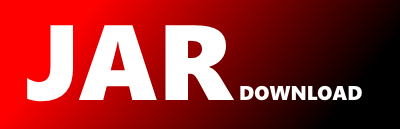
com.azure.resourcemanager.servicefabricmanagedclusters.models.StatelessServiceProperties Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.servicefabricmanagedclusters.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.util.List;
/**
* The properties of a stateless service resource.
*/
@Fluent
public final class StatelessServiceProperties extends ServiceResourceProperties {
/*
* The kind of service (Stateless or Stateful).
*/
private ServiceKind serviceKind = ServiceKind.STATELESS;
/*
* The instance count.
*/
private int instanceCount;
/*
* MinInstanceCount is the minimum number of instances that must be up to meet the EnsureAvailability safety check
* during operations like upgrade or deactivate node. The actual number that is used is max( MinInstanceCount, ceil(
* MinInstancePercentage/100.0 * InstanceCount) ). Note, if InstanceCount is set to -1, during MinInstanceCount
* computation -1 is first converted into the number of nodes on which the instances are allowed to be placed
* according to the placement constraints on the service.
*/
private Integer minInstanceCount;
/*
* MinInstancePercentage is the minimum percentage of InstanceCount that must be up to meet the EnsureAvailability
* safety check during operations like upgrade or deactivate node. The actual number that is used is max(
* MinInstanceCount, ceil( MinInstancePercentage/100.0 * InstanceCount) ). Note, if InstanceCount is set to -1,
* during MinInstancePercentage computation, -1 is first converted into the number of nodes on which the instances
* are allowed to be placed according to the placement constraints on the service.
*/
private Integer minInstancePercentage;
/*
* The current deployment or provisioning state, which only appears in the response
*/
private String provisioningState;
/**
* Creates an instance of StatelessServiceProperties class.
*/
public StatelessServiceProperties() {
}
/**
* Get the serviceKind property: The kind of service (Stateless or Stateful).
*
* @return the serviceKind value.
*/
@Override
public ServiceKind serviceKind() {
return this.serviceKind;
}
/**
* Get the instanceCount property: The instance count.
*
* @return the instanceCount value.
*/
public int instanceCount() {
return this.instanceCount;
}
/**
* Set the instanceCount property: The instance count.
*
* @param instanceCount the instanceCount value to set.
* @return the StatelessServiceProperties object itself.
*/
public StatelessServiceProperties withInstanceCount(int instanceCount) {
this.instanceCount = instanceCount;
return this;
}
/**
* Get the minInstanceCount property: MinInstanceCount is the minimum number of instances that must be up to meet
* the EnsureAvailability safety check during operations like upgrade or deactivate node. The actual number that is
* used is max( MinInstanceCount, ceil( MinInstancePercentage/100.0 * InstanceCount) ). Note, if InstanceCount is
* set to -1, during MinInstanceCount computation -1 is first converted into the number of nodes on which the
* instances are allowed to be placed according to the placement constraints on the service.
*
* @return the minInstanceCount value.
*/
public Integer minInstanceCount() {
return this.minInstanceCount;
}
/**
* Set the minInstanceCount property: MinInstanceCount is the minimum number of instances that must be up to meet
* the EnsureAvailability safety check during operations like upgrade or deactivate node. The actual number that is
* used is max( MinInstanceCount, ceil( MinInstancePercentage/100.0 * InstanceCount) ). Note, if InstanceCount is
* set to -1, during MinInstanceCount computation -1 is first converted into the number of nodes on which the
* instances are allowed to be placed according to the placement constraints on the service.
*
* @param minInstanceCount the minInstanceCount value to set.
* @return the StatelessServiceProperties object itself.
*/
public StatelessServiceProperties withMinInstanceCount(Integer minInstanceCount) {
this.minInstanceCount = minInstanceCount;
return this;
}
/**
* Get the minInstancePercentage property: MinInstancePercentage is the minimum percentage of InstanceCount that
* must be up to meet the EnsureAvailability safety check during operations like upgrade or deactivate node. The
* actual number that is used is max( MinInstanceCount, ceil( MinInstancePercentage/100.0 * InstanceCount) ). Note,
* if InstanceCount is set to -1, during MinInstancePercentage computation, -1 is first converted into the number of
* nodes on which the instances are allowed to be placed according to the placement constraints on the service.
*
* @return the minInstancePercentage value.
*/
public Integer minInstancePercentage() {
return this.minInstancePercentage;
}
/**
* Set the minInstancePercentage property: MinInstancePercentage is the minimum percentage of InstanceCount that
* must be up to meet the EnsureAvailability safety check during operations like upgrade or deactivate node. The
* actual number that is used is max( MinInstanceCount, ceil( MinInstancePercentage/100.0 * InstanceCount) ). Note,
* if InstanceCount is set to -1, during MinInstancePercentage computation, -1 is first converted into the number of
* nodes on which the instances are allowed to be placed according to the placement constraints on the service.
*
* @param minInstancePercentage the minInstancePercentage value to set.
* @return the StatelessServiceProperties object itself.
*/
public StatelessServiceProperties withMinInstancePercentage(Integer minInstancePercentage) {
this.minInstancePercentage = minInstancePercentage;
return this;
}
/**
* Get the provisioningState property: The current deployment or provisioning state, which only appears in the
* response.
*
* @return the provisioningState value.
*/
@Override
public String provisioningState() {
return this.provisioningState;
}
/**
* {@inheritDoc}
*/
@Override
public StatelessServiceProperties withServiceTypeName(String serviceTypeName) {
super.withServiceTypeName(serviceTypeName);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public StatelessServiceProperties withPartitionDescription(Partition partitionDescription) {
super.withPartitionDescription(partitionDescription);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public StatelessServiceProperties
withServicePackageActivationMode(ServicePackageActivationMode servicePackageActivationMode) {
super.withServicePackageActivationMode(servicePackageActivationMode);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public StatelessServiceProperties withServiceDnsName(String serviceDnsName) {
super.withServiceDnsName(serviceDnsName);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public StatelessServiceProperties withPlacementConstraints(String placementConstraints) {
super.withPlacementConstraints(placementConstraints);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public StatelessServiceProperties withCorrelationScheme(List correlationScheme) {
super.withCorrelationScheme(correlationScheme);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public StatelessServiceProperties withServiceLoadMetrics(List serviceLoadMetrics) {
super.withServiceLoadMetrics(serviceLoadMetrics);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public StatelessServiceProperties
withServicePlacementPolicies(List servicePlacementPolicies) {
super.withServicePlacementPolicies(servicePlacementPolicies);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public StatelessServiceProperties withDefaultMoveCost(MoveCost defaultMoveCost) {
super.withDefaultMoveCost(defaultMoveCost);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public StatelessServiceProperties withScalingPolicies(List scalingPolicies) {
super.withScalingPolicies(scalingPolicies);
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
@Override
public void validate() {
super.validate();
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("serviceTypeName", serviceTypeName());
jsonWriter.writeJsonField("partitionDescription", partitionDescription());
jsonWriter.writeStringField("placementConstraints", placementConstraints());
jsonWriter.writeArrayField("correlationScheme", correlationScheme(),
(writer, element) -> writer.writeJson(element));
jsonWriter.writeArrayField("serviceLoadMetrics", serviceLoadMetrics(),
(writer, element) -> writer.writeJson(element));
jsonWriter.writeArrayField("servicePlacementPolicies", servicePlacementPolicies(),
(writer, element) -> writer.writeJson(element));
jsonWriter.writeStringField("defaultMoveCost", defaultMoveCost() == null ? null : defaultMoveCost().toString());
jsonWriter.writeArrayField("scalingPolicies", scalingPolicies(),
(writer, element) -> writer.writeJson(element));
jsonWriter.writeStringField("servicePackageActivationMode",
servicePackageActivationMode() == null ? null : servicePackageActivationMode().toString());
jsonWriter.writeStringField("serviceDnsName", serviceDnsName());
jsonWriter.writeIntField("instanceCount", this.instanceCount);
jsonWriter.writeStringField("serviceKind", this.serviceKind == null ? null : this.serviceKind.toString());
jsonWriter.writeNumberField("minInstanceCount", this.minInstanceCount);
jsonWriter.writeNumberField("minInstancePercentage", this.minInstancePercentage);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of StatelessServiceProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of StatelessServiceProperties if the JsonReader was pointing to an instance of it, or null if
* it was pointing to JSON null.
* @throws IllegalStateException If the deserialized JSON object was missing any required properties.
* @throws IOException If an error occurs while reading the StatelessServiceProperties.
*/
public static StatelessServiceProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
StatelessServiceProperties deserializedStatelessServiceProperties = new StatelessServiceProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("serviceTypeName".equals(fieldName)) {
deserializedStatelessServiceProperties.withServiceTypeName(reader.getString());
} else if ("partitionDescription".equals(fieldName)) {
deserializedStatelessServiceProperties.withPartitionDescription(Partition.fromJson(reader));
} else if ("placementConstraints".equals(fieldName)) {
deserializedStatelessServiceProperties.withPlacementConstraints(reader.getString());
} else if ("correlationScheme".equals(fieldName)) {
List correlationScheme
= reader.readArray(reader1 -> ServiceCorrelation.fromJson(reader1));
deserializedStatelessServiceProperties.withCorrelationScheme(correlationScheme);
} else if ("serviceLoadMetrics".equals(fieldName)) {
List serviceLoadMetrics
= reader.readArray(reader1 -> ServiceLoadMetric.fromJson(reader1));
deserializedStatelessServiceProperties.withServiceLoadMetrics(serviceLoadMetrics);
} else if ("servicePlacementPolicies".equals(fieldName)) {
List servicePlacementPolicies
= reader.readArray(reader1 -> ServicePlacementPolicy.fromJson(reader1));
deserializedStatelessServiceProperties.withServicePlacementPolicies(servicePlacementPolicies);
} else if ("defaultMoveCost".equals(fieldName)) {
deserializedStatelessServiceProperties.withDefaultMoveCost(MoveCost.fromString(reader.getString()));
} else if ("scalingPolicies".equals(fieldName)) {
List scalingPolicies = reader.readArray(reader1 -> ScalingPolicy.fromJson(reader1));
deserializedStatelessServiceProperties.withScalingPolicies(scalingPolicies);
} else if ("provisioningState".equals(fieldName)) {
deserializedStatelessServiceProperties.provisioningState = reader.getString();
} else if ("servicePackageActivationMode".equals(fieldName)) {
deserializedStatelessServiceProperties
.withServicePackageActivationMode(ServicePackageActivationMode.fromString(reader.getString()));
} else if ("serviceDnsName".equals(fieldName)) {
deserializedStatelessServiceProperties.withServiceDnsName(reader.getString());
} else if ("instanceCount".equals(fieldName)) {
deserializedStatelessServiceProperties.instanceCount = reader.getInt();
} else if ("serviceKind".equals(fieldName)) {
deserializedStatelessServiceProperties.serviceKind = ServiceKind.fromString(reader.getString());
} else if ("minInstanceCount".equals(fieldName)) {
deserializedStatelessServiceProperties.minInstanceCount = reader.getNullable(JsonReader::getInt);
} else if ("minInstancePercentage".equals(fieldName)) {
deserializedStatelessServiceProperties.minInstancePercentage
= reader.getNullable(JsonReader::getInt);
} else {
reader.skipChildren();
}
}
return deserializedStatelessServiceProperties;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy