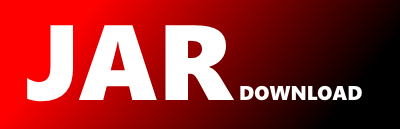
com.azure.resourcemanager.servicefabricmanagedclusters.fluent.ServicesClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-servicefabricmanagedclusters Show documentation
Show all versions of azure-resourcemanager-servicefabricmanagedclusters Show documentation
This package contains Microsoft Azure SDK for Service Fabric Managed Clusters Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Service Fabric Managed Clusters Management Client. Package tag package-2024-04.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.servicefabricmanagedclusters.fluent;
import com.azure.core.annotation.ReturnType;
import com.azure.core.annotation.ServiceMethod;
import com.azure.core.http.rest.PagedIterable;
import com.azure.core.http.rest.Response;
import com.azure.core.management.polling.PollResult;
import com.azure.core.util.Context;
import com.azure.core.util.polling.SyncPoller;
import com.azure.resourcemanager.servicefabricmanagedclusters.fluent.models.ServiceResourceInner;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.ServiceUpdateParameters;
/**
* An instance of this class provides access to all the operations defined in ServicesClient.
*/
public interface ServicesClient {
/**
* Gets a Service Fabric managed service resource.
*
* Get a Service Fabric service resource created or in the process of being created in the Service Fabric managed
* application resource.
*
* @param resourceGroupName The name of the resource group.
* @param clusterName The name of the cluster resource.
* @param applicationName The name of the application resource.
* @param serviceName The name of the service resource in the format of {applicationName}~{serviceName}.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a Service Fabric service resource created or in the process of being created in the Service Fabric
* managed application resource along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response getWithResponse(String resourceGroupName, String clusterName, String applicationName,
String serviceName, Context context);
/**
* Gets a Service Fabric managed service resource.
*
* Get a Service Fabric service resource created or in the process of being created in the Service Fabric managed
* application resource.
*
* @param resourceGroupName The name of the resource group.
* @param clusterName The name of the cluster resource.
* @param applicationName The name of the application resource.
* @param serviceName The name of the service resource in the format of {applicationName}~{serviceName}.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a Service Fabric service resource created or in the process of being created in the Service Fabric
* managed application resource.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ServiceResourceInner get(String resourceGroupName, String clusterName, String applicationName, String serviceName);
/**
* Creates or updates a Service Fabric managed service resource.
*
* Create or update a Service Fabric managed service resource with the specified name.
*
* @param resourceGroupName The name of the resource group.
* @param clusterName The name of the cluster resource.
* @param applicationName The name of the application resource.
* @param serviceName The name of the service resource in the format of {applicationName}~{serviceName}.
* @param parameters The service resource.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of the service resource.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, ServiceResourceInner> beginCreateOrUpdate(String resourceGroupName,
String clusterName, String applicationName, String serviceName, ServiceResourceInner parameters);
/**
* Creates or updates a Service Fabric managed service resource.
*
* Create or update a Service Fabric managed service resource with the specified name.
*
* @param resourceGroupName The name of the resource group.
* @param clusterName The name of the cluster resource.
* @param applicationName The name of the application resource.
* @param serviceName The name of the service resource in the format of {applicationName}~{serviceName}.
* @param parameters The service resource.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of the service resource.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, ServiceResourceInner> beginCreateOrUpdate(String resourceGroupName,
String clusterName, String applicationName, String serviceName, ServiceResourceInner parameters,
Context context);
/**
* Creates or updates a Service Fabric managed service resource.
*
* Create or update a Service Fabric managed service resource with the specified name.
*
* @param resourceGroupName The name of the resource group.
* @param clusterName The name of the cluster resource.
* @param applicationName The name of the application resource.
* @param serviceName The name of the service resource in the format of {applicationName}~{serviceName}.
* @param parameters The service resource.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the service resource.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ServiceResourceInner createOrUpdate(String resourceGroupName, String clusterName, String applicationName,
String serviceName, ServiceResourceInner parameters);
/**
* Creates or updates a Service Fabric managed service resource.
*
* Create or update a Service Fabric managed service resource with the specified name.
*
* @param resourceGroupName The name of the resource group.
* @param clusterName The name of the cluster resource.
* @param applicationName The name of the application resource.
* @param serviceName The name of the service resource in the format of {applicationName}~{serviceName}.
* @param parameters The service resource.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the service resource.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ServiceResourceInner createOrUpdate(String resourceGroupName, String clusterName, String applicationName,
String serviceName, ServiceResourceInner parameters, Context context);
/**
* Updates the tags of a service resource of a given managed cluster.
*
* @param resourceGroupName The name of the resource group.
* @param clusterName The name of the cluster resource.
* @param applicationName The name of the application resource.
* @param serviceName The name of the service resource in the format of {applicationName}~{serviceName}.
* @param parameters The service resource updated tags.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the service resource along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response updateWithResponse(String resourceGroupName, String clusterName,
String applicationName, String serviceName, ServiceUpdateParameters parameters, Context context);
/**
* Updates the tags of a service resource of a given managed cluster.
*
* @param resourceGroupName The name of the resource group.
* @param clusterName The name of the cluster resource.
* @param applicationName The name of the application resource.
* @param serviceName The name of the service resource in the format of {applicationName}~{serviceName}.
* @param parameters The service resource updated tags.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the service resource.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ServiceResourceInner update(String resourceGroupName, String clusterName, String applicationName,
String serviceName, ServiceUpdateParameters parameters);
/**
* Deletes a Service Fabric managed service resource.
*
* Delete a Service Fabric managed service resource with the specified name.
*
* @param resourceGroupName The name of the resource group.
* @param clusterName The name of the cluster resource.
* @param applicationName The name of the application resource.
* @param serviceName The name of the service resource in the format of {applicationName}~{serviceName}.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDelete(String resourceGroupName, String clusterName, String applicationName,
String serviceName);
/**
* Deletes a Service Fabric managed service resource.
*
* Delete a Service Fabric managed service resource with the specified name.
*
* @param resourceGroupName The name of the resource group.
* @param clusterName The name of the cluster resource.
* @param applicationName The name of the application resource.
* @param serviceName The name of the service resource in the format of {applicationName}~{serviceName}.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDelete(String resourceGroupName, String clusterName, String applicationName,
String serviceName, Context context);
/**
* Deletes a Service Fabric managed service resource.
*
* Delete a Service Fabric managed service resource with the specified name.
*
* @param resourceGroupName The name of the resource group.
* @param clusterName The name of the cluster resource.
* @param applicationName The name of the application resource.
* @param serviceName The name of the service resource in the format of {applicationName}~{serviceName}.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void delete(String resourceGroupName, String clusterName, String applicationName, String serviceName);
/**
* Deletes a Service Fabric managed service resource.
*
* Delete a Service Fabric managed service resource with the specified name.
*
* @param resourceGroupName The name of the resource group.
* @param clusterName The name of the cluster resource.
* @param applicationName The name of the application resource.
* @param serviceName The name of the service resource in the format of {applicationName}~{serviceName}.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void delete(String resourceGroupName, String clusterName, String applicationName, String serviceName,
Context context);
/**
* Gets the list of service resources created in the specified Service Fabric managed application resource.
*
* Gets all service resources created or in the process of being created in the Service Fabric managed application
* resource.
*
* @param resourceGroupName The name of the resource group.
* @param clusterName The name of the cluster resource.
* @param applicationName The name of the application resource.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return all service resources created or in the process of being created in the Service Fabric managed
* application resource as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listByApplications(String resourceGroupName, String clusterName,
String applicationName);
/**
* Gets the list of service resources created in the specified Service Fabric managed application resource.
*
* Gets all service resources created or in the process of being created in the Service Fabric managed application
* resource.
*
* @param resourceGroupName The name of the resource group.
* @param clusterName The name of the cluster resource.
* @param applicationName The name of the application resource.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return all service resources created or in the process of being created in the Service Fabric managed
* application resource as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listByApplications(String resourceGroupName, String clusterName,
String applicationName, Context context);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy