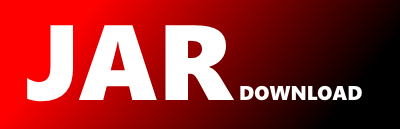
com.azure.resourcemanager.sql.fluent.models.ElasticPoolUpdateProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-sql Show documentation
Show all versions of azure-resourcemanager-sql Show documentation
This package contains Microsoft Azure Sql Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.sql.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.sql.models.ElasticPoolLicenseType;
import com.azure.resourcemanager.sql.models.ElasticPoolPerDatabaseSettings;
import java.io.IOException;
/**
* Properties of an elastic pool.
*/
@Fluent
public final class ElasticPoolUpdateProperties implements JsonSerializable {
/*
* The storage limit for the database elastic pool in bytes.
*/
private Long maxSizeBytes;
/*
* Minimal capacity that serverless pool will not shrink below, if not paused
*/
private Double minCapacity;
/*
* The per database settings for the elastic pool.
*/
private ElasticPoolPerDatabaseSettings perDatabaseSettings;
/*
* Whether or not this elastic pool is zone redundant, which means the replicas of this elastic pool will be spread
* across multiple availability zones.
*/
private Boolean zoneRedundant;
/*
* The license type to apply for this elastic pool.
*/
private ElasticPoolLicenseType licenseType;
/*
* Maintenance configuration id assigned to the elastic pool. This configuration defines the period when the
* maintenance updates will will occur.
*/
private String maintenanceConfigurationId;
/*
* The number of secondary replicas associated with the elastic pool that are used to provide high availability.
* Applicable only to Hyperscale elastic pools.
*/
private Integer highAvailabilityReplicaCount;
/**
* Creates an instance of ElasticPoolUpdateProperties class.
*/
public ElasticPoolUpdateProperties() {
}
/**
* Get the maxSizeBytes property: The storage limit for the database elastic pool in bytes.
*
* @return the maxSizeBytes value.
*/
public Long maxSizeBytes() {
return this.maxSizeBytes;
}
/**
* Set the maxSizeBytes property: The storage limit for the database elastic pool in bytes.
*
* @param maxSizeBytes the maxSizeBytes value to set.
* @return the ElasticPoolUpdateProperties object itself.
*/
public ElasticPoolUpdateProperties withMaxSizeBytes(Long maxSizeBytes) {
this.maxSizeBytes = maxSizeBytes;
return this;
}
/**
* Get the minCapacity property: Minimal capacity that serverless pool will not shrink below, if not paused.
*
* @return the minCapacity value.
*/
public Double minCapacity() {
return this.minCapacity;
}
/**
* Set the minCapacity property: Minimal capacity that serverless pool will not shrink below, if not paused.
*
* @param minCapacity the minCapacity value to set.
* @return the ElasticPoolUpdateProperties object itself.
*/
public ElasticPoolUpdateProperties withMinCapacity(Double minCapacity) {
this.minCapacity = minCapacity;
return this;
}
/**
* Get the perDatabaseSettings property: The per database settings for the elastic pool.
*
* @return the perDatabaseSettings value.
*/
public ElasticPoolPerDatabaseSettings perDatabaseSettings() {
return this.perDatabaseSettings;
}
/**
* Set the perDatabaseSettings property: The per database settings for the elastic pool.
*
* @param perDatabaseSettings the perDatabaseSettings value to set.
* @return the ElasticPoolUpdateProperties object itself.
*/
public ElasticPoolUpdateProperties withPerDatabaseSettings(ElasticPoolPerDatabaseSettings perDatabaseSettings) {
this.perDatabaseSettings = perDatabaseSettings;
return this;
}
/**
* Get the zoneRedundant property: Whether or not this elastic pool is zone redundant, which means the replicas of
* this elastic pool will be spread across multiple availability zones.
*
* @return the zoneRedundant value.
*/
public Boolean zoneRedundant() {
return this.zoneRedundant;
}
/**
* Set the zoneRedundant property: Whether or not this elastic pool is zone redundant, which means the replicas of
* this elastic pool will be spread across multiple availability zones.
*
* @param zoneRedundant the zoneRedundant value to set.
* @return the ElasticPoolUpdateProperties object itself.
*/
public ElasticPoolUpdateProperties withZoneRedundant(Boolean zoneRedundant) {
this.zoneRedundant = zoneRedundant;
return this;
}
/**
* Get the licenseType property: The license type to apply for this elastic pool.
*
* @return the licenseType value.
*/
public ElasticPoolLicenseType licenseType() {
return this.licenseType;
}
/**
* Set the licenseType property: The license type to apply for this elastic pool.
*
* @param licenseType the licenseType value to set.
* @return the ElasticPoolUpdateProperties object itself.
*/
public ElasticPoolUpdateProperties withLicenseType(ElasticPoolLicenseType licenseType) {
this.licenseType = licenseType;
return this;
}
/**
* Get the maintenanceConfigurationId property: Maintenance configuration id assigned to the elastic pool. This
* configuration defines the period when the maintenance updates will will occur.
*
* @return the maintenanceConfigurationId value.
*/
public String maintenanceConfigurationId() {
return this.maintenanceConfigurationId;
}
/**
* Set the maintenanceConfigurationId property: Maintenance configuration id assigned to the elastic pool. This
* configuration defines the period when the maintenance updates will will occur.
*
* @param maintenanceConfigurationId the maintenanceConfigurationId value to set.
* @return the ElasticPoolUpdateProperties object itself.
*/
public ElasticPoolUpdateProperties withMaintenanceConfigurationId(String maintenanceConfigurationId) {
this.maintenanceConfigurationId = maintenanceConfigurationId;
return this;
}
/**
* Get the highAvailabilityReplicaCount property: The number of secondary replicas associated with the elastic pool
* that are used to provide high availability. Applicable only to Hyperscale elastic pools.
*
* @return the highAvailabilityReplicaCount value.
*/
public Integer highAvailabilityReplicaCount() {
return this.highAvailabilityReplicaCount;
}
/**
* Set the highAvailabilityReplicaCount property: The number of secondary replicas associated with the elastic pool
* that are used to provide high availability. Applicable only to Hyperscale elastic pools.
*
* @param highAvailabilityReplicaCount the highAvailabilityReplicaCount value to set.
* @return the ElasticPoolUpdateProperties object itself.
*/
public ElasticPoolUpdateProperties withHighAvailabilityReplicaCount(Integer highAvailabilityReplicaCount) {
this.highAvailabilityReplicaCount = highAvailabilityReplicaCount;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (perDatabaseSettings() != null) {
perDatabaseSettings().validate();
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeNumberField("maxSizeBytes", this.maxSizeBytes);
jsonWriter.writeNumberField("minCapacity", this.minCapacity);
jsonWriter.writeJsonField("perDatabaseSettings", this.perDatabaseSettings);
jsonWriter.writeBooleanField("zoneRedundant", this.zoneRedundant);
jsonWriter.writeStringField("licenseType", this.licenseType == null ? null : this.licenseType.toString());
jsonWriter.writeStringField("maintenanceConfigurationId", this.maintenanceConfigurationId);
jsonWriter.writeNumberField("highAvailabilityReplicaCount", this.highAvailabilityReplicaCount);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of ElasticPoolUpdateProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of ElasticPoolUpdateProperties if the JsonReader was pointing to an instance of it, or null
* if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the ElasticPoolUpdateProperties.
*/
public static ElasticPoolUpdateProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
ElasticPoolUpdateProperties deserializedElasticPoolUpdateProperties = new ElasticPoolUpdateProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("maxSizeBytes".equals(fieldName)) {
deserializedElasticPoolUpdateProperties.maxSizeBytes = reader.getNullable(JsonReader::getLong);
} else if ("minCapacity".equals(fieldName)) {
deserializedElasticPoolUpdateProperties.minCapacity = reader.getNullable(JsonReader::getDouble);
} else if ("perDatabaseSettings".equals(fieldName)) {
deserializedElasticPoolUpdateProperties.perDatabaseSettings
= ElasticPoolPerDatabaseSettings.fromJson(reader);
} else if ("zoneRedundant".equals(fieldName)) {
deserializedElasticPoolUpdateProperties.zoneRedundant = reader.getNullable(JsonReader::getBoolean);
} else if ("licenseType".equals(fieldName)) {
deserializedElasticPoolUpdateProperties.licenseType
= ElasticPoolLicenseType.fromString(reader.getString());
} else if ("maintenanceConfigurationId".equals(fieldName)) {
deserializedElasticPoolUpdateProperties.maintenanceConfigurationId = reader.getString();
} else if ("highAvailabilityReplicaCount".equals(fieldName)) {
deserializedElasticPoolUpdateProperties.highAvailabilityReplicaCount
= reader.getNullable(JsonReader::getInt);
} else {
reader.skipChildren();
}
}
return deserializedElasticPoolUpdateProperties;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy