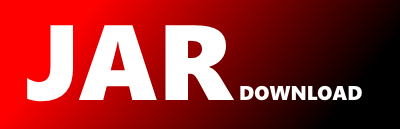
com.azure.resourcemanager.sql.fluent.models.ManagedDatabaseProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-sql Show documentation
Show all versions of azure-resourcemanager-sql Show documentation
This package contains Microsoft Azure Sql Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.sql.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.CoreUtils;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.sql.models.CatalogCollationType;
import com.azure.resourcemanager.sql.models.ManagedDatabaseCreateMode;
import com.azure.resourcemanager.sql.models.ManagedDatabaseStatus;
import java.io.IOException;
import java.time.OffsetDateTime;
import java.time.format.DateTimeFormatter;
/**
* The managed database's properties.
*/
@Fluent
public final class ManagedDatabaseProperties implements JsonSerializable {
/*
* Collation of the managed database.
*/
private String collation;
/*
* Status of the database.
*/
private ManagedDatabaseStatus status;
/*
* Creation date of the database.
*/
private OffsetDateTime creationDate;
/*
* Earliest restore point in time for point in time restore.
*/
private OffsetDateTime earliestRestorePoint;
/*
* Conditional. If createMode is PointInTimeRestore, this value is required. Specifies the point in time (ISO8601
* format) of the source database that will be restored to create the new database.
*/
private OffsetDateTime restorePointInTime;
/*
* Geo paired region.
*/
private String defaultSecondaryLocation;
/*
* Collation of the metadata catalog.
*/
private CatalogCollationType catalogCollation;
/*
* Managed database create mode. PointInTimeRestore: Create a database by restoring a point in time backup of an
* existing database. SourceDatabaseName, SourceManagedInstanceName and PointInTime must be specified.
* RestoreExternalBackup: Create a database by restoring from external backup files. Collation, StorageContainerUri
* and StorageContainerSasToken must be specified. Recovery: Creates a database by restoring a geo-replicated
* backup. RecoverableDatabaseId must be specified as the recoverable database resource ID to restore.
* RestoreLongTermRetentionBackup: Create a database by restoring from a long term retention backup
* (longTermRetentionBackupResourceId required).
*/
private ManagedDatabaseCreateMode createMode;
/*
* Conditional. If createMode is RestoreExternalBackup, this value is required. Specifies the uri of the storage
* container where backups for this restore are stored.
*/
private String storageContainerUri;
/*
* The resource identifier of the source database associated with create operation of this database.
*/
private String sourceDatabaseId;
/*
* The restorable dropped database resource id to restore when creating this database.
*/
private String restorableDroppedDatabaseId;
/*
* Conditional. If createMode is RestoreExternalBackup, this value is required. Specifies the storage container sas
* token.
*/
private String storageContainerSasToken;
/*
* Instance Failover Group resource identifier that this managed database belongs to.
*/
private String failoverGroupId;
/*
* The resource identifier of the recoverable database associated with create operation of this database.
*/
private String recoverableDatabaseId;
/*
* The name of the Long Term Retention backup to be used for restore of this managed database.
*/
private String longTermRetentionBackupResourceId;
/*
* Whether to auto complete restore of this managed database.
*/
private Boolean autoCompleteRestore;
/*
* Last backup file name for restore of this managed database.
*/
private String lastBackupName;
/**
* Creates an instance of ManagedDatabaseProperties class.
*/
public ManagedDatabaseProperties() {
}
/**
* Get the collation property: Collation of the managed database.
*
* @return the collation value.
*/
public String collation() {
return this.collation;
}
/**
* Set the collation property: Collation of the managed database.
*
* @param collation the collation value to set.
* @return the ManagedDatabaseProperties object itself.
*/
public ManagedDatabaseProperties withCollation(String collation) {
this.collation = collation;
return this;
}
/**
* Get the status property: Status of the database.
*
* @return the status value.
*/
public ManagedDatabaseStatus status() {
return this.status;
}
/**
* Get the creationDate property: Creation date of the database.
*
* @return the creationDate value.
*/
public OffsetDateTime creationDate() {
return this.creationDate;
}
/**
* Get the earliestRestorePoint property: Earliest restore point in time for point in time restore.
*
* @return the earliestRestorePoint value.
*/
public OffsetDateTime earliestRestorePoint() {
return this.earliestRestorePoint;
}
/**
* Get the restorePointInTime property: Conditional. If createMode is PointInTimeRestore, this value is required.
* Specifies the point in time (ISO8601 format) of the source database that will be restored to create the new
* database.
*
* @return the restorePointInTime value.
*/
public OffsetDateTime restorePointInTime() {
return this.restorePointInTime;
}
/**
* Set the restorePointInTime property: Conditional. If createMode is PointInTimeRestore, this value is required.
* Specifies the point in time (ISO8601 format) of the source database that will be restored to create the new
* database.
*
* @param restorePointInTime the restorePointInTime value to set.
* @return the ManagedDatabaseProperties object itself.
*/
public ManagedDatabaseProperties withRestorePointInTime(OffsetDateTime restorePointInTime) {
this.restorePointInTime = restorePointInTime;
return this;
}
/**
* Get the defaultSecondaryLocation property: Geo paired region.
*
* @return the defaultSecondaryLocation value.
*/
public String defaultSecondaryLocation() {
return this.defaultSecondaryLocation;
}
/**
* Get the catalogCollation property: Collation of the metadata catalog.
*
* @return the catalogCollation value.
*/
public CatalogCollationType catalogCollation() {
return this.catalogCollation;
}
/**
* Set the catalogCollation property: Collation of the metadata catalog.
*
* @param catalogCollation the catalogCollation value to set.
* @return the ManagedDatabaseProperties object itself.
*/
public ManagedDatabaseProperties withCatalogCollation(CatalogCollationType catalogCollation) {
this.catalogCollation = catalogCollation;
return this;
}
/**
* Get the createMode property: Managed database create mode. PointInTimeRestore: Create a database by restoring a
* point in time backup of an existing database. SourceDatabaseName, SourceManagedInstanceName and PointInTime must
* be specified. RestoreExternalBackup: Create a database by restoring from external backup files. Collation,
* StorageContainerUri and StorageContainerSasToken must be specified. Recovery: Creates a database by restoring a
* geo-replicated backup. RecoverableDatabaseId must be specified as the recoverable database resource ID to
* restore. RestoreLongTermRetentionBackup: Create a database by restoring from a long term retention backup
* (longTermRetentionBackupResourceId required).
*
* @return the createMode value.
*/
public ManagedDatabaseCreateMode createMode() {
return this.createMode;
}
/**
* Set the createMode property: Managed database create mode. PointInTimeRestore: Create a database by restoring a
* point in time backup of an existing database. SourceDatabaseName, SourceManagedInstanceName and PointInTime must
* be specified. RestoreExternalBackup: Create a database by restoring from external backup files. Collation,
* StorageContainerUri and StorageContainerSasToken must be specified. Recovery: Creates a database by restoring a
* geo-replicated backup. RecoverableDatabaseId must be specified as the recoverable database resource ID to
* restore. RestoreLongTermRetentionBackup: Create a database by restoring from a long term retention backup
* (longTermRetentionBackupResourceId required).
*
* @param createMode the createMode value to set.
* @return the ManagedDatabaseProperties object itself.
*/
public ManagedDatabaseProperties withCreateMode(ManagedDatabaseCreateMode createMode) {
this.createMode = createMode;
return this;
}
/**
* Get the storageContainerUri property: Conditional. If createMode is RestoreExternalBackup, this value is
* required. Specifies the uri of the storage container where backups for this restore are stored.
*
* @return the storageContainerUri value.
*/
public String storageContainerUri() {
return this.storageContainerUri;
}
/**
* Set the storageContainerUri property: Conditional. If createMode is RestoreExternalBackup, this value is
* required. Specifies the uri of the storage container where backups for this restore are stored.
*
* @param storageContainerUri the storageContainerUri value to set.
* @return the ManagedDatabaseProperties object itself.
*/
public ManagedDatabaseProperties withStorageContainerUri(String storageContainerUri) {
this.storageContainerUri = storageContainerUri;
return this;
}
/**
* Get the sourceDatabaseId property: The resource identifier of the source database associated with create
* operation of this database.
*
* @return the sourceDatabaseId value.
*/
public String sourceDatabaseId() {
return this.sourceDatabaseId;
}
/**
* Set the sourceDatabaseId property: The resource identifier of the source database associated with create
* operation of this database.
*
* @param sourceDatabaseId the sourceDatabaseId value to set.
* @return the ManagedDatabaseProperties object itself.
*/
public ManagedDatabaseProperties withSourceDatabaseId(String sourceDatabaseId) {
this.sourceDatabaseId = sourceDatabaseId;
return this;
}
/**
* Get the restorableDroppedDatabaseId property: The restorable dropped database resource id to restore when
* creating this database.
*
* @return the restorableDroppedDatabaseId value.
*/
public String restorableDroppedDatabaseId() {
return this.restorableDroppedDatabaseId;
}
/**
* Set the restorableDroppedDatabaseId property: The restorable dropped database resource id to restore when
* creating this database.
*
* @param restorableDroppedDatabaseId the restorableDroppedDatabaseId value to set.
* @return the ManagedDatabaseProperties object itself.
*/
public ManagedDatabaseProperties withRestorableDroppedDatabaseId(String restorableDroppedDatabaseId) {
this.restorableDroppedDatabaseId = restorableDroppedDatabaseId;
return this;
}
/**
* Get the storageContainerSasToken property: Conditional. If createMode is RestoreExternalBackup, this value is
* required. Specifies the storage container sas token.
*
* @return the storageContainerSasToken value.
*/
public String storageContainerSasToken() {
return this.storageContainerSasToken;
}
/**
* Set the storageContainerSasToken property: Conditional. If createMode is RestoreExternalBackup, this value is
* required. Specifies the storage container sas token.
*
* @param storageContainerSasToken the storageContainerSasToken value to set.
* @return the ManagedDatabaseProperties object itself.
*/
public ManagedDatabaseProperties withStorageContainerSasToken(String storageContainerSasToken) {
this.storageContainerSasToken = storageContainerSasToken;
return this;
}
/**
* Get the failoverGroupId property: Instance Failover Group resource identifier that this managed database belongs
* to.
*
* @return the failoverGroupId value.
*/
public String failoverGroupId() {
return this.failoverGroupId;
}
/**
* Get the recoverableDatabaseId property: The resource identifier of the recoverable database associated with
* create operation of this database.
*
* @return the recoverableDatabaseId value.
*/
public String recoverableDatabaseId() {
return this.recoverableDatabaseId;
}
/**
* Set the recoverableDatabaseId property: The resource identifier of the recoverable database associated with
* create operation of this database.
*
* @param recoverableDatabaseId the recoverableDatabaseId value to set.
* @return the ManagedDatabaseProperties object itself.
*/
public ManagedDatabaseProperties withRecoverableDatabaseId(String recoverableDatabaseId) {
this.recoverableDatabaseId = recoverableDatabaseId;
return this;
}
/**
* Get the longTermRetentionBackupResourceId property: The name of the Long Term Retention backup to be used for
* restore of this managed database.
*
* @return the longTermRetentionBackupResourceId value.
*/
public String longTermRetentionBackupResourceId() {
return this.longTermRetentionBackupResourceId;
}
/**
* Set the longTermRetentionBackupResourceId property: The name of the Long Term Retention backup to be used for
* restore of this managed database.
*
* @param longTermRetentionBackupResourceId the longTermRetentionBackupResourceId value to set.
* @return the ManagedDatabaseProperties object itself.
*/
public ManagedDatabaseProperties withLongTermRetentionBackupResourceId(String longTermRetentionBackupResourceId) {
this.longTermRetentionBackupResourceId = longTermRetentionBackupResourceId;
return this;
}
/**
* Get the autoCompleteRestore property: Whether to auto complete restore of this managed database.
*
* @return the autoCompleteRestore value.
*/
public Boolean autoCompleteRestore() {
return this.autoCompleteRestore;
}
/**
* Set the autoCompleteRestore property: Whether to auto complete restore of this managed database.
*
* @param autoCompleteRestore the autoCompleteRestore value to set.
* @return the ManagedDatabaseProperties object itself.
*/
public ManagedDatabaseProperties withAutoCompleteRestore(Boolean autoCompleteRestore) {
this.autoCompleteRestore = autoCompleteRestore;
return this;
}
/**
* Get the lastBackupName property: Last backup file name for restore of this managed database.
*
* @return the lastBackupName value.
*/
public String lastBackupName() {
return this.lastBackupName;
}
/**
* Set the lastBackupName property: Last backup file name for restore of this managed database.
*
* @param lastBackupName the lastBackupName value to set.
* @return the ManagedDatabaseProperties object itself.
*/
public ManagedDatabaseProperties withLastBackupName(String lastBackupName) {
this.lastBackupName = lastBackupName;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("collation", this.collation);
jsonWriter.writeStringField("restorePointInTime",
this.restorePointInTime == null
? null
: DateTimeFormatter.ISO_OFFSET_DATE_TIME.format(this.restorePointInTime));
jsonWriter.writeStringField("catalogCollation",
this.catalogCollation == null ? null : this.catalogCollation.toString());
jsonWriter.writeStringField("createMode", this.createMode == null ? null : this.createMode.toString());
jsonWriter.writeStringField("storageContainerUri", this.storageContainerUri);
jsonWriter.writeStringField("sourceDatabaseId", this.sourceDatabaseId);
jsonWriter.writeStringField("restorableDroppedDatabaseId", this.restorableDroppedDatabaseId);
jsonWriter.writeStringField("storageContainerSasToken", this.storageContainerSasToken);
jsonWriter.writeStringField("recoverableDatabaseId", this.recoverableDatabaseId);
jsonWriter.writeStringField("longTermRetentionBackupResourceId", this.longTermRetentionBackupResourceId);
jsonWriter.writeBooleanField("autoCompleteRestore", this.autoCompleteRestore);
jsonWriter.writeStringField("lastBackupName", this.lastBackupName);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of ManagedDatabaseProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of ManagedDatabaseProperties if the JsonReader was pointing to an instance of it, or null if
* it was pointing to JSON null.
* @throws IOException If an error occurs while reading the ManagedDatabaseProperties.
*/
public static ManagedDatabaseProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
ManagedDatabaseProperties deserializedManagedDatabaseProperties = new ManagedDatabaseProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("collation".equals(fieldName)) {
deserializedManagedDatabaseProperties.collation = reader.getString();
} else if ("status".equals(fieldName)) {
deserializedManagedDatabaseProperties.status = ManagedDatabaseStatus.fromString(reader.getString());
} else if ("creationDate".equals(fieldName)) {
deserializedManagedDatabaseProperties.creationDate = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("earliestRestorePoint".equals(fieldName)) {
deserializedManagedDatabaseProperties.earliestRestorePoint = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("restorePointInTime".equals(fieldName)) {
deserializedManagedDatabaseProperties.restorePointInTime = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("defaultSecondaryLocation".equals(fieldName)) {
deserializedManagedDatabaseProperties.defaultSecondaryLocation = reader.getString();
} else if ("catalogCollation".equals(fieldName)) {
deserializedManagedDatabaseProperties.catalogCollation
= CatalogCollationType.fromString(reader.getString());
} else if ("createMode".equals(fieldName)) {
deserializedManagedDatabaseProperties.createMode
= ManagedDatabaseCreateMode.fromString(reader.getString());
} else if ("storageContainerUri".equals(fieldName)) {
deserializedManagedDatabaseProperties.storageContainerUri = reader.getString();
} else if ("sourceDatabaseId".equals(fieldName)) {
deserializedManagedDatabaseProperties.sourceDatabaseId = reader.getString();
} else if ("restorableDroppedDatabaseId".equals(fieldName)) {
deserializedManagedDatabaseProperties.restorableDroppedDatabaseId = reader.getString();
} else if ("storageContainerSasToken".equals(fieldName)) {
deserializedManagedDatabaseProperties.storageContainerSasToken = reader.getString();
} else if ("failoverGroupId".equals(fieldName)) {
deserializedManagedDatabaseProperties.failoverGroupId = reader.getString();
} else if ("recoverableDatabaseId".equals(fieldName)) {
deserializedManagedDatabaseProperties.recoverableDatabaseId = reader.getString();
} else if ("longTermRetentionBackupResourceId".equals(fieldName)) {
deserializedManagedDatabaseProperties.longTermRetentionBackupResourceId = reader.getString();
} else if ("autoCompleteRestore".equals(fieldName)) {
deserializedManagedDatabaseProperties.autoCompleteRestore
= reader.getNullable(JsonReader::getBoolean);
} else if ("lastBackupName".equals(fieldName)) {
deserializedManagedDatabaseProperties.lastBackupName = reader.getString();
} else {
reader.skipChildren();
}
}
return deserializedManagedDatabaseProperties;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy