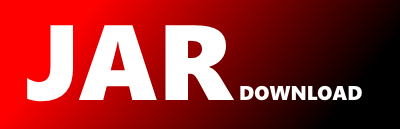
com.azure.resourcemanager.servicefabricmanagedclusters.models.ApplicationUpgradePolicy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-servicefabricmanagedclusters Show documentation
Show all versions of azure-resourcemanager-servicefabricmanagedclusters Show documentation
This package contains Microsoft Azure SDK for Service Fabric Managed Clusters Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Service Fabric Managed Clusters Management Client. Package tag package-2024-04.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.servicefabricmanagedclusters.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
/**
* Describes the policy for a monitored application upgrade.
*/
@Fluent
public final class ApplicationUpgradePolicy implements JsonSerializable {
/*
* Defines a health policy used to evaluate the health of an application or one of its children entities.
*/
private ApplicationHealthPolicy applicationHealthPolicy;
/*
* If true, then processes are forcefully restarted during upgrade even when the code version has not changed (the
* upgrade only changes configuration or data).
*/
private Boolean forceRestart;
/*
* The policy used for monitoring the application upgrade
*/
private RollingUpgradeMonitoringPolicy rollingUpgradeMonitoringPolicy;
/*
* Duration in seconds, to wait before a stateless instance is closed, to allow the active requests to drain
* gracefully. This would be effective when the instance is closing during the application/cluster upgrade, only for
* those instances which have a non-zero delay duration configured in the service description.
*/
private Long instanceCloseDelayDuration;
/*
* The mode used to monitor health during a rolling upgrade. The values are Monitored, and UnmonitoredAuto.
*/
private RollingUpgradeMode upgradeMode;
/*
* The maximum amount of time to block processing of an upgrade domain and prevent loss of availability when there
* are unexpected issues. When this timeout expires, processing of the upgrade domain will proceed regardless of
* availability loss issues. The timeout is reset at the start of each upgrade domain. Valid values are between 0
* and 42949672925 inclusive. (unsigned 32-bit integer).
*/
private Long upgradeReplicaSetCheckTimeout;
/*
* Determines whether the application should be recreated on update. If value=true, the rest of the upgrade policy
* parameters are not allowed.
*/
private Boolean recreateApplication;
/**
* Creates an instance of ApplicationUpgradePolicy class.
*/
public ApplicationUpgradePolicy() {
}
/**
* Get the applicationHealthPolicy property: Defines a health policy used to evaluate the health of an application
* or one of its children entities.
*
* @return the applicationHealthPolicy value.
*/
public ApplicationHealthPolicy applicationHealthPolicy() {
return this.applicationHealthPolicy;
}
/**
* Set the applicationHealthPolicy property: Defines a health policy used to evaluate the health of an application
* or one of its children entities.
*
* @param applicationHealthPolicy the applicationHealthPolicy value to set.
* @return the ApplicationUpgradePolicy object itself.
*/
public ApplicationUpgradePolicy withApplicationHealthPolicy(ApplicationHealthPolicy applicationHealthPolicy) {
this.applicationHealthPolicy = applicationHealthPolicy;
return this;
}
/**
* Get the forceRestart property: If true, then processes are forcefully restarted during upgrade even when the code
* version has not changed (the upgrade only changes configuration or data).
*
* @return the forceRestart value.
*/
public Boolean forceRestart() {
return this.forceRestart;
}
/**
* Set the forceRestart property: If true, then processes are forcefully restarted during upgrade even when the code
* version has not changed (the upgrade only changes configuration or data).
*
* @param forceRestart the forceRestart value to set.
* @return the ApplicationUpgradePolicy object itself.
*/
public ApplicationUpgradePolicy withForceRestart(Boolean forceRestart) {
this.forceRestart = forceRestart;
return this;
}
/**
* Get the rollingUpgradeMonitoringPolicy property: The policy used for monitoring the application upgrade.
*
* @return the rollingUpgradeMonitoringPolicy value.
*/
public RollingUpgradeMonitoringPolicy rollingUpgradeMonitoringPolicy() {
return this.rollingUpgradeMonitoringPolicy;
}
/**
* Set the rollingUpgradeMonitoringPolicy property: The policy used for monitoring the application upgrade.
*
* @param rollingUpgradeMonitoringPolicy the rollingUpgradeMonitoringPolicy value to set.
* @return the ApplicationUpgradePolicy object itself.
*/
public ApplicationUpgradePolicy
withRollingUpgradeMonitoringPolicy(RollingUpgradeMonitoringPolicy rollingUpgradeMonitoringPolicy) {
this.rollingUpgradeMonitoringPolicy = rollingUpgradeMonitoringPolicy;
return this;
}
/**
* Get the instanceCloseDelayDuration property: Duration in seconds, to wait before a stateless instance is closed,
* to allow the active requests to drain gracefully. This would be effective when the instance is closing during the
* application/cluster upgrade, only for those instances which have a non-zero delay duration configured in the
* service description.
*
* @return the instanceCloseDelayDuration value.
*/
public Long instanceCloseDelayDuration() {
return this.instanceCloseDelayDuration;
}
/**
* Set the instanceCloseDelayDuration property: Duration in seconds, to wait before a stateless instance is closed,
* to allow the active requests to drain gracefully. This would be effective when the instance is closing during the
* application/cluster upgrade, only for those instances which have a non-zero delay duration configured in the
* service description.
*
* @param instanceCloseDelayDuration the instanceCloseDelayDuration value to set.
* @return the ApplicationUpgradePolicy object itself.
*/
public ApplicationUpgradePolicy withInstanceCloseDelayDuration(Long instanceCloseDelayDuration) {
this.instanceCloseDelayDuration = instanceCloseDelayDuration;
return this;
}
/**
* Get the upgradeMode property: The mode used to monitor health during a rolling upgrade. The values are Monitored,
* and UnmonitoredAuto.
*
* @return the upgradeMode value.
*/
public RollingUpgradeMode upgradeMode() {
return this.upgradeMode;
}
/**
* Set the upgradeMode property: The mode used to monitor health during a rolling upgrade. The values are Monitored,
* and UnmonitoredAuto.
*
* @param upgradeMode the upgradeMode value to set.
* @return the ApplicationUpgradePolicy object itself.
*/
public ApplicationUpgradePolicy withUpgradeMode(RollingUpgradeMode upgradeMode) {
this.upgradeMode = upgradeMode;
return this;
}
/**
* Get the upgradeReplicaSetCheckTimeout property: The maximum amount of time to block processing of an upgrade
* domain and prevent loss of availability when there are unexpected issues. When this timeout expires, processing
* of the upgrade domain will proceed regardless of availability loss issues. The timeout is reset at the start of
* each upgrade domain. Valid values are between 0 and 42949672925 inclusive. (unsigned 32-bit integer).
*
* @return the upgradeReplicaSetCheckTimeout value.
*/
public Long upgradeReplicaSetCheckTimeout() {
return this.upgradeReplicaSetCheckTimeout;
}
/**
* Set the upgradeReplicaSetCheckTimeout property: The maximum amount of time to block processing of an upgrade
* domain and prevent loss of availability when there are unexpected issues. When this timeout expires, processing
* of the upgrade domain will proceed regardless of availability loss issues. The timeout is reset at the start of
* each upgrade domain. Valid values are between 0 and 42949672925 inclusive. (unsigned 32-bit integer).
*
* @param upgradeReplicaSetCheckTimeout the upgradeReplicaSetCheckTimeout value to set.
* @return the ApplicationUpgradePolicy object itself.
*/
public ApplicationUpgradePolicy withUpgradeReplicaSetCheckTimeout(Long upgradeReplicaSetCheckTimeout) {
this.upgradeReplicaSetCheckTimeout = upgradeReplicaSetCheckTimeout;
return this;
}
/**
* Get the recreateApplication property: Determines whether the application should be recreated on update. If
* value=true, the rest of the upgrade policy parameters are not allowed.
*
* @return the recreateApplication value.
*/
public Boolean recreateApplication() {
return this.recreateApplication;
}
/**
* Set the recreateApplication property: Determines whether the application should be recreated on update. If
* value=true, the rest of the upgrade policy parameters are not allowed.
*
* @param recreateApplication the recreateApplication value to set.
* @return the ApplicationUpgradePolicy object itself.
*/
public ApplicationUpgradePolicy withRecreateApplication(Boolean recreateApplication) {
this.recreateApplication = recreateApplication;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (applicationHealthPolicy() != null) {
applicationHealthPolicy().validate();
}
if (rollingUpgradeMonitoringPolicy() != null) {
rollingUpgradeMonitoringPolicy().validate();
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeJsonField("applicationHealthPolicy", this.applicationHealthPolicy);
jsonWriter.writeBooleanField("forceRestart", this.forceRestart);
jsonWriter.writeJsonField("rollingUpgradeMonitoringPolicy", this.rollingUpgradeMonitoringPolicy);
jsonWriter.writeNumberField("instanceCloseDelayDuration", this.instanceCloseDelayDuration);
jsonWriter.writeStringField("upgradeMode", this.upgradeMode == null ? null : this.upgradeMode.toString());
jsonWriter.writeNumberField("upgradeReplicaSetCheckTimeout", this.upgradeReplicaSetCheckTimeout);
jsonWriter.writeBooleanField("recreateApplication", this.recreateApplication);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of ApplicationUpgradePolicy from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of ApplicationUpgradePolicy if the JsonReader was pointing to an instance of it, or null if
* it was pointing to JSON null.
* @throws IOException If an error occurs while reading the ApplicationUpgradePolicy.
*/
public static ApplicationUpgradePolicy fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
ApplicationUpgradePolicy deserializedApplicationUpgradePolicy = new ApplicationUpgradePolicy();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("applicationHealthPolicy".equals(fieldName)) {
deserializedApplicationUpgradePolicy.applicationHealthPolicy
= ApplicationHealthPolicy.fromJson(reader);
} else if ("forceRestart".equals(fieldName)) {
deserializedApplicationUpgradePolicy.forceRestart = reader.getNullable(JsonReader::getBoolean);
} else if ("rollingUpgradeMonitoringPolicy".equals(fieldName)) {
deserializedApplicationUpgradePolicy.rollingUpgradeMonitoringPolicy
= RollingUpgradeMonitoringPolicy.fromJson(reader);
} else if ("instanceCloseDelayDuration".equals(fieldName)) {
deserializedApplicationUpgradePolicy.instanceCloseDelayDuration
= reader.getNullable(JsonReader::getLong);
} else if ("upgradeMode".equals(fieldName)) {
deserializedApplicationUpgradePolicy.upgradeMode
= RollingUpgradeMode.fromString(reader.getString());
} else if ("upgradeReplicaSetCheckTimeout".equals(fieldName)) {
deserializedApplicationUpgradePolicy.upgradeReplicaSetCheckTimeout
= reader.getNullable(JsonReader::getLong);
} else if ("recreateApplication".equals(fieldName)) {
deserializedApplicationUpgradePolicy.recreateApplication
= reader.getNullable(JsonReader::getBoolean);
} else {
reader.skipChildren();
}
}
return deserializedApplicationUpgradePolicy;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy