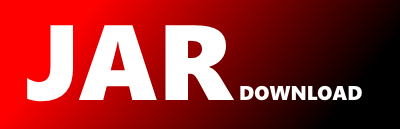
com.azure.resourcemanager.servicefabricmanagedclusters.models.ServiceResourceProperties Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.servicefabricmanagedclusters.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.logging.ClientLogger;
import com.azure.json.JsonReader;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.util.List;
/**
* The service resource properties.
*/
@Fluent
public class ServiceResourceProperties extends ServiceResourcePropertiesBase {
/*
* The kind of service (Stateless or Stateful).
*/
private ServiceKind serviceKind = ServiceKind.fromString("ServiceResourceProperties");
/*
* The current deployment or provisioning state, which only appears in the response
*/
private String provisioningState;
/*
* The name of the service type
*/
private String serviceTypeName;
/*
* Describes how the service is partitioned.
*/
private Partition partitionDescription;
/*
* The activation Mode of the service package
*/
private ServicePackageActivationMode servicePackageActivationMode;
/*
* Dns name used for the service. If this is specified, then the DNS name can be used to return the IP addresses of
* service endpoints for application layer protocols (e.g., HTTP).
* When updating serviceDnsName, old name may be temporarily resolvable. However, rely on new name.
* When removing serviceDnsName, removed name may temporarily be resolvable. Do not rely on the name being
* unresolvable.
*/
private String serviceDnsName;
/**
* Creates an instance of ServiceResourceProperties class.
*/
public ServiceResourceProperties() {
}
/**
* Get the serviceKind property: The kind of service (Stateless or Stateful).
*
* @return the serviceKind value.
*/
public ServiceKind serviceKind() {
return this.serviceKind;
}
/**
* Get the provisioningState property: The current deployment or provisioning state, which only appears in the
* response.
*
* @return the provisioningState value.
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* Set the provisioningState property: The current deployment or provisioning state, which only appears in the
* response.
*
* @param provisioningState the provisioningState value to set.
* @return the ServiceResourceProperties object itself.
*/
ServiceResourceProperties withProvisioningState(String provisioningState) {
this.provisioningState = provisioningState;
return this;
}
/**
* Get the serviceTypeName property: The name of the service type.
*
* @return the serviceTypeName value.
*/
public String serviceTypeName() {
return this.serviceTypeName;
}
/**
* Set the serviceTypeName property: The name of the service type.
*
* @param serviceTypeName the serviceTypeName value to set.
* @return the ServiceResourceProperties object itself.
*/
public ServiceResourceProperties withServiceTypeName(String serviceTypeName) {
this.serviceTypeName = serviceTypeName;
return this;
}
/**
* Get the partitionDescription property: Describes how the service is partitioned.
*
* @return the partitionDescription value.
*/
public Partition partitionDescription() {
return this.partitionDescription;
}
/**
* Set the partitionDescription property: Describes how the service is partitioned.
*
* @param partitionDescription the partitionDescription value to set.
* @return the ServiceResourceProperties object itself.
*/
public ServiceResourceProperties withPartitionDescription(Partition partitionDescription) {
this.partitionDescription = partitionDescription;
return this;
}
/**
* Get the servicePackageActivationMode property: The activation Mode of the service package.
*
* @return the servicePackageActivationMode value.
*/
public ServicePackageActivationMode servicePackageActivationMode() {
return this.servicePackageActivationMode;
}
/**
* Set the servicePackageActivationMode property: The activation Mode of the service package.
*
* @param servicePackageActivationMode the servicePackageActivationMode value to set.
* @return the ServiceResourceProperties object itself.
*/
public ServiceResourceProperties
withServicePackageActivationMode(ServicePackageActivationMode servicePackageActivationMode) {
this.servicePackageActivationMode = servicePackageActivationMode;
return this;
}
/**
* Get the serviceDnsName property: Dns name used for the service. If this is specified, then the DNS name can be
* used to return the IP addresses of service endpoints for application layer protocols (e.g., HTTP).
* When updating serviceDnsName, old name may be temporarily resolvable. However, rely on new name.
* When removing serviceDnsName, removed name may temporarily be resolvable. Do not rely on the name being
* unresolvable.
*
* @return the serviceDnsName value.
*/
public String serviceDnsName() {
return this.serviceDnsName;
}
/**
* Set the serviceDnsName property: Dns name used for the service. If this is specified, then the DNS name can be
* used to return the IP addresses of service endpoints for application layer protocols (e.g., HTTP).
* When updating serviceDnsName, old name may be temporarily resolvable. However, rely on new name.
* When removing serviceDnsName, removed name may temporarily be resolvable. Do not rely on the name being
* unresolvable.
*
* @param serviceDnsName the serviceDnsName value to set.
* @return the ServiceResourceProperties object itself.
*/
public ServiceResourceProperties withServiceDnsName(String serviceDnsName) {
this.serviceDnsName = serviceDnsName;
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ServiceResourceProperties withPlacementConstraints(String placementConstraints) {
super.withPlacementConstraints(placementConstraints);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ServiceResourceProperties withCorrelationScheme(List correlationScheme) {
super.withCorrelationScheme(correlationScheme);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ServiceResourceProperties withServiceLoadMetrics(List serviceLoadMetrics) {
super.withServiceLoadMetrics(serviceLoadMetrics);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ServiceResourceProperties
withServicePlacementPolicies(List servicePlacementPolicies) {
super.withServicePlacementPolicies(servicePlacementPolicies);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ServiceResourceProperties withDefaultMoveCost(MoveCost defaultMoveCost) {
super.withDefaultMoveCost(defaultMoveCost);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ServiceResourceProperties withScalingPolicies(List scalingPolicies) {
super.withScalingPolicies(scalingPolicies);
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
@Override
public void validate() {
super.validate();
if (serviceTypeName() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property serviceTypeName in model ServiceResourceProperties"));
}
if (partitionDescription() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property partitionDescription in model ServiceResourceProperties"));
} else {
partitionDescription().validate();
}
}
private static final ClientLogger LOGGER = new ClientLogger(ServiceResourceProperties.class);
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("placementConstraints", placementConstraints());
jsonWriter.writeArrayField("correlationScheme", correlationScheme(),
(writer, element) -> writer.writeJson(element));
jsonWriter.writeArrayField("serviceLoadMetrics", serviceLoadMetrics(),
(writer, element) -> writer.writeJson(element));
jsonWriter.writeArrayField("servicePlacementPolicies", servicePlacementPolicies(),
(writer, element) -> writer.writeJson(element));
jsonWriter.writeStringField("defaultMoveCost", defaultMoveCost() == null ? null : defaultMoveCost().toString());
jsonWriter.writeArrayField("scalingPolicies", scalingPolicies(),
(writer, element) -> writer.writeJson(element));
jsonWriter.writeStringField("serviceTypeName", this.serviceTypeName);
jsonWriter.writeJsonField("partitionDescription", this.partitionDescription);
jsonWriter.writeStringField("serviceKind", this.serviceKind == null ? null : this.serviceKind.toString());
jsonWriter.writeStringField("servicePackageActivationMode",
this.servicePackageActivationMode == null ? null : this.servicePackageActivationMode.toString());
jsonWriter.writeStringField("serviceDnsName", this.serviceDnsName);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of ServiceResourceProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of ServiceResourceProperties if the JsonReader was pointing to an instance of it, or null if
* it was pointing to JSON null.
* @throws IllegalStateException If the deserialized JSON object was missing any required properties.
* @throws IOException If an error occurs while reading the ServiceResourceProperties.
*/
public static ServiceResourceProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
String discriminatorValue = null;
try (JsonReader readerToUse = reader.bufferObject()) {
readerToUse.nextToken(); // Prepare for reading
while (readerToUse.nextToken() != JsonToken.END_OBJECT) {
String fieldName = readerToUse.getFieldName();
readerToUse.nextToken();
if ("serviceKind".equals(fieldName)) {
discriminatorValue = readerToUse.getString();
break;
} else {
readerToUse.skipChildren();
}
}
// Use the discriminator value to determine which subtype should be deserialized.
if ("Stateful".equals(discriminatorValue)) {
return StatefulServiceProperties.fromJson(readerToUse.reset());
} else if ("Stateless".equals(discriminatorValue)) {
return StatelessServiceProperties.fromJson(readerToUse.reset());
} else {
return fromJsonKnownDiscriminator(readerToUse.reset());
}
}
});
}
static ServiceResourceProperties fromJsonKnownDiscriminator(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
ServiceResourceProperties deserializedServiceResourceProperties = new ServiceResourceProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("placementConstraints".equals(fieldName)) {
deserializedServiceResourceProperties.withPlacementConstraints(reader.getString());
} else if ("correlationScheme".equals(fieldName)) {
List correlationScheme
= reader.readArray(reader1 -> ServiceCorrelation.fromJson(reader1));
deserializedServiceResourceProperties.withCorrelationScheme(correlationScheme);
} else if ("serviceLoadMetrics".equals(fieldName)) {
List serviceLoadMetrics
= reader.readArray(reader1 -> ServiceLoadMetric.fromJson(reader1));
deserializedServiceResourceProperties.withServiceLoadMetrics(serviceLoadMetrics);
} else if ("servicePlacementPolicies".equals(fieldName)) {
List servicePlacementPolicies
= reader.readArray(reader1 -> ServicePlacementPolicy.fromJson(reader1));
deserializedServiceResourceProperties.withServicePlacementPolicies(servicePlacementPolicies);
} else if ("defaultMoveCost".equals(fieldName)) {
deserializedServiceResourceProperties.withDefaultMoveCost(MoveCost.fromString(reader.getString()));
} else if ("scalingPolicies".equals(fieldName)) {
List scalingPolicies = reader.readArray(reader1 -> ScalingPolicy.fromJson(reader1));
deserializedServiceResourceProperties.withScalingPolicies(scalingPolicies);
} else if ("serviceTypeName".equals(fieldName)) {
deserializedServiceResourceProperties.serviceTypeName = reader.getString();
} else if ("partitionDescription".equals(fieldName)) {
deserializedServiceResourceProperties.partitionDescription = Partition.fromJson(reader);
} else if ("serviceKind".equals(fieldName)) {
deserializedServiceResourceProperties.serviceKind = ServiceKind.fromString(reader.getString());
} else if ("provisioningState".equals(fieldName)) {
deserializedServiceResourceProperties.provisioningState = reader.getString();
} else if ("servicePackageActivationMode".equals(fieldName)) {
deserializedServiceResourceProperties.servicePackageActivationMode
= ServicePackageActivationMode.fromString(reader.getString());
} else if ("serviceDnsName".equals(fieldName)) {
deserializedServiceResourceProperties.serviceDnsName = reader.getString();
} else {
reader.skipChildren();
}
}
return deserializedServiceResourceProperties;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy