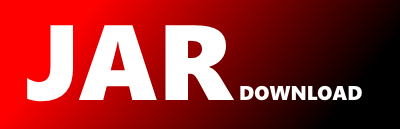
com.azure.resourcemanager.sql.fluent.models.JobExecutionProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-sql Show documentation
Show all versions of azure-resourcemanager-sql Show documentation
This package contains Microsoft Azure Sql Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.sql.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.CoreUtils;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.sql.models.JobExecutionLifecycle;
import com.azure.resourcemanager.sql.models.JobExecutionTarget;
import com.azure.resourcemanager.sql.models.ProvisioningState;
import java.io.IOException;
import java.time.OffsetDateTime;
import java.util.UUID;
/**
* Properties for an Azure SQL Database Elastic job execution.
*/
@Fluent
public final class JobExecutionProperties implements JsonSerializable {
/*
* The job version number.
*/
private Integer jobVersion;
/*
* The job step name.
*/
private String stepName;
/*
* The job step id.
*/
private Integer stepId;
/*
* The unique identifier of the job execution.
*/
private UUID jobExecutionId;
/*
* The detailed state of the job execution.
*/
private JobExecutionLifecycle lifecycle;
/*
* The ARM provisioning state of the job execution.
*/
private ProvisioningState provisioningState;
/*
* The time that the job execution was created.
*/
private OffsetDateTime createTime;
/*
* The time that the job execution started.
*/
private OffsetDateTime startTime;
/*
* The time that the job execution completed.
*/
private OffsetDateTime endTime;
/*
* Number of times the job execution has been attempted.
*/
private Integer currentAttempts;
/*
* Start time of the current attempt.
*/
private OffsetDateTime currentAttemptStartTime;
/*
* The last status or error message.
*/
private String lastMessage;
/*
* The target that this execution is executed on.
*/
private JobExecutionTarget target;
/**
* Creates an instance of JobExecutionProperties class.
*/
public JobExecutionProperties() {
}
/**
* Get the jobVersion property: The job version number.
*
* @return the jobVersion value.
*/
public Integer jobVersion() {
return this.jobVersion;
}
/**
* Get the stepName property: The job step name.
*
* @return the stepName value.
*/
public String stepName() {
return this.stepName;
}
/**
* Get the stepId property: The job step id.
*
* @return the stepId value.
*/
public Integer stepId() {
return this.stepId;
}
/**
* Get the jobExecutionId property: The unique identifier of the job execution.
*
* @return the jobExecutionId value.
*/
public UUID jobExecutionId() {
return this.jobExecutionId;
}
/**
* Get the lifecycle property: The detailed state of the job execution.
*
* @return the lifecycle value.
*/
public JobExecutionLifecycle lifecycle() {
return this.lifecycle;
}
/**
* Get the provisioningState property: The ARM provisioning state of the job execution.
*
* @return the provisioningState value.
*/
public ProvisioningState provisioningState() {
return this.provisioningState;
}
/**
* Get the createTime property: The time that the job execution was created.
*
* @return the createTime value.
*/
public OffsetDateTime createTime() {
return this.createTime;
}
/**
* Get the startTime property: The time that the job execution started.
*
* @return the startTime value.
*/
public OffsetDateTime startTime() {
return this.startTime;
}
/**
* Get the endTime property: The time that the job execution completed.
*
* @return the endTime value.
*/
public OffsetDateTime endTime() {
return this.endTime;
}
/**
* Get the currentAttempts property: Number of times the job execution has been attempted.
*
* @return the currentAttempts value.
*/
public Integer currentAttempts() {
return this.currentAttempts;
}
/**
* Set the currentAttempts property: Number of times the job execution has been attempted.
*
* @param currentAttempts the currentAttempts value to set.
* @return the JobExecutionProperties object itself.
*/
public JobExecutionProperties withCurrentAttempts(Integer currentAttempts) {
this.currentAttempts = currentAttempts;
return this;
}
/**
* Get the currentAttemptStartTime property: Start time of the current attempt.
*
* @return the currentAttemptStartTime value.
*/
public OffsetDateTime currentAttemptStartTime() {
return this.currentAttemptStartTime;
}
/**
* Get the lastMessage property: The last status or error message.
*
* @return the lastMessage value.
*/
public String lastMessage() {
return this.lastMessage;
}
/**
* Get the target property: The target that this execution is executed on.
*
* @return the target value.
*/
public JobExecutionTarget target() {
return this.target;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (target() != null) {
target().validate();
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeNumberField("currentAttempts", this.currentAttempts);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of JobExecutionProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of JobExecutionProperties if the JsonReader was pointing to an instance of it, or null if it
* was pointing to JSON null.
* @throws IOException If an error occurs while reading the JobExecutionProperties.
*/
public static JobExecutionProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
JobExecutionProperties deserializedJobExecutionProperties = new JobExecutionProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("jobVersion".equals(fieldName)) {
deserializedJobExecutionProperties.jobVersion = reader.getNullable(JsonReader::getInt);
} else if ("stepName".equals(fieldName)) {
deserializedJobExecutionProperties.stepName = reader.getString();
} else if ("stepId".equals(fieldName)) {
deserializedJobExecutionProperties.stepId = reader.getNullable(JsonReader::getInt);
} else if ("jobExecutionId".equals(fieldName)) {
deserializedJobExecutionProperties.jobExecutionId
= reader.getNullable(nonNullReader -> UUID.fromString(nonNullReader.getString()));
} else if ("lifecycle".equals(fieldName)) {
deserializedJobExecutionProperties.lifecycle = JobExecutionLifecycle.fromString(reader.getString());
} else if ("provisioningState".equals(fieldName)) {
deserializedJobExecutionProperties.provisioningState
= ProvisioningState.fromString(reader.getString());
} else if ("createTime".equals(fieldName)) {
deserializedJobExecutionProperties.createTime = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("startTime".equals(fieldName)) {
deserializedJobExecutionProperties.startTime = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("endTime".equals(fieldName)) {
deserializedJobExecutionProperties.endTime = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("currentAttempts".equals(fieldName)) {
deserializedJobExecutionProperties.currentAttempts = reader.getNullable(JsonReader::getInt);
} else if ("currentAttemptStartTime".equals(fieldName)) {
deserializedJobExecutionProperties.currentAttemptStartTime = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("lastMessage".equals(fieldName)) {
deserializedJobExecutionProperties.lastMessage = reader.getString();
} else if ("target".equals(fieldName)) {
deserializedJobExecutionProperties.target = JobExecutionTarget.fromJson(reader);
} else {
reader.skipChildren();
}
}
return deserializedJobExecutionProperties;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy