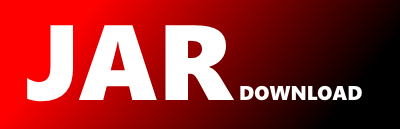
com.azure.resourcemanager.servicefabricmanagedclusters.models.ApplicationHealthPolicy Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.servicefabricmanagedclusters.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.util.Map;
/**
* Defines a health policy used to evaluate the health of an application or one of its children entities.
*/
@Fluent
public final class ApplicationHealthPolicy implements JsonSerializable {
/*
* Indicates whether warnings are treated with the same severity as errors.
*/
private boolean considerWarningAsError;
/*
* The maximum allowed percentage of unhealthy deployed applications. Allowed values are Byte values from zero to
* 100.
* The percentage represents the maximum tolerated percentage of deployed applications that can be unhealthy before
* the application is considered in error.
* This is calculated by dividing the number of unhealthy deployed applications over the number of nodes where the
* application is currently deployed on in the cluster.
* The computation rounds up to tolerate one failure on small numbers of nodes. Default percentage is zero.
*/
private int maxPercentUnhealthyDeployedApplications;
/*
* The health policy used by default to evaluate the health of a service type.
*/
private ServiceTypeHealthPolicy defaultServiceTypeHealthPolicy;
/*
* The map with service type health policy per service type name. The map is empty by default.
*/
private Map serviceTypeHealthPolicyMap;
/**
* Creates an instance of ApplicationHealthPolicy class.
*/
public ApplicationHealthPolicy() {
}
/**
* Get the considerWarningAsError property: Indicates whether warnings are treated with the same severity as errors.
*
* @return the considerWarningAsError value.
*/
public boolean considerWarningAsError() {
return this.considerWarningAsError;
}
/**
* Set the considerWarningAsError property: Indicates whether warnings are treated with the same severity as errors.
*
* @param considerWarningAsError the considerWarningAsError value to set.
* @return the ApplicationHealthPolicy object itself.
*/
public ApplicationHealthPolicy withConsiderWarningAsError(boolean considerWarningAsError) {
this.considerWarningAsError = considerWarningAsError;
return this;
}
/**
* Get the maxPercentUnhealthyDeployedApplications property: The maximum allowed percentage of unhealthy deployed
* applications. Allowed values are Byte values from zero to 100.
* The percentage represents the maximum tolerated percentage of deployed applications that can be unhealthy before
* the application is considered in error.
* This is calculated by dividing the number of unhealthy deployed applications over the number of nodes where the
* application is currently deployed on in the cluster.
* The computation rounds up to tolerate one failure on small numbers of nodes. Default percentage is zero.
*
* @return the maxPercentUnhealthyDeployedApplications value.
*/
public int maxPercentUnhealthyDeployedApplications() {
return this.maxPercentUnhealthyDeployedApplications;
}
/**
* Set the maxPercentUnhealthyDeployedApplications property: The maximum allowed percentage of unhealthy deployed
* applications. Allowed values are Byte values from zero to 100.
* The percentage represents the maximum tolerated percentage of deployed applications that can be unhealthy before
* the application is considered in error.
* This is calculated by dividing the number of unhealthy deployed applications over the number of nodes where the
* application is currently deployed on in the cluster.
* The computation rounds up to tolerate one failure on small numbers of nodes. Default percentage is zero.
*
* @param maxPercentUnhealthyDeployedApplications the maxPercentUnhealthyDeployedApplications value to set.
* @return the ApplicationHealthPolicy object itself.
*/
public ApplicationHealthPolicy
withMaxPercentUnhealthyDeployedApplications(int maxPercentUnhealthyDeployedApplications) {
this.maxPercentUnhealthyDeployedApplications = maxPercentUnhealthyDeployedApplications;
return this;
}
/**
* Get the defaultServiceTypeHealthPolicy property: The health policy used by default to evaluate the health of a
* service type.
*
* @return the defaultServiceTypeHealthPolicy value.
*/
public ServiceTypeHealthPolicy defaultServiceTypeHealthPolicy() {
return this.defaultServiceTypeHealthPolicy;
}
/**
* Set the defaultServiceTypeHealthPolicy property: The health policy used by default to evaluate the health of a
* service type.
*
* @param defaultServiceTypeHealthPolicy the defaultServiceTypeHealthPolicy value to set.
* @return the ApplicationHealthPolicy object itself.
*/
public ApplicationHealthPolicy
withDefaultServiceTypeHealthPolicy(ServiceTypeHealthPolicy defaultServiceTypeHealthPolicy) {
this.defaultServiceTypeHealthPolicy = defaultServiceTypeHealthPolicy;
return this;
}
/**
* Get the serviceTypeHealthPolicyMap property: The map with service type health policy per service type name. The
* map is empty by default.
*
* @return the serviceTypeHealthPolicyMap value.
*/
public Map serviceTypeHealthPolicyMap() {
return this.serviceTypeHealthPolicyMap;
}
/**
* Set the serviceTypeHealthPolicyMap property: The map with service type health policy per service type name. The
* map is empty by default.
*
* @param serviceTypeHealthPolicyMap the serviceTypeHealthPolicyMap value to set.
* @return the ApplicationHealthPolicy object itself.
*/
public ApplicationHealthPolicy
withServiceTypeHealthPolicyMap(Map serviceTypeHealthPolicyMap) {
this.serviceTypeHealthPolicyMap = serviceTypeHealthPolicyMap;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (defaultServiceTypeHealthPolicy() != null) {
defaultServiceTypeHealthPolicy().validate();
}
if (serviceTypeHealthPolicyMap() != null) {
serviceTypeHealthPolicyMap().values().forEach(e -> {
if (e != null) {
e.validate();
}
});
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeBooleanField("considerWarningAsError", this.considerWarningAsError);
jsonWriter.writeIntField("maxPercentUnhealthyDeployedApplications",
this.maxPercentUnhealthyDeployedApplications);
jsonWriter.writeJsonField("defaultServiceTypeHealthPolicy", this.defaultServiceTypeHealthPolicy);
jsonWriter.writeMapField("serviceTypeHealthPolicyMap", this.serviceTypeHealthPolicyMap,
(writer, element) -> writer.writeJson(element));
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of ApplicationHealthPolicy from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of ApplicationHealthPolicy if the JsonReader was pointing to an instance of it, or null if it
* was pointing to JSON null.
* @throws IllegalStateException If the deserialized JSON object was missing any required properties.
* @throws IOException If an error occurs while reading the ApplicationHealthPolicy.
*/
public static ApplicationHealthPolicy fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
ApplicationHealthPolicy deserializedApplicationHealthPolicy = new ApplicationHealthPolicy();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("considerWarningAsError".equals(fieldName)) {
deserializedApplicationHealthPolicy.considerWarningAsError = reader.getBoolean();
} else if ("maxPercentUnhealthyDeployedApplications".equals(fieldName)) {
deserializedApplicationHealthPolicy.maxPercentUnhealthyDeployedApplications = reader.getInt();
} else if ("defaultServiceTypeHealthPolicy".equals(fieldName)) {
deserializedApplicationHealthPolicy.defaultServiceTypeHealthPolicy
= ServiceTypeHealthPolicy.fromJson(reader);
} else if ("serviceTypeHealthPolicyMap".equals(fieldName)) {
Map serviceTypeHealthPolicyMap
= reader.readMap(reader1 -> ServiceTypeHealthPolicy.fromJson(reader1));
deserializedApplicationHealthPolicy.serviceTypeHealthPolicyMap = serviceTypeHealthPolicyMap;
} else {
reader.skipChildren();
}
}
return deserializedApplicationHealthPolicy;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy