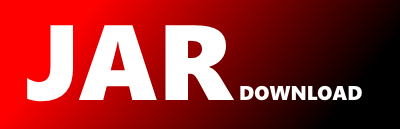
com.azure.resourcemanager.servicefabricmanagedclusters.implementation.ManagedClusterVersionsImpl Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.servicefabricmanagedclusters.implementation;
import com.azure.core.http.rest.Response;
import com.azure.core.http.rest.SimpleResponse;
import com.azure.core.util.Context;
import com.azure.core.util.logging.ClientLogger;
import com.azure.resourcemanager.servicefabricmanagedclusters.fluent.ManagedClusterVersionsClient;
import com.azure.resourcemanager.servicefabricmanagedclusters.fluent.models.ManagedClusterCodeVersionResultInner;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.ManagedClusterCodeVersionResult;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.ManagedClusterVersionEnvironment;
import com.azure.resourcemanager.servicefabricmanagedclusters.models.ManagedClusterVersions;
import java.util.Collections;
import java.util.List;
import java.util.stream.Collectors;
public final class ManagedClusterVersionsImpl implements ManagedClusterVersions {
private static final ClientLogger LOGGER = new ClientLogger(ManagedClusterVersionsImpl.class);
private final ManagedClusterVersionsClient innerClient;
private final com.azure.resourcemanager.servicefabricmanagedclusters.ServiceFabricManagedClustersManager serviceManager;
public ManagedClusterVersionsImpl(ManagedClusterVersionsClient innerClient,
com.azure.resourcemanager.servicefabricmanagedclusters.ServiceFabricManagedClustersManager serviceManager) {
this.innerClient = innerClient;
this.serviceManager = serviceManager;
}
public Response getWithResponse(String location, String clusterVersion,
Context context) {
Response inner
= this.serviceClient().getWithResponse(location, clusterVersion, context);
if (inner != null) {
return new SimpleResponse<>(inner.getRequest(), inner.getStatusCode(), inner.getHeaders(),
new ManagedClusterCodeVersionResultImpl(inner.getValue(), this.manager()));
} else {
return null;
}
}
public ManagedClusterCodeVersionResult get(String location, String clusterVersion) {
ManagedClusterCodeVersionResultInner inner = this.serviceClient().get(location, clusterVersion);
if (inner != null) {
return new ManagedClusterCodeVersionResultImpl(inner, this.manager());
} else {
return null;
}
}
public Response getByEnvironmentWithResponse(String location,
ManagedClusterVersionEnvironment environment, String clusterVersion, Context context) {
Response inner
= this.serviceClient().getByEnvironmentWithResponse(location, environment, clusterVersion, context);
if (inner != null) {
return new SimpleResponse<>(inner.getRequest(), inner.getStatusCode(), inner.getHeaders(),
new ManagedClusterCodeVersionResultImpl(inner.getValue(), this.manager()));
} else {
return null;
}
}
public ManagedClusterCodeVersionResult getByEnvironment(String location,
ManagedClusterVersionEnvironment environment, String clusterVersion) {
ManagedClusterCodeVersionResultInner inner
= this.serviceClient().getByEnvironment(location, environment, clusterVersion);
if (inner != null) {
return new ManagedClusterCodeVersionResultImpl(inner, this.manager());
} else {
return null;
}
}
public Response> listWithResponse(String location, Context context) {
Response> inner
= this.serviceClient().listWithResponse(location, context);
if (inner != null) {
return new SimpleResponse<>(inner.getRequest(), inner.getStatusCode(), inner.getHeaders(),
inner.getValue()
.stream()
.map(inner1 -> new ManagedClusterCodeVersionResultImpl(inner1, this.manager()))
.collect(Collectors.toList()));
} else {
return null;
}
}
public List list(String location) {
List inner = this.serviceClient().list(location);
if (inner != null) {
return Collections.unmodifiableList(inner.stream()
.map(inner1 -> new ManagedClusterCodeVersionResultImpl(inner1, this.manager()))
.collect(Collectors.toList()));
} else {
return Collections.emptyList();
}
}
public Response> listByEnvironmentWithResponse(String location,
ManagedClusterVersionEnvironment environment, Context context) {
Response> inner
= this.serviceClient().listByEnvironmentWithResponse(location, environment, context);
if (inner != null) {
return new SimpleResponse<>(inner.getRequest(), inner.getStatusCode(), inner.getHeaders(),
inner.getValue()
.stream()
.map(inner1 -> new ManagedClusterCodeVersionResultImpl(inner1, this.manager()))
.collect(Collectors.toList()));
} else {
return null;
}
}
public List listByEnvironment(String location,
ManagedClusterVersionEnvironment environment) {
List inner
= this.serviceClient().listByEnvironment(location, environment);
if (inner != null) {
return Collections.unmodifiableList(inner.stream()
.map(inner1 -> new ManagedClusterCodeVersionResultImpl(inner1, this.manager()))
.collect(Collectors.toList()));
} else {
return Collections.emptyList();
}
}
private ManagedClusterVersionsClient serviceClient() {
return this.innerClient;
}
private com.azure.resourcemanager.servicefabricmanagedclusters.ServiceFabricManagedClustersManager manager() {
return this.serviceManager;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy