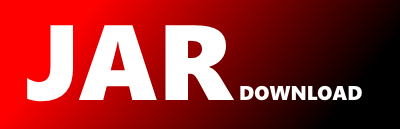
com.azure.resourcemanager.servicefabricmanagedclusters.models.ClusterMonitoringPolicy Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.servicefabricmanagedclusters.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.logging.ClientLogger;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
/**
* Describes the monitoring policies for the cluster upgrade.
*/
@Fluent
public final class ClusterMonitoringPolicy implements JsonSerializable {
/*
* The length of time to wait after completing an upgrade domain before performing health checks. The duration can
* be in either hh:mm:ss or in d.hh:mm:ss.ms format.
*/
private String healthCheckWaitDuration;
/*
* The amount of time that the application or cluster must remain healthy before the upgrade proceeds to the next
* upgrade domain. The duration can be in either hh:mm:ss or in d.hh:mm:ss.ms format.
*/
private String healthCheckStableDuration;
/*
* The amount of time to retry health evaluation when the application or cluster is unhealthy before the upgrade
* rolls back. The timeout can be in either hh:mm:ss or in d.hh:mm:ss.ms format.
*/
private String healthCheckRetryTimeout;
/*
* The amount of time the overall upgrade has to complete before the upgrade rolls back. The timeout can be in
* either hh:mm:ss or in d.hh:mm:ss.ms format.
*/
private String upgradeTimeout;
/*
* The amount of time each upgrade domain has to complete before the upgrade rolls back. The timeout can be in
* either hh:mm:ss or in d.hh:mm:ss.ms format.
*/
private String upgradeDomainTimeout;
/**
* Creates an instance of ClusterMonitoringPolicy class.
*/
public ClusterMonitoringPolicy() {
}
/**
* Get the healthCheckWaitDuration property: The length of time to wait after completing an upgrade domain before
* performing health checks. The duration can be in either hh:mm:ss or in d.hh:mm:ss.ms format.
*
* @return the healthCheckWaitDuration value.
*/
public String healthCheckWaitDuration() {
return this.healthCheckWaitDuration;
}
/**
* Set the healthCheckWaitDuration property: The length of time to wait after completing an upgrade domain before
* performing health checks. The duration can be in either hh:mm:ss or in d.hh:mm:ss.ms format.
*
* @param healthCheckWaitDuration the healthCheckWaitDuration value to set.
* @return the ClusterMonitoringPolicy object itself.
*/
public ClusterMonitoringPolicy withHealthCheckWaitDuration(String healthCheckWaitDuration) {
this.healthCheckWaitDuration = healthCheckWaitDuration;
return this;
}
/**
* Get the healthCheckStableDuration property: The amount of time that the application or cluster must remain
* healthy before the upgrade proceeds to the next upgrade domain. The duration can be in either hh:mm:ss or in
* d.hh:mm:ss.ms format.
*
* @return the healthCheckStableDuration value.
*/
public String healthCheckStableDuration() {
return this.healthCheckStableDuration;
}
/**
* Set the healthCheckStableDuration property: The amount of time that the application or cluster must remain
* healthy before the upgrade proceeds to the next upgrade domain. The duration can be in either hh:mm:ss or in
* d.hh:mm:ss.ms format.
*
* @param healthCheckStableDuration the healthCheckStableDuration value to set.
* @return the ClusterMonitoringPolicy object itself.
*/
public ClusterMonitoringPolicy withHealthCheckStableDuration(String healthCheckStableDuration) {
this.healthCheckStableDuration = healthCheckStableDuration;
return this;
}
/**
* Get the healthCheckRetryTimeout property: The amount of time to retry health evaluation when the application or
* cluster is unhealthy before the upgrade rolls back. The timeout can be in either hh:mm:ss or in d.hh:mm:ss.ms
* format.
*
* @return the healthCheckRetryTimeout value.
*/
public String healthCheckRetryTimeout() {
return this.healthCheckRetryTimeout;
}
/**
* Set the healthCheckRetryTimeout property: The amount of time to retry health evaluation when the application or
* cluster is unhealthy before the upgrade rolls back. The timeout can be in either hh:mm:ss or in d.hh:mm:ss.ms
* format.
*
* @param healthCheckRetryTimeout the healthCheckRetryTimeout value to set.
* @return the ClusterMonitoringPolicy object itself.
*/
public ClusterMonitoringPolicy withHealthCheckRetryTimeout(String healthCheckRetryTimeout) {
this.healthCheckRetryTimeout = healthCheckRetryTimeout;
return this;
}
/**
* Get the upgradeTimeout property: The amount of time the overall upgrade has to complete before the upgrade rolls
* back. The timeout can be in either hh:mm:ss or in d.hh:mm:ss.ms format.
*
* @return the upgradeTimeout value.
*/
public String upgradeTimeout() {
return this.upgradeTimeout;
}
/**
* Set the upgradeTimeout property: The amount of time the overall upgrade has to complete before the upgrade rolls
* back. The timeout can be in either hh:mm:ss or in d.hh:mm:ss.ms format.
*
* @param upgradeTimeout the upgradeTimeout value to set.
* @return the ClusterMonitoringPolicy object itself.
*/
public ClusterMonitoringPolicy withUpgradeTimeout(String upgradeTimeout) {
this.upgradeTimeout = upgradeTimeout;
return this;
}
/**
* Get the upgradeDomainTimeout property: The amount of time each upgrade domain has to complete before the upgrade
* rolls back. The timeout can be in either hh:mm:ss or in d.hh:mm:ss.ms format.
*
* @return the upgradeDomainTimeout value.
*/
public String upgradeDomainTimeout() {
return this.upgradeDomainTimeout;
}
/**
* Set the upgradeDomainTimeout property: The amount of time each upgrade domain has to complete before the upgrade
* rolls back. The timeout can be in either hh:mm:ss or in d.hh:mm:ss.ms format.
*
* @param upgradeDomainTimeout the upgradeDomainTimeout value to set.
* @return the ClusterMonitoringPolicy object itself.
*/
public ClusterMonitoringPolicy withUpgradeDomainTimeout(String upgradeDomainTimeout) {
this.upgradeDomainTimeout = upgradeDomainTimeout;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (healthCheckWaitDuration() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property healthCheckWaitDuration in model ClusterMonitoringPolicy"));
}
if (healthCheckStableDuration() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property healthCheckStableDuration in model ClusterMonitoringPolicy"));
}
if (healthCheckRetryTimeout() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property healthCheckRetryTimeout in model ClusterMonitoringPolicy"));
}
if (upgradeTimeout() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property upgradeTimeout in model ClusterMonitoringPolicy"));
}
if (upgradeDomainTimeout() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property upgradeDomainTimeout in model ClusterMonitoringPolicy"));
}
}
private static final ClientLogger LOGGER = new ClientLogger(ClusterMonitoringPolicy.class);
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("healthCheckWaitDuration", this.healthCheckWaitDuration);
jsonWriter.writeStringField("healthCheckStableDuration", this.healthCheckStableDuration);
jsonWriter.writeStringField("healthCheckRetryTimeout", this.healthCheckRetryTimeout);
jsonWriter.writeStringField("upgradeTimeout", this.upgradeTimeout);
jsonWriter.writeStringField("upgradeDomainTimeout", this.upgradeDomainTimeout);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of ClusterMonitoringPolicy from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of ClusterMonitoringPolicy if the JsonReader was pointing to an instance of it, or null if it
* was pointing to JSON null.
* @throws IllegalStateException If the deserialized JSON object was missing any required properties.
* @throws IOException If an error occurs while reading the ClusterMonitoringPolicy.
*/
public static ClusterMonitoringPolicy fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
ClusterMonitoringPolicy deserializedClusterMonitoringPolicy = new ClusterMonitoringPolicy();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("healthCheckWaitDuration".equals(fieldName)) {
deserializedClusterMonitoringPolicy.healthCheckWaitDuration = reader.getString();
} else if ("healthCheckStableDuration".equals(fieldName)) {
deserializedClusterMonitoringPolicy.healthCheckStableDuration = reader.getString();
} else if ("healthCheckRetryTimeout".equals(fieldName)) {
deserializedClusterMonitoringPolicy.healthCheckRetryTimeout = reader.getString();
} else if ("upgradeTimeout".equals(fieldName)) {
deserializedClusterMonitoringPolicy.upgradeTimeout = reader.getString();
} else if ("upgradeDomainTimeout".equals(fieldName)) {
deserializedClusterMonitoringPolicy.upgradeDomainTimeout = reader.getString();
} else {
reader.skipChildren();
}
}
return deserializedClusterMonitoringPolicy;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy