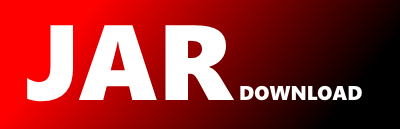
com.azure.resourcemanager.sql.fluent.models.DatabaseProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-sql Show documentation
Show all versions of azure-resourcemanager-sql Show documentation
This package contains Microsoft Azure Sql Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.sql.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.CoreUtils;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.sql.models.BackupStorageRedundancy;
import com.azure.resourcemanager.sql.models.CatalogCollationType;
import com.azure.resourcemanager.sql.models.CreateMode;
import com.azure.resourcemanager.sql.models.DatabaseLicenseType;
import com.azure.resourcemanager.sql.models.DatabaseReadScale;
import com.azure.resourcemanager.sql.models.DatabaseStatus;
import com.azure.resourcemanager.sql.models.SampleName;
import com.azure.resourcemanager.sql.models.SecondaryType;
import com.azure.resourcemanager.sql.models.Sku;
import java.io.IOException;
import java.time.OffsetDateTime;
import java.time.format.DateTimeFormatter;
import java.util.Objects;
import java.util.UUID;
/**
* The database's properties.
*/
@Fluent
public final class DatabaseProperties implements JsonSerializable {
/*
* Specifies the mode of database creation.
*
* Default: regular database creation.
*
* Copy: creates a database as a copy of an existing database. sourceDatabaseId must be specified as the resource ID
* of the source database.
*
* Secondary: creates a database as a secondary replica of an existing database. sourceDatabaseId must be specified
* as the resource ID of the existing primary database.
*
* PointInTimeRestore: Creates a database by restoring a point in time backup of an existing database.
* sourceDatabaseId must be specified as the resource ID of the existing database, and restorePointInTime must be
* specified.
*
* Recovery: Creates a database by restoring a geo-replicated backup. sourceDatabaseId must be specified as the
* recoverable database resource ID to restore.
*
* Restore: Creates a database by restoring a backup of a deleted database. sourceDatabaseId must be specified. If
* sourceDatabaseId is the database's original resource ID, then sourceDatabaseDeletionDate must be specified.
* Otherwise sourceDatabaseId must be the restorable dropped database resource ID and sourceDatabaseDeletionDate is
* ignored. restorePointInTime may also be specified to restore from an earlier point in time.
*
* RestoreLongTermRetentionBackup: Creates a database by restoring from a long term retention vault.
* recoveryServicesRecoveryPointResourceId must be specified as the recovery point resource ID.
*
* Copy, Secondary, and RestoreLongTermRetentionBackup are not supported for DataWarehouse edition.
*/
private CreateMode createMode;
/*
* The collation of the database.
*/
private String collation;
/*
* The max size of the database expressed in bytes.
*/
private Long maxSizeBytes;
/*
* The name of the sample schema to apply when creating this database.
*/
private SampleName sampleName;
/*
* The resource identifier of the elastic pool containing this database.
*/
private String elasticPoolId;
/*
* The resource identifier of the source database associated with create operation of this database.
*/
private String sourceDatabaseId;
/*
* The status of the database.
*/
private DatabaseStatus status;
/*
* The ID of the database.
*/
private UUID databaseId;
/*
* The creation date of the database (ISO8601 format).
*/
private OffsetDateTime creationDate;
/*
* The current service level objective name of the database.
*/
private String currentServiceObjectiveName;
/*
* The requested service level objective name of the database.
*/
private String requestedServiceObjectiveName;
/*
* The default secondary region for this database.
*/
private String defaultSecondaryLocation;
/*
* Failover Group resource identifier that this database belongs to.
*/
private String failoverGroupId;
/*
* Specifies the point in time (ISO8601 format) of the source database that will be restored to create the new
* database.
*/
private OffsetDateTime restorePointInTime;
/*
* Specifies the time that the database was deleted.
*/
private OffsetDateTime sourceDatabaseDeletionDate;
/*
* The resource identifier of the recovery point associated with create operation of this database.
*/
private String recoveryServicesRecoveryPointId;
/*
* The resource identifier of the long term retention backup associated with create operation of this database.
*/
private String longTermRetentionBackupResourceId;
/*
* The resource identifier of the recoverable database associated with create operation of this database.
*/
private String recoverableDatabaseId;
/*
* The resource identifier of the restorable dropped database associated with create operation of this database.
*/
private String restorableDroppedDatabaseId;
/*
* Collation of the metadata catalog.
*/
private CatalogCollationType catalogCollation;
/*
* Whether or not this database is zone redundant, which means the replicas of this database will be spread across
* multiple availability zones.
*/
private Boolean zoneRedundant;
/*
* The license type to apply for this database. `LicenseIncluded` if you need a license, or `BasePrice` if you have
* a license and are eligible for the Azure Hybrid Benefit.
*/
private DatabaseLicenseType licenseType;
/*
* The max log size for this database.
*/
private Long maxLogSizeBytes;
/*
* This records the earliest start date and time that restore is available for this database (ISO8601 format).
*/
private OffsetDateTime earliestRestoreDate;
/*
* The state of read-only routing. If enabled, connections that have application intent set to readonly in their
* connection string may be routed to a readonly secondary replica in the same region. Not applicable to a
* Hyperscale database within an elastic pool.
*/
private DatabaseReadScale readScale;
/*
* The number of secondary replicas associated with the database that are used to provide high availability. Not
* applicable to a Hyperscale database within an elastic pool.
*/
private Integer highAvailabilityReplicaCount;
/*
* The secondary type of the database if it is a secondary. Valid values are Geo and Named.
*/
private SecondaryType secondaryType;
/*
* The name and tier of the SKU.
*/
private Sku currentSku;
/*
* Time in minutes after which database is automatically paused. A value of -1 means that automatic pause is
* disabled
*/
private Integer autoPauseDelay;
/*
* The storage account type used to store backups for this database.
*/
private BackupStorageRedundancy currentBackupStorageRedundancy;
/*
* The storage account type to be used to store backups for this database.
*/
private BackupStorageRedundancy requestedBackupStorageRedundancy;
/*
* Minimal capacity that database will always have allocated, if not paused
*/
private Double minCapacity;
/*
* The date when database was paused by user configuration or action(ISO8601 format). Null if the database is ready.
*/
private OffsetDateTime pausedDate;
/*
* The date when database was resumed by user action or database login (ISO8601 format). Null if the database is
* paused.
*/
private OffsetDateTime resumedDate;
/*
* Maintenance configuration id assigned to the database. This configuration defines the period when the maintenance
* updates will occur.
*/
private String maintenanceConfigurationId;
/*
* Whether or not this database is a ledger database, which means all tables in the database are ledger tables.
* Note: the value of this property cannot be changed after the database has been created.
*/
private Boolean isLedgerOn;
/*
* Infra encryption is enabled for this database.
*/
private Boolean isInfraEncryptionEnabled;
/*
* The Client id used for cross tenant per database CMK scenario
*/
private UUID federatedClientId;
/*
* The resource identifier of the source associated with the create operation of this database.
*
* This property is only supported for DataWarehouse edition and allows to restore across subscriptions.
*
* When sourceResourceId is specified, sourceDatabaseId, recoverableDatabaseId, restorableDroppedDatabaseId and
* sourceDatabaseDeletionDate must not be specified and CreateMode must be PointInTimeRestore, Restore or Recover.
*
* When createMode is PointInTimeRestore, sourceResourceId must be the resource ID of the existing database or
* existing sql pool, and restorePointInTime must be specified.
*
* When createMode is Restore, sourceResourceId must be the resource ID of restorable dropped database or restorable
* dropped sql pool.
*
* When createMode is Recover, sourceResourceId must be the resource ID of recoverable database or recoverable sql
* pool.
*
* When source subscription belongs to a different tenant than target subscription, “x-ms-authorization-auxiliary”
* header must contain authentication token for the source tenant. For more details about
* “x-ms-authorization-auxiliary” header see
* https://docs.microsoft.com/en-us/azure/azure-resource-manager/management/authenticate-multi-tenant
*/
private String sourceResourceId;
/**
* Creates an instance of DatabaseProperties class.
*/
public DatabaseProperties() {
}
/**
* Get the createMode property: Specifies the mode of database creation.
*
* Default: regular database creation.
*
* Copy: creates a database as a copy of an existing database. sourceDatabaseId must be specified as the resource ID
* of the source database.
*
* Secondary: creates a database as a secondary replica of an existing database. sourceDatabaseId must be specified
* as the resource ID of the existing primary database.
*
* PointInTimeRestore: Creates a database by restoring a point in time backup of an existing database.
* sourceDatabaseId must be specified as the resource ID of the existing database, and restorePointInTime must be
* specified.
*
* Recovery: Creates a database by restoring a geo-replicated backup. sourceDatabaseId must be specified as the
* recoverable database resource ID to restore.
*
* Restore: Creates a database by restoring a backup of a deleted database. sourceDatabaseId must be specified. If
* sourceDatabaseId is the database's original resource ID, then sourceDatabaseDeletionDate must be specified.
* Otherwise sourceDatabaseId must be the restorable dropped database resource ID and sourceDatabaseDeletionDate is
* ignored. restorePointInTime may also be specified to restore from an earlier point in time.
*
* RestoreLongTermRetentionBackup: Creates a database by restoring from a long term retention vault.
* recoveryServicesRecoveryPointResourceId must be specified as the recovery point resource ID.
*
* Copy, Secondary, and RestoreLongTermRetentionBackup are not supported for DataWarehouse edition.
*
* @return the createMode value.
*/
public CreateMode createMode() {
return this.createMode;
}
/**
* Set the createMode property: Specifies the mode of database creation.
*
* Default: regular database creation.
*
* Copy: creates a database as a copy of an existing database. sourceDatabaseId must be specified as the resource ID
* of the source database.
*
* Secondary: creates a database as a secondary replica of an existing database. sourceDatabaseId must be specified
* as the resource ID of the existing primary database.
*
* PointInTimeRestore: Creates a database by restoring a point in time backup of an existing database.
* sourceDatabaseId must be specified as the resource ID of the existing database, and restorePointInTime must be
* specified.
*
* Recovery: Creates a database by restoring a geo-replicated backup. sourceDatabaseId must be specified as the
* recoverable database resource ID to restore.
*
* Restore: Creates a database by restoring a backup of a deleted database. sourceDatabaseId must be specified. If
* sourceDatabaseId is the database's original resource ID, then sourceDatabaseDeletionDate must be specified.
* Otherwise sourceDatabaseId must be the restorable dropped database resource ID and sourceDatabaseDeletionDate is
* ignored. restorePointInTime may also be specified to restore from an earlier point in time.
*
* RestoreLongTermRetentionBackup: Creates a database by restoring from a long term retention vault.
* recoveryServicesRecoveryPointResourceId must be specified as the recovery point resource ID.
*
* Copy, Secondary, and RestoreLongTermRetentionBackup are not supported for DataWarehouse edition.
*
* @param createMode the createMode value to set.
* @return the DatabaseProperties object itself.
*/
public DatabaseProperties withCreateMode(CreateMode createMode) {
this.createMode = createMode;
return this;
}
/**
* Get the collation property: The collation of the database.
*
* @return the collation value.
*/
public String collation() {
return this.collation;
}
/**
* Set the collation property: The collation of the database.
*
* @param collation the collation value to set.
* @return the DatabaseProperties object itself.
*/
public DatabaseProperties withCollation(String collation) {
this.collation = collation;
return this;
}
/**
* Get the maxSizeBytes property: The max size of the database expressed in bytes.
*
* @return the maxSizeBytes value.
*/
public Long maxSizeBytes() {
return this.maxSizeBytes;
}
/**
* Set the maxSizeBytes property: The max size of the database expressed in bytes.
*
* @param maxSizeBytes the maxSizeBytes value to set.
* @return the DatabaseProperties object itself.
*/
public DatabaseProperties withMaxSizeBytes(Long maxSizeBytes) {
this.maxSizeBytes = maxSizeBytes;
return this;
}
/**
* Get the sampleName property: The name of the sample schema to apply when creating this database.
*
* @return the sampleName value.
*/
public SampleName sampleName() {
return this.sampleName;
}
/**
* Set the sampleName property: The name of the sample schema to apply when creating this database.
*
* @param sampleName the sampleName value to set.
* @return the DatabaseProperties object itself.
*/
public DatabaseProperties withSampleName(SampleName sampleName) {
this.sampleName = sampleName;
return this;
}
/**
* Get the elasticPoolId property: The resource identifier of the elastic pool containing this database.
*
* @return the elasticPoolId value.
*/
public String elasticPoolId() {
return this.elasticPoolId;
}
/**
* Set the elasticPoolId property: The resource identifier of the elastic pool containing this database.
*
* @param elasticPoolId the elasticPoolId value to set.
* @return the DatabaseProperties object itself.
*/
public DatabaseProperties withElasticPoolId(String elasticPoolId) {
this.elasticPoolId = elasticPoolId;
return this;
}
/**
* Get the sourceDatabaseId property: The resource identifier of the source database associated with create
* operation of this database.
*
* @return the sourceDatabaseId value.
*/
public String sourceDatabaseId() {
return this.sourceDatabaseId;
}
/**
* Set the sourceDatabaseId property: The resource identifier of the source database associated with create
* operation of this database.
*
* @param sourceDatabaseId the sourceDatabaseId value to set.
* @return the DatabaseProperties object itself.
*/
public DatabaseProperties withSourceDatabaseId(String sourceDatabaseId) {
this.sourceDatabaseId = sourceDatabaseId;
return this;
}
/**
* Get the status property: The status of the database.
*
* @return the status value.
*/
public DatabaseStatus status() {
return this.status;
}
/**
* Get the databaseId property: The ID of the database.
*
* @return the databaseId value.
*/
public UUID databaseId() {
return this.databaseId;
}
/**
* Get the creationDate property: The creation date of the database (ISO8601 format).
*
* @return the creationDate value.
*/
public OffsetDateTime creationDate() {
return this.creationDate;
}
/**
* Get the currentServiceObjectiveName property: The current service level objective name of the database.
*
* @return the currentServiceObjectiveName value.
*/
public String currentServiceObjectiveName() {
return this.currentServiceObjectiveName;
}
/**
* Get the requestedServiceObjectiveName property: The requested service level objective name of the database.
*
* @return the requestedServiceObjectiveName value.
*/
public String requestedServiceObjectiveName() {
return this.requestedServiceObjectiveName;
}
/**
* Get the defaultSecondaryLocation property: The default secondary region for this database.
*
* @return the defaultSecondaryLocation value.
*/
public String defaultSecondaryLocation() {
return this.defaultSecondaryLocation;
}
/**
* Get the failoverGroupId property: Failover Group resource identifier that this database belongs to.
*
* @return the failoverGroupId value.
*/
public String failoverGroupId() {
return this.failoverGroupId;
}
/**
* Get the restorePointInTime property: Specifies the point in time (ISO8601 format) of the source database that
* will be restored to create the new database.
*
* @return the restorePointInTime value.
*/
public OffsetDateTime restorePointInTime() {
return this.restorePointInTime;
}
/**
* Set the restorePointInTime property: Specifies the point in time (ISO8601 format) of the source database that
* will be restored to create the new database.
*
* @param restorePointInTime the restorePointInTime value to set.
* @return the DatabaseProperties object itself.
*/
public DatabaseProperties withRestorePointInTime(OffsetDateTime restorePointInTime) {
this.restorePointInTime = restorePointInTime;
return this;
}
/**
* Get the sourceDatabaseDeletionDate property: Specifies the time that the database was deleted.
*
* @return the sourceDatabaseDeletionDate value.
*/
public OffsetDateTime sourceDatabaseDeletionDate() {
return this.sourceDatabaseDeletionDate;
}
/**
* Set the sourceDatabaseDeletionDate property: Specifies the time that the database was deleted.
*
* @param sourceDatabaseDeletionDate the sourceDatabaseDeletionDate value to set.
* @return the DatabaseProperties object itself.
*/
public DatabaseProperties withSourceDatabaseDeletionDate(OffsetDateTime sourceDatabaseDeletionDate) {
this.sourceDatabaseDeletionDate = sourceDatabaseDeletionDate;
return this;
}
/**
* Get the recoveryServicesRecoveryPointId property: The resource identifier of the recovery point associated with
* create operation of this database.
*
* @return the recoveryServicesRecoveryPointId value.
*/
public String recoveryServicesRecoveryPointId() {
return this.recoveryServicesRecoveryPointId;
}
/**
* Set the recoveryServicesRecoveryPointId property: The resource identifier of the recovery point associated with
* create operation of this database.
*
* @param recoveryServicesRecoveryPointId the recoveryServicesRecoveryPointId value to set.
* @return the DatabaseProperties object itself.
*/
public DatabaseProperties withRecoveryServicesRecoveryPointId(String recoveryServicesRecoveryPointId) {
this.recoveryServicesRecoveryPointId = recoveryServicesRecoveryPointId;
return this;
}
/**
* Get the longTermRetentionBackupResourceId property: The resource identifier of the long term retention backup
* associated with create operation of this database.
*
* @return the longTermRetentionBackupResourceId value.
*/
public String longTermRetentionBackupResourceId() {
return this.longTermRetentionBackupResourceId;
}
/**
* Set the longTermRetentionBackupResourceId property: The resource identifier of the long term retention backup
* associated with create operation of this database.
*
* @param longTermRetentionBackupResourceId the longTermRetentionBackupResourceId value to set.
* @return the DatabaseProperties object itself.
*/
public DatabaseProperties withLongTermRetentionBackupResourceId(String longTermRetentionBackupResourceId) {
this.longTermRetentionBackupResourceId = longTermRetentionBackupResourceId;
return this;
}
/**
* Get the recoverableDatabaseId property: The resource identifier of the recoverable database associated with
* create operation of this database.
*
* @return the recoverableDatabaseId value.
*/
public String recoverableDatabaseId() {
return this.recoverableDatabaseId;
}
/**
* Set the recoverableDatabaseId property: The resource identifier of the recoverable database associated with
* create operation of this database.
*
* @param recoverableDatabaseId the recoverableDatabaseId value to set.
* @return the DatabaseProperties object itself.
*/
public DatabaseProperties withRecoverableDatabaseId(String recoverableDatabaseId) {
this.recoverableDatabaseId = recoverableDatabaseId;
return this;
}
/**
* Get the restorableDroppedDatabaseId property: The resource identifier of the restorable dropped database
* associated with create operation of this database.
*
* @return the restorableDroppedDatabaseId value.
*/
public String restorableDroppedDatabaseId() {
return this.restorableDroppedDatabaseId;
}
/**
* Set the restorableDroppedDatabaseId property: The resource identifier of the restorable dropped database
* associated with create operation of this database.
*
* @param restorableDroppedDatabaseId the restorableDroppedDatabaseId value to set.
* @return the DatabaseProperties object itself.
*/
public DatabaseProperties withRestorableDroppedDatabaseId(String restorableDroppedDatabaseId) {
this.restorableDroppedDatabaseId = restorableDroppedDatabaseId;
return this;
}
/**
* Get the catalogCollation property: Collation of the metadata catalog.
*
* @return the catalogCollation value.
*/
public CatalogCollationType catalogCollation() {
return this.catalogCollation;
}
/**
* Set the catalogCollation property: Collation of the metadata catalog.
*
* @param catalogCollation the catalogCollation value to set.
* @return the DatabaseProperties object itself.
*/
public DatabaseProperties withCatalogCollation(CatalogCollationType catalogCollation) {
this.catalogCollation = catalogCollation;
return this;
}
/**
* Get the zoneRedundant property: Whether or not this database is zone redundant, which means the replicas of this
* database will be spread across multiple availability zones.
*
* @return the zoneRedundant value.
*/
public Boolean zoneRedundant() {
return this.zoneRedundant;
}
/**
* Set the zoneRedundant property: Whether or not this database is zone redundant, which means the replicas of this
* database will be spread across multiple availability zones.
*
* @param zoneRedundant the zoneRedundant value to set.
* @return the DatabaseProperties object itself.
*/
public DatabaseProperties withZoneRedundant(Boolean zoneRedundant) {
this.zoneRedundant = zoneRedundant;
return this;
}
/**
* Get the licenseType property: The license type to apply for this database. `LicenseIncluded` if you need a
* license, or `BasePrice` if you have a license and are eligible for the Azure Hybrid Benefit.
*
* @return the licenseType value.
*/
public DatabaseLicenseType licenseType() {
return this.licenseType;
}
/**
* Set the licenseType property: The license type to apply for this database. `LicenseIncluded` if you need a
* license, or `BasePrice` if you have a license and are eligible for the Azure Hybrid Benefit.
*
* @param licenseType the licenseType value to set.
* @return the DatabaseProperties object itself.
*/
public DatabaseProperties withLicenseType(DatabaseLicenseType licenseType) {
this.licenseType = licenseType;
return this;
}
/**
* Get the maxLogSizeBytes property: The max log size for this database.
*
* @return the maxLogSizeBytes value.
*/
public Long maxLogSizeBytes() {
return this.maxLogSizeBytes;
}
/**
* Get the earliestRestoreDate property: This records the earliest start date and time that restore is available for
* this database (ISO8601 format).
*
* @return the earliestRestoreDate value.
*/
public OffsetDateTime earliestRestoreDate() {
return this.earliestRestoreDate;
}
/**
* Get the readScale property: The state of read-only routing. If enabled, connections that have application intent
* set to readonly in their connection string may be routed to a readonly secondary replica in the same region. Not
* applicable to a Hyperscale database within an elastic pool.
*
* @return the readScale value.
*/
public DatabaseReadScale readScale() {
return this.readScale;
}
/**
* Set the readScale property: The state of read-only routing. If enabled, connections that have application intent
* set to readonly in their connection string may be routed to a readonly secondary replica in the same region. Not
* applicable to a Hyperscale database within an elastic pool.
*
* @param readScale the readScale value to set.
* @return the DatabaseProperties object itself.
*/
public DatabaseProperties withReadScale(DatabaseReadScale readScale) {
this.readScale = readScale;
return this;
}
/**
* Get the highAvailabilityReplicaCount property: The number of secondary replicas associated with the database that
* are used to provide high availability. Not applicable to a Hyperscale database within an elastic pool.
*
* @return the highAvailabilityReplicaCount value.
*/
public Integer highAvailabilityReplicaCount() {
return this.highAvailabilityReplicaCount;
}
/**
* Set the highAvailabilityReplicaCount property: The number of secondary replicas associated with the database that
* are used to provide high availability. Not applicable to a Hyperscale database within an elastic pool.
*
* @param highAvailabilityReplicaCount the highAvailabilityReplicaCount value to set.
* @return the DatabaseProperties object itself.
*/
public DatabaseProperties withHighAvailabilityReplicaCount(Integer highAvailabilityReplicaCount) {
this.highAvailabilityReplicaCount = highAvailabilityReplicaCount;
return this;
}
/**
* Get the secondaryType property: The secondary type of the database if it is a secondary. Valid values are Geo and
* Named.
*
* @return the secondaryType value.
*/
public SecondaryType secondaryType() {
return this.secondaryType;
}
/**
* Set the secondaryType property: The secondary type of the database if it is a secondary. Valid values are Geo and
* Named.
*
* @param secondaryType the secondaryType value to set.
* @return the DatabaseProperties object itself.
*/
public DatabaseProperties withSecondaryType(SecondaryType secondaryType) {
this.secondaryType = secondaryType;
return this;
}
/**
* Get the currentSku property: The name and tier of the SKU.
*
* @return the currentSku value.
*/
public Sku currentSku() {
return this.currentSku;
}
/**
* Get the autoPauseDelay property: Time in minutes after which database is automatically paused. A value of -1
* means that automatic pause is disabled.
*
* @return the autoPauseDelay value.
*/
public Integer autoPauseDelay() {
return this.autoPauseDelay;
}
/**
* Set the autoPauseDelay property: Time in minutes after which database is automatically paused. A value of -1
* means that automatic pause is disabled.
*
* @param autoPauseDelay the autoPauseDelay value to set.
* @return the DatabaseProperties object itself.
*/
public DatabaseProperties withAutoPauseDelay(Integer autoPauseDelay) {
this.autoPauseDelay = autoPauseDelay;
return this;
}
/**
* Get the currentBackupStorageRedundancy property: The storage account type used to store backups for this
* database.
*
* @return the currentBackupStorageRedundancy value.
*/
public BackupStorageRedundancy currentBackupStorageRedundancy() {
return this.currentBackupStorageRedundancy;
}
/**
* Get the requestedBackupStorageRedundancy property: The storage account type to be used to store backups for this
* database.
*
* @return the requestedBackupStorageRedundancy value.
*/
public BackupStorageRedundancy requestedBackupStorageRedundancy() {
return this.requestedBackupStorageRedundancy;
}
/**
* Set the requestedBackupStorageRedundancy property: The storage account type to be used to store backups for this
* database.
*
* @param requestedBackupStorageRedundancy the requestedBackupStorageRedundancy value to set.
* @return the DatabaseProperties object itself.
*/
public DatabaseProperties
withRequestedBackupStorageRedundancy(BackupStorageRedundancy requestedBackupStorageRedundancy) {
this.requestedBackupStorageRedundancy = requestedBackupStorageRedundancy;
return this;
}
/**
* Get the minCapacity property: Minimal capacity that database will always have allocated, if not paused.
*
* @return the minCapacity value.
*/
public Double minCapacity() {
return this.minCapacity;
}
/**
* Set the minCapacity property: Minimal capacity that database will always have allocated, if not paused.
*
* @param minCapacity the minCapacity value to set.
* @return the DatabaseProperties object itself.
*/
public DatabaseProperties withMinCapacity(Double minCapacity) {
this.minCapacity = minCapacity;
return this;
}
/**
* Get the pausedDate property: The date when database was paused by user configuration or action(ISO8601 format).
* Null if the database is ready.
*
* @return the pausedDate value.
*/
public OffsetDateTime pausedDate() {
return this.pausedDate;
}
/**
* Get the resumedDate property: The date when database was resumed by user action or database login (ISO8601
* format). Null if the database is paused.
*
* @return the resumedDate value.
*/
public OffsetDateTime resumedDate() {
return this.resumedDate;
}
/**
* Get the maintenanceConfigurationId property: Maintenance configuration id assigned to the database. This
* configuration defines the period when the maintenance updates will occur.
*
* @return the maintenanceConfigurationId value.
*/
public String maintenanceConfigurationId() {
return this.maintenanceConfigurationId;
}
/**
* Set the maintenanceConfigurationId property: Maintenance configuration id assigned to the database. This
* configuration defines the period when the maintenance updates will occur.
*
* @param maintenanceConfigurationId the maintenanceConfigurationId value to set.
* @return the DatabaseProperties object itself.
*/
public DatabaseProperties withMaintenanceConfigurationId(String maintenanceConfigurationId) {
this.maintenanceConfigurationId = maintenanceConfigurationId;
return this;
}
/**
* Get the isLedgerOn property: Whether or not this database is a ledger database, which means all tables in the
* database are ledger tables. Note: the value of this property cannot be changed after the database has been
* created.
*
* @return the isLedgerOn value.
*/
public Boolean isLedgerOn() {
return this.isLedgerOn;
}
/**
* Set the isLedgerOn property: Whether or not this database is a ledger database, which means all tables in the
* database are ledger tables. Note: the value of this property cannot be changed after the database has been
* created.
*
* @param isLedgerOn the isLedgerOn value to set.
* @return the DatabaseProperties object itself.
*/
public DatabaseProperties withIsLedgerOn(Boolean isLedgerOn) {
this.isLedgerOn = isLedgerOn;
return this;
}
/**
* Get the isInfraEncryptionEnabled property: Infra encryption is enabled for this database.
*
* @return the isInfraEncryptionEnabled value.
*/
public Boolean isInfraEncryptionEnabled() {
return this.isInfraEncryptionEnabled;
}
/**
* Get the federatedClientId property: The Client id used for cross tenant per database CMK scenario.
*
* @return the federatedClientId value.
*/
public UUID federatedClientId() {
return this.federatedClientId;
}
/**
* Set the federatedClientId property: The Client id used for cross tenant per database CMK scenario.
*
* @param federatedClientId the federatedClientId value to set.
* @return the DatabaseProperties object itself.
*/
public DatabaseProperties withFederatedClientId(UUID federatedClientId) {
this.federatedClientId = federatedClientId;
return this;
}
/**
* Get the sourceResourceId property: The resource identifier of the source associated with the create operation of
* this database.
*
* This property is only supported for DataWarehouse edition and allows to restore across subscriptions.
*
* When sourceResourceId is specified, sourceDatabaseId, recoverableDatabaseId, restorableDroppedDatabaseId and
* sourceDatabaseDeletionDate must not be specified and CreateMode must be PointInTimeRestore, Restore or Recover.
*
* When createMode is PointInTimeRestore, sourceResourceId must be the resource ID of the existing database or
* existing sql pool, and restorePointInTime must be specified.
*
* When createMode is Restore, sourceResourceId must be the resource ID of restorable dropped database or restorable
* dropped sql pool.
*
* When createMode is Recover, sourceResourceId must be the resource ID of recoverable database or recoverable sql
* pool.
*
* When source subscription belongs to a different tenant than target subscription, “x-ms-authorization-auxiliary”
* header must contain authentication token for the source tenant. For more details about
* “x-ms-authorization-auxiliary” header see
* https://docs.microsoft.com/en-us/azure/azure-resource-manager/management/authenticate-multi-tenant.
*
* @return the sourceResourceId value.
*/
public String sourceResourceId() {
return this.sourceResourceId;
}
/**
* Set the sourceResourceId property: The resource identifier of the source associated with the create operation of
* this database.
*
* This property is only supported for DataWarehouse edition and allows to restore across subscriptions.
*
* When sourceResourceId is specified, sourceDatabaseId, recoverableDatabaseId, restorableDroppedDatabaseId and
* sourceDatabaseDeletionDate must not be specified and CreateMode must be PointInTimeRestore, Restore or Recover.
*
* When createMode is PointInTimeRestore, sourceResourceId must be the resource ID of the existing database or
* existing sql pool, and restorePointInTime must be specified.
*
* When createMode is Restore, sourceResourceId must be the resource ID of restorable dropped database or restorable
* dropped sql pool.
*
* When createMode is Recover, sourceResourceId must be the resource ID of recoverable database or recoverable sql
* pool.
*
* When source subscription belongs to a different tenant than target subscription, “x-ms-authorization-auxiliary”
* header must contain authentication token for the source tenant. For more details about
* “x-ms-authorization-auxiliary” header see
* https://docs.microsoft.com/en-us/azure/azure-resource-manager/management/authenticate-multi-tenant.
*
* @param sourceResourceId the sourceResourceId value to set.
* @return the DatabaseProperties object itself.
*/
public DatabaseProperties withSourceResourceId(String sourceResourceId) {
this.sourceResourceId = sourceResourceId;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (currentSku() != null) {
currentSku().validate();
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("createMode", this.createMode == null ? null : this.createMode.toString());
jsonWriter.writeStringField("collation", this.collation);
jsonWriter.writeNumberField("maxSizeBytes", this.maxSizeBytes);
jsonWriter.writeStringField("sampleName", this.sampleName == null ? null : this.sampleName.toString());
jsonWriter.writeStringField("elasticPoolId", this.elasticPoolId);
jsonWriter.writeStringField("sourceDatabaseId", this.sourceDatabaseId);
jsonWriter.writeStringField("restorePointInTime",
this.restorePointInTime == null
? null
: DateTimeFormatter.ISO_OFFSET_DATE_TIME.format(this.restorePointInTime));
jsonWriter.writeStringField("sourceDatabaseDeletionDate",
this.sourceDatabaseDeletionDate == null
? null
: DateTimeFormatter.ISO_OFFSET_DATE_TIME.format(this.sourceDatabaseDeletionDate));
jsonWriter.writeStringField("recoveryServicesRecoveryPointId", this.recoveryServicesRecoveryPointId);
jsonWriter.writeStringField("longTermRetentionBackupResourceId", this.longTermRetentionBackupResourceId);
jsonWriter.writeStringField("recoverableDatabaseId", this.recoverableDatabaseId);
jsonWriter.writeStringField("restorableDroppedDatabaseId", this.restorableDroppedDatabaseId);
jsonWriter.writeStringField("catalogCollation",
this.catalogCollation == null ? null : this.catalogCollation.toString());
jsonWriter.writeBooleanField("zoneRedundant", this.zoneRedundant);
jsonWriter.writeStringField("licenseType", this.licenseType == null ? null : this.licenseType.toString());
jsonWriter.writeStringField("readScale", this.readScale == null ? null : this.readScale.toString());
jsonWriter.writeNumberField("highAvailabilityReplicaCount", this.highAvailabilityReplicaCount);
jsonWriter.writeStringField("secondaryType", this.secondaryType == null ? null : this.secondaryType.toString());
jsonWriter.writeNumberField("autoPauseDelay", this.autoPauseDelay);
jsonWriter.writeStringField("requestedBackupStorageRedundancy",
this.requestedBackupStorageRedundancy == null ? null : this.requestedBackupStorageRedundancy.toString());
jsonWriter.writeNumberField("minCapacity", this.minCapacity);
jsonWriter.writeStringField("maintenanceConfigurationId", this.maintenanceConfigurationId);
jsonWriter.writeBooleanField("isLedgerOn", this.isLedgerOn);
jsonWriter.writeStringField("federatedClientId", Objects.toString(this.federatedClientId, null));
jsonWriter.writeStringField("sourceResourceId", this.sourceResourceId);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of DatabaseProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of DatabaseProperties if the JsonReader was pointing to an instance of it, or null if it was
* pointing to JSON null.
* @throws IOException If an error occurs while reading the DatabaseProperties.
*/
public static DatabaseProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
DatabaseProperties deserializedDatabaseProperties = new DatabaseProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("createMode".equals(fieldName)) {
deserializedDatabaseProperties.createMode = CreateMode.fromString(reader.getString());
} else if ("collation".equals(fieldName)) {
deserializedDatabaseProperties.collation = reader.getString();
} else if ("maxSizeBytes".equals(fieldName)) {
deserializedDatabaseProperties.maxSizeBytes = reader.getNullable(JsonReader::getLong);
} else if ("sampleName".equals(fieldName)) {
deserializedDatabaseProperties.sampleName = SampleName.fromString(reader.getString());
} else if ("elasticPoolId".equals(fieldName)) {
deserializedDatabaseProperties.elasticPoolId = reader.getString();
} else if ("sourceDatabaseId".equals(fieldName)) {
deserializedDatabaseProperties.sourceDatabaseId = reader.getString();
} else if ("status".equals(fieldName)) {
deserializedDatabaseProperties.status = DatabaseStatus.fromString(reader.getString());
} else if ("databaseId".equals(fieldName)) {
deserializedDatabaseProperties.databaseId
= reader.getNullable(nonNullReader -> UUID.fromString(nonNullReader.getString()));
} else if ("creationDate".equals(fieldName)) {
deserializedDatabaseProperties.creationDate = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("currentServiceObjectiveName".equals(fieldName)) {
deserializedDatabaseProperties.currentServiceObjectiveName = reader.getString();
} else if ("requestedServiceObjectiveName".equals(fieldName)) {
deserializedDatabaseProperties.requestedServiceObjectiveName = reader.getString();
} else if ("defaultSecondaryLocation".equals(fieldName)) {
deserializedDatabaseProperties.defaultSecondaryLocation = reader.getString();
} else if ("failoverGroupId".equals(fieldName)) {
deserializedDatabaseProperties.failoverGroupId = reader.getString();
} else if ("restorePointInTime".equals(fieldName)) {
deserializedDatabaseProperties.restorePointInTime = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("sourceDatabaseDeletionDate".equals(fieldName)) {
deserializedDatabaseProperties.sourceDatabaseDeletionDate = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("recoveryServicesRecoveryPointId".equals(fieldName)) {
deserializedDatabaseProperties.recoveryServicesRecoveryPointId = reader.getString();
} else if ("longTermRetentionBackupResourceId".equals(fieldName)) {
deserializedDatabaseProperties.longTermRetentionBackupResourceId = reader.getString();
} else if ("recoverableDatabaseId".equals(fieldName)) {
deserializedDatabaseProperties.recoverableDatabaseId = reader.getString();
} else if ("restorableDroppedDatabaseId".equals(fieldName)) {
deserializedDatabaseProperties.restorableDroppedDatabaseId = reader.getString();
} else if ("catalogCollation".equals(fieldName)) {
deserializedDatabaseProperties.catalogCollation
= CatalogCollationType.fromString(reader.getString());
} else if ("zoneRedundant".equals(fieldName)) {
deserializedDatabaseProperties.zoneRedundant = reader.getNullable(JsonReader::getBoolean);
} else if ("licenseType".equals(fieldName)) {
deserializedDatabaseProperties.licenseType = DatabaseLicenseType.fromString(reader.getString());
} else if ("maxLogSizeBytes".equals(fieldName)) {
deserializedDatabaseProperties.maxLogSizeBytes = reader.getNullable(JsonReader::getLong);
} else if ("earliestRestoreDate".equals(fieldName)) {
deserializedDatabaseProperties.earliestRestoreDate = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("readScale".equals(fieldName)) {
deserializedDatabaseProperties.readScale = DatabaseReadScale.fromString(reader.getString());
} else if ("highAvailabilityReplicaCount".equals(fieldName)) {
deserializedDatabaseProperties.highAvailabilityReplicaCount
= reader.getNullable(JsonReader::getInt);
} else if ("secondaryType".equals(fieldName)) {
deserializedDatabaseProperties.secondaryType = SecondaryType.fromString(reader.getString());
} else if ("currentSku".equals(fieldName)) {
deserializedDatabaseProperties.currentSku = Sku.fromJson(reader);
} else if ("autoPauseDelay".equals(fieldName)) {
deserializedDatabaseProperties.autoPauseDelay = reader.getNullable(JsonReader::getInt);
} else if ("currentBackupStorageRedundancy".equals(fieldName)) {
deserializedDatabaseProperties.currentBackupStorageRedundancy
= BackupStorageRedundancy.fromString(reader.getString());
} else if ("requestedBackupStorageRedundancy".equals(fieldName)) {
deserializedDatabaseProperties.requestedBackupStorageRedundancy
= BackupStorageRedundancy.fromString(reader.getString());
} else if ("minCapacity".equals(fieldName)) {
deserializedDatabaseProperties.minCapacity = reader.getNullable(JsonReader::getDouble);
} else if ("pausedDate".equals(fieldName)) {
deserializedDatabaseProperties.pausedDate = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("resumedDate".equals(fieldName)) {
deserializedDatabaseProperties.resumedDate = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("maintenanceConfigurationId".equals(fieldName)) {
deserializedDatabaseProperties.maintenanceConfigurationId = reader.getString();
} else if ("isLedgerOn".equals(fieldName)) {
deserializedDatabaseProperties.isLedgerOn = reader.getNullable(JsonReader::getBoolean);
} else if ("isInfraEncryptionEnabled".equals(fieldName)) {
deserializedDatabaseProperties.isInfraEncryptionEnabled
= reader.getNullable(JsonReader::getBoolean);
} else if ("federatedClientId".equals(fieldName)) {
deserializedDatabaseProperties.federatedClientId
= reader.getNullable(nonNullReader -> UUID.fromString(nonNullReader.getString()));
} else if ("sourceResourceId".equals(fieldName)) {
deserializedDatabaseProperties.sourceResourceId = reader.getString();
} else {
reader.skipChildren();
}
}
return deserializedDatabaseProperties;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy