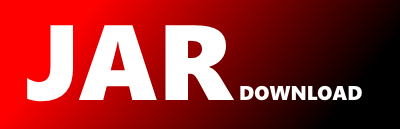
com.azure.resourcemanager.sql.fluent.models.DataMaskingRuleProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-sql Show documentation
Show all versions of azure-resourcemanager-sql Show documentation
This package contains Microsoft Azure Sql Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.sql.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.logging.ClientLogger;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.sql.models.DataMaskingFunction;
import com.azure.resourcemanager.sql.models.DataMaskingRuleState;
import java.io.IOException;
/**
* The properties of a database data masking rule.
*/
@Fluent
public final class DataMaskingRuleProperties implements JsonSerializable {
/*
* The rule Id.
*/
private String id;
/*
* The rule state. Used to delete a rule. To delete an existing rule, specify the schemaName, tableName, columnName,
* maskingFunction, and specify ruleState as disabled. However, if the rule doesn't already exist, the rule will be
* created with ruleState set to enabled, regardless of the provided value of ruleState.
*/
private DataMaskingRuleState ruleState;
/*
* The schema name on which the data masking rule is applied.
*/
private String schemaName;
/*
* The table name on which the data masking rule is applied.
*/
private String tableName;
/*
* The column name on which the data masking rule is applied.
*/
private String columnName;
/*
* The alias name. This is a legacy parameter and is no longer used.
*/
private String aliasName;
/*
* The masking function that is used for the data masking rule.
*/
private DataMaskingFunction maskingFunction;
/*
* The numberFrom property of the masking rule. Required if maskingFunction is set to Number, otherwise this
* parameter will be ignored.
*/
private String numberFrom;
/*
* The numberTo property of the data masking rule. Required if maskingFunction is set to Number, otherwise this
* parameter will be ignored.
*/
private String numberTo;
/*
* If maskingFunction is set to Text, the number of characters to show unmasked in the beginning of the string.
* Otherwise, this parameter will be ignored.
*/
private String prefixSize;
/*
* If maskingFunction is set to Text, the number of characters to show unmasked at the end of the string. Otherwise,
* this parameter will be ignored.
*/
private String suffixSize;
/*
* If maskingFunction is set to Text, the character to use for masking the unexposed part of the string. Otherwise,
* this parameter will be ignored.
*/
private String replacementString;
/**
* Creates an instance of DataMaskingRuleProperties class.
*/
public DataMaskingRuleProperties() {
}
/**
* Get the id property: The rule Id.
*
* @return the id value.
*/
public String id() {
return this.id;
}
/**
* Get the ruleState property: The rule state. Used to delete a rule. To delete an existing rule, specify the
* schemaName, tableName, columnName, maskingFunction, and specify ruleState as disabled. However, if the rule
* doesn't already exist, the rule will be created with ruleState set to enabled, regardless of the provided value
* of ruleState.
*
* @return the ruleState value.
*/
public DataMaskingRuleState ruleState() {
return this.ruleState;
}
/**
* Set the ruleState property: The rule state. Used to delete a rule. To delete an existing rule, specify the
* schemaName, tableName, columnName, maskingFunction, and specify ruleState as disabled. However, if the rule
* doesn't already exist, the rule will be created with ruleState set to enabled, regardless of the provided value
* of ruleState.
*
* @param ruleState the ruleState value to set.
* @return the DataMaskingRuleProperties object itself.
*/
public DataMaskingRuleProperties withRuleState(DataMaskingRuleState ruleState) {
this.ruleState = ruleState;
return this;
}
/**
* Get the schemaName property: The schema name on which the data masking rule is applied.
*
* @return the schemaName value.
*/
public String schemaName() {
return this.schemaName;
}
/**
* Set the schemaName property: The schema name on which the data masking rule is applied.
*
* @param schemaName the schemaName value to set.
* @return the DataMaskingRuleProperties object itself.
*/
public DataMaskingRuleProperties withSchemaName(String schemaName) {
this.schemaName = schemaName;
return this;
}
/**
* Get the tableName property: The table name on which the data masking rule is applied.
*
* @return the tableName value.
*/
public String tableName() {
return this.tableName;
}
/**
* Set the tableName property: The table name on which the data masking rule is applied.
*
* @param tableName the tableName value to set.
* @return the DataMaskingRuleProperties object itself.
*/
public DataMaskingRuleProperties withTableName(String tableName) {
this.tableName = tableName;
return this;
}
/**
* Get the columnName property: The column name on which the data masking rule is applied.
*
* @return the columnName value.
*/
public String columnName() {
return this.columnName;
}
/**
* Set the columnName property: The column name on which the data masking rule is applied.
*
* @param columnName the columnName value to set.
* @return the DataMaskingRuleProperties object itself.
*/
public DataMaskingRuleProperties withColumnName(String columnName) {
this.columnName = columnName;
return this;
}
/**
* Get the aliasName property: The alias name. This is a legacy parameter and is no longer used.
*
* @return the aliasName value.
*/
public String aliasName() {
return this.aliasName;
}
/**
* Set the aliasName property: The alias name. This is a legacy parameter and is no longer used.
*
* @param aliasName the aliasName value to set.
* @return the DataMaskingRuleProperties object itself.
*/
public DataMaskingRuleProperties withAliasName(String aliasName) {
this.aliasName = aliasName;
return this;
}
/**
* Get the maskingFunction property: The masking function that is used for the data masking rule.
*
* @return the maskingFunction value.
*/
public DataMaskingFunction maskingFunction() {
return this.maskingFunction;
}
/**
* Set the maskingFunction property: The masking function that is used for the data masking rule.
*
* @param maskingFunction the maskingFunction value to set.
* @return the DataMaskingRuleProperties object itself.
*/
public DataMaskingRuleProperties withMaskingFunction(DataMaskingFunction maskingFunction) {
this.maskingFunction = maskingFunction;
return this;
}
/**
* Get the numberFrom property: The numberFrom property of the masking rule. Required if maskingFunction is set to
* Number, otherwise this parameter will be ignored.
*
* @return the numberFrom value.
*/
public String numberFrom() {
return this.numberFrom;
}
/**
* Set the numberFrom property: The numberFrom property of the masking rule. Required if maskingFunction is set to
* Number, otherwise this parameter will be ignored.
*
* @param numberFrom the numberFrom value to set.
* @return the DataMaskingRuleProperties object itself.
*/
public DataMaskingRuleProperties withNumberFrom(String numberFrom) {
this.numberFrom = numberFrom;
return this;
}
/**
* Get the numberTo property: The numberTo property of the data masking rule. Required if maskingFunction is set to
* Number, otherwise this parameter will be ignored.
*
* @return the numberTo value.
*/
public String numberTo() {
return this.numberTo;
}
/**
* Set the numberTo property: The numberTo property of the data masking rule. Required if maskingFunction is set to
* Number, otherwise this parameter will be ignored.
*
* @param numberTo the numberTo value to set.
* @return the DataMaskingRuleProperties object itself.
*/
public DataMaskingRuleProperties withNumberTo(String numberTo) {
this.numberTo = numberTo;
return this;
}
/**
* Get the prefixSize property: If maskingFunction is set to Text, the number of characters to show unmasked in the
* beginning of the string. Otherwise, this parameter will be ignored.
*
* @return the prefixSize value.
*/
public String prefixSize() {
return this.prefixSize;
}
/**
* Set the prefixSize property: If maskingFunction is set to Text, the number of characters to show unmasked in the
* beginning of the string. Otherwise, this parameter will be ignored.
*
* @param prefixSize the prefixSize value to set.
* @return the DataMaskingRuleProperties object itself.
*/
public DataMaskingRuleProperties withPrefixSize(String prefixSize) {
this.prefixSize = prefixSize;
return this;
}
/**
* Get the suffixSize property: If maskingFunction is set to Text, the number of characters to show unmasked at the
* end of the string. Otherwise, this parameter will be ignored.
*
* @return the suffixSize value.
*/
public String suffixSize() {
return this.suffixSize;
}
/**
* Set the suffixSize property: If maskingFunction is set to Text, the number of characters to show unmasked at the
* end of the string. Otherwise, this parameter will be ignored.
*
* @param suffixSize the suffixSize value to set.
* @return the DataMaskingRuleProperties object itself.
*/
public DataMaskingRuleProperties withSuffixSize(String suffixSize) {
this.suffixSize = suffixSize;
return this;
}
/**
* Get the replacementString property: If maskingFunction is set to Text, the character to use for masking the
* unexposed part of the string. Otherwise, this parameter will be ignored.
*
* @return the replacementString value.
*/
public String replacementString() {
return this.replacementString;
}
/**
* Set the replacementString property: If maskingFunction is set to Text, the character to use for masking the
* unexposed part of the string. Otherwise, this parameter will be ignored.
*
* @param replacementString the replacementString value to set.
* @return the DataMaskingRuleProperties object itself.
*/
public DataMaskingRuleProperties withReplacementString(String replacementString) {
this.replacementString = replacementString;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (schemaName() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property schemaName in model DataMaskingRuleProperties"));
}
if (tableName() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property tableName in model DataMaskingRuleProperties"));
}
if (columnName() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property columnName in model DataMaskingRuleProperties"));
}
if (maskingFunction() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property maskingFunction in model DataMaskingRuleProperties"));
}
}
private static final ClientLogger LOGGER = new ClientLogger(DataMaskingRuleProperties.class);
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("schemaName", this.schemaName);
jsonWriter.writeStringField("tableName", this.tableName);
jsonWriter.writeStringField("columnName", this.columnName);
jsonWriter.writeStringField("maskingFunction",
this.maskingFunction == null ? null : this.maskingFunction.toString());
jsonWriter.writeStringField("ruleState", this.ruleState == null ? null : this.ruleState.toString());
jsonWriter.writeStringField("aliasName", this.aliasName);
jsonWriter.writeStringField("numberFrom", this.numberFrom);
jsonWriter.writeStringField("numberTo", this.numberTo);
jsonWriter.writeStringField("prefixSize", this.prefixSize);
jsonWriter.writeStringField("suffixSize", this.suffixSize);
jsonWriter.writeStringField("replacementString", this.replacementString);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of DataMaskingRuleProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of DataMaskingRuleProperties if the JsonReader was pointing to an instance of it, or null if
* it was pointing to JSON null.
* @throws IllegalStateException If the deserialized JSON object was missing any required properties.
* @throws IOException If an error occurs while reading the DataMaskingRuleProperties.
*/
public static DataMaskingRuleProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
DataMaskingRuleProperties deserializedDataMaskingRuleProperties = new DataMaskingRuleProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("schemaName".equals(fieldName)) {
deserializedDataMaskingRuleProperties.schemaName = reader.getString();
} else if ("tableName".equals(fieldName)) {
deserializedDataMaskingRuleProperties.tableName = reader.getString();
} else if ("columnName".equals(fieldName)) {
deserializedDataMaskingRuleProperties.columnName = reader.getString();
} else if ("maskingFunction".equals(fieldName)) {
deserializedDataMaskingRuleProperties.maskingFunction
= DataMaskingFunction.fromString(reader.getString());
} else if ("id".equals(fieldName)) {
deserializedDataMaskingRuleProperties.id = reader.getString();
} else if ("ruleState".equals(fieldName)) {
deserializedDataMaskingRuleProperties.ruleState
= DataMaskingRuleState.fromString(reader.getString());
} else if ("aliasName".equals(fieldName)) {
deserializedDataMaskingRuleProperties.aliasName = reader.getString();
} else if ("numberFrom".equals(fieldName)) {
deserializedDataMaskingRuleProperties.numberFrom = reader.getString();
} else if ("numberTo".equals(fieldName)) {
deserializedDataMaskingRuleProperties.numberTo = reader.getString();
} else if ("prefixSize".equals(fieldName)) {
deserializedDataMaskingRuleProperties.prefixSize = reader.getString();
} else if ("suffixSize".equals(fieldName)) {
deserializedDataMaskingRuleProperties.suffixSize = reader.getString();
} else if ("replacementString".equals(fieldName)) {
deserializedDataMaskingRuleProperties.replacementString = reader.getString();
} else {
reader.skipChildren();
}
}
return deserializedDataMaskingRuleProperties;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy