipelineBuilder;
import com.azure.core.http.HttpPipelinePosition;
import com.azure.core.http.policy.AddDatePolicy;
import com.azure.core.http.policy.AddHeadersFromContextPolicy;
import com.azure.core.http.policy.HttpLoggingPolicy;
import com.azure.core.http.policy.HttpLogOptions;
import com.azure.core.http.policy.HttpPipelinePolicy;
import com.azure.core.http.policy.HttpPolicyProviders;
import com.azure.core.http.policy.RequestIdPolicy;
import com.azure.core.http.policy.RetryOptions;
import com.azure.core.http.policy.RetryPolicy;
import com.azure.core.http.policy.UserAgentPolicy;
import com.azure.core.management.http.policy.ArmChallengeAuthenticationPolicy;
import com.azure.core.management.profile.AzureProfile;
import com.azure.core.util.Configuration;
import com.azure.core.util.logging.ClientLogger;
import com.azure.resourcemanager.servicefabricmanagedclusters.fluent.ServiceFabricManagedClustersMgmtClient;
import com.azure.resourcemanager.servicefabricmanagedclusters.implementation.ApplicationsImpl;
import com.azure.resourcemanager.servicefabricmanagedclusters.implementation.ApplicationTypesImpl;
import com.azure.resourcemanager.servicefabricmanagedclusters.implementation.ApplicationTypeVersionsImpl;
import com.azure.resourcemanager.servicefabricmanagedclusters.implementation.ManagedApplyMaintenanceWindowsImpl;
import com.azure.resourcemanager.servicefabricmanagedclusters.implementation.ManagedAzResiliencyStatusesImpl;
import com.azure.resourcemanager.servicefabricmanagedclusters.implementation.ManagedClustersImpl;
import com.azure.resourcemanager.servicefabricmanagedclusters.implementation.ManagedClusterVersionsImpl;
import com.azure.resourcemanager.servicefabricmanagedclusters.implementation.ManagedMaintenanceWindowStatusesImpl;
import com.azure.resourcemanager.servicefabricmanagedclusters.implementation.ManagedUnsupportedVMSizesImpl;
import com.azure.resourcemanager.servicefabricmanagedclusters.implementation.NodeTypesImpl;
import com.azure.resourcemanager.servicefabricmanagedclusters.implementation.NodeTypeSkusImpl;
import com.azure.resourcemanager.servicefabricmanagedclusters.implementation.OperationResultsImpl;
import com.azure.resourcemanager.servicefabricmanagedclusters.implementation.OperationsImpl;
import com.azure.resourcemanager.servicefabricmanagedclusters.implementation.OperationStatusImpl;
import com.azure.resourcemanager.servicefabricmanagedclusters.implementation.ServiceFabricManagedClustersMgmtClientBuilder;
import com.azure.resourcemanager.servicefabricmanagedclusters.implementation.ServicesImpl;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.Applications;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.ApplicationTypes;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.ApplicationTypeVersions;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.ManagedApplyMaintenanceWindows;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.ManagedAzResiliencyStatuses;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.ManagedClusters;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.ManagedClusterVersions;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.ManagedMaintenanceWindowStatuses;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.ManagedUnsupportedVMSizes;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.NodeTypes;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.NodeTypeSkus;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.OperationResults;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.Operations;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.OperationStatus;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.Services;
import java.time.Duration;
import java.time.temporal.ChronoUnit;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import java.util.stream.Collectors;
/**
* Entry point to ServiceFabricManagedClustersManager.
* Service Fabric Managed Clusters Management Client.
*/
public final class ServiceFabricManagedClustersManager {
private ApplicationTypes applicationTypes;
private ApplicationTypeVersions applicationTypeVersions;
private Applications applications;
private Services services;
private ManagedClusters managedClusters;
private ManagedAzResiliencyStatuses managedAzResiliencyStatuses;
private ManagedMaintenanceWindowStatuses managedMaintenanceWindowStatuses;
private ManagedApplyMaintenanceWindows managedApplyMaintenanceWindows;
private ManagedClusterVersions managedClusterVersions;
private ManagedUnsupportedVMSizes managedUnsupportedVMSizes;
private OperationStatus operationStatus;
private OperationResults operationResults;
private Operations operations;
private NodeTypes nodeTypes;
private NodeTypeSkus nodeTypeSkus;
private final ServiceFabricManagedClustersMgmtClient clientObject;
private ServiceFabricManagedClustersManager(HttpPipeline httpPipeline, AzureProfile profile,
Duration defaultPollInterval) {
Objects.requireNonNull(httpPipeline, "'httpPipeline' cannot be null.");
Objects.requireNonNull(profile, "'profile' cannot be null.");
this.clientObject = new ServiceFabricManagedClustersMgmtClientBuilder().pipeline(httpPipeline)
.endpoint(profile.getEnvironment().getResourceManagerEndpoint())
.subscriptionId(profile.getSubscriptionId())
.defaultPollInterval(defaultPollInterval)
.buildClient();
}
/**
* Creates an instance of Service Fabric Managed Clusters service API entry point.
*
* @param credential the credential to use.
* @param profile the Azure profile for client.
* @return the Service Fabric Managed Clusters service API instance.
*/
public static ServiceFabricManagedClustersManager authenticate(TokenCredential credential, AzureProfile profile) {
Objects.requireNonNull(credential, "'credential' cannot be null.");
Objects.requireNonNull(profile, "'profile' cannot be null.");
return configure().authenticate(credential, profile);
}
/**
* Creates an instance of Service Fabric Managed Clusters service API entry point.
*
* @param httpPipeline the {@link HttpPipeline} configured with Azure authentication credential.
* @param profile the Azure profile for client.
* @return the Service Fabric Managed Clusters service API instance.
*/
public static ServiceFabricManagedClustersManager authenticate(HttpPipeline httpPipeline, AzureProfile profile) {
Objects.requireNonNull(httpPipeline, "'httpPipeline' cannot be null.");
Objects.requireNonNull(profile, "'profile' cannot be null.");
return new ServiceFabricManagedClustersManager(httpPipeline, profile, null);
}
/**
* Gets a Configurable instance that can be used to create ServiceFabricManagedClustersManager with optional
* configuration.
*
* @return the Configurable instance allowing configurations.
*/
public static Configurable configure() {
return new ServiceFabricManagedClustersManager.Configurable();
}
/**
* The Configurable allowing configurations to be set.
*/
public static final class Configurable {
private static final ClientLogger LOGGER = new ClientLogger(Configurable.class);
private HttpClient httpClient;
private HttpLogOptions httpLogOptions;
private final List policies = new ArrayList<>();
private final List scopes = new ArrayList<>();
private RetryPolicy retryPolicy;
private RetryOptions retryOptions;
private Duration defaultPollInterval;
private Configurable() {
}
/**
* Sets the http client.
*
* @param httpClient the HTTP client.
* @return the configurable object itself.
*/
public Configurable withHttpClient(HttpClient httpClient) {
this.httpClient = Objects.requireNonNull(httpClient, "'httpClient' cannot be null.");
return this;
}
/**
* Sets the logging options to the HTTP pipeline.
*
* @param httpLogOptions the HTTP log options.
* @return the configurable object itself.
*/
public Configurable withLogOptions(HttpLogOptions httpLogOptions) {
this.httpLogOptions = Objects.requireNonNull(httpLogOptions, "'httpLogOptions' cannot be null.");
return this;
}
/**
* Adds the pipeline policy to the HTTP pipeline.
*
* @param policy the HTTP pipeline policy.
* @return the configurable object itself.
*/
public Configurable withPolicy(HttpPipelinePolicy policy) {
this.policies.add(Objects.requireNonNull(policy, "'policy' cannot be null."));
return this;
}
/**
* Adds the scope to permission sets.
*
* @param scope the scope.
* @return the configurable object itself.
*/
public Configurable withScope(String scope) {
this.scopes.add(Objects.requireNonNull(scope, "'scope' cannot be null."));
return this;
}
/**
* Sets the retry policy to the HTTP pipeline.
*
* @param retryPolicy the HTTP pipeline retry policy.
* @return the configurable object itself.
*/
public Configurable withRetryPolicy(RetryPolicy retryPolicy) {
this.retryPolicy = Objects.requireNonNull(retryPolicy, "'retryPolicy' cannot be null.");
return this;
}
/**
* Sets the retry options for the HTTP pipeline retry policy.
*
* This setting has no effect, if retry policy is set via {@link #withRetryPolicy(RetryPolicy)}.
*
* @param retryOptions the retry options for the HTTP pipeline retry policy.
* @return the configurable object itself.
*/
public Configurable withRetryOptions(RetryOptions retryOptions) {
this.retryOptions = Objects.requireNonNull(retryOptions, "'retryOptions' cannot be null.");
return this;
}
/**
* Sets the default poll interval, used when service does not provide "Retry-After" header.
*
* @param defaultPollInterval the default poll interval.
* @return the configurable object itself.
*/
public Configurable withDefaultPollInterval(Duration defaultPollInterval) {
this.defaultPollInterval
= Objects.requireNonNull(defaultPollInterval, "'defaultPollInterval' cannot be null.");
if (this.defaultPollInterval.isNegative()) {
throw LOGGER
.logExceptionAsError(new IllegalArgumentException("'defaultPollInterval' cannot be negative"));
}
return this;
}
/**
* Creates an instance of Service Fabric Managed Clusters service API entry point.
*
* @param credential the credential to use.
* @param profile the Azure profile for client.
* @return the Service Fabric Managed Clusters service API instance.
*/
public ServiceFabricManagedClustersManager authenticate(TokenCredential credential, AzureProfile profile) {
Objects.requireNonNull(credential, "'credential' cannot be null.");
Objects.requireNonNull(profile, "'profile' cannot be null.");
StringBuilder userAgentBuilder = new StringBuilder();
userAgentBuilder.append("azsdk-java")
.append("-")
.append("com.azure.resourcemanager.servicefabricmanagedclusters")
.append("/")
.append("1.0.0-beta.1");
if (!Configuration.getGlobalConfiguration().get("AZURE_TELEMETRY_DISABLED", false)) {
userAgentBuilder.append(" (")
.append(Configuration.getGlobalConfiguration().get("java.version"))
.append("; ")
.append(Configuration.getGlobalConfiguration().get("os.name"))
.append("; ")
.append(Configuration.getGlobalConfiguration().get("os.version"))
.append("; auto-generated)");
} else {
userAgentBuilder.append(" (auto-generated)");
}
if (scopes.isEmpty()) {
scopes.add(profile.getEnvironment().getManagementEndpoint() + "/.default");
}
if (retryPolicy == null) {
if (retryOptions != null) {
retryPolicy = new RetryPolicy(retryOptions);
} else {
retryPolicy = new RetryPolicy("Retry-After", ChronoUnit.SECONDS);
}
}
List policies = new ArrayList<>();
policies.add(new UserAgentPolicy(userAgentBuilder.toString()));
policies.add(new AddHeadersFromContextPolicy());
policies.add(new RequestIdPolicy());
policies.addAll(this.policies.stream()
.filter(p -> p.getPipelinePosition() == HttpPipelinePosition.PER_CALL)
.collect(Collectors.toList()));
HttpPolicyProviders.addBeforeRetryPolicies(policies);
policies.add(retryPolicy);
policies.add(new AddDatePolicy());
policies.add(new ArmChallengeAuthenticationPolicy(credential, scopes.toArray(new String[0])));
policies.addAll(this.policies.stream()
.filter(p -> p.getPipelinePosition() == HttpPipelinePosition.PER_RETRY)
.collect(Collectors.toList()));
HttpPolicyProviders.addAfterRetryPolicies(policies);
policies.add(new HttpLoggingPolicy(httpLogOptions));
HttpPipeline httpPipeline = new HttpPipelineBuilder().httpClient(httpClient)
.policies(policies.toArray(new HttpPipelinePolicy[0]))
.build();
return new ServiceFabricManagedClustersManager(httpPipeline, profile, defaultPollInterval);
}
}
/**
* Gets the resource collection API of ApplicationTypes. It manages ApplicationTypeResource.
*
* @return Resource collection API of ApplicationTypes.
*/
public ApplicationTypes applicationTypes() {
if (this.applicationTypes == null) {
this.applicationTypes = new ApplicationTypesImpl(clientObject.getApplicationTypes(), this);
}
return applicationTypes;
}
/**
* Gets the resource collection API of ApplicationTypeVersions. It manages ApplicationTypeVersionResource.
*
* @return Resource collection API of ApplicationTypeVersions.
*/
public ApplicationTypeVersions applicationTypeVersions() {
if (this.applicationTypeVersions == null) {
this.applicationTypeVersions
= new ApplicationTypeVersionsImpl(clientObject.getApplicationTypeVersions(), this);
}
return applicationTypeVersions;
}
/**
* Gets the resource collection API of Applications. It manages ApplicationResource.
*
* @return Resource collection API of Applications.
*/
public Applications applications() {
if (this.applications == null) {
this.applications = new ApplicationsImpl(clientObject.getApplications(), this);
}
return applications;
}
/**
* Gets the resource collection API of Services. It manages ServiceResource.
*
* @return Resource collection API of Services.
*/
public Services services() {
if (this.services == null) {
this.services = new ServicesImpl(clientObject.getServices(), this);
}
return services;
}
/**
* Gets the resource collection API of ManagedClusters. It manages ManagedCluster.
*
* @return Resource collection API of ManagedClusters.
*/
public ManagedClusters managedClusters() {
if (this.managedClusters == null) {
this.managedClusters = new ManagedClustersImpl(clientObject.getManagedClusters(), this);
}
return managedClusters;
}
/**
* Gets the resource collection API of ManagedAzResiliencyStatuses.
*
* @return Resource collection API of ManagedAzResiliencyStatuses.
*/
public ManagedAzResiliencyStatuses managedAzResiliencyStatuses() {
if (this.managedAzResiliencyStatuses == null) {
this.managedAzResiliencyStatuses
= new ManagedAzResiliencyStatusesImpl(clientObject.getManagedAzResiliencyStatuses(), this);
}
return managedAzResiliencyStatuses;
}
/**
* Gets the resource collection API of ManagedMaintenanceWindowStatuses.
*
* @return Resource collection API of ManagedMaintenanceWindowStatuses.
*/
public ManagedMaintenanceWindowStatuses managedMaintenanceWindowStatuses() {
if (this.managedMaintenanceWindowStatuses == null) {
this.managedMaintenanceWindowStatuses
= new ManagedMaintenanceWindowStatusesImpl(clientObject.getManagedMaintenanceWindowStatuses(), this);
}
return managedMaintenanceWindowStatuses;
}
/**
* Gets the resource collection API of ManagedApplyMaintenanceWindows.
*
* @return Resource collection API of ManagedApplyMaintenanceWindows.
*/
public ManagedApplyMaintenanceWindows managedApplyMaintenanceWindows() {
if (this.managedApplyMaintenanceWindows == null) {
this.managedApplyMaintenanceWindows
= new ManagedApplyMaintenanceWindowsImpl(clientObject.getManagedApplyMaintenanceWindows(), this);
}
return managedApplyMaintenanceWindows;
}
/**
* Gets the resource collection API of ManagedClusterVersions.
*
* @return Resource collection API of ManagedClusterVersions.
*/
public ManagedClusterVersions managedClusterVersions() {
if (this.managedClusterVersions == null) {
this.managedClusterVersions
= new ManagedClusterVersionsImpl(clientObject.getManagedClusterVersions(), this);
}
return managedClusterVersions;
}
/**
* Gets the resource collection API of ManagedUnsupportedVMSizes.
*
* @return Resource collection API of ManagedUnsupportedVMSizes.
*/
public ManagedUnsupportedVMSizes managedUnsupportedVMSizes() {
if (this.managedUnsupportedVMSizes == null) {
this.managedUnsupportedVMSizes
= new ManagedUnsupportedVMSizesImpl(clientObject.getManagedUnsupportedVMSizes(), this);
}
return managedUnsupportedVMSizes;
}
/**
* Gets the resource collection API of OperationStatus.
*
* @return Resource collection API of OperationStatus.
*/
public OperationStatus operationStatus() {
if (this.operationStatus == null) {
this.operationStatus = new OperationStatusImpl(clientObject.getOperationStatus(), this);
}
return operationStatus;
}
/**
* Gets the resource collection API of OperationResults.
*
* @return Resource collection API of OperationResults.
*/
public OperationResults operationResults() {
if (this.operationResults == null) {
this.operationResults = new OperationResultsImpl(clientObject.getOperationResults(), this);
}
return operationResults;
}
/**
* Gets the resource collection API of Operations.
*
* @return Resource collection API of Operations.
*/
public Operations operations() {
if (this.operations == null) {
this.operations = new OperationsImpl(clientObject.getOperations(), this);
}
return operations;
}
/**
* Gets the resource collection API of NodeTypes. It manages NodeType.
*
* @return Resource collection API of NodeTypes.
*/
public NodeTypes nodeTypes() {
if (this.nodeTypes == null) {
this.nodeTypes = new NodeTypesImpl(clientObject.getNodeTypes(), this);
}
return nodeTypes;
}
/**
* Gets the resource collection API of NodeTypeSkus.
*
* @return Resource collection API of NodeTypeSkus.
*/
public NodeTypeSkus nodeTypeSkus() {
if (this.nodeTypeSkus == null) {
this.nodeTypeSkus = new NodeTypeSkusImpl(clientObject.getNodeTypeSkus(), this);
}
return nodeTypeSkus;
}
/**
* Gets wrapped service client ServiceFabricManagedClustersMgmtClient providing direct access to the underlying
* auto-generated API implementation, based on Azure REST API.
*
* @return Wrapped service client ServiceFabricManagedClustersMgmtClient.
*/
public ServiceFabricManagedClustersMgmtClient serviceClient() {
return this.clientObject;
}
}