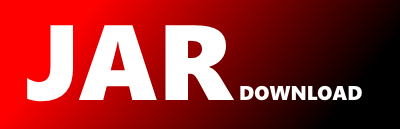
com.azure.resourcemanager.servicefabricmanagedclusters.fluent.models.ManagedClusterInner Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-servicefabricmanagedclusters Show documentation
Show all versions of azure-resourcemanager-servicefabricmanagedclusters Show documentation
This package contains Microsoft Azure SDK for Service Fabric Managed Clusters Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Service Fabric Managed Clusters Management Client. Package tag package-2024-04.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.servicefabricmanagedclusters.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.management.Resource;
import com.azure.core.management.SystemData;
import com.azure.core.util.logging.ClientLogger;
import com.azure.json.JsonReader;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.ApplicationTypeVersionsCleanupPolicy;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.AzureActiveDirectory;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.ClientCertificate;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.ClusterState;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.ClusterUpgradeCadence;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.ClusterUpgradeMode;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.ClusterUpgradePolicy;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.IpTag;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.LoadBalancingRule;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.ManagedClusterAddOnFeature;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.ManagedResourceProvisioningState;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.NetworkSecurityRule;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.ServiceEndpoint;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.SettingsSectionDescription;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.Sku;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.Subnet;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.ZonalUpdateMode;
import java.io.IOException;
import java.util.List;
import java.util.Map;
/**
* The managed cluster resource.
*/
@Fluent
public final class ManagedClusterInner extends Resource {
/*
* The managed cluster resource properties
*/
private ManagedClusterProperties innerProperties;
/*
* The sku of the managed cluster
*/
private Sku sku;
/*
* Azure resource etag.
*/
private String etag;
/*
* Metadata pertaining to creation and last modification of the resource.
*/
private SystemData systemData;
/*
* Fully qualified resource Id for the resource.
*/
private String id;
/*
* The name of the resource.
*/
private String name;
/*
* The type of the resource.
*/
private String type;
/**
* Creates an instance of ManagedClusterInner class.
*/
public ManagedClusterInner() {
}
/**
* Get the innerProperties property: The managed cluster resource properties.
*
* @return the innerProperties value.
*/
private ManagedClusterProperties innerProperties() {
return this.innerProperties;
}
/**
* Get the sku property: The sku of the managed cluster.
*
* @return the sku value.
*/
public Sku sku() {
return this.sku;
}
/**
* Set the sku property: The sku of the managed cluster.
*
* @param sku the sku value to set.
* @return the ManagedClusterInner object itself.
*/
public ManagedClusterInner withSku(Sku sku) {
this.sku = sku;
return this;
}
/**
* Get the etag property: Azure resource etag.
*
* @return the etag value.
*/
public String etag() {
return this.etag;
}
/**
* Get the systemData property: Metadata pertaining to creation and last modification of the resource.
*
* @return the systemData value.
*/
public SystemData systemData() {
return this.systemData;
}
/**
* Get the id property: Fully qualified resource Id for the resource.
*
* @return the id value.
*/
@Override
public String id() {
return this.id;
}
/**
* Get the name property: The name of the resource.
*
* @return the name value.
*/
@Override
public String name() {
return this.name;
}
/**
* Get the type property: The type of the resource.
*
* @return the type value.
*/
@Override
public String type() {
return this.type;
}
/**
* {@inheritDoc}
*/
@Override
public ManagedClusterInner withLocation(String location) {
super.withLocation(location);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ManagedClusterInner withTags(Map tags) {
super.withTags(tags);
return this;
}
/**
* Get the dnsName property: The cluster dns name.
*
* @return the dnsName value.
*/
public String dnsName() {
return this.innerProperties() == null ? null : this.innerProperties().dnsName();
}
/**
* Set the dnsName property: The cluster dns name.
*
* @param dnsName the dnsName value to set.
* @return the ManagedClusterInner object itself.
*/
public ManagedClusterInner withDnsName(String dnsName) {
if (this.innerProperties() == null) {
this.innerProperties = new ManagedClusterProperties();
}
this.innerProperties().withDnsName(dnsName);
return this;
}
/**
* Get the fqdn property: The fully qualified domain name associated with the public load balancer of the cluster.
*
* @return the fqdn value.
*/
public String fqdn() {
return this.innerProperties() == null ? null : this.innerProperties().fqdn();
}
/**
* Get the ipv4Address property: The IPv4 address associated with the public load balancer of the cluster.
*
* @return the ipv4Address value.
*/
public String ipv4Address() {
return this.innerProperties() == null ? null : this.innerProperties().ipv4Address();
}
/**
* Get the clusterId property: A service generated unique identifier for the cluster resource.
*
* @return the clusterId value.
*/
public String clusterId() {
return this.innerProperties() == null ? null : this.innerProperties().clusterId();
}
/**
* Get the clusterState property: The current state of the cluster.
*
* @return the clusterState value.
*/
public ClusterState clusterState() {
return this.innerProperties() == null ? null : this.innerProperties().clusterState();
}
/**
* Get the clusterCertificateThumbprints property: List of thumbprints of the cluster certificates.
*
* @return the clusterCertificateThumbprints value.
*/
public List clusterCertificateThumbprints() {
return this.innerProperties() == null ? null : this.innerProperties().clusterCertificateThumbprints();
}
/**
* Get the clientConnectionPort property: The port used for client connections to the cluster.
*
* @return the clientConnectionPort value.
*/
public Integer clientConnectionPort() {
return this.innerProperties() == null ? null : this.innerProperties().clientConnectionPort();
}
/**
* Set the clientConnectionPort property: The port used for client connections to the cluster.
*
* @param clientConnectionPort the clientConnectionPort value to set.
* @return the ManagedClusterInner object itself.
*/
public ManagedClusterInner withClientConnectionPort(Integer clientConnectionPort) {
if (this.innerProperties() == null) {
this.innerProperties = new ManagedClusterProperties();
}
this.innerProperties().withClientConnectionPort(clientConnectionPort);
return this;
}
/**
* Get the httpGatewayConnectionPort property: The port used for HTTP connections to the cluster.
*
* @return the httpGatewayConnectionPort value.
*/
public Integer httpGatewayConnectionPort() {
return this.innerProperties() == null ? null : this.innerProperties().httpGatewayConnectionPort();
}
/**
* Set the httpGatewayConnectionPort property: The port used for HTTP connections to the cluster.
*
* @param httpGatewayConnectionPort the httpGatewayConnectionPort value to set.
* @return the ManagedClusterInner object itself.
*/
public ManagedClusterInner withHttpGatewayConnectionPort(Integer httpGatewayConnectionPort) {
if (this.innerProperties() == null) {
this.innerProperties = new ManagedClusterProperties();
}
this.innerProperties().withHttpGatewayConnectionPort(httpGatewayConnectionPort);
return this;
}
/**
* Get the adminUsername property: VM admin user name.
*
* @return the adminUsername value.
*/
public String adminUsername() {
return this.innerProperties() == null ? null : this.innerProperties().adminUsername();
}
/**
* Set the adminUsername property: VM admin user name.
*
* @param adminUsername the adminUsername value to set.
* @return the ManagedClusterInner object itself.
*/
public ManagedClusterInner withAdminUsername(String adminUsername) {
if (this.innerProperties() == null) {
this.innerProperties = new ManagedClusterProperties();
}
this.innerProperties().withAdminUsername(adminUsername);
return this;
}
/**
* Get the adminPassword property: VM admin user password.
*
* @return the adminPassword value.
*/
public String adminPassword() {
return this.innerProperties() == null ? null : this.innerProperties().adminPassword();
}
/**
* Set the adminPassword property: VM admin user password.
*
* @param adminPassword the adminPassword value to set.
* @return the ManagedClusterInner object itself.
*/
public ManagedClusterInner withAdminPassword(String adminPassword) {
if (this.innerProperties() == null) {
this.innerProperties = new ManagedClusterProperties();
}
this.innerProperties().withAdminPassword(adminPassword);
return this;
}
/**
* Get the loadBalancingRules property: Load balancing rules that are applied to the public load balancer of the
* cluster.
*
* @return the loadBalancingRules value.
*/
public List loadBalancingRules() {
return this.innerProperties() == null ? null : this.innerProperties().loadBalancingRules();
}
/**
* Set the loadBalancingRules property: Load balancing rules that are applied to the public load balancer of the
* cluster.
*
* @param loadBalancingRules the loadBalancingRules value to set.
* @return the ManagedClusterInner object itself.
*/
public ManagedClusterInner withLoadBalancingRules(List loadBalancingRules) {
if (this.innerProperties() == null) {
this.innerProperties = new ManagedClusterProperties();
}
this.innerProperties().withLoadBalancingRules(loadBalancingRules);
return this;
}
/**
* Get the allowRdpAccess property: Setting this to true enables RDP access to the VM. The default NSG rule opens
* RDP port to Internet which can be overridden with custom Network Security Rules. The default value for this
* setting is false.
*
* @return the allowRdpAccess value.
*/
public Boolean allowRdpAccess() {
return this.innerProperties() == null ? null : this.innerProperties().allowRdpAccess();
}
/**
* Set the allowRdpAccess property: Setting this to true enables RDP access to the VM. The default NSG rule opens
* RDP port to Internet which can be overridden with custom Network Security Rules. The default value for this
* setting is false.
*
* @param allowRdpAccess the allowRdpAccess value to set.
* @return the ManagedClusterInner object itself.
*/
public ManagedClusterInner withAllowRdpAccess(Boolean allowRdpAccess) {
if (this.innerProperties() == null) {
this.innerProperties = new ManagedClusterProperties();
}
this.innerProperties().withAllowRdpAccess(allowRdpAccess);
return this;
}
/**
* Get the networkSecurityRules property: Custom Network Security Rules that are applied to the Virtual Network of
* the cluster.
*
* @return the networkSecurityRules value.
*/
public List networkSecurityRules() {
return this.innerProperties() == null ? null : this.innerProperties().networkSecurityRules();
}
/**
* Set the networkSecurityRules property: Custom Network Security Rules that are applied to the Virtual Network of
* the cluster.
*
* @param networkSecurityRules the networkSecurityRules value to set.
* @return the ManagedClusterInner object itself.
*/
public ManagedClusterInner withNetworkSecurityRules(List networkSecurityRules) {
if (this.innerProperties() == null) {
this.innerProperties = new ManagedClusterProperties();
}
this.innerProperties().withNetworkSecurityRules(networkSecurityRules);
return this;
}
/**
* Get the clients property: Client certificates that are allowed to manage the cluster.
*
* @return the clients value.
*/
public List clients() {
return this.innerProperties() == null ? null : this.innerProperties().clients();
}
/**
* Set the clients property: Client certificates that are allowed to manage the cluster.
*
* @param clients the clients value to set.
* @return the ManagedClusterInner object itself.
*/
public ManagedClusterInner withClients(List clients) {
if (this.innerProperties() == null) {
this.innerProperties = new ManagedClusterProperties();
}
this.innerProperties().withClients(clients);
return this;
}
/**
* Get the azureActiveDirectory property: The AAD authentication settings of the cluster.
*
* @return the azureActiveDirectory value.
*/
public AzureActiveDirectory azureActiveDirectory() {
return this.innerProperties() == null ? null : this.innerProperties().azureActiveDirectory();
}
/**
* Set the azureActiveDirectory property: The AAD authentication settings of the cluster.
*
* @param azureActiveDirectory the azureActiveDirectory value to set.
* @return the ManagedClusterInner object itself.
*/
public ManagedClusterInner withAzureActiveDirectory(AzureActiveDirectory azureActiveDirectory) {
if (this.innerProperties() == null) {
this.innerProperties = new ManagedClusterProperties();
}
this.innerProperties().withAzureActiveDirectory(azureActiveDirectory);
return this;
}
/**
* Get the fabricSettings property: The list of custom fabric settings to configure the cluster.
*
* @return the fabricSettings value.
*/
public List fabricSettings() {
return this.innerProperties() == null ? null : this.innerProperties().fabricSettings();
}
/**
* Set the fabricSettings property: The list of custom fabric settings to configure the cluster.
*
* @param fabricSettings the fabricSettings value to set.
* @return the ManagedClusterInner object itself.
*/
public ManagedClusterInner withFabricSettings(List fabricSettings) {
if (this.innerProperties() == null) {
this.innerProperties = new ManagedClusterProperties();
}
this.innerProperties().withFabricSettings(fabricSettings);
return this;
}
/**
* Get the provisioningState property: The provisioning state of the managed cluster resource.
*
* @return the provisioningState value.
*/
public ManagedResourceProvisioningState provisioningState() {
return this.innerProperties() == null ? null : this.innerProperties().provisioningState();
}
/**
* Get the clusterCodeVersion property: The Service Fabric runtime version of the cluster. This property is required
* when **clusterUpgradeMode** is set to 'Manual'. To get list of available Service Fabric versions for new clusters
* use [ClusterVersion API](./ClusterVersion.md). To get the list of available version for existing clusters use
* **availableClusterVersions**.
*
* @return the clusterCodeVersion value.
*/
public String clusterCodeVersion() {
return this.innerProperties() == null ? null : this.innerProperties().clusterCodeVersion();
}
/**
* Set the clusterCodeVersion property: The Service Fabric runtime version of the cluster. This property is required
* when **clusterUpgradeMode** is set to 'Manual'. To get list of available Service Fabric versions for new clusters
* use [ClusterVersion API](./ClusterVersion.md). To get the list of available version for existing clusters use
* **availableClusterVersions**.
*
* @param clusterCodeVersion the clusterCodeVersion value to set.
* @return the ManagedClusterInner object itself.
*/
public ManagedClusterInner withClusterCodeVersion(String clusterCodeVersion) {
if (this.innerProperties() == null) {
this.innerProperties = new ManagedClusterProperties();
}
this.innerProperties().withClusterCodeVersion(clusterCodeVersion);
return this;
}
/**
* Get the clusterUpgradeMode property: The upgrade mode of the cluster when new Service Fabric runtime version is
* available.
*
* @return the clusterUpgradeMode value.
*/
public ClusterUpgradeMode clusterUpgradeMode() {
return this.innerProperties() == null ? null : this.innerProperties().clusterUpgradeMode();
}
/**
* Set the clusterUpgradeMode property: The upgrade mode of the cluster when new Service Fabric runtime version is
* available.
*
* @param clusterUpgradeMode the clusterUpgradeMode value to set.
* @return the ManagedClusterInner object itself.
*/
public ManagedClusterInner withClusterUpgradeMode(ClusterUpgradeMode clusterUpgradeMode) {
if (this.innerProperties() == null) {
this.innerProperties = new ManagedClusterProperties();
}
this.innerProperties().withClusterUpgradeMode(clusterUpgradeMode);
return this;
}
/**
* Get the clusterUpgradeCadence property: Indicates when new cluster runtime version upgrades will be applied after
* they are released. By default is Wave0. Only applies when **clusterUpgradeMode** is set to 'Automatic'.
*
* @return the clusterUpgradeCadence value.
*/
public ClusterUpgradeCadence clusterUpgradeCadence() {
return this.innerProperties() == null ? null : this.innerProperties().clusterUpgradeCadence();
}
/**
* Set the clusterUpgradeCadence property: Indicates when new cluster runtime version upgrades will be applied after
* they are released. By default is Wave0. Only applies when **clusterUpgradeMode** is set to 'Automatic'.
*
* @param clusterUpgradeCadence the clusterUpgradeCadence value to set.
* @return the ManagedClusterInner object itself.
*/
public ManagedClusterInner withClusterUpgradeCadence(ClusterUpgradeCadence clusterUpgradeCadence) {
if (this.innerProperties() == null) {
this.innerProperties = new ManagedClusterProperties();
}
this.innerProperties().withClusterUpgradeCadence(clusterUpgradeCadence);
return this;
}
/**
* Get the addonFeatures property: List of add-on features to enable on the cluster.
*
* @return the addonFeatures value.
*/
public List addonFeatures() {
return this.innerProperties() == null ? null : this.innerProperties().addonFeatures();
}
/**
* Set the addonFeatures property: List of add-on features to enable on the cluster.
*
* @param addonFeatures the addonFeatures value to set.
* @return the ManagedClusterInner object itself.
*/
public ManagedClusterInner withAddonFeatures(List addonFeatures) {
if (this.innerProperties() == null) {
this.innerProperties = new ManagedClusterProperties();
}
this.innerProperties().withAddonFeatures(addonFeatures);
return this;
}
/**
* Get the enableAutoOSUpgrade property: Setting this to true enables automatic OS upgrade for the node types that
* are created using any platform OS image with version 'latest'. The default value for this setting is false.
*
* @return the enableAutoOSUpgrade value.
*/
public Boolean enableAutoOSUpgrade() {
return this.innerProperties() == null ? null : this.innerProperties().enableAutoOSUpgrade();
}
/**
* Set the enableAutoOSUpgrade property: Setting this to true enables automatic OS upgrade for the node types that
* are created using any platform OS image with version 'latest'. The default value for this setting is false.
*
* @param enableAutoOSUpgrade the enableAutoOSUpgrade value to set.
* @return the ManagedClusterInner object itself.
*/
public ManagedClusterInner withEnableAutoOSUpgrade(Boolean enableAutoOSUpgrade) {
if (this.innerProperties() == null) {
this.innerProperties = new ManagedClusterProperties();
}
this.innerProperties().withEnableAutoOSUpgrade(enableAutoOSUpgrade);
return this;
}
/**
* Get the zonalResiliency property: Indicates if the cluster has zone resiliency.
*
* @return the zonalResiliency value.
*/
public Boolean zonalResiliency() {
return this.innerProperties() == null ? null : this.innerProperties().zonalResiliency();
}
/**
* Set the zonalResiliency property: Indicates if the cluster has zone resiliency.
*
* @param zonalResiliency the zonalResiliency value to set.
* @return the ManagedClusterInner object itself.
*/
public ManagedClusterInner withZonalResiliency(Boolean zonalResiliency) {
if (this.innerProperties() == null) {
this.innerProperties = new ManagedClusterProperties();
}
this.innerProperties().withZonalResiliency(zonalResiliency);
return this;
}
/**
* Get the applicationTypeVersionsCleanupPolicy property: The policy used to clean up unused versions.
*
* @return the applicationTypeVersionsCleanupPolicy value.
*/
public ApplicationTypeVersionsCleanupPolicy applicationTypeVersionsCleanupPolicy() {
return this.innerProperties() == null ? null : this.innerProperties().applicationTypeVersionsCleanupPolicy();
}
/**
* Set the applicationTypeVersionsCleanupPolicy property: The policy used to clean up unused versions.
*
* @param applicationTypeVersionsCleanupPolicy the applicationTypeVersionsCleanupPolicy value to set.
* @return the ManagedClusterInner object itself.
*/
public ManagedClusterInner withApplicationTypeVersionsCleanupPolicy(
ApplicationTypeVersionsCleanupPolicy applicationTypeVersionsCleanupPolicy) {
if (this.innerProperties() == null) {
this.innerProperties = new ManagedClusterProperties();
}
this.innerProperties().withApplicationTypeVersionsCleanupPolicy(applicationTypeVersionsCleanupPolicy);
return this;
}
/**
* Get the enableIpv6 property: Setting this to true creates IPv6 address space for the default VNet used by the
* cluster. This setting cannot be changed once the cluster is created. The default value for this setting is false.
*
* @return the enableIpv6 value.
*/
public Boolean enableIpv6() {
return this.innerProperties() == null ? null : this.innerProperties().enableIpv6();
}
/**
* Set the enableIpv6 property: Setting this to true creates IPv6 address space for the default VNet used by the
* cluster. This setting cannot be changed once the cluster is created. The default value for this setting is false.
*
* @param enableIpv6 the enableIpv6 value to set.
* @return the ManagedClusterInner object itself.
*/
public ManagedClusterInner withEnableIpv6(Boolean enableIpv6) {
if (this.innerProperties() == null) {
this.innerProperties = new ManagedClusterProperties();
}
this.innerProperties().withEnableIpv6(enableIpv6);
return this;
}
/**
* Get the subnetId property: If specified, the node types for the cluster are created in this subnet instead of the
* default VNet. The **networkSecurityRules** specified for the cluster are also applied to this subnet. This
* setting cannot be changed once the cluster is created.
*
* @return the subnetId value.
*/
public String subnetId() {
return this.innerProperties() == null ? null : this.innerProperties().subnetId();
}
/**
* Set the subnetId property: If specified, the node types for the cluster are created in this subnet instead of the
* default VNet. The **networkSecurityRules** specified for the cluster are also applied to this subnet. This
* setting cannot be changed once the cluster is created.
*
* @param subnetId the subnetId value to set.
* @return the ManagedClusterInner object itself.
*/
public ManagedClusterInner withSubnetId(String subnetId) {
if (this.innerProperties() == null) {
this.innerProperties = new ManagedClusterProperties();
}
this.innerProperties().withSubnetId(subnetId);
return this;
}
/**
* Get the ipTags property: The list of IP tags associated with the default public IP address of the cluster.
*
* @return the ipTags value.
*/
public List ipTags() {
return this.innerProperties() == null ? null : this.innerProperties().ipTags();
}
/**
* Set the ipTags property: The list of IP tags associated with the default public IP address of the cluster.
*
* @param ipTags the ipTags value to set.
* @return the ManagedClusterInner object itself.
*/
public ManagedClusterInner withIpTags(List ipTags) {
if (this.innerProperties() == null) {
this.innerProperties = new ManagedClusterProperties();
}
this.innerProperties().withIpTags(ipTags);
return this;
}
/**
* Get the ipv6Address property: IPv6 address for the cluster if IPv6 is enabled.
*
* @return the ipv6Address value.
*/
public String ipv6Address() {
return this.innerProperties() == null ? null : this.innerProperties().ipv6Address();
}
/**
* Get the enableServicePublicIp property: Setting this to true will link the IPv4 address as the ServicePublicIP of
* the IPv6 address. It can only be set to True if IPv6 is enabled on the cluster.
*
* @return the enableServicePublicIp value.
*/
public Boolean enableServicePublicIp() {
return this.innerProperties() == null ? null : this.innerProperties().enableServicePublicIp();
}
/**
* Set the enableServicePublicIp property: Setting this to true will link the IPv4 address as the ServicePublicIP of
* the IPv6 address. It can only be set to True if IPv6 is enabled on the cluster.
*
* @param enableServicePublicIp the enableServicePublicIp value to set.
* @return the ManagedClusterInner object itself.
*/
public ManagedClusterInner withEnableServicePublicIp(Boolean enableServicePublicIp) {
if (this.innerProperties() == null) {
this.innerProperties = new ManagedClusterProperties();
}
this.innerProperties().withEnableServicePublicIp(enableServicePublicIp);
return this;
}
/**
* Get the auxiliarySubnets property: Auxiliary subnets for the cluster.
*
* @return the auxiliarySubnets value.
*/
public List auxiliarySubnets() {
return this.innerProperties() == null ? null : this.innerProperties().auxiliarySubnets();
}
/**
* Set the auxiliarySubnets property: Auxiliary subnets for the cluster.
*
* @param auxiliarySubnets the auxiliarySubnets value to set.
* @return the ManagedClusterInner object itself.
*/
public ManagedClusterInner withAuxiliarySubnets(List auxiliarySubnets) {
if (this.innerProperties() == null) {
this.innerProperties = new ManagedClusterProperties();
}
this.innerProperties().withAuxiliarySubnets(auxiliarySubnets);
return this;
}
/**
* Get the serviceEndpoints property: Service endpoints for subnets in the cluster.
*
* @return the serviceEndpoints value.
*/
public List serviceEndpoints() {
return this.innerProperties() == null ? null : this.innerProperties().serviceEndpoints();
}
/**
* Set the serviceEndpoints property: Service endpoints for subnets in the cluster.
*
* @param serviceEndpoints the serviceEndpoints value to set.
* @return the ManagedClusterInner object itself.
*/
public ManagedClusterInner withServiceEndpoints(List serviceEndpoints) {
if (this.innerProperties() == null) {
this.innerProperties = new ManagedClusterProperties();
}
this.innerProperties().withServiceEndpoints(serviceEndpoints);
return this;
}
/**
* Get the zonalUpdateMode property: Indicates the update mode for Cross Az clusters.
*
* @return the zonalUpdateMode value.
*/
public ZonalUpdateMode zonalUpdateMode() {
return this.innerProperties() == null ? null : this.innerProperties().zonalUpdateMode();
}
/**
* Set the zonalUpdateMode property: Indicates the update mode for Cross Az clusters.
*
* @param zonalUpdateMode the zonalUpdateMode value to set.
* @return the ManagedClusterInner object itself.
*/
public ManagedClusterInner withZonalUpdateMode(ZonalUpdateMode zonalUpdateMode) {
if (this.innerProperties() == null) {
this.innerProperties = new ManagedClusterProperties();
}
this.innerProperties().withZonalUpdateMode(zonalUpdateMode);
return this;
}
/**
* Get the useCustomVnet property: For new clusters, this parameter indicates that it uses Bring your own VNet, but
* the subnet is specified at node type level; and for such clusters, the subnetId property is required for node
* types.
*
* @return the useCustomVnet value.
*/
public Boolean useCustomVnet() {
return this.innerProperties() == null ? null : this.innerProperties().useCustomVnet();
}
/**
* Set the useCustomVnet property: For new clusters, this parameter indicates that it uses Bring your own VNet, but
* the subnet is specified at node type level; and for such clusters, the subnetId property is required for node
* types.
*
* @param useCustomVnet the useCustomVnet value to set.
* @return the ManagedClusterInner object itself.
*/
public ManagedClusterInner withUseCustomVnet(Boolean useCustomVnet) {
if (this.innerProperties() == null) {
this.innerProperties = new ManagedClusterProperties();
}
this.innerProperties().withUseCustomVnet(useCustomVnet);
return this;
}
/**
* Get the publicIpPrefixId property: Specify the resource id of a public IPv4 prefix that the load balancer will
* allocate a public IPv4 address from. This setting cannot be changed once the cluster is created.
*
* @return the publicIpPrefixId value.
*/
public String publicIpPrefixId() {
return this.innerProperties() == null ? null : this.innerProperties().publicIpPrefixId();
}
/**
* Set the publicIpPrefixId property: Specify the resource id of a public IPv4 prefix that the load balancer will
* allocate a public IPv4 address from. This setting cannot be changed once the cluster is created.
*
* @param publicIpPrefixId the publicIpPrefixId value to set.
* @return the ManagedClusterInner object itself.
*/
public ManagedClusterInner withPublicIpPrefixId(String publicIpPrefixId) {
if (this.innerProperties() == null) {
this.innerProperties = new ManagedClusterProperties();
}
this.innerProperties().withPublicIpPrefixId(publicIpPrefixId);
return this;
}
/**
* Get the publicIPv6PrefixId property: Specify the resource id of a public IPv6 prefix that the load balancer will
* allocate a public IPv6 address from. This setting cannot be changed once the cluster is created.
*
* @return the publicIPv6PrefixId value.
*/
public String publicIPv6PrefixId() {
return this.innerProperties() == null ? null : this.innerProperties().publicIPv6PrefixId();
}
/**
* Set the publicIPv6PrefixId property: Specify the resource id of a public IPv6 prefix that the load balancer will
* allocate a public IPv6 address from. This setting cannot be changed once the cluster is created.
*
* @param publicIPv6PrefixId the publicIPv6PrefixId value to set.
* @return the ManagedClusterInner object itself.
*/
public ManagedClusterInner withPublicIPv6PrefixId(String publicIPv6PrefixId) {
if (this.innerProperties() == null) {
this.innerProperties = new ManagedClusterProperties();
}
this.innerProperties().withPublicIPv6PrefixId(publicIPv6PrefixId);
return this;
}
/**
* Get the ddosProtectionPlanId property: Specify the resource id of a DDoS network protection plan that will be
* associated with the virtual network of the cluster.
*
* @return the ddosProtectionPlanId value.
*/
public String ddosProtectionPlanId() {
return this.innerProperties() == null ? null : this.innerProperties().ddosProtectionPlanId();
}
/**
* Set the ddosProtectionPlanId property: Specify the resource id of a DDoS network protection plan that will be
* associated with the virtual network of the cluster.
*
* @param ddosProtectionPlanId the ddosProtectionPlanId value to set.
* @return the ManagedClusterInner object itself.
*/
public ManagedClusterInner withDdosProtectionPlanId(String ddosProtectionPlanId) {
if (this.innerProperties() == null) {
this.innerProperties = new ManagedClusterProperties();
}
this.innerProperties().withDdosProtectionPlanId(ddosProtectionPlanId);
return this;
}
/**
* Get the upgradeDescription property: The policy to use when upgrading the cluster.
*
* @return the upgradeDescription value.
*/
public ClusterUpgradePolicy upgradeDescription() {
return this.innerProperties() == null ? null : this.innerProperties().upgradeDescription();
}
/**
* Set the upgradeDescription property: The policy to use when upgrading the cluster.
*
* @param upgradeDescription the upgradeDescription value to set.
* @return the ManagedClusterInner object itself.
*/
public ManagedClusterInner withUpgradeDescription(ClusterUpgradePolicy upgradeDescription) {
if (this.innerProperties() == null) {
this.innerProperties = new ManagedClusterProperties();
}
this.innerProperties().withUpgradeDescription(upgradeDescription);
return this;
}
/**
* Get the httpGatewayTokenAuthConnectionPort property: The port used for token-auth based HTTPS connections to the
* cluster. Cannot be set to the same port as HttpGatewayEndpoint.
*
* @return the httpGatewayTokenAuthConnectionPort value.
*/
public Integer httpGatewayTokenAuthConnectionPort() {
return this.innerProperties() == null ? null : this.innerProperties().httpGatewayTokenAuthConnectionPort();
}
/**
* Set the httpGatewayTokenAuthConnectionPort property: The port used for token-auth based HTTPS connections to the
* cluster. Cannot be set to the same port as HttpGatewayEndpoint.
*
* @param httpGatewayTokenAuthConnectionPort the httpGatewayTokenAuthConnectionPort value to set.
* @return the ManagedClusterInner object itself.
*/
public ManagedClusterInner withHttpGatewayTokenAuthConnectionPort(Integer httpGatewayTokenAuthConnectionPort) {
if (this.innerProperties() == null) {
this.innerProperties = new ManagedClusterProperties();
}
this.innerProperties().withHttpGatewayTokenAuthConnectionPort(httpGatewayTokenAuthConnectionPort);
return this;
}
/**
* Get the enableHttpGatewayExclusiveAuthMode property: If true, token-based authentication is not allowed on the
* HttpGatewayEndpoint. This is required to support TLS versions 1.3 and above. If token-based authentication is
* used, HttpGatewayTokenAuthConnectionPort must be defined.
*
* @return the enableHttpGatewayExclusiveAuthMode value.
*/
public Boolean enableHttpGatewayExclusiveAuthMode() {
return this.innerProperties() == null ? null : this.innerProperties().enableHttpGatewayExclusiveAuthMode();
}
/**
* Set the enableHttpGatewayExclusiveAuthMode property: If true, token-based authentication is not allowed on the
* HttpGatewayEndpoint. This is required to support TLS versions 1.3 and above. If token-based authentication is
* used, HttpGatewayTokenAuthConnectionPort must be defined.
*
* @param enableHttpGatewayExclusiveAuthMode the enableHttpGatewayExclusiveAuthMode value to set.
* @return the ManagedClusterInner object itself.
*/
public ManagedClusterInner withEnableHttpGatewayExclusiveAuthMode(Boolean enableHttpGatewayExclusiveAuthMode) {
if (this.innerProperties() == null) {
this.innerProperties = new ManagedClusterProperties();
}
this.innerProperties().withEnableHttpGatewayExclusiveAuthMode(enableHttpGatewayExclusiveAuthMode);
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (innerProperties() != null) {
innerProperties().validate();
}
if (sku() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException("Missing required property sku in model ManagedClusterInner"));
} else {
sku().validate();
}
}
private static final ClientLogger LOGGER = new ClientLogger(ManagedClusterInner.class);
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("location", location());
jsonWriter.writeMapField("tags", tags(), (writer, element) -> writer.writeString(element));
jsonWriter.writeJsonField("sku", this.sku);
jsonWriter.writeJsonField("properties", this.innerProperties);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of ManagedClusterInner from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of ManagedClusterInner if the JsonReader was pointing to an instance of it, or null if it was
* pointing to JSON null.
* @throws IllegalStateException If the deserialized JSON object was missing any required properties.
* @throws IOException If an error occurs while reading the ManagedClusterInner.
*/
public static ManagedClusterInner fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
ManagedClusterInner deserializedManagedClusterInner = new ManagedClusterInner();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("id".equals(fieldName)) {
deserializedManagedClusterInner.id = reader.getString();
} else if ("name".equals(fieldName)) {
deserializedManagedClusterInner.name = reader.getString();
} else if ("type".equals(fieldName)) {
deserializedManagedClusterInner.type = reader.getString();
} else if ("location".equals(fieldName)) {
deserializedManagedClusterInner.withLocation(reader.getString());
} else if ("tags".equals(fieldName)) {
Map tags = reader.readMap(reader1 -> reader1.getString());
deserializedManagedClusterInner.withTags(tags);
} else if ("sku".equals(fieldName)) {
deserializedManagedClusterInner.sku = Sku.fromJson(reader);
} else if ("properties".equals(fieldName)) {
deserializedManagedClusterInner.innerProperties = ManagedClusterProperties.fromJson(reader);
} else if ("etag".equals(fieldName)) {
deserializedManagedClusterInner.etag = reader.getString();
} else if ("systemData".equals(fieldName)) {
deserializedManagedClusterInner.systemData = SystemData.fromJson(reader);
} else {
reader.skipChildren();
}
}
return deserializedManagedClusterInner;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy