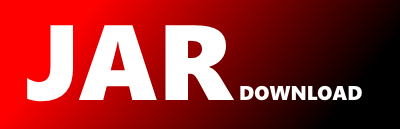
com.azure.resourcemanager.sql.fluent.models.DatabaseInner Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-sql Show documentation
Show all versions of azure-resourcemanager-sql Show documentation
This package contains Microsoft Azure Sql Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.sql.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.management.Resource;
import com.azure.json.JsonReader;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.sql.models.BackupStorageRedundancy;
import com.azure.resourcemanager.sql.models.CatalogCollationType;
import com.azure.resourcemanager.sql.models.CreateMode;
import com.azure.resourcemanager.sql.models.DatabaseIdentity;
import com.azure.resourcemanager.sql.models.DatabaseLicenseType;
import com.azure.resourcemanager.sql.models.DatabaseReadScale;
import com.azure.resourcemanager.sql.models.DatabaseStatus;
import com.azure.resourcemanager.sql.models.SampleName;
import com.azure.resourcemanager.sql.models.SecondaryType;
import com.azure.resourcemanager.sql.models.Sku;
import java.io.IOException;
import java.time.OffsetDateTime;
import java.util.Map;
import java.util.UUID;
/**
* A database resource.
*/
@Fluent
public final class DatabaseInner extends Resource {
/*
* The database SKU.
*
* The list of SKUs may vary by region and support offer. To determine the SKUs (including the SKU name,
* tier/edition, family, and capacity) that are available to your subscription in an Azure region, use the
* `Capabilities_ListByLocation` REST API or one of the following commands:
*
* ```azurecli
* az sql db list-editions -l -o table
* ````
*
* ```powershell
* Get-AzSqlServerServiceObjective -Location
* ````
*/
private Sku sku;
/*
* Kind of database. This is metadata used for the Azure portal experience.
*/
private String kind;
/*
* Resource that manages the database.
*/
private String managedBy;
/*
* The Azure Active Directory identity of the database.
*/
private DatabaseIdentity identity;
/*
* Resource properties.
*/
private DatabaseProperties innerProperties;
/*
* The type of the resource.
*/
private String type;
/*
* The name of the resource.
*/
private String name;
/*
* Fully qualified resource Id for the resource.
*/
private String id;
/**
* Creates an instance of DatabaseInner class.
*/
public DatabaseInner() {
}
/**
* Get the sku property: The database SKU.
*
* The list of SKUs may vary by region and support offer. To determine the SKUs (including the SKU name,
* tier/edition, family, and capacity) that are available to your subscription in an Azure region, use the
* `Capabilities_ListByLocation` REST API or one of the following commands:
*
* ```azurecli
* az sql db list-editions -l <location> -o table
* ````
*
* ```powershell
* Get-AzSqlServerServiceObjective -Location <location>
* ````.
*
* @return the sku value.
*/
public Sku sku() {
return this.sku;
}
/**
* Set the sku property: The database SKU.
*
* The list of SKUs may vary by region and support offer. To determine the SKUs (including the SKU name,
* tier/edition, family, and capacity) that are available to your subscription in an Azure region, use the
* `Capabilities_ListByLocation` REST API or one of the following commands:
*
* ```azurecli
* az sql db list-editions -l <location> -o table
* ````
*
* ```powershell
* Get-AzSqlServerServiceObjective -Location <location>
* ````.
*
* @param sku the sku value to set.
* @return the DatabaseInner object itself.
*/
public DatabaseInner withSku(Sku sku) {
this.sku = sku;
return this;
}
/**
* Get the kind property: Kind of database. This is metadata used for the Azure portal experience.
*
* @return the kind value.
*/
public String kind() {
return this.kind;
}
/**
* Get the managedBy property: Resource that manages the database.
*
* @return the managedBy value.
*/
public String managedBy() {
return this.managedBy;
}
/**
* Get the identity property: The Azure Active Directory identity of the database.
*
* @return the identity value.
*/
public DatabaseIdentity identity() {
return this.identity;
}
/**
* Set the identity property: The Azure Active Directory identity of the database.
*
* @param identity the identity value to set.
* @return the DatabaseInner object itself.
*/
public DatabaseInner withIdentity(DatabaseIdentity identity) {
this.identity = identity;
return this;
}
/**
* Get the innerProperties property: Resource properties.
*
* @return the innerProperties value.
*/
private DatabaseProperties innerProperties() {
return this.innerProperties;
}
/**
* Get the type property: The type of the resource.
*
* @return the type value.
*/
@Override
public String type() {
return this.type;
}
/**
* Get the name property: The name of the resource.
*
* @return the name value.
*/
@Override
public String name() {
return this.name;
}
/**
* Get the id property: Fully qualified resource Id for the resource.
*
* @return the id value.
*/
@Override
public String id() {
return this.id;
}
/**
* {@inheritDoc}
*/
@Override
public DatabaseInner withLocation(String location) {
super.withLocation(location);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public DatabaseInner withTags(Map tags) {
super.withTags(tags);
return this;
}
/**
* Get the createMode property: Specifies the mode of database creation.
*
* Default: regular database creation.
*
* Copy: creates a database as a copy of an existing database. sourceDatabaseId must be specified as the resource ID
* of the source database.
*
* Secondary: creates a database as a secondary replica of an existing database. sourceDatabaseId must be specified
* as the resource ID of the existing primary database.
*
* PointInTimeRestore: Creates a database by restoring a point in time backup of an existing database.
* sourceDatabaseId must be specified as the resource ID of the existing database, and restorePointInTime must be
* specified.
*
* Recovery: Creates a database by restoring a geo-replicated backup. sourceDatabaseId must be specified as the
* recoverable database resource ID to restore.
*
* Restore: Creates a database by restoring a backup of a deleted database. sourceDatabaseId must be specified. If
* sourceDatabaseId is the database's original resource ID, then sourceDatabaseDeletionDate must be specified.
* Otherwise sourceDatabaseId must be the restorable dropped database resource ID and sourceDatabaseDeletionDate is
* ignored. restorePointInTime may also be specified to restore from an earlier point in time.
*
* RestoreLongTermRetentionBackup: Creates a database by restoring from a long term retention vault.
* recoveryServicesRecoveryPointResourceId must be specified as the recovery point resource ID.
*
* Copy, Secondary, and RestoreLongTermRetentionBackup are not supported for DataWarehouse edition.
*
* @return the createMode value.
*/
public CreateMode createMode() {
return this.innerProperties() == null ? null : this.innerProperties().createMode();
}
/**
* Set the createMode property: Specifies the mode of database creation.
*
* Default: regular database creation.
*
* Copy: creates a database as a copy of an existing database. sourceDatabaseId must be specified as the resource ID
* of the source database.
*
* Secondary: creates a database as a secondary replica of an existing database. sourceDatabaseId must be specified
* as the resource ID of the existing primary database.
*
* PointInTimeRestore: Creates a database by restoring a point in time backup of an existing database.
* sourceDatabaseId must be specified as the resource ID of the existing database, and restorePointInTime must be
* specified.
*
* Recovery: Creates a database by restoring a geo-replicated backup. sourceDatabaseId must be specified as the
* recoverable database resource ID to restore.
*
* Restore: Creates a database by restoring a backup of a deleted database. sourceDatabaseId must be specified. If
* sourceDatabaseId is the database's original resource ID, then sourceDatabaseDeletionDate must be specified.
* Otherwise sourceDatabaseId must be the restorable dropped database resource ID and sourceDatabaseDeletionDate is
* ignored. restorePointInTime may also be specified to restore from an earlier point in time.
*
* RestoreLongTermRetentionBackup: Creates a database by restoring from a long term retention vault.
* recoveryServicesRecoveryPointResourceId must be specified as the recovery point resource ID.
*
* Copy, Secondary, and RestoreLongTermRetentionBackup are not supported for DataWarehouse edition.
*
* @param createMode the createMode value to set.
* @return the DatabaseInner object itself.
*/
public DatabaseInner withCreateMode(CreateMode createMode) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseProperties();
}
this.innerProperties().withCreateMode(createMode);
return this;
}
/**
* Get the collation property: The collation of the database.
*
* @return the collation value.
*/
public String collation() {
return this.innerProperties() == null ? null : this.innerProperties().collation();
}
/**
* Set the collation property: The collation of the database.
*
* @param collation the collation value to set.
* @return the DatabaseInner object itself.
*/
public DatabaseInner withCollation(String collation) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseProperties();
}
this.innerProperties().withCollation(collation);
return this;
}
/**
* Get the maxSizeBytes property: The max size of the database expressed in bytes.
*
* @return the maxSizeBytes value.
*/
public Long maxSizeBytes() {
return this.innerProperties() == null ? null : this.innerProperties().maxSizeBytes();
}
/**
* Set the maxSizeBytes property: The max size of the database expressed in bytes.
*
* @param maxSizeBytes the maxSizeBytes value to set.
* @return the DatabaseInner object itself.
*/
public DatabaseInner withMaxSizeBytes(Long maxSizeBytes) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseProperties();
}
this.innerProperties().withMaxSizeBytes(maxSizeBytes);
return this;
}
/**
* Get the sampleName property: The name of the sample schema to apply when creating this database.
*
* @return the sampleName value.
*/
public SampleName sampleName() {
return this.innerProperties() == null ? null : this.innerProperties().sampleName();
}
/**
* Set the sampleName property: The name of the sample schema to apply when creating this database.
*
* @param sampleName the sampleName value to set.
* @return the DatabaseInner object itself.
*/
public DatabaseInner withSampleName(SampleName sampleName) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseProperties();
}
this.innerProperties().withSampleName(sampleName);
return this;
}
/**
* Get the elasticPoolId property: The resource identifier of the elastic pool containing this database.
*
* @return the elasticPoolId value.
*/
public String elasticPoolId() {
return this.innerProperties() == null ? null : this.innerProperties().elasticPoolId();
}
/**
* Set the elasticPoolId property: The resource identifier of the elastic pool containing this database.
*
* @param elasticPoolId the elasticPoolId value to set.
* @return the DatabaseInner object itself.
*/
public DatabaseInner withElasticPoolId(String elasticPoolId) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseProperties();
}
this.innerProperties().withElasticPoolId(elasticPoolId);
return this;
}
/**
* Get the sourceDatabaseId property: The resource identifier of the source database associated with create
* operation of this database.
*
* @return the sourceDatabaseId value.
*/
public String sourceDatabaseId() {
return this.innerProperties() == null ? null : this.innerProperties().sourceDatabaseId();
}
/**
* Set the sourceDatabaseId property: The resource identifier of the source database associated with create
* operation of this database.
*
* @param sourceDatabaseId the sourceDatabaseId value to set.
* @return the DatabaseInner object itself.
*/
public DatabaseInner withSourceDatabaseId(String sourceDatabaseId) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseProperties();
}
this.innerProperties().withSourceDatabaseId(sourceDatabaseId);
return this;
}
/**
* Get the status property: The status of the database.
*
* @return the status value.
*/
public DatabaseStatus status() {
return this.innerProperties() == null ? null : this.innerProperties().status();
}
/**
* Get the databaseId property: The ID of the database.
*
* @return the databaseId value.
*/
public UUID databaseId() {
return this.innerProperties() == null ? null : this.innerProperties().databaseId();
}
/**
* Get the creationDate property: The creation date of the database (ISO8601 format).
*
* @return the creationDate value.
*/
public OffsetDateTime creationDate() {
return this.innerProperties() == null ? null : this.innerProperties().creationDate();
}
/**
* Get the currentServiceObjectiveName property: The current service level objective name of the database.
*
* @return the currentServiceObjectiveName value.
*/
public String currentServiceObjectiveName() {
return this.innerProperties() == null ? null : this.innerProperties().currentServiceObjectiveName();
}
/**
* Get the requestedServiceObjectiveName property: The requested service level objective name of the database.
*
* @return the requestedServiceObjectiveName value.
*/
public String requestedServiceObjectiveName() {
return this.innerProperties() == null ? null : this.innerProperties().requestedServiceObjectiveName();
}
/**
* Get the defaultSecondaryLocation property: The default secondary region for this database.
*
* @return the defaultSecondaryLocation value.
*/
public String defaultSecondaryLocation() {
return this.innerProperties() == null ? null : this.innerProperties().defaultSecondaryLocation();
}
/**
* Get the failoverGroupId property: Failover Group resource identifier that this database belongs to.
*
* @return the failoverGroupId value.
*/
public String failoverGroupId() {
return this.innerProperties() == null ? null : this.innerProperties().failoverGroupId();
}
/**
* Get the restorePointInTime property: Specifies the point in time (ISO8601 format) of the source database that
* will be restored to create the new database.
*
* @return the restorePointInTime value.
*/
public OffsetDateTime restorePointInTime() {
return this.innerProperties() == null ? null : this.innerProperties().restorePointInTime();
}
/**
* Set the restorePointInTime property: Specifies the point in time (ISO8601 format) of the source database that
* will be restored to create the new database.
*
* @param restorePointInTime the restorePointInTime value to set.
* @return the DatabaseInner object itself.
*/
public DatabaseInner withRestorePointInTime(OffsetDateTime restorePointInTime) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseProperties();
}
this.innerProperties().withRestorePointInTime(restorePointInTime);
return this;
}
/**
* Get the sourceDatabaseDeletionDate property: Specifies the time that the database was deleted.
*
* @return the sourceDatabaseDeletionDate value.
*/
public OffsetDateTime sourceDatabaseDeletionDate() {
return this.innerProperties() == null ? null : this.innerProperties().sourceDatabaseDeletionDate();
}
/**
* Set the sourceDatabaseDeletionDate property: Specifies the time that the database was deleted.
*
* @param sourceDatabaseDeletionDate the sourceDatabaseDeletionDate value to set.
* @return the DatabaseInner object itself.
*/
public DatabaseInner withSourceDatabaseDeletionDate(OffsetDateTime sourceDatabaseDeletionDate) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseProperties();
}
this.innerProperties().withSourceDatabaseDeletionDate(sourceDatabaseDeletionDate);
return this;
}
/**
* Get the recoveryServicesRecoveryPointId property: The resource identifier of the recovery point associated with
* create operation of this database.
*
* @return the recoveryServicesRecoveryPointId value.
*/
public String recoveryServicesRecoveryPointId() {
return this.innerProperties() == null ? null : this.innerProperties().recoveryServicesRecoveryPointId();
}
/**
* Set the recoveryServicesRecoveryPointId property: The resource identifier of the recovery point associated with
* create operation of this database.
*
* @param recoveryServicesRecoveryPointId the recoveryServicesRecoveryPointId value to set.
* @return the DatabaseInner object itself.
*/
public DatabaseInner withRecoveryServicesRecoveryPointId(String recoveryServicesRecoveryPointId) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseProperties();
}
this.innerProperties().withRecoveryServicesRecoveryPointId(recoveryServicesRecoveryPointId);
return this;
}
/**
* Get the longTermRetentionBackupResourceId property: The resource identifier of the long term retention backup
* associated with create operation of this database.
*
* @return the longTermRetentionBackupResourceId value.
*/
public String longTermRetentionBackupResourceId() {
return this.innerProperties() == null ? null : this.innerProperties().longTermRetentionBackupResourceId();
}
/**
* Set the longTermRetentionBackupResourceId property: The resource identifier of the long term retention backup
* associated with create operation of this database.
*
* @param longTermRetentionBackupResourceId the longTermRetentionBackupResourceId value to set.
* @return the DatabaseInner object itself.
*/
public DatabaseInner withLongTermRetentionBackupResourceId(String longTermRetentionBackupResourceId) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseProperties();
}
this.innerProperties().withLongTermRetentionBackupResourceId(longTermRetentionBackupResourceId);
return this;
}
/**
* Get the recoverableDatabaseId property: The resource identifier of the recoverable database associated with
* create operation of this database.
*
* @return the recoverableDatabaseId value.
*/
public String recoverableDatabaseId() {
return this.innerProperties() == null ? null : this.innerProperties().recoverableDatabaseId();
}
/**
* Set the recoverableDatabaseId property: The resource identifier of the recoverable database associated with
* create operation of this database.
*
* @param recoverableDatabaseId the recoverableDatabaseId value to set.
* @return the DatabaseInner object itself.
*/
public DatabaseInner withRecoverableDatabaseId(String recoverableDatabaseId) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseProperties();
}
this.innerProperties().withRecoverableDatabaseId(recoverableDatabaseId);
return this;
}
/**
* Get the restorableDroppedDatabaseId property: The resource identifier of the restorable dropped database
* associated with create operation of this database.
*
* @return the restorableDroppedDatabaseId value.
*/
public String restorableDroppedDatabaseId() {
return this.innerProperties() == null ? null : this.innerProperties().restorableDroppedDatabaseId();
}
/**
* Set the restorableDroppedDatabaseId property: The resource identifier of the restorable dropped database
* associated with create operation of this database.
*
* @param restorableDroppedDatabaseId the restorableDroppedDatabaseId value to set.
* @return the DatabaseInner object itself.
*/
public DatabaseInner withRestorableDroppedDatabaseId(String restorableDroppedDatabaseId) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseProperties();
}
this.innerProperties().withRestorableDroppedDatabaseId(restorableDroppedDatabaseId);
return this;
}
/**
* Get the catalogCollation property: Collation of the metadata catalog.
*
* @return the catalogCollation value.
*/
public CatalogCollationType catalogCollation() {
return this.innerProperties() == null ? null : this.innerProperties().catalogCollation();
}
/**
* Set the catalogCollation property: Collation of the metadata catalog.
*
* @param catalogCollation the catalogCollation value to set.
* @return the DatabaseInner object itself.
*/
public DatabaseInner withCatalogCollation(CatalogCollationType catalogCollation) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseProperties();
}
this.innerProperties().withCatalogCollation(catalogCollation);
return this;
}
/**
* Get the zoneRedundant property: Whether or not this database is zone redundant, which means the replicas of this
* database will be spread across multiple availability zones.
*
* @return the zoneRedundant value.
*/
public Boolean zoneRedundant() {
return this.innerProperties() == null ? null : this.innerProperties().zoneRedundant();
}
/**
* Set the zoneRedundant property: Whether or not this database is zone redundant, which means the replicas of this
* database will be spread across multiple availability zones.
*
* @param zoneRedundant the zoneRedundant value to set.
* @return the DatabaseInner object itself.
*/
public DatabaseInner withZoneRedundant(Boolean zoneRedundant) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseProperties();
}
this.innerProperties().withZoneRedundant(zoneRedundant);
return this;
}
/**
* Get the licenseType property: The license type to apply for this database. `LicenseIncluded` if you need a
* license, or `BasePrice` if you have a license and are eligible for the Azure Hybrid Benefit.
*
* @return the licenseType value.
*/
public DatabaseLicenseType licenseType() {
return this.innerProperties() == null ? null : this.innerProperties().licenseType();
}
/**
* Set the licenseType property: The license type to apply for this database. `LicenseIncluded` if you need a
* license, or `BasePrice` if you have a license and are eligible for the Azure Hybrid Benefit.
*
* @param licenseType the licenseType value to set.
* @return the DatabaseInner object itself.
*/
public DatabaseInner withLicenseType(DatabaseLicenseType licenseType) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseProperties();
}
this.innerProperties().withLicenseType(licenseType);
return this;
}
/**
* Get the maxLogSizeBytes property: The max log size for this database.
*
* @return the maxLogSizeBytes value.
*/
public Long maxLogSizeBytes() {
return this.innerProperties() == null ? null : this.innerProperties().maxLogSizeBytes();
}
/**
* Get the earliestRestoreDate property: This records the earliest start date and time that restore is available for
* this database (ISO8601 format).
*
* @return the earliestRestoreDate value.
*/
public OffsetDateTime earliestRestoreDate() {
return this.innerProperties() == null ? null : this.innerProperties().earliestRestoreDate();
}
/**
* Get the readScale property: The state of read-only routing. If enabled, connections that have application intent
* set to readonly in their connection string may be routed to a readonly secondary replica in the same region. Not
* applicable to a Hyperscale database within an elastic pool.
*
* @return the readScale value.
*/
public DatabaseReadScale readScale() {
return this.innerProperties() == null ? null : this.innerProperties().readScale();
}
/**
* Set the readScale property: The state of read-only routing. If enabled, connections that have application intent
* set to readonly in their connection string may be routed to a readonly secondary replica in the same region. Not
* applicable to a Hyperscale database within an elastic pool.
*
* @param readScale the readScale value to set.
* @return the DatabaseInner object itself.
*/
public DatabaseInner withReadScale(DatabaseReadScale readScale) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseProperties();
}
this.innerProperties().withReadScale(readScale);
return this;
}
/**
* Get the highAvailabilityReplicaCount property: The number of secondary replicas associated with the database that
* are used to provide high availability. Not applicable to a Hyperscale database within an elastic pool.
*
* @return the highAvailabilityReplicaCount value.
*/
public Integer highAvailabilityReplicaCount() {
return this.innerProperties() == null ? null : this.innerProperties().highAvailabilityReplicaCount();
}
/**
* Set the highAvailabilityReplicaCount property: The number of secondary replicas associated with the database that
* are used to provide high availability. Not applicable to a Hyperscale database within an elastic pool.
*
* @param highAvailabilityReplicaCount the highAvailabilityReplicaCount value to set.
* @return the DatabaseInner object itself.
*/
public DatabaseInner withHighAvailabilityReplicaCount(Integer highAvailabilityReplicaCount) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseProperties();
}
this.innerProperties().withHighAvailabilityReplicaCount(highAvailabilityReplicaCount);
return this;
}
/**
* Get the secondaryType property: The secondary type of the database if it is a secondary. Valid values are Geo and
* Named.
*
* @return the secondaryType value.
*/
public SecondaryType secondaryType() {
return this.innerProperties() == null ? null : this.innerProperties().secondaryType();
}
/**
* Set the secondaryType property: The secondary type of the database if it is a secondary. Valid values are Geo and
* Named.
*
* @param secondaryType the secondaryType value to set.
* @return the DatabaseInner object itself.
*/
public DatabaseInner withSecondaryType(SecondaryType secondaryType) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseProperties();
}
this.innerProperties().withSecondaryType(secondaryType);
return this;
}
/**
* Get the currentSku property: The name and tier of the SKU.
*
* @return the currentSku value.
*/
public Sku currentSku() {
return this.innerProperties() == null ? null : this.innerProperties().currentSku();
}
/**
* Get the autoPauseDelay property: Time in minutes after which database is automatically paused. A value of -1
* means that automatic pause is disabled.
*
* @return the autoPauseDelay value.
*/
public Integer autoPauseDelay() {
return this.innerProperties() == null ? null : this.innerProperties().autoPauseDelay();
}
/**
* Set the autoPauseDelay property: Time in minutes after which database is automatically paused. A value of -1
* means that automatic pause is disabled.
*
* @param autoPauseDelay the autoPauseDelay value to set.
* @return the DatabaseInner object itself.
*/
public DatabaseInner withAutoPauseDelay(Integer autoPauseDelay) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseProperties();
}
this.innerProperties().withAutoPauseDelay(autoPauseDelay);
return this;
}
/**
* Get the currentBackupStorageRedundancy property: The storage account type used to store backups for this
* database.
*
* @return the currentBackupStorageRedundancy value.
*/
public BackupStorageRedundancy currentBackupStorageRedundancy() {
return this.innerProperties() == null ? null : this.innerProperties().currentBackupStorageRedundancy();
}
/**
* Get the requestedBackupStorageRedundancy property: The storage account type to be used to store backups for this
* database.
*
* @return the requestedBackupStorageRedundancy value.
*/
public BackupStorageRedundancy requestedBackupStorageRedundancy() {
return this.innerProperties() == null ? null : this.innerProperties().requestedBackupStorageRedundancy();
}
/**
* Set the requestedBackupStorageRedundancy property: The storage account type to be used to store backups for this
* database.
*
* @param requestedBackupStorageRedundancy the requestedBackupStorageRedundancy value to set.
* @return the DatabaseInner object itself.
*/
public DatabaseInner
withRequestedBackupStorageRedundancy(BackupStorageRedundancy requestedBackupStorageRedundancy) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseProperties();
}
this.innerProperties().withRequestedBackupStorageRedundancy(requestedBackupStorageRedundancy);
return this;
}
/**
* Get the minCapacity property: Minimal capacity that database will always have allocated, if not paused.
*
* @return the minCapacity value.
*/
public Double minCapacity() {
return this.innerProperties() == null ? null : this.innerProperties().minCapacity();
}
/**
* Set the minCapacity property: Minimal capacity that database will always have allocated, if not paused.
*
* @param minCapacity the minCapacity value to set.
* @return the DatabaseInner object itself.
*/
public DatabaseInner withMinCapacity(Double minCapacity) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseProperties();
}
this.innerProperties().withMinCapacity(minCapacity);
return this;
}
/**
* Get the pausedDate property: The date when database was paused by user configuration or action(ISO8601 format).
* Null if the database is ready.
*
* @return the pausedDate value.
*/
public OffsetDateTime pausedDate() {
return this.innerProperties() == null ? null : this.innerProperties().pausedDate();
}
/**
* Get the resumedDate property: The date when database was resumed by user action or database login (ISO8601
* format). Null if the database is paused.
*
* @return the resumedDate value.
*/
public OffsetDateTime resumedDate() {
return this.innerProperties() == null ? null : this.innerProperties().resumedDate();
}
/**
* Get the maintenanceConfigurationId property: Maintenance configuration id assigned to the database. This
* configuration defines the period when the maintenance updates will occur.
*
* @return the maintenanceConfigurationId value.
*/
public String maintenanceConfigurationId() {
return this.innerProperties() == null ? null : this.innerProperties().maintenanceConfigurationId();
}
/**
* Set the maintenanceConfigurationId property: Maintenance configuration id assigned to the database. This
* configuration defines the period when the maintenance updates will occur.
*
* @param maintenanceConfigurationId the maintenanceConfigurationId value to set.
* @return the DatabaseInner object itself.
*/
public DatabaseInner withMaintenanceConfigurationId(String maintenanceConfigurationId) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseProperties();
}
this.innerProperties().withMaintenanceConfigurationId(maintenanceConfigurationId);
return this;
}
/**
* Get the isLedgerOn property: Whether or not this database is a ledger database, which means all tables in the
* database are ledger tables. Note: the value of this property cannot be changed after the database has been
* created.
*
* @return the isLedgerOn value.
*/
public Boolean isLedgerOn() {
return this.innerProperties() == null ? null : this.innerProperties().isLedgerOn();
}
/**
* Set the isLedgerOn property: Whether or not this database is a ledger database, which means all tables in the
* database are ledger tables. Note: the value of this property cannot be changed after the database has been
* created.
*
* @param isLedgerOn the isLedgerOn value to set.
* @return the DatabaseInner object itself.
*/
public DatabaseInner withIsLedgerOn(Boolean isLedgerOn) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseProperties();
}
this.innerProperties().withIsLedgerOn(isLedgerOn);
return this;
}
/**
* Get the isInfraEncryptionEnabled property: Infra encryption is enabled for this database.
*
* @return the isInfraEncryptionEnabled value.
*/
public Boolean isInfraEncryptionEnabled() {
return this.innerProperties() == null ? null : this.innerProperties().isInfraEncryptionEnabled();
}
/**
* Get the federatedClientId property: The Client id used for cross tenant per database CMK scenario.
*
* @return the federatedClientId value.
*/
public UUID federatedClientId() {
return this.innerProperties() == null ? null : this.innerProperties().federatedClientId();
}
/**
* Set the federatedClientId property: The Client id used for cross tenant per database CMK scenario.
*
* @param federatedClientId the federatedClientId value to set.
* @return the DatabaseInner object itself.
*/
public DatabaseInner withFederatedClientId(UUID federatedClientId) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseProperties();
}
this.innerProperties().withFederatedClientId(federatedClientId);
return this;
}
/**
* Get the sourceResourceId property: The resource identifier of the source associated with the create operation of
* this database.
*
* This property is only supported for DataWarehouse edition and allows to restore across subscriptions.
*
* When sourceResourceId is specified, sourceDatabaseId, recoverableDatabaseId, restorableDroppedDatabaseId and
* sourceDatabaseDeletionDate must not be specified and CreateMode must be PointInTimeRestore, Restore or Recover.
*
* When createMode is PointInTimeRestore, sourceResourceId must be the resource ID of the existing database or
* existing sql pool, and restorePointInTime must be specified.
*
* When createMode is Restore, sourceResourceId must be the resource ID of restorable dropped database or restorable
* dropped sql pool.
*
* When createMode is Recover, sourceResourceId must be the resource ID of recoverable database or recoverable sql
* pool.
*
* When source subscription belongs to a different tenant than target subscription, “x-ms-authorization-auxiliary”
* header must contain authentication token for the source tenant. For more details about
* “x-ms-authorization-auxiliary” header see
* https://docs.microsoft.com/en-us/azure/azure-resource-manager/management/authenticate-multi-tenant.
*
* @return the sourceResourceId value.
*/
public String sourceResourceId() {
return this.innerProperties() == null ? null : this.innerProperties().sourceResourceId();
}
/**
* Set the sourceResourceId property: The resource identifier of the source associated with the create operation of
* this database.
*
* This property is only supported for DataWarehouse edition and allows to restore across subscriptions.
*
* When sourceResourceId is specified, sourceDatabaseId, recoverableDatabaseId, restorableDroppedDatabaseId and
* sourceDatabaseDeletionDate must not be specified and CreateMode must be PointInTimeRestore, Restore or Recover.
*
* When createMode is PointInTimeRestore, sourceResourceId must be the resource ID of the existing database or
* existing sql pool, and restorePointInTime must be specified.
*
* When createMode is Restore, sourceResourceId must be the resource ID of restorable dropped database or restorable
* dropped sql pool.
*
* When createMode is Recover, sourceResourceId must be the resource ID of recoverable database or recoverable sql
* pool.
*
* When source subscription belongs to a different tenant than target subscription, “x-ms-authorization-auxiliary”
* header must contain authentication token for the source tenant. For more details about
* “x-ms-authorization-auxiliary” header see
* https://docs.microsoft.com/en-us/azure/azure-resource-manager/management/authenticate-multi-tenant.
*
* @param sourceResourceId the sourceResourceId value to set.
* @return the DatabaseInner object itself.
*/
public DatabaseInner withSourceResourceId(String sourceResourceId) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseProperties();
}
this.innerProperties().withSourceResourceId(sourceResourceId);
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (sku() != null) {
sku().validate();
}
if (identity() != null) {
identity().validate();
}
if (innerProperties() != null) {
innerProperties().validate();
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("location", location());
jsonWriter.writeMapField("tags", tags(), (writer, element) -> writer.writeString(element));
jsonWriter.writeJsonField("sku", this.sku);
jsonWriter.writeJsonField("identity", this.identity);
jsonWriter.writeJsonField("properties", this.innerProperties);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of DatabaseInner from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of DatabaseInner if the JsonReader was pointing to an instance of it, or null if it was
* pointing to JSON null.
* @throws IllegalStateException If the deserialized JSON object was missing any required properties.
* @throws IOException If an error occurs while reading the DatabaseInner.
*/
public static DatabaseInner fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
DatabaseInner deserializedDatabaseInner = new DatabaseInner();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("id".equals(fieldName)) {
deserializedDatabaseInner.id = reader.getString();
} else if ("name".equals(fieldName)) {
deserializedDatabaseInner.name = reader.getString();
} else if ("type".equals(fieldName)) {
deserializedDatabaseInner.type = reader.getString();
} else if ("location".equals(fieldName)) {
deserializedDatabaseInner.withLocation(reader.getString());
} else if ("tags".equals(fieldName)) {
Map tags = reader.readMap(reader1 -> reader1.getString());
deserializedDatabaseInner.withTags(tags);
} else if ("sku".equals(fieldName)) {
deserializedDatabaseInner.sku = Sku.fromJson(reader);
} else if ("kind".equals(fieldName)) {
deserializedDatabaseInner.kind = reader.getString();
} else if ("managedBy".equals(fieldName)) {
deserializedDatabaseInner.managedBy = reader.getString();
} else if ("identity".equals(fieldName)) {
deserializedDatabaseInner.identity = DatabaseIdentity.fromJson(reader);
} else if ("properties".equals(fieldName)) {
deserializedDatabaseInner.innerProperties = DatabaseProperties.fromJson(reader);
} else {
reader.skipChildren();
}
}
return deserializedDatabaseInner;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy