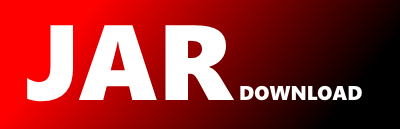
com.azure.resourcemanager.servicefabricmanagedclusters.models.AverageServiceLoadScalingTrigger Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-servicefabricmanagedclusters Show documentation
Show all versions of azure-resourcemanager-servicefabricmanagedclusters Show documentation
This package contains Microsoft Azure SDK for Service Fabric Managed Clusters Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Service Fabric Managed Clusters Management Client. Package tag package-2024-04.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.servicefabricmanagedclusters.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.logging.ClientLogger;
import com.azure.json.JsonReader;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
/**
* Represents a scaling policy related to an average load of a metric/resource of a service.
*/
@Fluent
public final class AverageServiceLoadScalingTrigger extends ScalingTrigger {
/*
* Specifies the trigger associated with this scaling policy.
*/
private ServiceScalingTriggerKind kind = ServiceScalingTriggerKind.AVERAGE_SERVICE_LOAD_TRIGGER;
/*
* The name of the metric for which usage should be tracked.
*/
private String metricName;
/*
* The lower limit of the load below which a scale in operation should be performed.
*/
private double lowerLoadThreshold;
/*
* The upper limit of the load beyond which a scale out operation should be performed.
*/
private double upperLoadThreshold;
/*
* The period in seconds on which a decision is made whether to scale or not. This property should come in ISO 8601
* format "hh:mm:ss".
*/
private String scaleInterval;
/*
* Flag determines whether only the load of primary replica should be considered for scaling. If set to true, then
* trigger will only consider the load of primary replicas of stateful service. If set to false, trigger will
* consider load of all replicas. This parameter cannot be set to true for stateless service.
*/
private boolean useOnlyPrimaryLoad;
/**
* Creates an instance of AverageServiceLoadScalingTrigger class.
*/
public AverageServiceLoadScalingTrigger() {
}
/**
* Get the kind property: Specifies the trigger associated with this scaling policy.
*
* @return the kind value.
*/
@Override
public ServiceScalingTriggerKind kind() {
return this.kind;
}
/**
* Get the metricName property: The name of the metric for which usage should be tracked.
*
* @return the metricName value.
*/
public String metricName() {
return this.metricName;
}
/**
* Set the metricName property: The name of the metric for which usage should be tracked.
*
* @param metricName the metricName value to set.
* @return the AverageServiceLoadScalingTrigger object itself.
*/
public AverageServiceLoadScalingTrigger withMetricName(String metricName) {
this.metricName = metricName;
return this;
}
/**
* Get the lowerLoadThreshold property: The lower limit of the load below which a scale in operation should be
* performed.
*
* @return the lowerLoadThreshold value.
*/
public double lowerLoadThreshold() {
return this.lowerLoadThreshold;
}
/**
* Set the lowerLoadThreshold property: The lower limit of the load below which a scale in operation should be
* performed.
*
* @param lowerLoadThreshold the lowerLoadThreshold value to set.
* @return the AverageServiceLoadScalingTrigger object itself.
*/
public AverageServiceLoadScalingTrigger withLowerLoadThreshold(double lowerLoadThreshold) {
this.lowerLoadThreshold = lowerLoadThreshold;
return this;
}
/**
* Get the upperLoadThreshold property: The upper limit of the load beyond which a scale out operation should be
* performed.
*
* @return the upperLoadThreshold value.
*/
public double upperLoadThreshold() {
return this.upperLoadThreshold;
}
/**
* Set the upperLoadThreshold property: The upper limit of the load beyond which a scale out operation should be
* performed.
*
* @param upperLoadThreshold the upperLoadThreshold value to set.
* @return the AverageServiceLoadScalingTrigger object itself.
*/
public AverageServiceLoadScalingTrigger withUpperLoadThreshold(double upperLoadThreshold) {
this.upperLoadThreshold = upperLoadThreshold;
return this;
}
/**
* Get the scaleInterval property: The period in seconds on which a decision is made whether to scale or not. This
* property should come in ISO 8601 format "hh:mm:ss".
*
* @return the scaleInterval value.
*/
public String scaleInterval() {
return this.scaleInterval;
}
/**
* Set the scaleInterval property: The period in seconds on which a decision is made whether to scale or not. This
* property should come in ISO 8601 format "hh:mm:ss".
*
* @param scaleInterval the scaleInterval value to set.
* @return the AverageServiceLoadScalingTrigger object itself.
*/
public AverageServiceLoadScalingTrigger withScaleInterval(String scaleInterval) {
this.scaleInterval = scaleInterval;
return this;
}
/**
* Get the useOnlyPrimaryLoad property: Flag determines whether only the load of primary replica should be
* considered for scaling. If set to true, then trigger will only consider the load of primary replicas of stateful
* service. If set to false, trigger will consider load of all replicas. This parameter cannot be set to true for
* stateless service.
*
* @return the useOnlyPrimaryLoad value.
*/
public boolean useOnlyPrimaryLoad() {
return this.useOnlyPrimaryLoad;
}
/**
* Set the useOnlyPrimaryLoad property: Flag determines whether only the load of primary replica should be
* considered for scaling. If set to true, then trigger will only consider the load of primary replicas of stateful
* service. If set to false, trigger will consider load of all replicas. This parameter cannot be set to true for
* stateless service.
*
* @param useOnlyPrimaryLoad the useOnlyPrimaryLoad value to set.
* @return the AverageServiceLoadScalingTrigger object itself.
*/
public AverageServiceLoadScalingTrigger withUseOnlyPrimaryLoad(boolean useOnlyPrimaryLoad) {
this.useOnlyPrimaryLoad = useOnlyPrimaryLoad;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
@Override
public void validate() {
super.validate();
if (metricName() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property metricName in model AverageServiceLoadScalingTrigger"));
}
if (scaleInterval() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property scaleInterval in model AverageServiceLoadScalingTrigger"));
}
}
private static final ClientLogger LOGGER = new ClientLogger(AverageServiceLoadScalingTrigger.class);
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("metricName", this.metricName);
jsonWriter.writeDoubleField("lowerLoadThreshold", this.lowerLoadThreshold);
jsonWriter.writeDoubleField("upperLoadThreshold", this.upperLoadThreshold);
jsonWriter.writeStringField("scaleInterval", this.scaleInterval);
jsonWriter.writeBooleanField("useOnlyPrimaryLoad", this.useOnlyPrimaryLoad);
jsonWriter.writeStringField("kind", this.kind == null ? null : this.kind.toString());
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of AverageServiceLoadScalingTrigger from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of AverageServiceLoadScalingTrigger if the JsonReader was pointing to an instance of it, or
* null if it was pointing to JSON null.
* @throws IllegalStateException If the deserialized JSON object was missing any required properties.
* @throws IOException If an error occurs while reading the AverageServiceLoadScalingTrigger.
*/
public static AverageServiceLoadScalingTrigger fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
AverageServiceLoadScalingTrigger deserializedAverageServiceLoadScalingTrigger
= new AverageServiceLoadScalingTrigger();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("metricName".equals(fieldName)) {
deserializedAverageServiceLoadScalingTrigger.metricName = reader.getString();
} else if ("lowerLoadThreshold".equals(fieldName)) {
deserializedAverageServiceLoadScalingTrigger.lowerLoadThreshold = reader.getDouble();
} else if ("upperLoadThreshold".equals(fieldName)) {
deserializedAverageServiceLoadScalingTrigger.upperLoadThreshold = reader.getDouble();
} else if ("scaleInterval".equals(fieldName)) {
deserializedAverageServiceLoadScalingTrigger.scaleInterval = reader.getString();
} else if ("useOnlyPrimaryLoad".equals(fieldName)) {
deserializedAverageServiceLoadScalingTrigger.useOnlyPrimaryLoad = reader.getBoolean();
} else if ("kind".equals(fieldName)) {
deserializedAverageServiceLoadScalingTrigger.kind
= ServiceScalingTriggerKind.fromString(reader.getString());
} else {
reader.skipChildren();
}
}
return deserializedAverageServiceLoadScalingTrigger;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy