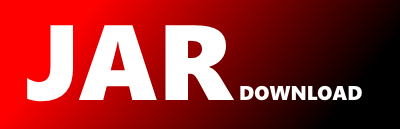
com.azure.resourcemanager.servicefabricmanagedclusters.models.ClusterUpgradePolicy Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.servicefabricmanagedclusters.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
/**
* Describes the policy used when upgrading the cluster.
*/
@Fluent
public final class ClusterUpgradePolicy implements JsonSerializable {
/*
* If true, then processes are forcefully restarted during upgrade even when the code version has not changed (the
* upgrade only changes configuration or data).
*/
private Boolean forceRestart;
/*
* The cluster health policy defines a health policy used to evaluate the health of the cluster during a cluster
* upgrade.
*/
private ClusterHealthPolicy healthPolicy;
/*
* The cluster delta health policy defines a health policy used to evaluate the health of the cluster during a
* cluster upgrade.
*/
private ClusterUpgradeDeltaHealthPolicy deltaHealthPolicy;
/*
* The cluster monitoring policy describes the parameters for monitoring an upgrade in Monitored mode.
*/
private ClusterMonitoringPolicy monitoringPolicy;
/*
* The maximum amount of time to block processing of an upgrade domain and prevent loss of availability when there
* are unexpected issues.
* When this timeout expires, processing of the upgrade domain will proceed regardless of availability loss issues.
* The timeout is reset at the start of each upgrade domain. The timeout can be in either hh:mm:ss or in
* d.hh:mm:ss.ms format.
* This value must be between 00:00:00 and 49710.06:28:15 (unsigned 32 bit integer for seconds)
*/
private String upgradeReplicaSetCheckTimeout;
/**
* Creates an instance of ClusterUpgradePolicy class.
*/
public ClusterUpgradePolicy() {
}
/**
* Get the forceRestart property: If true, then processes are forcefully restarted during upgrade even when the code
* version has not changed (the upgrade only changes configuration or data).
*
* @return the forceRestart value.
*/
public Boolean forceRestart() {
return this.forceRestart;
}
/**
* Set the forceRestart property: If true, then processes are forcefully restarted during upgrade even when the code
* version has not changed (the upgrade only changes configuration or data).
*
* @param forceRestart the forceRestart value to set.
* @return the ClusterUpgradePolicy object itself.
*/
public ClusterUpgradePolicy withForceRestart(Boolean forceRestart) {
this.forceRestart = forceRestart;
return this;
}
/**
* Get the healthPolicy property: The cluster health policy defines a health policy used to evaluate the health of
* the cluster during a cluster upgrade.
*
* @return the healthPolicy value.
*/
public ClusterHealthPolicy healthPolicy() {
return this.healthPolicy;
}
/**
* Set the healthPolicy property: The cluster health policy defines a health policy used to evaluate the health of
* the cluster during a cluster upgrade.
*
* @param healthPolicy the healthPolicy value to set.
* @return the ClusterUpgradePolicy object itself.
*/
public ClusterUpgradePolicy withHealthPolicy(ClusterHealthPolicy healthPolicy) {
this.healthPolicy = healthPolicy;
return this;
}
/**
* Get the deltaHealthPolicy property: The cluster delta health policy defines a health policy used to evaluate the
* health of the cluster during a cluster upgrade.
*
* @return the deltaHealthPolicy value.
*/
public ClusterUpgradeDeltaHealthPolicy deltaHealthPolicy() {
return this.deltaHealthPolicy;
}
/**
* Set the deltaHealthPolicy property: The cluster delta health policy defines a health policy used to evaluate the
* health of the cluster during a cluster upgrade.
*
* @param deltaHealthPolicy the deltaHealthPolicy value to set.
* @return the ClusterUpgradePolicy object itself.
*/
public ClusterUpgradePolicy withDeltaHealthPolicy(ClusterUpgradeDeltaHealthPolicy deltaHealthPolicy) {
this.deltaHealthPolicy = deltaHealthPolicy;
return this;
}
/**
* Get the monitoringPolicy property: The cluster monitoring policy describes the parameters for monitoring an
* upgrade in Monitored mode.
*
* @return the monitoringPolicy value.
*/
public ClusterMonitoringPolicy monitoringPolicy() {
return this.monitoringPolicy;
}
/**
* Set the monitoringPolicy property: The cluster monitoring policy describes the parameters for monitoring an
* upgrade in Monitored mode.
*
* @param monitoringPolicy the monitoringPolicy value to set.
* @return the ClusterUpgradePolicy object itself.
*/
public ClusterUpgradePolicy withMonitoringPolicy(ClusterMonitoringPolicy monitoringPolicy) {
this.monitoringPolicy = monitoringPolicy;
return this;
}
/**
* Get the upgradeReplicaSetCheckTimeout property: The maximum amount of time to block processing of an upgrade
* domain and prevent loss of availability when there are unexpected issues.
* When this timeout expires, processing of the upgrade domain will proceed regardless of availability loss issues.
* The timeout is reset at the start of each upgrade domain. The timeout can be in either hh:mm:ss or in
* d.hh:mm:ss.ms format.
* This value must be between 00:00:00 and 49710.06:28:15 (unsigned 32 bit integer for seconds).
*
* @return the upgradeReplicaSetCheckTimeout value.
*/
public String upgradeReplicaSetCheckTimeout() {
return this.upgradeReplicaSetCheckTimeout;
}
/**
* Set the upgradeReplicaSetCheckTimeout property: The maximum amount of time to block processing of an upgrade
* domain and prevent loss of availability when there are unexpected issues.
* When this timeout expires, processing of the upgrade domain will proceed regardless of availability loss issues.
* The timeout is reset at the start of each upgrade domain. The timeout can be in either hh:mm:ss or in
* d.hh:mm:ss.ms format.
* This value must be between 00:00:00 and 49710.06:28:15 (unsigned 32 bit integer for seconds).
*
* @param upgradeReplicaSetCheckTimeout the upgradeReplicaSetCheckTimeout value to set.
* @return the ClusterUpgradePolicy object itself.
*/
public ClusterUpgradePolicy withUpgradeReplicaSetCheckTimeout(String upgradeReplicaSetCheckTimeout) {
this.upgradeReplicaSetCheckTimeout = upgradeReplicaSetCheckTimeout;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (healthPolicy() != null) {
healthPolicy().validate();
}
if (deltaHealthPolicy() != null) {
deltaHealthPolicy().validate();
}
if (monitoringPolicy() != null) {
monitoringPolicy().validate();
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeBooleanField("forceRestart", this.forceRestart);
jsonWriter.writeJsonField("healthPolicy", this.healthPolicy);
jsonWriter.writeJsonField("deltaHealthPolicy", this.deltaHealthPolicy);
jsonWriter.writeJsonField("monitoringPolicy", this.monitoringPolicy);
jsonWriter.writeStringField("upgradeReplicaSetCheckTimeout", this.upgradeReplicaSetCheckTimeout);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of ClusterUpgradePolicy from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of ClusterUpgradePolicy if the JsonReader was pointing to an instance of it, or null if it
* was pointing to JSON null.
* @throws IOException If an error occurs while reading the ClusterUpgradePolicy.
*/
public static ClusterUpgradePolicy fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
ClusterUpgradePolicy deserializedClusterUpgradePolicy = new ClusterUpgradePolicy();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("forceRestart".equals(fieldName)) {
deserializedClusterUpgradePolicy.forceRestart = reader.getNullable(JsonReader::getBoolean);
} else if ("healthPolicy".equals(fieldName)) {
deserializedClusterUpgradePolicy.healthPolicy = ClusterHealthPolicy.fromJson(reader);
} else if ("deltaHealthPolicy".equals(fieldName)) {
deserializedClusterUpgradePolicy.deltaHealthPolicy
= ClusterUpgradeDeltaHealthPolicy.fromJson(reader);
} else if ("monitoringPolicy".equals(fieldName)) {
deserializedClusterUpgradePolicy.monitoringPolicy = ClusterMonitoringPolicy.fromJson(reader);
} else if ("upgradeReplicaSetCheckTimeout".equals(fieldName)) {
deserializedClusterUpgradePolicy.upgradeReplicaSetCheckTimeout = reader.getString();
} else {
reader.skipChildren();
}
}
return deserializedClusterUpgradePolicy;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy