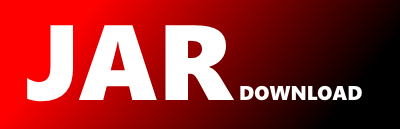
com.azure.resourcemanager.servicefabricmanagedclusters.implementation.ManagedClusterImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-servicefabricmanagedclusters Show documentation
Show all versions of azure-resourcemanager-servicefabricmanagedclusters Show documentation
This package contains Microsoft Azure SDK for Service Fabric Managed Clusters Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Service Fabric Managed Clusters Management Client. Package tag package-2024-04.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.servicefabricmanagedclusters.implementation;
import com.azure.core.management.Region;
import com.azure.core.management.SystemData;
import com.azure.core.util.Context;
import com.azure.resourcemanager.servicefabricmanagedclusters.fluent.models.ManagedClusterInner;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.ApplicationTypeVersionsCleanupPolicy;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.AzureActiveDirectory;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.ClientCertificate;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.ClusterState;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.ClusterUpgradeCadence;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.ClusterUpgradeMode;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.ClusterUpgradePolicy;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.IpTag;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.LoadBalancingRule;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.ManagedCluster;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.ManagedClusterAddOnFeature;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.ManagedClusterUpdateParameters;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.ManagedResourceProvisioningState;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.NetworkSecurityRule;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.ServiceEndpoint;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.SettingsSectionDescription;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.Sku;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.Subnet;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.ZonalUpdateMode;
import java.util.Collections;
import java.util.List;
import java.util.Map;
public final class ManagedClusterImpl implements ManagedCluster, ManagedCluster.Definition, ManagedCluster.Update {
private ManagedClusterInner innerObject;
private final com.azure.resourcemanager.servicefabricmanagedclusters.ServiceFabricManagedClustersManager serviceManager;
public String id() {
return this.innerModel().id();
}
public String name() {
return this.innerModel().name();
}
public String type() {
return this.innerModel().type();
}
public String location() {
return this.innerModel().location();
}
public Map tags() {
Map inner = this.innerModel().tags();
if (inner != null) {
return Collections.unmodifiableMap(inner);
} else {
return Collections.emptyMap();
}
}
public Sku sku() {
return this.innerModel().sku();
}
public String etag() {
return this.innerModel().etag();
}
public SystemData systemData() {
return this.innerModel().systemData();
}
public String dnsName() {
return this.innerModel().dnsName();
}
public String fqdn() {
return this.innerModel().fqdn();
}
public String ipv4Address() {
return this.innerModel().ipv4Address();
}
public String clusterId() {
return this.innerModel().clusterId();
}
public ClusterState clusterState() {
return this.innerModel().clusterState();
}
public List clusterCertificateThumbprints() {
List inner = this.innerModel().clusterCertificateThumbprints();
if (inner != null) {
return Collections.unmodifiableList(inner);
} else {
return Collections.emptyList();
}
}
public Integer clientConnectionPort() {
return this.innerModel().clientConnectionPort();
}
public Integer httpGatewayConnectionPort() {
return this.innerModel().httpGatewayConnectionPort();
}
public String adminUsername() {
return this.innerModel().adminUsername();
}
public String adminPassword() {
return this.innerModel().adminPassword();
}
public List loadBalancingRules() {
List inner = this.innerModel().loadBalancingRules();
if (inner != null) {
return Collections.unmodifiableList(inner);
} else {
return Collections.emptyList();
}
}
public Boolean allowRdpAccess() {
return this.innerModel().allowRdpAccess();
}
public List networkSecurityRules() {
List inner = this.innerModel().networkSecurityRules();
if (inner != null) {
return Collections.unmodifiableList(inner);
} else {
return Collections.emptyList();
}
}
public List clients() {
List inner = this.innerModel().clients();
if (inner != null) {
return Collections.unmodifiableList(inner);
} else {
return Collections.emptyList();
}
}
public AzureActiveDirectory azureActiveDirectory() {
return this.innerModel().azureActiveDirectory();
}
public List fabricSettings() {
List inner = this.innerModel().fabricSettings();
if (inner != null) {
return Collections.unmodifiableList(inner);
} else {
return Collections.emptyList();
}
}
public ManagedResourceProvisioningState provisioningState() {
return this.innerModel().provisioningState();
}
public String clusterCodeVersion() {
return this.innerModel().clusterCodeVersion();
}
public ClusterUpgradeMode clusterUpgradeMode() {
return this.innerModel().clusterUpgradeMode();
}
public ClusterUpgradeCadence clusterUpgradeCadence() {
return this.innerModel().clusterUpgradeCadence();
}
public List addonFeatures() {
List inner = this.innerModel().addonFeatures();
if (inner != null) {
return Collections.unmodifiableList(inner);
} else {
return Collections.emptyList();
}
}
public Boolean enableAutoOSUpgrade() {
return this.innerModel().enableAutoOSUpgrade();
}
public Boolean zonalResiliency() {
return this.innerModel().zonalResiliency();
}
public ApplicationTypeVersionsCleanupPolicy applicationTypeVersionsCleanupPolicy() {
return this.innerModel().applicationTypeVersionsCleanupPolicy();
}
public Boolean enableIpv6() {
return this.innerModel().enableIpv6();
}
public String subnetId() {
return this.innerModel().subnetId();
}
public List ipTags() {
List inner = this.innerModel().ipTags();
if (inner != null) {
return Collections.unmodifiableList(inner);
} else {
return Collections.emptyList();
}
}
public String ipv6Address() {
return this.innerModel().ipv6Address();
}
public Boolean enableServicePublicIp() {
return this.innerModel().enableServicePublicIp();
}
public List auxiliarySubnets() {
List inner = this.innerModel().auxiliarySubnets();
if (inner != null) {
return Collections.unmodifiableList(inner);
} else {
return Collections.emptyList();
}
}
public List serviceEndpoints() {
List inner = this.innerModel().serviceEndpoints();
if (inner != null) {
return Collections.unmodifiableList(inner);
} else {
return Collections.emptyList();
}
}
public ZonalUpdateMode zonalUpdateMode() {
return this.innerModel().zonalUpdateMode();
}
public Boolean useCustomVnet() {
return this.innerModel().useCustomVnet();
}
public String publicIpPrefixId() {
return this.innerModel().publicIpPrefixId();
}
public String publicIPv6PrefixId() {
return this.innerModel().publicIPv6PrefixId();
}
public String ddosProtectionPlanId() {
return this.innerModel().ddosProtectionPlanId();
}
public ClusterUpgradePolicy upgradeDescription() {
return this.innerModel().upgradeDescription();
}
public Integer httpGatewayTokenAuthConnectionPort() {
return this.innerModel().httpGatewayTokenAuthConnectionPort();
}
public Boolean enableHttpGatewayExclusiveAuthMode() {
return this.innerModel().enableHttpGatewayExclusiveAuthMode();
}
public Region region() {
return Region.fromName(this.regionName());
}
public String regionName() {
return this.location();
}
public String resourceGroupName() {
return resourceGroupName;
}
public ManagedClusterInner innerModel() {
return this.innerObject;
}
private com.azure.resourcemanager.servicefabricmanagedclusters.ServiceFabricManagedClustersManager manager() {
return this.serviceManager;
}
private String resourceGroupName;
private String clusterName;
private ManagedClusterUpdateParameters updateParameters;
public ManagedClusterImpl withExistingResourceGroup(String resourceGroupName) {
this.resourceGroupName = resourceGroupName;
return this;
}
public ManagedCluster create() {
this.innerObject = serviceManager.serviceClient()
.getManagedClusters()
.createOrUpdate(resourceGroupName, clusterName, this.innerModel(), Context.NONE);
return this;
}
public ManagedCluster create(Context context) {
this.innerObject = serviceManager.serviceClient()
.getManagedClusters()
.createOrUpdate(resourceGroupName, clusterName, this.innerModel(), context);
return this;
}
ManagedClusterImpl(String name,
com.azure.resourcemanager.servicefabricmanagedclusters.ServiceFabricManagedClustersManager serviceManager) {
this.innerObject = new ManagedClusterInner();
this.serviceManager = serviceManager;
this.clusterName = name;
}
public ManagedClusterImpl update() {
this.updateParameters = new ManagedClusterUpdateParameters();
return this;
}
public ManagedCluster apply() {
this.innerObject = serviceManager.serviceClient()
.getManagedClusters()
.updateWithResponse(resourceGroupName, clusterName, updateParameters, Context.NONE)
.getValue();
return this;
}
public ManagedCluster apply(Context context) {
this.innerObject = serviceManager.serviceClient()
.getManagedClusters()
.updateWithResponse(resourceGroupName, clusterName, updateParameters, context)
.getValue();
return this;
}
ManagedClusterImpl(ManagedClusterInner innerObject,
com.azure.resourcemanager.servicefabricmanagedclusters.ServiceFabricManagedClustersManager serviceManager) {
this.innerObject = innerObject;
this.serviceManager = serviceManager;
this.resourceGroupName = ResourceManagerUtils.getValueFromIdByName(innerObject.id(), "resourceGroups");
this.clusterName = ResourceManagerUtils.getValueFromIdByName(innerObject.id(), "managedClusters");
}
public ManagedCluster refresh() {
this.innerObject = serviceManager.serviceClient()
.getManagedClusters()
.getByResourceGroupWithResponse(resourceGroupName, clusterName, Context.NONE)
.getValue();
return this;
}
public ManagedCluster refresh(Context context) {
this.innerObject = serviceManager.serviceClient()
.getManagedClusters()
.getByResourceGroupWithResponse(resourceGroupName, clusterName, context)
.getValue();
return this;
}
public ManagedClusterImpl withRegion(Region location) {
this.innerModel().withLocation(location.toString());
return this;
}
public ManagedClusterImpl withRegion(String location) {
this.innerModel().withLocation(location);
return this;
}
public ManagedClusterImpl withSku(Sku sku) {
this.innerModel().withSku(sku);
return this;
}
public ManagedClusterImpl withTags(Map tags) {
if (isInCreateMode()) {
this.innerModel().withTags(tags);
return this;
} else {
this.updateParameters.withTags(tags);
return this;
}
}
public ManagedClusterImpl withDnsName(String dnsName) {
this.innerModel().withDnsName(dnsName);
return this;
}
public ManagedClusterImpl withClientConnectionPort(Integer clientConnectionPort) {
this.innerModel().withClientConnectionPort(clientConnectionPort);
return this;
}
public ManagedClusterImpl withHttpGatewayConnectionPort(Integer httpGatewayConnectionPort) {
this.innerModel().withHttpGatewayConnectionPort(httpGatewayConnectionPort);
return this;
}
public ManagedClusterImpl withAdminUsername(String adminUsername) {
this.innerModel().withAdminUsername(adminUsername);
return this;
}
public ManagedClusterImpl withAdminPassword(String adminPassword) {
this.innerModel().withAdminPassword(adminPassword);
return this;
}
public ManagedClusterImpl withLoadBalancingRules(List loadBalancingRules) {
this.innerModel().withLoadBalancingRules(loadBalancingRules);
return this;
}
public ManagedClusterImpl withAllowRdpAccess(Boolean allowRdpAccess) {
this.innerModel().withAllowRdpAccess(allowRdpAccess);
return this;
}
public ManagedClusterImpl withNetworkSecurityRules(List networkSecurityRules) {
this.innerModel().withNetworkSecurityRules(networkSecurityRules);
return this;
}
public ManagedClusterImpl withClients(List clients) {
this.innerModel().withClients(clients);
return this;
}
public ManagedClusterImpl withAzureActiveDirectory(AzureActiveDirectory azureActiveDirectory) {
this.innerModel().withAzureActiveDirectory(azureActiveDirectory);
return this;
}
public ManagedClusterImpl withFabricSettings(List fabricSettings) {
this.innerModel().withFabricSettings(fabricSettings);
return this;
}
public ManagedClusterImpl withClusterCodeVersion(String clusterCodeVersion) {
this.innerModel().withClusterCodeVersion(clusterCodeVersion);
return this;
}
public ManagedClusterImpl withClusterUpgradeMode(ClusterUpgradeMode clusterUpgradeMode) {
this.innerModel().withClusterUpgradeMode(clusterUpgradeMode);
return this;
}
public ManagedClusterImpl withClusterUpgradeCadence(ClusterUpgradeCadence clusterUpgradeCadence) {
this.innerModel().withClusterUpgradeCadence(clusterUpgradeCadence);
return this;
}
public ManagedClusterImpl withAddonFeatures(List addonFeatures) {
this.innerModel().withAddonFeatures(addonFeatures);
return this;
}
public ManagedClusterImpl withEnableAutoOSUpgrade(Boolean enableAutoOSUpgrade) {
this.innerModel().withEnableAutoOSUpgrade(enableAutoOSUpgrade);
return this;
}
public ManagedClusterImpl withZonalResiliency(Boolean zonalResiliency) {
this.innerModel().withZonalResiliency(zonalResiliency);
return this;
}
public ManagedClusterImpl withApplicationTypeVersionsCleanupPolicy(
ApplicationTypeVersionsCleanupPolicy applicationTypeVersionsCleanupPolicy) {
this.innerModel().withApplicationTypeVersionsCleanupPolicy(applicationTypeVersionsCleanupPolicy);
return this;
}
public ManagedClusterImpl withEnableIpv6(Boolean enableIpv6) {
this.innerModel().withEnableIpv6(enableIpv6);
return this;
}
public ManagedClusterImpl withSubnetId(String subnetId) {
this.innerModel().withSubnetId(subnetId);
return this;
}
public ManagedClusterImpl withIpTags(List ipTags) {
this.innerModel().withIpTags(ipTags);
return this;
}
public ManagedClusterImpl withEnableServicePublicIp(Boolean enableServicePublicIp) {
this.innerModel().withEnableServicePublicIp(enableServicePublicIp);
return this;
}
public ManagedClusterImpl withAuxiliarySubnets(List auxiliarySubnets) {
this.innerModel().withAuxiliarySubnets(auxiliarySubnets);
return this;
}
public ManagedClusterImpl withServiceEndpoints(List serviceEndpoints) {
this.innerModel().withServiceEndpoints(serviceEndpoints);
return this;
}
public ManagedClusterImpl withZonalUpdateMode(ZonalUpdateMode zonalUpdateMode) {
this.innerModel().withZonalUpdateMode(zonalUpdateMode);
return this;
}
public ManagedClusterImpl withUseCustomVnet(Boolean useCustomVnet) {
this.innerModel().withUseCustomVnet(useCustomVnet);
return this;
}
public ManagedClusterImpl withPublicIpPrefixId(String publicIpPrefixId) {
this.innerModel().withPublicIpPrefixId(publicIpPrefixId);
return this;
}
public ManagedClusterImpl withPublicIPv6PrefixId(String publicIPv6PrefixId) {
this.innerModel().withPublicIPv6PrefixId(publicIPv6PrefixId);
return this;
}
public ManagedClusterImpl withDdosProtectionPlanId(String ddosProtectionPlanId) {
this.innerModel().withDdosProtectionPlanId(ddosProtectionPlanId);
return this;
}
public ManagedClusterImpl withUpgradeDescription(ClusterUpgradePolicy upgradeDescription) {
this.innerModel().withUpgradeDescription(upgradeDescription);
return this;
}
public ManagedClusterImpl withHttpGatewayTokenAuthConnectionPort(Integer httpGatewayTokenAuthConnectionPort) {
this.innerModel().withHttpGatewayTokenAuthConnectionPort(httpGatewayTokenAuthConnectionPort);
return this;
}
public ManagedClusterImpl withEnableHttpGatewayExclusiveAuthMode(Boolean enableHttpGatewayExclusiveAuthMode) {
this.innerModel().withEnableHttpGatewayExclusiveAuthMode(enableHttpGatewayExclusiveAuthMode);
return this;
}
private boolean isInCreateMode() {
return this.innerModel().id() == null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy