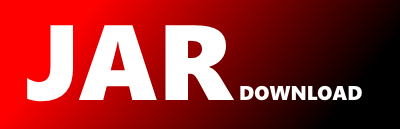
com.azure.resourcemanager.sql.fluent.models.LongTermRetentionBackupProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-sql Show documentation
Show all versions of azure-resourcemanager-sql Show documentation
This package contains Microsoft Azure Sql Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.sql.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.CoreUtils;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.sql.models.BackupStorageRedundancy;
import java.io.IOException;
import java.time.OffsetDateTime;
/**
* Properties of a long term retention backup.
*/
@Fluent
public final class LongTermRetentionBackupProperties implements JsonSerializable {
/*
* The server name that the backup database belong to.
*/
private String serverName;
/*
* The create time of the server.
*/
private OffsetDateTime serverCreateTime;
/*
* The name of the database the backup belong to
*/
private String databaseName;
/*
* The delete time of the database
*/
private OffsetDateTime databaseDeletionTime;
/*
* The time the backup was taken
*/
private OffsetDateTime backupTime;
/*
* The time the long term retention backup will expire.
*/
private OffsetDateTime backupExpirationTime;
/*
* The storage redundancy type of the backup
*/
private BackupStorageRedundancy backupStorageRedundancy;
/*
* The storage redundancy type of the backup
*/
private BackupStorageRedundancy requestedBackupStorageRedundancy;
/**
* Creates an instance of LongTermRetentionBackupProperties class.
*/
public LongTermRetentionBackupProperties() {
}
/**
* Get the serverName property: The server name that the backup database belong to.
*
* @return the serverName value.
*/
public String serverName() {
return this.serverName;
}
/**
* Get the serverCreateTime property: The create time of the server.
*
* @return the serverCreateTime value.
*/
public OffsetDateTime serverCreateTime() {
return this.serverCreateTime;
}
/**
* Get the databaseName property: The name of the database the backup belong to.
*
* @return the databaseName value.
*/
public String databaseName() {
return this.databaseName;
}
/**
* Get the databaseDeletionTime property: The delete time of the database.
*
* @return the databaseDeletionTime value.
*/
public OffsetDateTime databaseDeletionTime() {
return this.databaseDeletionTime;
}
/**
* Get the backupTime property: The time the backup was taken.
*
* @return the backupTime value.
*/
public OffsetDateTime backupTime() {
return this.backupTime;
}
/**
* Get the backupExpirationTime property: The time the long term retention backup will expire.
*
* @return the backupExpirationTime value.
*/
public OffsetDateTime backupExpirationTime() {
return this.backupExpirationTime;
}
/**
* Get the backupStorageRedundancy property: The storage redundancy type of the backup.
*
* @return the backupStorageRedundancy value.
*/
public BackupStorageRedundancy backupStorageRedundancy() {
return this.backupStorageRedundancy;
}
/**
* Get the requestedBackupStorageRedundancy property: The storage redundancy type of the backup.
*
* @return the requestedBackupStorageRedundancy value.
*/
public BackupStorageRedundancy requestedBackupStorageRedundancy() {
return this.requestedBackupStorageRedundancy;
}
/**
* Set the requestedBackupStorageRedundancy property: The storage redundancy type of the backup.
*
* @param requestedBackupStorageRedundancy the requestedBackupStorageRedundancy value to set.
* @return the LongTermRetentionBackupProperties object itself.
*/
public LongTermRetentionBackupProperties
withRequestedBackupStorageRedundancy(BackupStorageRedundancy requestedBackupStorageRedundancy) {
this.requestedBackupStorageRedundancy = requestedBackupStorageRedundancy;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("requestedBackupStorageRedundancy",
this.requestedBackupStorageRedundancy == null ? null : this.requestedBackupStorageRedundancy.toString());
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of LongTermRetentionBackupProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of LongTermRetentionBackupProperties if the JsonReader was pointing to an instance of it, or
* null if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the LongTermRetentionBackupProperties.
*/
public static LongTermRetentionBackupProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
LongTermRetentionBackupProperties deserializedLongTermRetentionBackupProperties
= new LongTermRetentionBackupProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("serverName".equals(fieldName)) {
deserializedLongTermRetentionBackupProperties.serverName = reader.getString();
} else if ("serverCreateTime".equals(fieldName)) {
deserializedLongTermRetentionBackupProperties.serverCreateTime = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("databaseName".equals(fieldName)) {
deserializedLongTermRetentionBackupProperties.databaseName = reader.getString();
} else if ("databaseDeletionTime".equals(fieldName)) {
deserializedLongTermRetentionBackupProperties.databaseDeletionTime = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("backupTime".equals(fieldName)) {
deserializedLongTermRetentionBackupProperties.backupTime = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("backupExpirationTime".equals(fieldName)) {
deserializedLongTermRetentionBackupProperties.backupExpirationTime = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("backupStorageRedundancy".equals(fieldName)) {
deserializedLongTermRetentionBackupProperties.backupStorageRedundancy
= BackupStorageRedundancy.fromString(reader.getString());
} else if ("requestedBackupStorageRedundancy".equals(fieldName)) {
deserializedLongTermRetentionBackupProperties.requestedBackupStorageRedundancy
= BackupStorageRedundancy.fromString(reader.getString());
} else {
reader.skipChildren();
}
}
return deserializedLongTermRetentionBackupProperties;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy