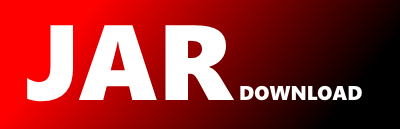
com.azure.resourcemanager.sql.fluent.models.ManagedDatabaseRestoreDetailsProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-sql Show documentation
Show all versions of azure-resourcemanager-sql Show documentation
This package contains Microsoft Azure Sql Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.sql.fluent.models;
import com.azure.core.annotation.Immutable;
import com.azure.core.util.CoreUtils;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.time.OffsetDateTime;
import java.util.List;
/**
* The managed database's restore details properties.
*/
@Immutable
public final class ManagedDatabaseRestoreDetailsProperties
implements JsonSerializable {
/*
* Restore status.
*/
private String status;
/*
* Current restoring file name.
*/
private String currentRestoringFileName;
/*
* Last restored file name.
*/
private String lastRestoredFileName;
/*
* Last restored file time.
*/
private OffsetDateTime lastRestoredFileTime;
/*
* Percent completed.
*/
private Double percentCompleted;
/*
* List of unrestorable files.
*/
private List unrestorableFiles;
/*
* Number of files detected.
*/
private Long numberOfFilesDetected;
/*
* Last uploaded file name.
*/
private String lastUploadedFileName;
/*
* Last uploaded file time.
*/
private OffsetDateTime lastUploadedFileTime;
/*
* The reason why restore is in Blocked state.
*/
private String blockReason;
/**
* Creates an instance of ManagedDatabaseRestoreDetailsProperties class.
*/
public ManagedDatabaseRestoreDetailsProperties() {
}
/**
* Get the status property: Restore status.
*
* @return the status value.
*/
public String status() {
return this.status;
}
/**
* Get the currentRestoringFileName property: Current restoring file name.
*
* @return the currentRestoringFileName value.
*/
public String currentRestoringFileName() {
return this.currentRestoringFileName;
}
/**
* Get the lastRestoredFileName property: Last restored file name.
*
* @return the lastRestoredFileName value.
*/
public String lastRestoredFileName() {
return this.lastRestoredFileName;
}
/**
* Get the lastRestoredFileTime property: Last restored file time.
*
* @return the lastRestoredFileTime value.
*/
public OffsetDateTime lastRestoredFileTime() {
return this.lastRestoredFileTime;
}
/**
* Get the percentCompleted property: Percent completed.
*
* @return the percentCompleted value.
*/
public Double percentCompleted() {
return this.percentCompleted;
}
/**
* Get the unrestorableFiles property: List of unrestorable files.
*
* @return the unrestorableFiles value.
*/
public List unrestorableFiles() {
return this.unrestorableFiles;
}
/**
* Get the numberOfFilesDetected property: Number of files detected.
*
* @return the numberOfFilesDetected value.
*/
public Long numberOfFilesDetected() {
return this.numberOfFilesDetected;
}
/**
* Get the lastUploadedFileName property: Last uploaded file name.
*
* @return the lastUploadedFileName value.
*/
public String lastUploadedFileName() {
return this.lastUploadedFileName;
}
/**
* Get the lastUploadedFileTime property: Last uploaded file time.
*
* @return the lastUploadedFileTime value.
*/
public OffsetDateTime lastUploadedFileTime() {
return this.lastUploadedFileTime;
}
/**
* Get the blockReason property: The reason why restore is in Blocked state.
*
* @return the blockReason value.
*/
public String blockReason() {
return this.blockReason;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of ManagedDatabaseRestoreDetailsProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of ManagedDatabaseRestoreDetailsProperties if the JsonReader was pointing to an instance of
* it, or null if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the ManagedDatabaseRestoreDetailsProperties.
*/
public static ManagedDatabaseRestoreDetailsProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
ManagedDatabaseRestoreDetailsProperties deserializedManagedDatabaseRestoreDetailsProperties
= new ManagedDatabaseRestoreDetailsProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("status".equals(fieldName)) {
deserializedManagedDatabaseRestoreDetailsProperties.status = reader.getString();
} else if ("currentRestoringFileName".equals(fieldName)) {
deserializedManagedDatabaseRestoreDetailsProperties.currentRestoringFileName = reader.getString();
} else if ("lastRestoredFileName".equals(fieldName)) {
deserializedManagedDatabaseRestoreDetailsProperties.lastRestoredFileName = reader.getString();
} else if ("lastRestoredFileTime".equals(fieldName)) {
deserializedManagedDatabaseRestoreDetailsProperties.lastRestoredFileTime = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("percentCompleted".equals(fieldName)) {
deserializedManagedDatabaseRestoreDetailsProperties.percentCompleted
= reader.getNullable(JsonReader::getDouble);
} else if ("unrestorableFiles".equals(fieldName)) {
List unrestorableFiles = reader.readArray(reader1 -> reader1.getString());
deserializedManagedDatabaseRestoreDetailsProperties.unrestorableFiles = unrestorableFiles;
} else if ("numberOfFilesDetected".equals(fieldName)) {
deserializedManagedDatabaseRestoreDetailsProperties.numberOfFilesDetected
= reader.getNullable(JsonReader::getLong);
} else if ("lastUploadedFileName".equals(fieldName)) {
deserializedManagedDatabaseRestoreDetailsProperties.lastUploadedFileName = reader.getString();
} else if ("lastUploadedFileTime".equals(fieldName)) {
deserializedManagedDatabaseRestoreDetailsProperties.lastUploadedFileTime = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("blockReason".equals(fieldName)) {
deserializedManagedDatabaseRestoreDetailsProperties.blockReason = reader.getString();
} else {
reader.skipChildren();
}
}
return deserializedManagedDatabaseRestoreDetailsProperties;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy