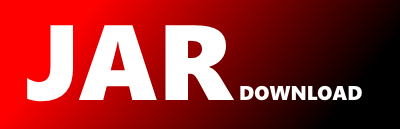
com.azure.resourcemanager.sql.fluent.models.AutomaticTuningServerProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-sql Show documentation
Show all versions of azure-resourcemanager-sql Show documentation
This package contains Microsoft Azure Sql Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.sql.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.sql.models.AutomaticTuningServerMode;
import com.azure.resourcemanager.sql.models.AutomaticTuningServerOptions;
import java.io.IOException;
import java.util.Map;
/**
* Server-level Automatic Tuning properties.
*/
@Fluent
public final class AutomaticTuningServerProperties implements JsonSerializable {
/*
* Automatic tuning desired state.
*/
private AutomaticTuningServerMode desiredState;
/*
* Automatic tuning actual state.
*/
private AutomaticTuningServerMode actualState;
/*
* Automatic tuning options definition.
*/
private Map options;
/**
* Creates an instance of AutomaticTuningServerProperties class.
*/
public AutomaticTuningServerProperties() {
}
/**
* Get the desiredState property: Automatic tuning desired state.
*
* @return the desiredState value.
*/
public AutomaticTuningServerMode desiredState() {
return this.desiredState;
}
/**
* Set the desiredState property: Automatic tuning desired state.
*
* @param desiredState the desiredState value to set.
* @return the AutomaticTuningServerProperties object itself.
*/
public AutomaticTuningServerProperties withDesiredState(AutomaticTuningServerMode desiredState) {
this.desiredState = desiredState;
return this;
}
/**
* Get the actualState property: Automatic tuning actual state.
*
* @return the actualState value.
*/
public AutomaticTuningServerMode actualState() {
return this.actualState;
}
/**
* Get the options property: Automatic tuning options definition.
*
* @return the options value.
*/
public Map options() {
return this.options;
}
/**
* Set the options property: Automatic tuning options definition.
*
* @param options the options value to set.
* @return the AutomaticTuningServerProperties object itself.
*/
public AutomaticTuningServerProperties withOptions(Map options) {
this.options = options;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (options() != null) {
options().values().forEach(e -> {
if (e != null) {
e.validate();
}
});
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("desiredState", this.desiredState == null ? null : this.desiredState.toString());
jsonWriter.writeMapField("options", this.options, (writer, element) -> writer.writeJson(element));
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of AutomaticTuningServerProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of AutomaticTuningServerProperties if the JsonReader was pointing to an instance of it, or
* null if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the AutomaticTuningServerProperties.
*/
public static AutomaticTuningServerProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
AutomaticTuningServerProperties deserializedAutomaticTuningServerProperties
= new AutomaticTuningServerProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("desiredState".equals(fieldName)) {
deserializedAutomaticTuningServerProperties.desiredState
= AutomaticTuningServerMode.fromString(reader.getString());
} else if ("actualState".equals(fieldName)) {
deserializedAutomaticTuningServerProperties.actualState
= AutomaticTuningServerMode.fromString(reader.getString());
} else if ("options".equals(fieldName)) {
Map options
= reader.readMap(reader1 -> AutomaticTuningServerOptions.fromJson(reader1));
deserializedAutomaticTuningServerProperties.options = options;
} else {
reader.skipChildren();
}
}
return deserializedAutomaticTuningServerProperties;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy